C++ closures are functions that capture the local variables from their surrounding scope, allowing them to maintain state even when called outside their original context.
#include <iostream>
#include <functional>
int main() {
int x = 10;
auto closure = [&]() { return x + 5; }; // captures x by reference
std::cout << closure() << std::endl; // Output: 15
return 0;
}
Understanding Closures in C++
What is a Closure?
A closure in C++ is a powerful and convenient feature that enables the creation of function objects which capture and retain the context in which they were defined. This includes not only the function parameters but also local variables. Closures allow developers to write inline functions that can operate with variable contexts without the boilerplate of explicitly managing the state.
The Importance of Closures
Why should you use closures in C++? Here are a few compelling reasons:
- Encapsulate behavior and state: Closures package both function logic and their environment, making your code more modular and easier to refactor.
- Create callbacks and event handlers: In modern C++ development, particularly in GUI applications or asynchronous programming, closures allow for a streamlined approach to defining actions tied to events.
- Simplify complex code: Rather than creating separate named functions for simple operations, closures allow you to express functionality inline, making the code more compact and easier to read.
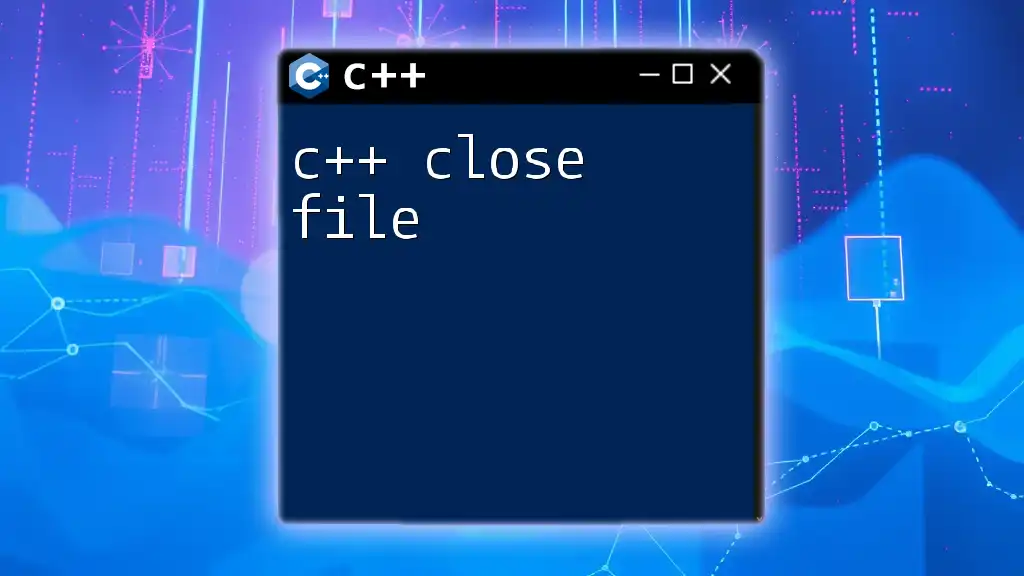
The Anatomy of a C++ Closure
Syntax of a Closure
In C++, closures are often defined using lambda expressions, a feature introduced in C++11. A lambda expression provides a concise and intuitive way to create closures in your code.
Example:
auto closure = [](int x) { return x * x; };
In this example, `closure` is a lambda that takes an integer `x` and returns its square. This function can be invoked with different values of `x`, retaining its behavior regardless of context.
Capturing Variables
One of the most defining characteristics of closures is how they handle variable capture. C++ provides two main capture modes:
-
Capture by Value (`[=]`): When a closure captures variables by value, it creates a copy of the variables, allowing you to work with their values independently of the original variables.
-
Capture by Reference (`[&]`): When a closure captures variables by reference, it retains a link to the original variables. This means changes made to the variables in the enclosing function are reflected inside the closure.
Example:
int a = 10;
auto byValue = [=]() { return a + 5; }; // Captures by value
auto byReference = [&]() { return a + 5; }; // Captures by reference
Here, `byValue` uses the value of `a` at the time it was defined, while `byReference` will always reflect the current value of `a`, allowing updates outside the closure to affect its output.
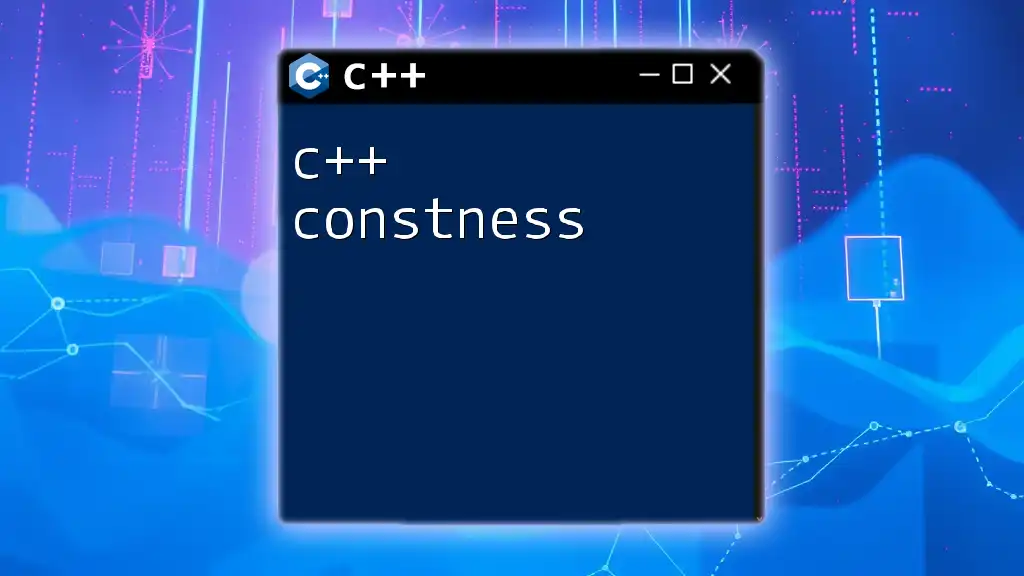
Creating and Using Closures in Functions
Defining a Standard Function with a Closure
Closures can be extremely useful as parameters to functions. By defining a function that accepts a closure, you can customize its behavior dynamically.
Example:
#include <iostream>
#include <functional>
void applyFunction(const std::function<int(int)>& func, int number) {
std::cout << "Result: " << func(number) << std::endl;
}
int main() {
auto doubler = [](int x) { return x * 2; };
applyFunction(doubler, 5); // Output: Result: 10
}
In this code, a function `applyFunction` takes a closure as a parameter. When `doubler` is passed in, it applies the provided logic to compute and print `10`.
Closures in STL Algorithms
The Standard Template Library (STL) in C++ provides numerous algorithms that work seamlessly with closures, enhancing their usability even more.
Example:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::for_each(numbers.begin(), numbers.end(), [](int n) {
std::cout << n * 2 << " ";
});
}
In this example, `std::for_each` is employed to iterate through the `numbers` vector. The inline closure doubles each number and prints the result, illustrating a concise and effective use of closures in C++.
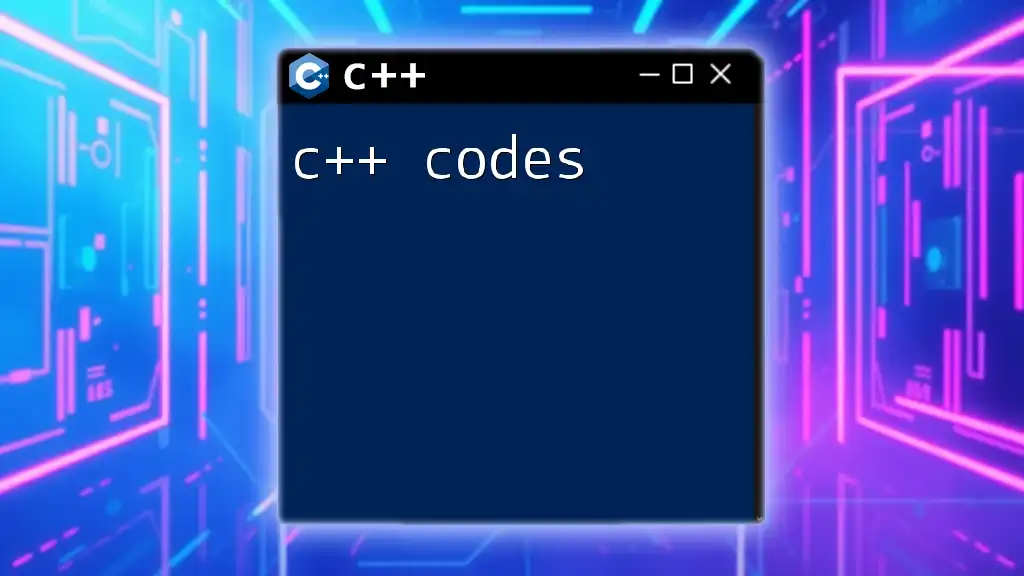
Advanced Closure Concepts
Mutable Lambdas
Closures created with lambda expressions can be modified using the `mutable` keyword, which allows the internal state of captured variables to be changed even if they are captured by value.
Example:
#include <iostream>
int main() {
int count = 0;
auto incrementer = [count]() mutable {
return ++count;
};
// Calling incrementer doesn't change the outer count
std::cout << incrementer() << std::endl; // Outputs: 1
std::cout << incrementer() << std::endl; // Outputs: 2
}
Here, `incrementer` is a closure that increments its copied version of `count` each time it is called, demonstrating how `mutable` allows internal state adjustments without affecting the original variable.
Nested Closures
C++ also allows nesting closures. A closure can be defined within another closure, allowing you to build complex behaviors using context from multiple levels.
Example:
#include <iostream>
int main() {
auto outer = [](int x) {
return [=](int y) { return x + y; };
};
auto addFive = outer(5);
std::cout << addFive(10); // Outputs: 15
}
In this case, `outer` returns a closure that captures the value `x`. When invoked with `y`, it combines both values to produce the result, showcasing the ability to create rich function behaviors within various contexts.
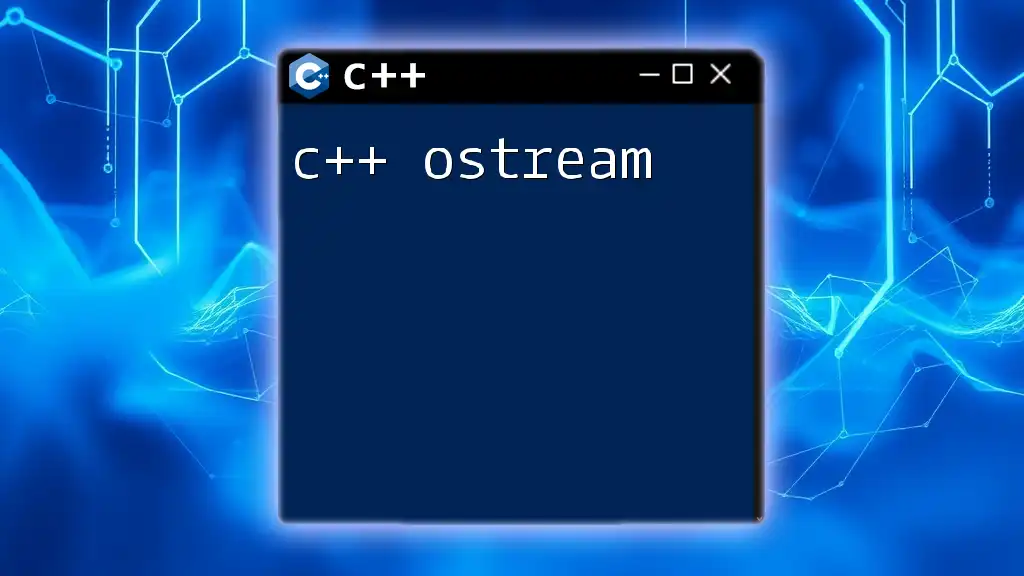
Practical Applications of C++ Closures
Event Handling in GUI Applications
Closures lend themselves especially well to handling events in graphical user interface (GUI) libraries. Instead of creating separate named functions for every event, closures can streamline code, providing better readability and decreased overhead.
Shortening Code with Closures
One of the primary advantages of using closures is the ability to reduce code complexity by enabling the definition of small inline functions. This not only simplifies your code but also allows for greater expressiveness in the way functionality is represented in your program.
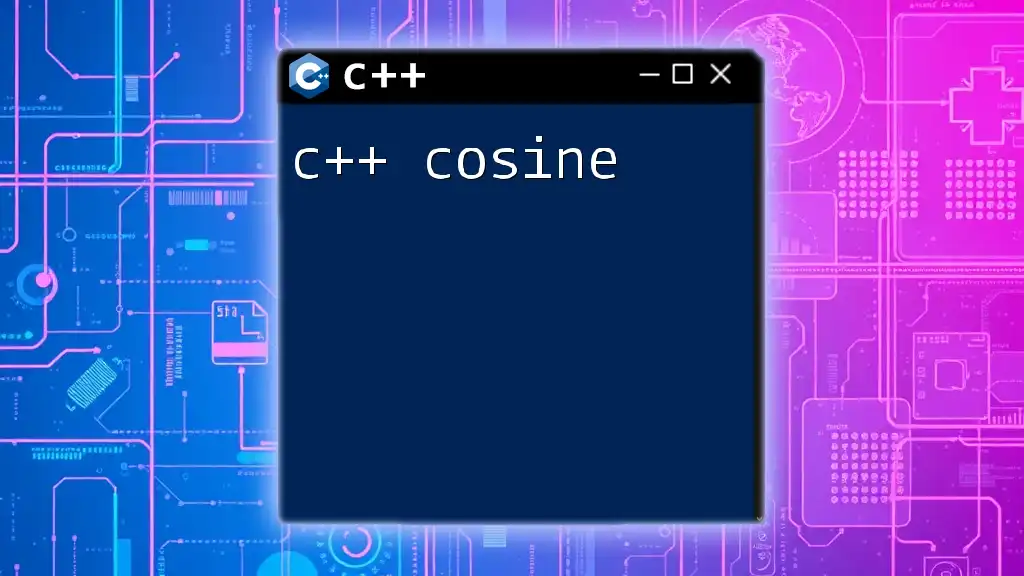
Common Pitfalls and Best Practices
Common Mistakes When Using Closures
One of the common pitfalls developers face when using closures is inadvertently creating dangling references. This often occurs when capturing local variables by reference from a function, leading to undefined behavior once the function scope is exited.
Best Practices
To ensure the effective use of closures, adhere to these best practices:
- Keep closures simple and clear: Function logic should be immediately understandable for maintainability and testing purposes.
- Avoid capturing references unless necessary: When possible, prefer capturing by value to retain a consistent and bug-free state across function calls.
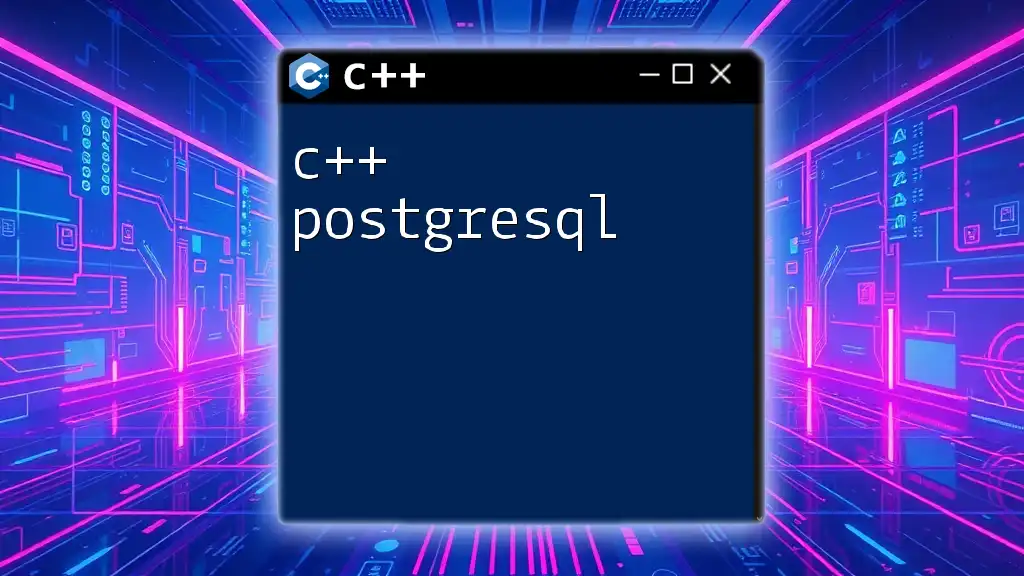
Summary of Key Points
In summary, C++ closures are a sophisticated feature that extends the language's capabilities in functional programming. By allowing functions to capture the context of local variables, closures create opportunities for more elegant, reusable, and concise code structures. The flexible syntax and potential for integration with STL algorithms further streamline development processes, making closures a vital tool in any C++ programmer’s toolkit.
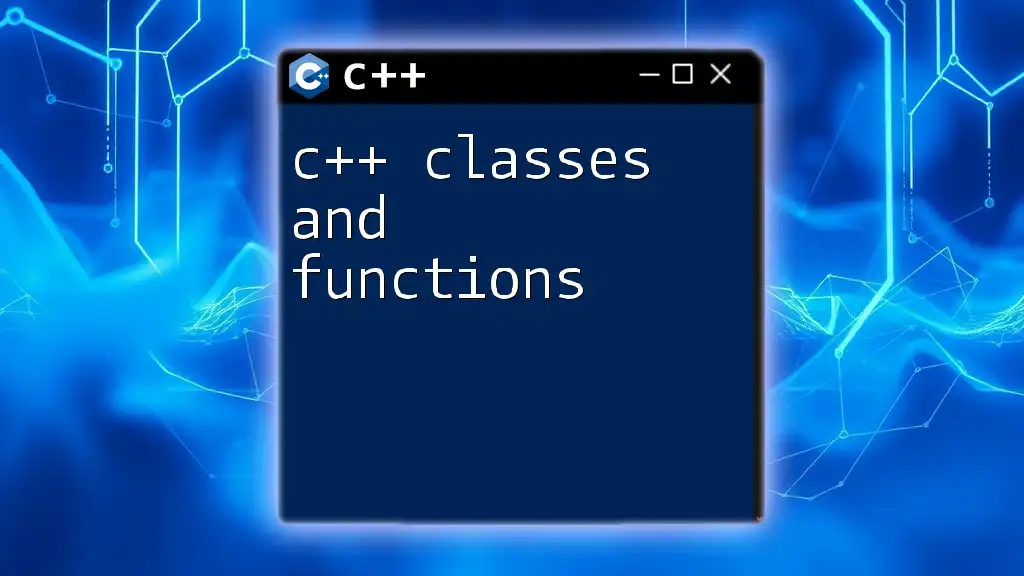
Additional Resources
For further reading and deeper dives into C++ closures, consider exploring C++ documentation, tutorials on functional programming, and advanced articles related to lambda expressions and their applications. These resources can enhance your understanding and enable you to leverage C++ closures effectively in your projects.