In C++, the `close()` function is used to close a file that has been opened with a file stream object, freeing up system resources and ensuring that all buffered output data is written to the file.
Here’s a code snippet demonstrating how to close a file in C++:
#include <fstream>
int main() {
std::ofstream outFile("example.txt"); // Open a file for writing
outFile << "Hello, World!"; // Write to the file
outFile.close(); // Close the file
return 0;
}
Understanding File Streams in C++
What are File Streams?
In C++, file streams refer to the input and output methods used to read and write data to files. They allow programmers to interact with the file system effectively, treating the file as a stream of data. Understanding file streams is essential for efficient file handling in your applications.
Types of File Streams in C++
There are three primary types of file streams in C++:
-
ifstream: This stands for "input file stream," and it is used for reading data from files. When you create an `ifstream`, it opens a file for reading.
-
ofstream: This stands for "output file stream," and it is utilized for writing data to files. An `ofstream` opens a file to enable the programmer to write data.
-
fstream: This is short for "file stream," and it allows both reading and writing to the same file. It enables more complex file operations compared to `ifstream` and `ofstream`.
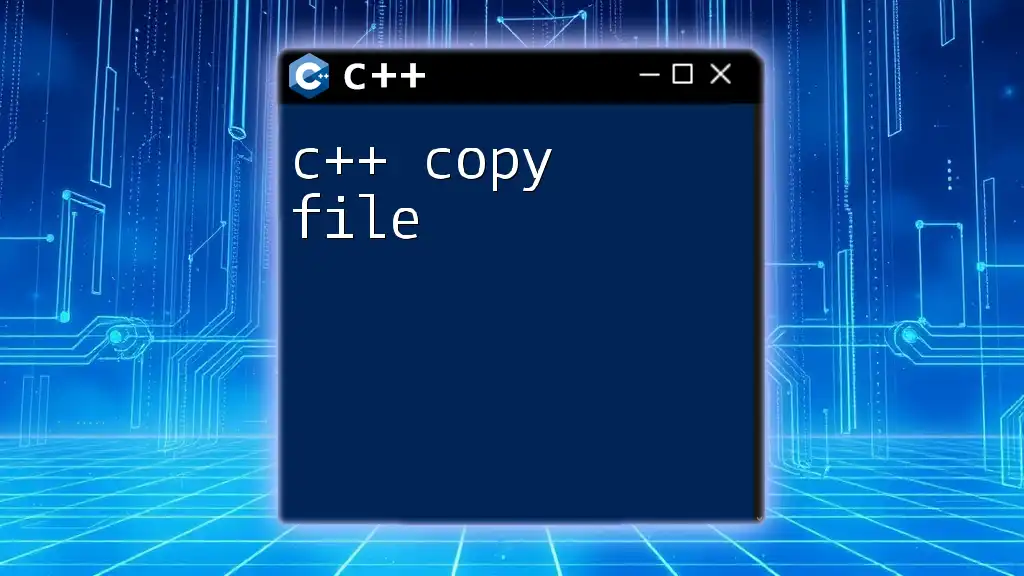
The Importance of Closing Files
Resource Management
Closing files is crucial for resource management. Each open file consumes system resources, including memory and file handles. If you fail to close files, it may lead to resource leaks, which can degrade application performance. Properly managing resources ensures that your application runs smoothly and efficiently.
Data Integrity
One of the primary reasons for closing files is to maintain data integrity. When a file remains open, the changes you make may be stored in temporary buffers rather than actually written to disk. In the event of a program crash or unexpected termination, these buffered changes may be lost, leading to irreversible data loss. Always closing your files guarantees that all data is written and preserved correctly.
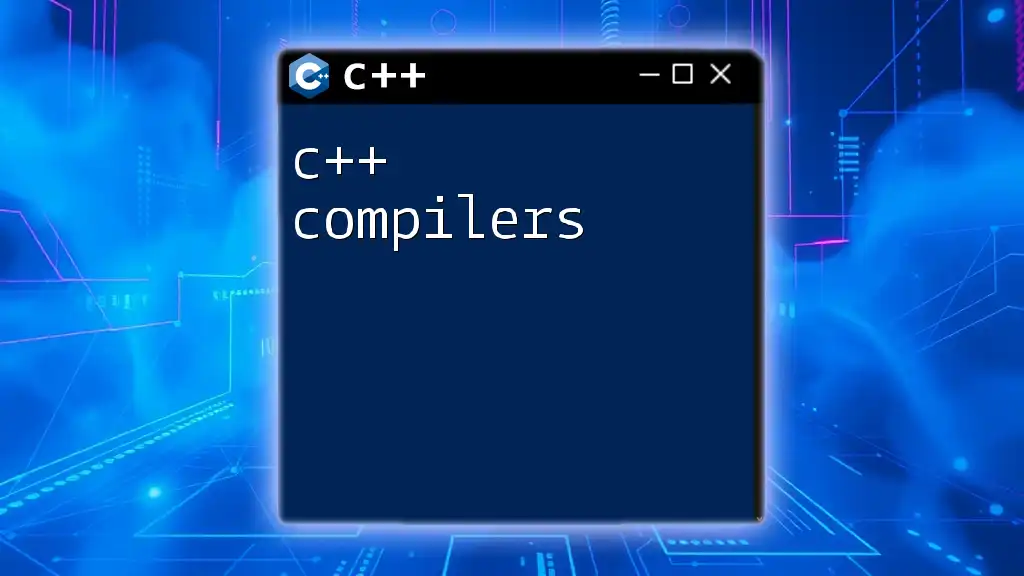
How to Close a File in C++
Using the `close()` Method
In C++, files are closed using the `close()` method, which is a member function of file stream classes such as `ifstream`, `ofstream`, and `fstream`. The syntax for closing a file is as follows:
fileStream.close();
Here's a simple example demonstrating how to close a file after writing data to it:
#include <iostream>
#include <fstream>
int main() {
std::ofstream myfile;
myfile.open("example.txt");
if (myfile.is_open()) {
myfile << "Hello, World!";
myfile.close(); // Closing the file
}
return 0;
}
In this example, the `close()` method is invoked after data is written, ensuring that the file is properly closed.
Why You Should Always Close Your Files
Always using the `close()` method is essential for a few reasons. When you call `close()`, it flushes the buffers and ensures that all written data is saved on disk. If you neglect to close your files, you may encounter underlying issues such as:
- Data corruption due to unflushed buffers.
- Resource leaks that inhibit performance, particularly in applications that handle numerous files.
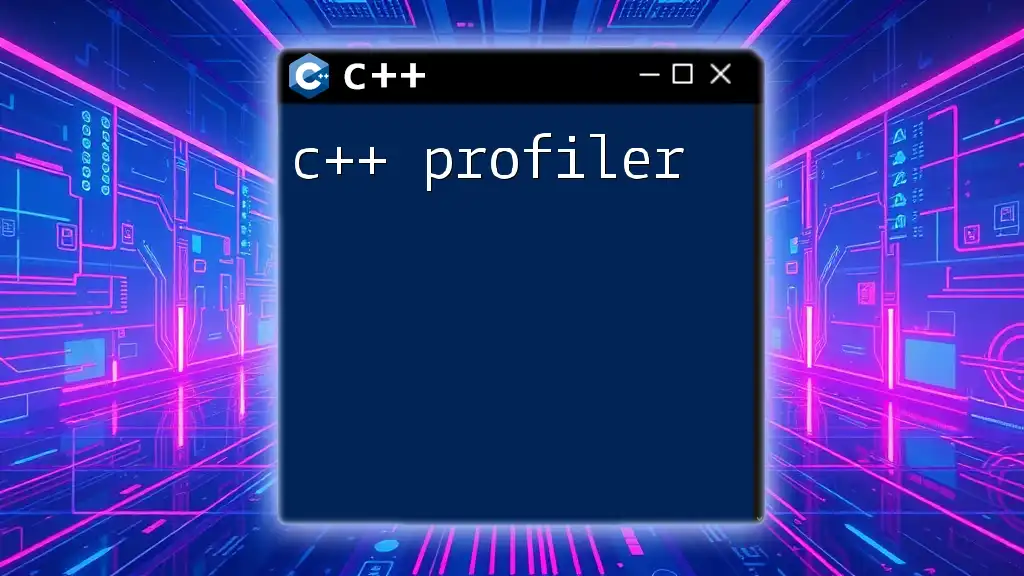
Best Practices for Closing Files
Always Use the Close Method
When dealing with file streams in C++, it is essential to explicitly call the `close()` method to ensure files are closed safely. Failing to do so can lead to unexpected behaviors and potential data loss. Here’s an example of the consequences of not explicitly closing a file:
#include <iostream>
#include <fstream>
int main() {
std::ofstream myfile("example.txt");
// Omitted myfile.close(); - File remains open
myfile << "This is an open file!";
// Possible data loss or corruption when the program ends without closing
return 0;
}
In this case, if the program crashes, you risk losing the written data.
Using RAII for Automatic Resource Management
RAII stands for Resource Acquisition Is Initialization, a programming technique that ties resource management to object lifetime. In C++, when you use RAII with file streams, the file will be automatically closed when the stream object goes out of scope, eliminating the need to explicitly call `close()`. Here’s how you can implement it:
#include <iostream>
#include <fstream>
void writeToFile(const std::string& filename) {
std::ofstream myfile(filename); // Automatically closes when out of scope
if (myfile.is_open()) {
myfile << "Hello, RAII!";
} // File is closed automatically here
}
By using RAII, you simplify your code and increase reliability, ensuring that files are closed even if an exception occurs.
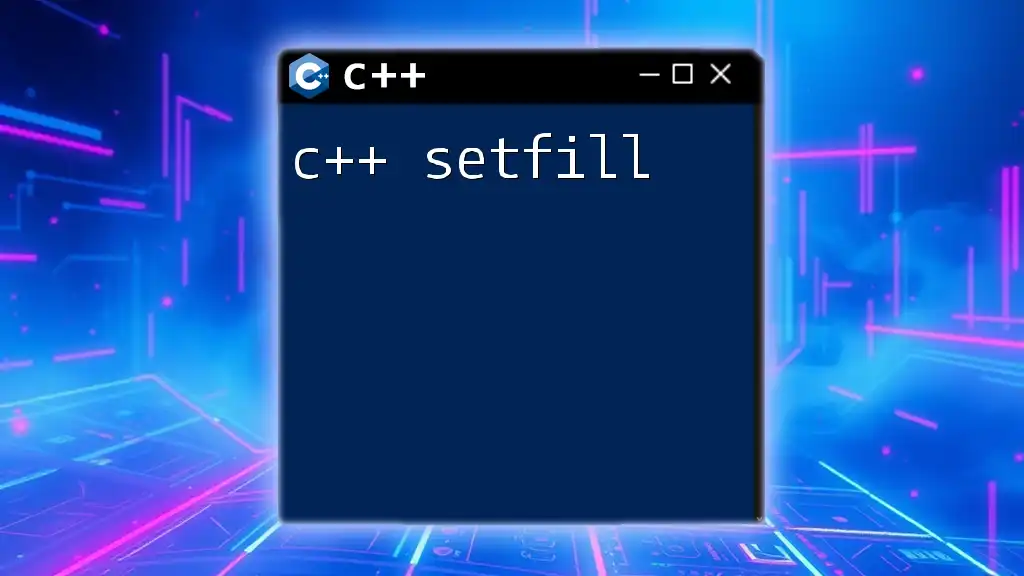
Error Handling While Closing Files
Checking if the File is Open
A good practice when working with file streams is to check whether the file is open before attempting to close it. This avoids unnecessary calls to `close()` and potential issues. Here is an example demonstrating error handling:
#include <iostream>
#include <fstream>
int main() {
std::ofstream myfile("example.txt");
if (myfile) { // File is open
myfile << "Hello, Error Handling!";
}
if (myfile.is_open()) {
myfile.close(); // Safely closing the file
} else {
std::cerr << "Failed to close file." << std::endl;
}
return 0;
}
This code ensures that `close()` is called only if the file is indeed open, thereby preventing potential errors.
Exception Handling and File Errors
In C++, exceptions play a crucial role in managing errors. It's beneficial to wrap file operations in a `try-catch` block to handle any exceptions smoothly. Here is an example:
#include <iostream>
#include <fstream>
int main() {
try {
std::ofstream myfile("example.txt");
if (!myfile) throw std::ios_base::failure("File could not be opened.");
myfile << "Hello, Exception Handling!";
myfile.close();
} catch (const std::ios_base::failure& e) {
std::cerr << e.what() << std::endl; // Handling the exception
}
return 0;
}
Using exception handling, this code manages potential issues while opening or writing to files and provides a robust mechanism to inform the user of any underlying problems.
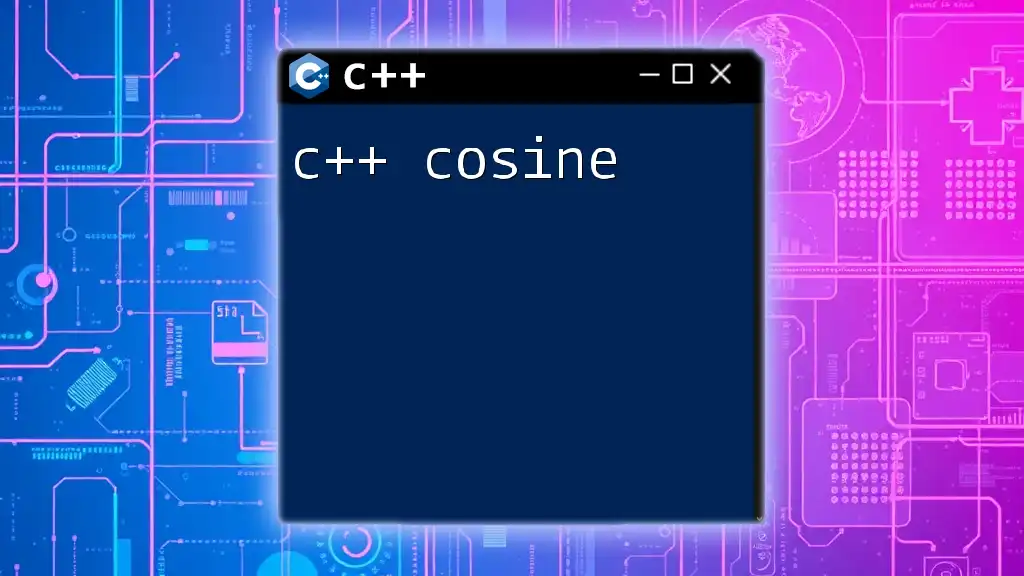
Conclusion
In conclusion, understanding how to c++ close file operations effectively is vital for maintaining data integrity and resource management. Remembering to close files, utilizing RAII, and implementing robust error handling techniques will improve your C++ programming and file management practices. These practices not only streamline your code but also safeguard your application against common pitfalls associated with file handling. By adhering to these guidelines, you can develop a strong foundation in file I/O operations in C++.