A C++ crash course quickly equips learners with essential C++ commands through practical examples and hands-on coding practice.
Here's a simple code snippet demonstrating a basic "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Getting Started with C++
Setting Up the Environment
Choosing an IDE (Integrated Development Environment) is crucial for a smooth coding experience. Popular options include Visual Studio, Code::Blocks, and CLion. Each IDE comes with unique features that can enhance productivity, such as code completion and debugging tools.
To get started, download the IDE of your choice and follow the installation steps. Configuration might involve setting up paths for your C++ compiler, which we will discuss next.
Installing a C++ Compiler is essential for translating your code into executable programs. On Linux, the G++ compiler can be installed with package managers, while Windows users might prefer MinGW. Mac users often use the built-in Xcode tools. Understanding how compilers work will deepen your knowledge. A compiler translates your high-level C++ code into machine code, allowing your programs to run on your computer.
Your First C++ Program
Let's dive into the basic structure of a C++ program. Every C++ program includes `#include` directives which allow access to libraries, and it always contains a `main()` function, the entry point of execution.
Here is a simple example of a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This code prints "Hello, World!" to the console. You can compile and run this program by typing the appropriate commands into your terminal or through your IDE.
Common Errors and Debugging Tips include recognizing syntax errors, which occur when rules of the language are violated, and runtime errors that emerge during program execution. Familiarizing yourself with common errors can shorten your debugging time.
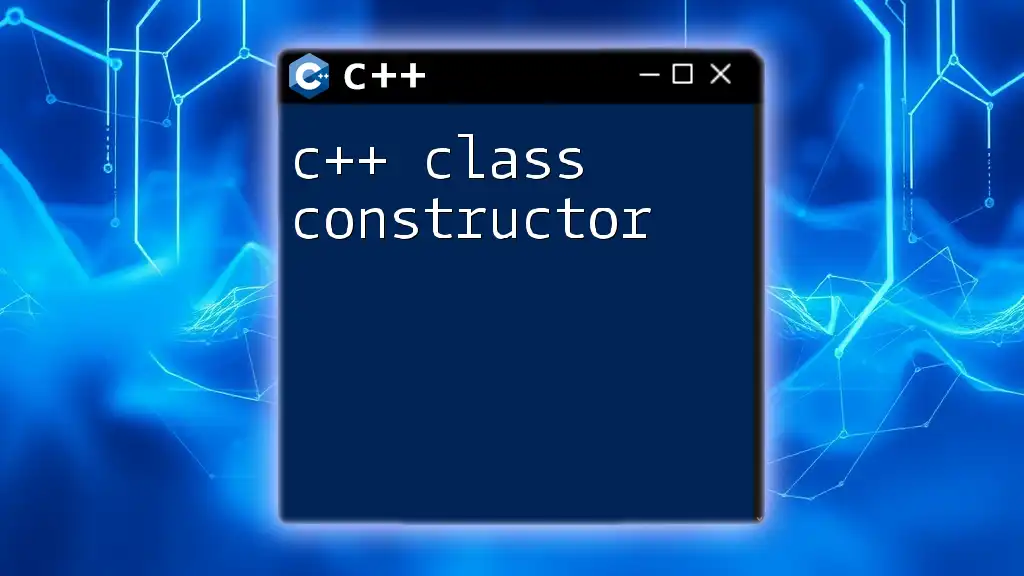
Fundamentals of C++
Data Types and Variables
Understanding data types is crucial for efficient programming. C++ provides a variety of types, including primitive types like `int`, `float`, `char`, and `double`. Each type serves a different purpose and has a specific range of values.
Here’s how you can declare and initialize variables in C++:
int age = 30;
float height = 5.9;
char initial = 'A';
In this example, we declare three variables of different types and assign them values.
Operators and Expressions
C++ provides a rich set of arithmetic operators to perform calculations:
- `+` for addition
- `-` for subtraction
- `*` for multiplication
- `/` for division
- `%` for modulus
In addition, relational and logical operators allow for comparison and logical operations:
- `==` checks for equality
- `!=` checks for inequality
- `>` checks if one value is greater than another
Here's how you can use these in code:
int a = 10;
int b = 20;
bool isGreater = (a > b); // returns false
Control Structures
C++ allows for conditional statements to control the flow of execution based on certain conditions. The most common are `if`, `if-else`, and `switch`.
Example using `if-else`:
int number = 10;
if (number > 0) {
std::cout << "Positive number" << std::endl;
} else {
std::cout << "Negative number" << std::endl;
}
Loops are used to execute a block of code multiple times. For instance, the `for` loop can repeat a specific action:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
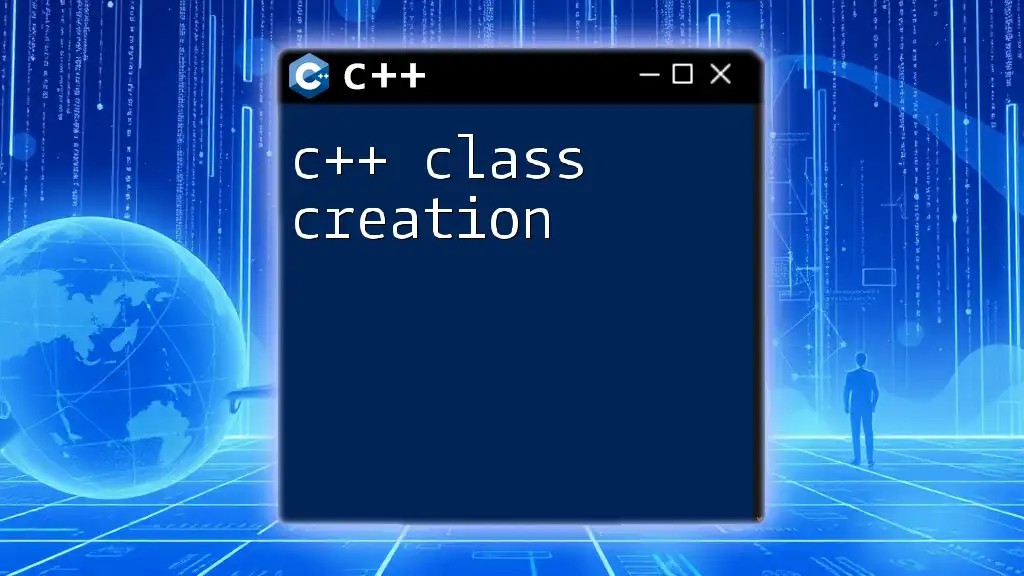
Functions in C++
Function Basics
A function in C++ groups instructions together and can return a value. Here’s how you would define a function:
int add(int a, int b) {
return a + b;
}
This function takes two integers, `a` and `b`, and returns their sum.
Scope and Lifetime
Understanding scope is important in controlling the accessibility of variables throughout your program. Local variables are accessible only within the function they are defined in, while global variables can be accessed throughout the entire program. The lifetime of a variable refers to how long it exists in memory.
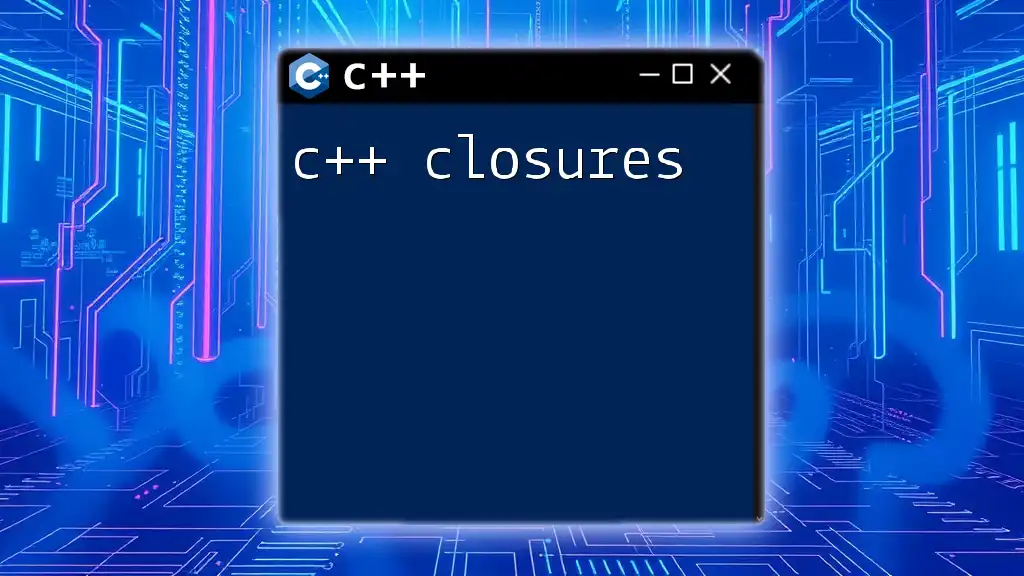
Object-Oriented Programming (OOP) in C++
Understanding OOP Concepts
C++ is a powerful object-oriented programming language that allows for encapsulation, inheritance, and polymorphism.
Classes and Objects are foundational to OOP. Classes define blueprints from which objects (instances of classes) are created. Here’s a simple class example:
class Animal {
public:
void speak() {
std::cout << "Animal speaks!" << std::endl;
}
};
In this case, `Animal` is a class that has a method `speak` which outputs a message.
Encapsulation restricts access to certain details of an object by using access modifiers like `public`, `private`, and `protected`.
Inheritance allows a new class (derived class) to inherit attributes and methods from an existing class (base class). Here’s an example:
class Dog : public Animal {
public:
void speak() {
std::cout << "Dog barks!" << std::endl;
}
};
Polymorphism allows for methods to be used in different contexts. With C++, you can achieve this through both compile-time (function overloading) and run-time (function overriding) polymorphism.
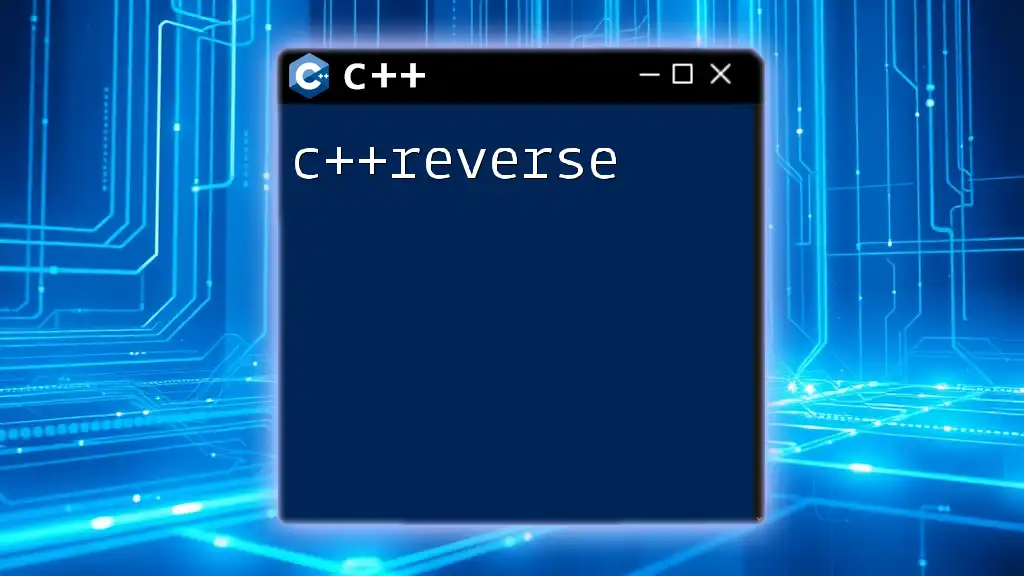
Advanced Topics
Pointers and Memory Management
Pointers are variables that hold memory addresses, allowing for dynamic memory management:
int* ptr = new int(5);
std::cout << *ptr << std::endl; // Outputs: 5
delete ptr; // Freeing the memory
This code snippet dynamically allocates an integer, assigns it the value `5`, and later frees that memory.
Standard Template Library (STL)
The Standard Template Library (STL) is a powerful library that provides ready-to-use templates for data structures like vectors, lists, and maps.
Containers are used to store collections of data. For example, here is how to use a vector:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4};
for (int num : numbers) {
std::cout << num << std::endl;
}
Iterators provide a means to traverse the elements of containers, simplifying access.
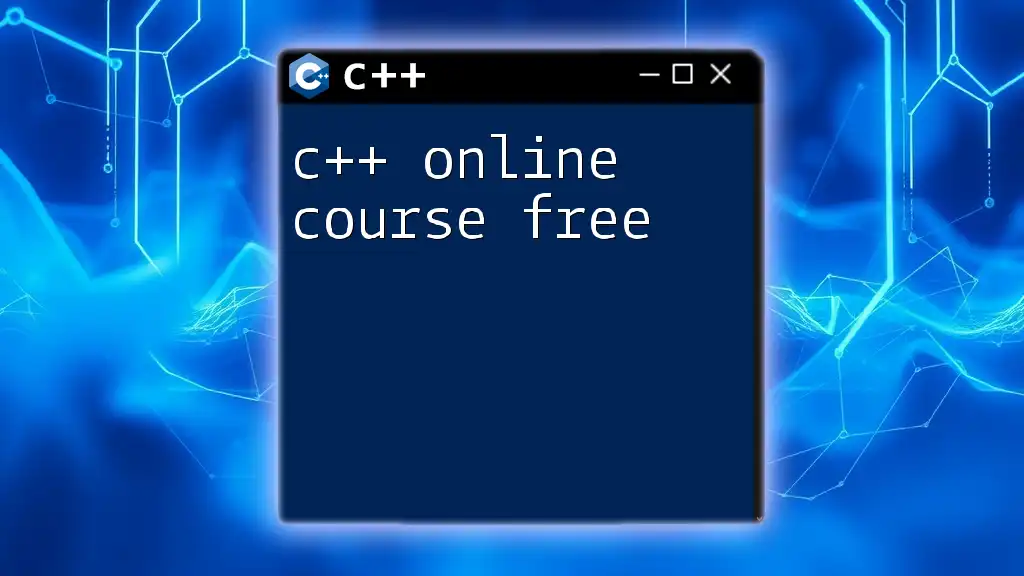
Best Practices and Learning Resources
Writing Clean Code
Code readability is vital for ensuring your code can be understood by yourself and others. Use meaningful variable names and include comments to explain complex sections.
Where to Go from Here
Once comfortable with the basics, consider exploring further learning resources. Online platforms like Coursera, Udacity, and freeCodeCamp offer comprehensive C++ courses that take you deeper into advanced topics.
Practice platforms such as LeetCode, HackerRank, and Codecademy offer challenges that allow you to apply what you've learned in practical situations—essential for honing your skills.
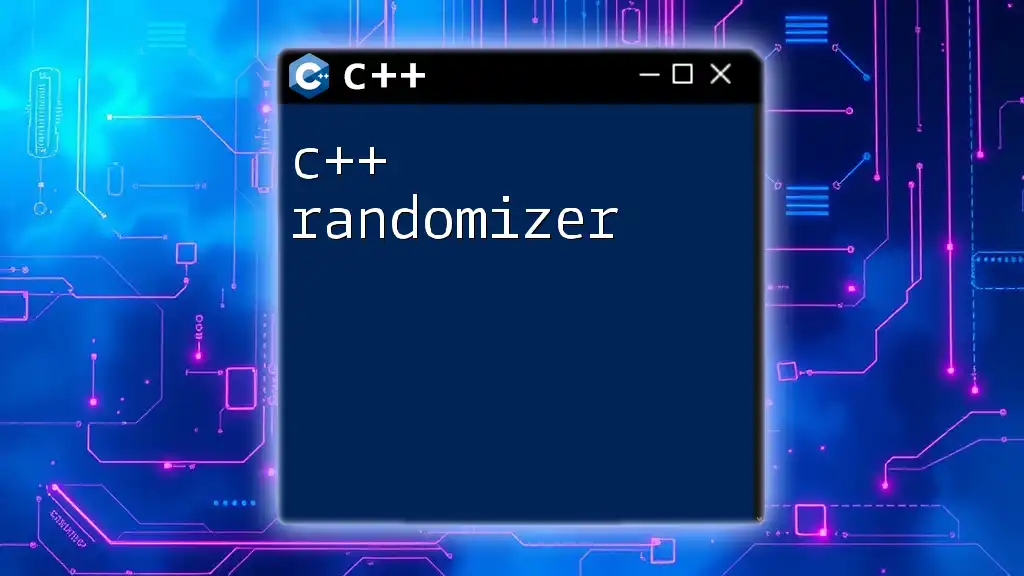
Conclusion
C++ is a versatile and powerful language that forms the backbone of many applications, from systems programming to game development. By mastering the fundamentals and applying best practices, you will set yourself up for success in your programming journey. Remember to keep exploring and practicing, and don't hesitate to seek additional resources to enhance your learning in this captivating field.
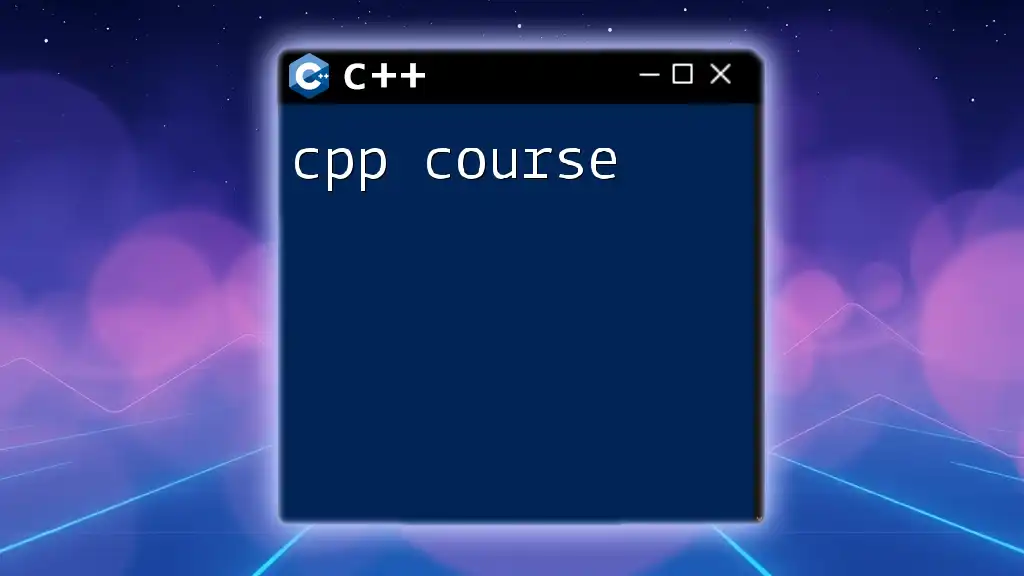
FAQs
How long does it take to learn C++? The timeline varies by individual. With dedicated practice, foundational understanding can be achieved in a few months.
What projects should I try first? Start with small projects such as calculator applications, simple games, or text-based interfaces to strengthen your grasp of the language.
Dive into this C++ crash course and unlock the potential to become a proficient programmer!