The `C++` `writefile` concept involves using file output streams to create and write data to a file efficiently. Here's a simple example:
#include <iostream>
#include <fstream>
int main() {
std::ofstream outfile("example.txt");
outfile << "Hello, World!" << std::endl;
outfile.close();
return 0;
}
Understanding Files in C++
What Are Files?
In the context of programming, files are essential for storing and retrieving data. They act as a long-term storage medium, allowing programs to preserve information beyond their runtime. In C++, files can either be text files (which store data in a human-readable form) or binary files (which store data in a format that is efficient for machines but not easily readable by humans). Writing data to a file is a fundamental task that enables applications to log information, save user data, or manage configuration settings.
Types of Files
- Text Files: These files contain data in a plain text format. They can typically be opened and edited using any text editor. Examples include `.txt`, `.csv`, and `.json`.
- Binary Files: Binary files store data in a format specific to the application and are generally not human-readable. Common types include `.bin`, `.exe`, and image formats.
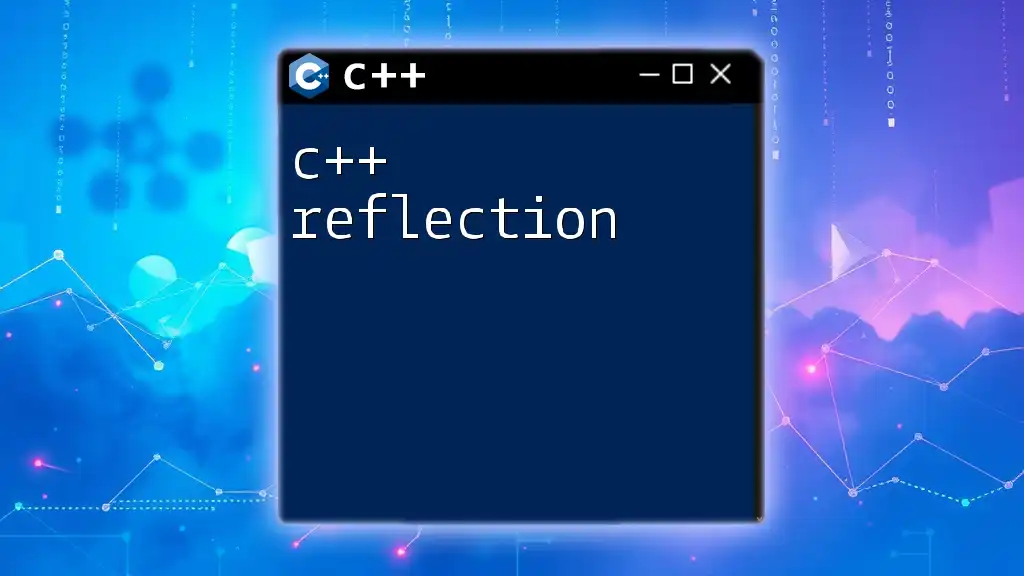
Setting Up Your Environment
Tools Required
To work with file operations in C++, you will need a few essential tools:
- Compiler: A C++ compiler such as GCC, Clang, or Visual Studio is necessary for compiling your code.
- IDE: Integrated Development Environments like Visual Studio Code or Code::Blocks can help enhance productivity with features like syntax highlighting and debugging facilities.
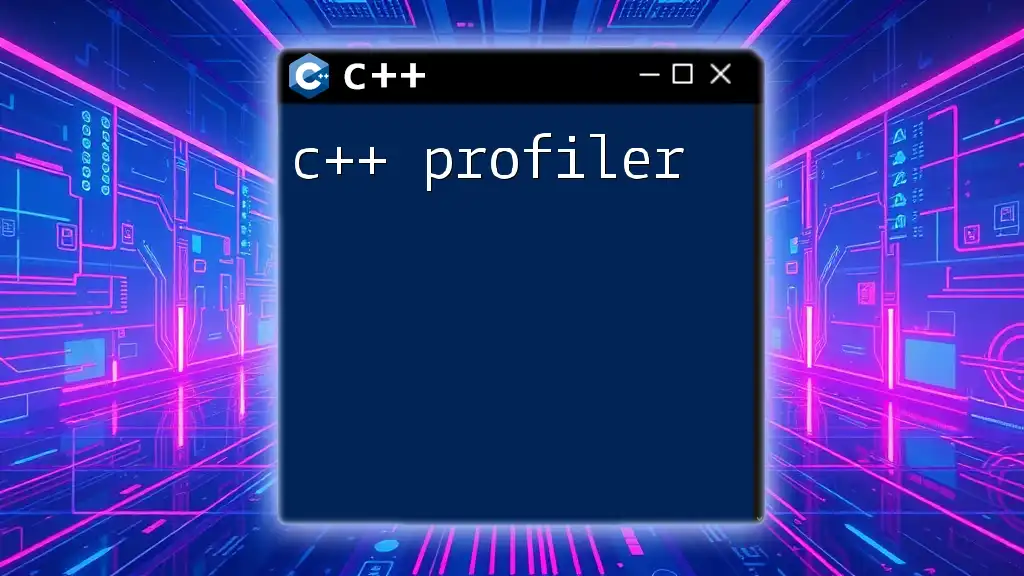
Basic File Operations
Open a File
Opening a file in C++ is the first step toward writing to it. This is typically done using the `std::ofstream` class, which is part of the `<fstream>` header. Here’s a simple example of how to open a file:
#include <fstream>
std::ofstream outputFile("example.txt");
In this code, we create an instance of `std::ofstream`, specifying the file name. If the file does not exist, it will be created automatically.
Writing to a File
Once you have a file open, you can write data to it. The `<<` operator allows you to send various types of data to the file stream. Here's how you can write a string followed by a newline to a file:
outputFile << "Hello, World!" << std::endl;
This line adds the text "Hello, World!" to `example.txt`, followed by a line break.
Closing a File
Closing the file after writing is crucial to ensure that all data is flushed to disk and resources are freed. Always remember to close your files like this:
outputFile.close();
Failing to close a file can lead to data loss or corruption.
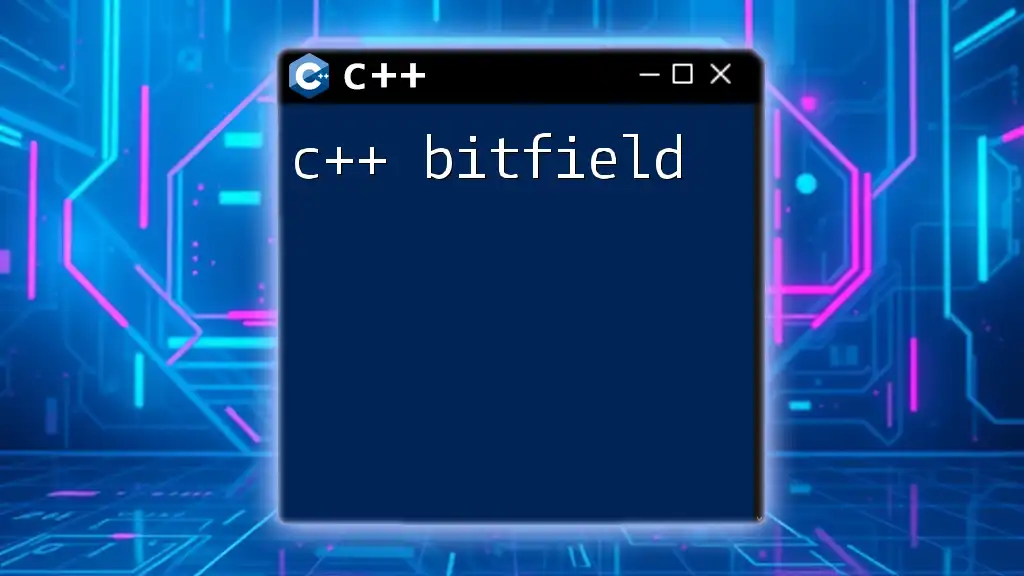
The Complete WriteFile Function
Creating the WriteFile Function
To streamline the process of writing to files, you can create a reusable function. The purpose of this function is to take a filename and data as inputs and write the data to the specified file. Below is the definition of such a function:
void writeFile(const std::string& filename, const std::string& data) {
std::ofstream outputFile(filename);
if (outputFile.is_open()) {
outputFile << data;
outputFile.close();
} else {
std::cerr << "Error opening file!" << std::endl;
}
}
This function checks if the file opened successfully before writing the data. If it fails to open, it prints an error message.
Example: Using the WriteFile Function
To illustrate how the `writeFile` function can be utilized, consider the following code snippet where we allow the user to input data, which will then be written to a file:
#include <iostream>
int main() {
std::string data;
std::cout << "Enter data to write to file: ";
std::getline(std::cin, data);
writeFile("user_data.txt", data);
return 0;
}
In this example, when the program runs, it prompts the user to enter some data, which is then saved in `user_data.txt`.
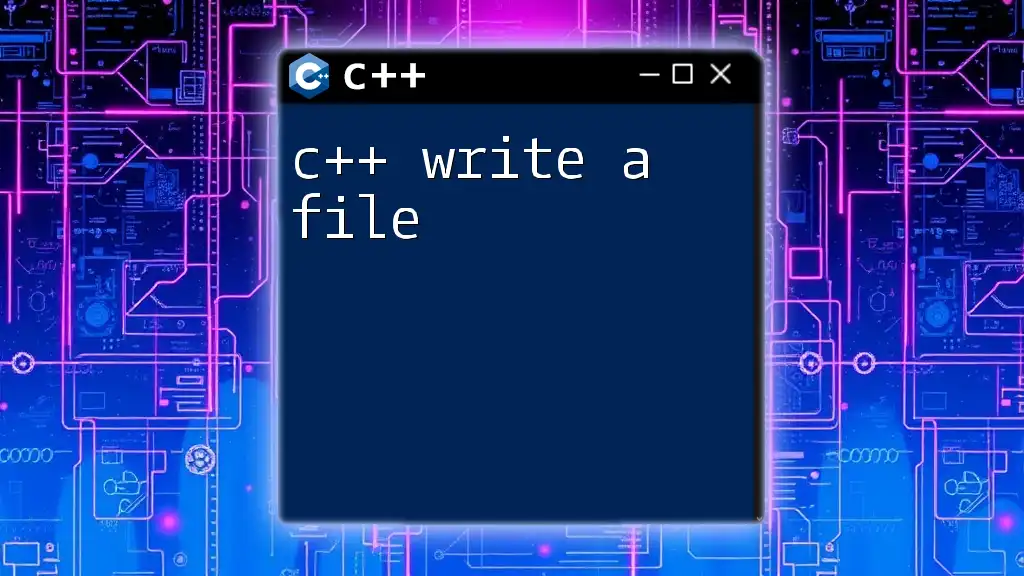
Advanced File Writing Techniques
Writing in Different Formats
C++ allows you to write files in various formats; you can opt for either text or binary depending on your requirements. Using binary mode can yield performance benefits and save space. Consider this code snippet to write binary data to a file:
std::ofstream binaryFile("data.bin", std::ios::binary);
int number = 42;
binaryFile.write(reinterpret_cast<char*>(&number), sizeof(number));
binaryFile.close();
In this instance, we opened `data.bin` in binary mode and wrote an integer value to the file.
Appending to a File
Sometimes you may want to add content to an existing file without overwriting its current contents. To achieve this, you can open the file in append mode using `std::ios::app`. Here’s how:
std::ofstream appendFile("example.txt", std::ios::app);
appendFile << "Appending new line." << std::endl;
appendFile.close();
By using the append mode, you can add new data at the end of `example.txt`, preserving any existing content.
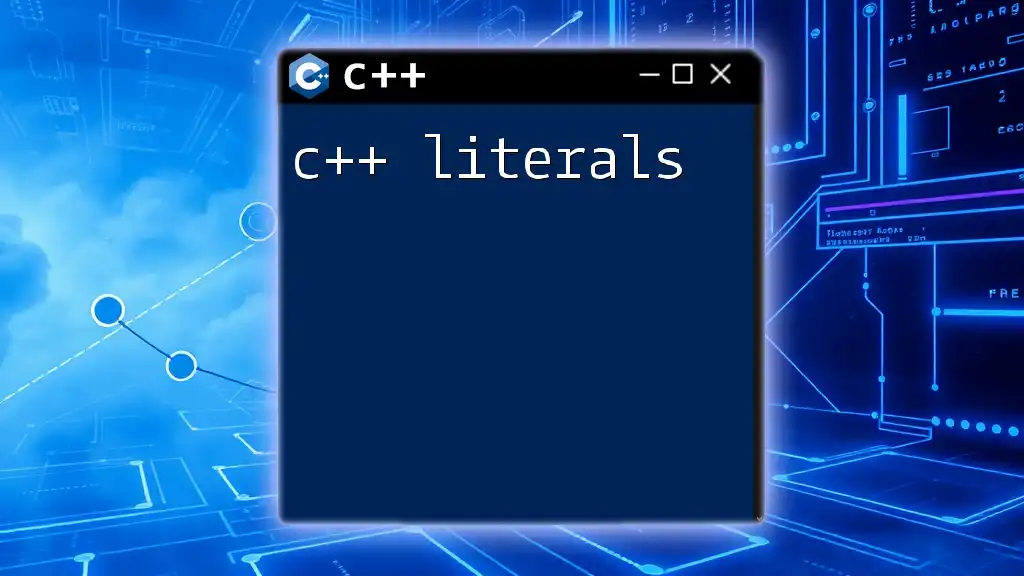
Error Handling in File Operations
Common File Errors
There are various types of errors that can occur while handling files in C++. Some common issues include:
- The file does not exist.
- The program lacks permission to open or modify the file.
Implementing Error Handling
It’s vital to implement error handling to prevent your program from crashing or producing unexpected results. Here’s an example of safe file writing using exception handling:
try {
writeFile("example.txt", "Safe write!");
} catch (const std::exception& e) {
std::cerr << "An error occurred: " << e.what() << std::endl;
}
By wrapping the function call in a try-catch block, any errors thrown during file operations can be caught and managed gracefully.
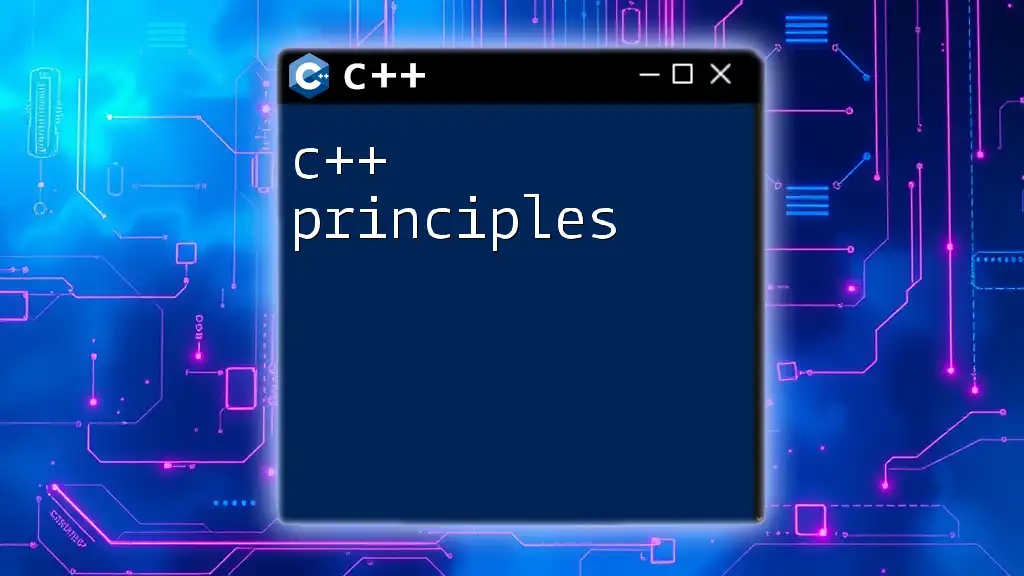
Best Practices for File Handling
Regularly Closing Files
Always ensure that you close open files when they are no longer needed. This practice helps prevent resource leaks and data inconsistency.
Using RAII Pattern
The Resource Acquisition Is Initialization (RAII) pattern is a powerful C++ technique where resource management is tied to object lifetime. Using smart pointers can enhance safety. Instead of manually managing `std::ofstream` instances, you might consider using RAII-compliant wrappers for automatic cleanup.
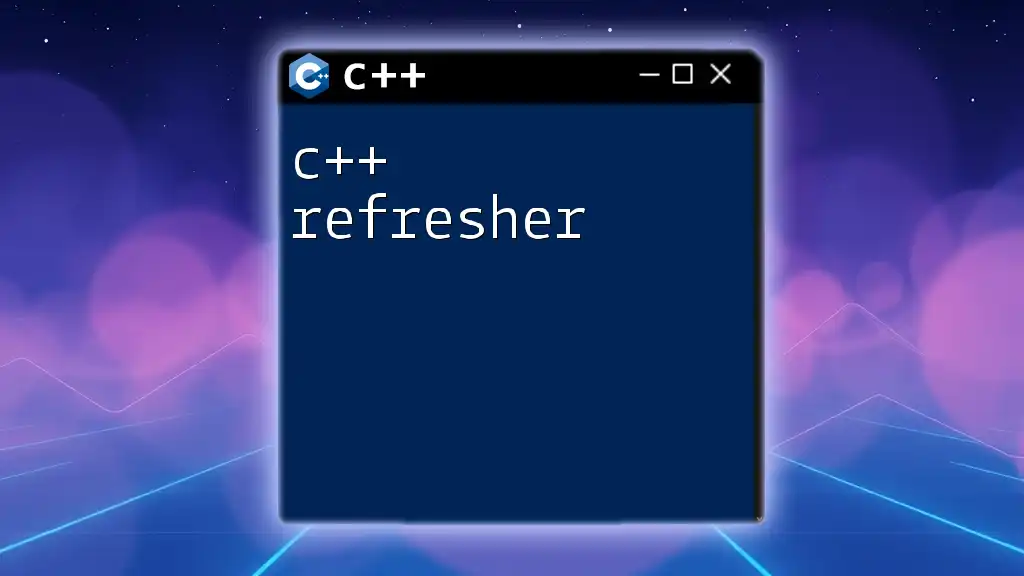
Conclusion
C++ file handling, particularly the ability to write to files, is a fundamental skill that enables programmers to create robust applications. Mastering the intricacies of the `c++ writefile` operation can significantly enhance your software’s capabilities, providing the means for effective data management and storage.
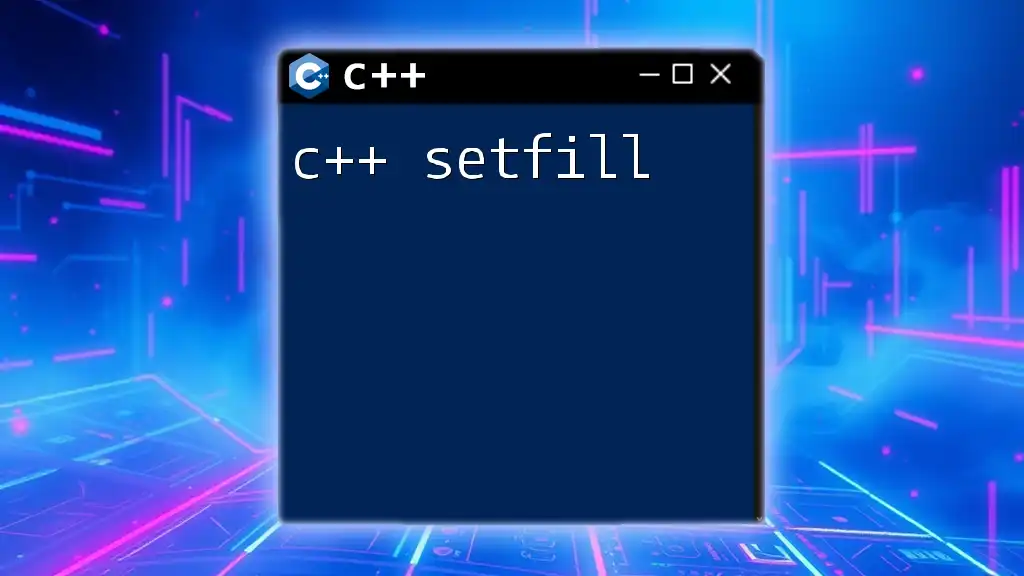
Additional Resources
Further Reading
To deepen your understanding of file handling in C++, various resources such as books, online courses, and relevant articles can provide invaluable knowledge. Look for resources that cover both the basics and advanced topics related to file input and output.
Sample Projects
Starting with simple projects, such as creating a logging system or a basic data storage application, can solidify your skills. For those wanting to challenge themselves, consider working on applications that require data serialization or sophisticated file management techniques.
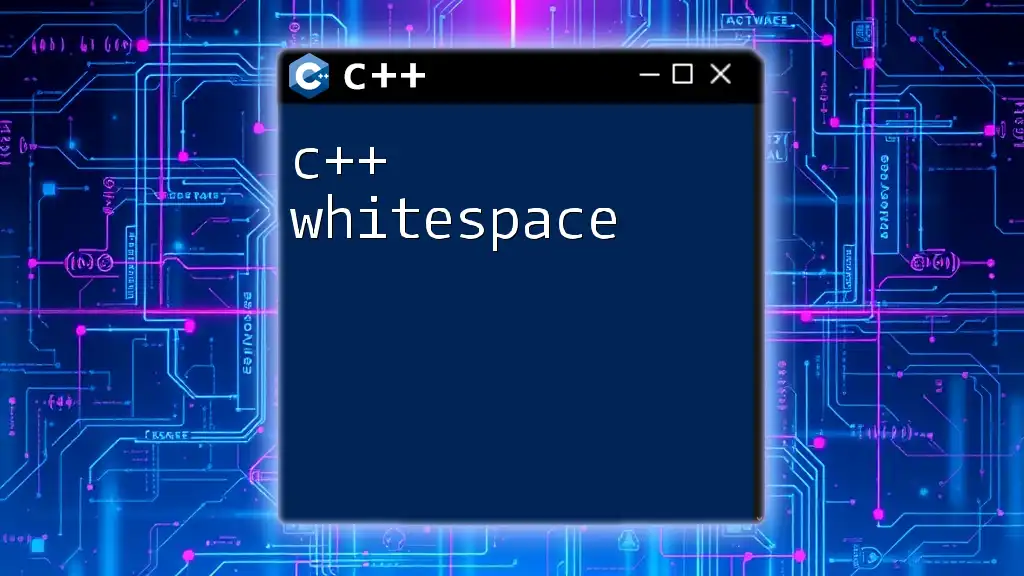
Call to Action
If you're interested in more tutorials on C++, make sure to subscribe to stay updated. We would also love to hear about your experiences or questions regarding file handling in C++ in the comments below!