C++ programming in Eclipse is facilitated through the Eclipse IDE, which provides powerful tools and plugins for coding, debugging, and compiling C++ projects efficiently.
Here's a simple C++ program that you can run in Eclipse:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Setting Up Eclipse for C++
Downloading and Installing Eclipse
Choosing the Right Version of Eclipse
When you decide to work with C++ and Eclipse, it’s critical to select the right IDE version. The Eclipse IDE for C/C++ Developers is specifically tailored for C++ programming, including the necessary tools and features that support development processes.
Installation Steps
Begin by downloading the appropriate version of Eclipse from the [Eclipse website](https://www.eclipse.org/downloads/). After downloading, unzip the package and initiate the `eclipse` executable. During the first startup, you will be prompted to select a workspace. Choose a convenient directory where your projects will be stored, making it easier to navigate and organize your files later on.
Configuring Eclipse for C++ Development
Setting Up the C++ Compiler
Before you can write and execute C++ programs, it’s essential to have a C++ compiler installed. For Windows users, MinGW (Minimalist GNU for Windows) provides the necessary tools. On Linux and macOS, GCC (GNU Compiler Collection) typically comes pre-installed or can be added with package management systems.
To configure MinGW with Eclipse:
- Download and install MinGW.
- Add the bin directory of MinGW to your system’s PATH variable to make its command-line tools accessible globally.
- Open Eclipse, go to `Window -> Preferences -> C/C++ -> Build -> Environment`, and ensure the path is correctly set.
Building Your First C++ Project
Create a simple "Hello, World!" project to familiarize yourself with the workings of Eclipse.
- Choose `File -> New -> C++ Project`.
- Name your project (for instance, `HelloWorld`) and select the `Hello World C++ Project` template under the `C++ Project` type.
- Click `Finish` to create the project.
Once your project is created, it will have a folder structure that includes `src`, where your code will reside.
Enter the following code in the `main.cpp` file, which should be automatically created in the `src` folder:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
This code represents the entry point of a C++ program, where `#include <iostream>` allows the program to utilize standard input and output streams, and `cout` prints text to the console.
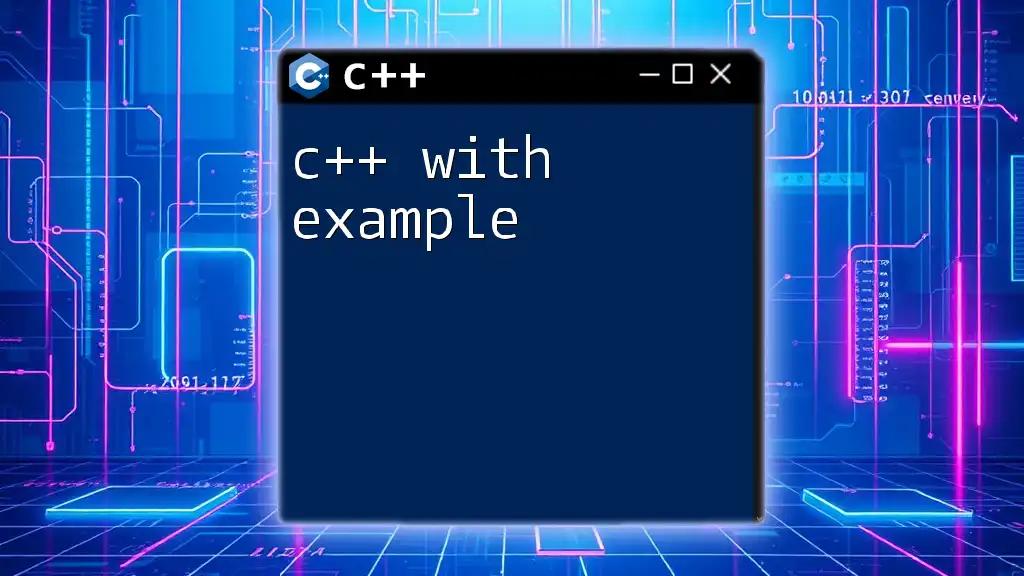
Writing and Compiling C++ Code in Eclipse
Using the Editor Features
Code Completion and Syntax Highlighting
Eclipse offers robust code completion features that speed up your coding process. As you type, suggestions for functions, variables, and parameters will appear, enabling you to choose from existing code elements. This is particularly useful for minimizing errors from typos.
Tips for Using the Editor Effectively
Getting comfortable with the following keyboard shortcuts can improve productivity:
- `Ctrl + Space`: Trigger code completion.
- `Ctrl + Shift + R`: Open a resource (like files) quickly.
- `F3`: Jump to the declaration of a variable or function.
Compiling and Running Your Code
Build Process in Eclipse
In C++ development with Eclipse, files are compiled automatically when you save your code. However, you can also build manually by selecting `Project -> Build All`. If there are any compilation errors, they will appear in the Console or Problems view, allowing you to address them promptly.
Running Your C++ Application
To execute your code, right-click on the `main.cpp` file and choose `Run As -> Local C/C++ Application`. This runs the program using the default debugger. The output will display in the Console view, where you should see:
Hello, World!
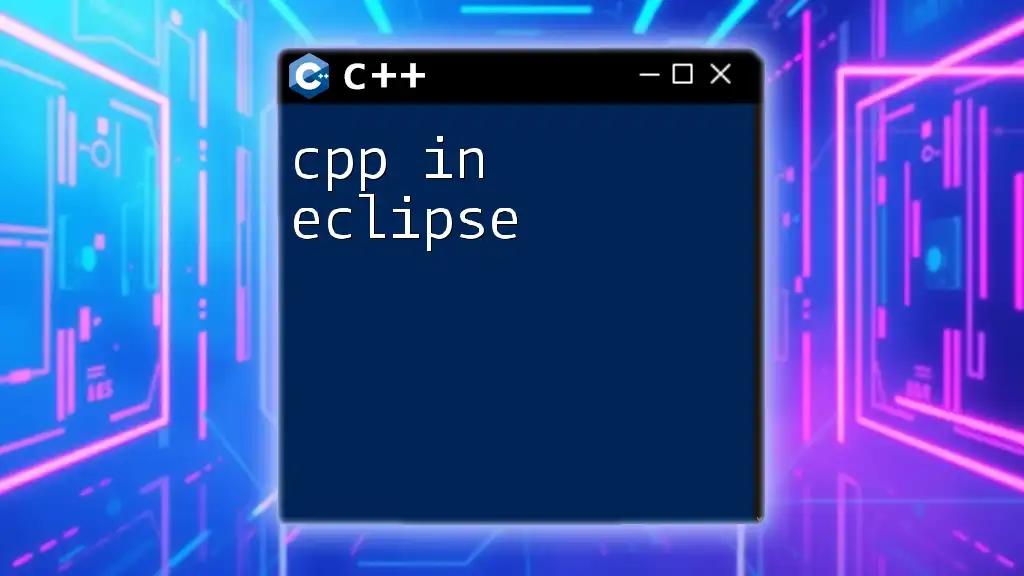
Debugging C++ Applications in Eclipse
Introduction to Debugging
Why Debugging is Important
Debugging is an essential part of the development cycle. It allows you to identify and eliminate errors in your code, improving the overall quality and reliability of your application.
Using Eclipse Debugger
Setting Breakpoints
Breakpoints are markers you can set on a specific line of code where the program execution will pause. This is invaluable for analyzing variable states and program flow. To set a breakpoint in Eclipse, double-click in the margin next to the line number where you want the execution to halt.
Step Through Your Code
During debugging, you can control the execution flow of your application:
- Step Into (`F5`): Enter into functions to follow the execution.
- Step Over (`F6`): Execute the current line and move to the next line in the same function.
- Step Out (`F7`): Continue running until the current function exits.
Analyzing Variables and Call Stack
Watching Variables
Utilize the "Variables" view in Eclipse during a debug session to monitor the current values of variables. You can even edit these values to test different outcomes without changing the source code.
Understanding the Call Stack
The "Call Stack" view shows the current state of function calls. This helps trace back through the flow of execution, providing insights into how different functions interact, which is particularly useful when troubleshooting complex code.
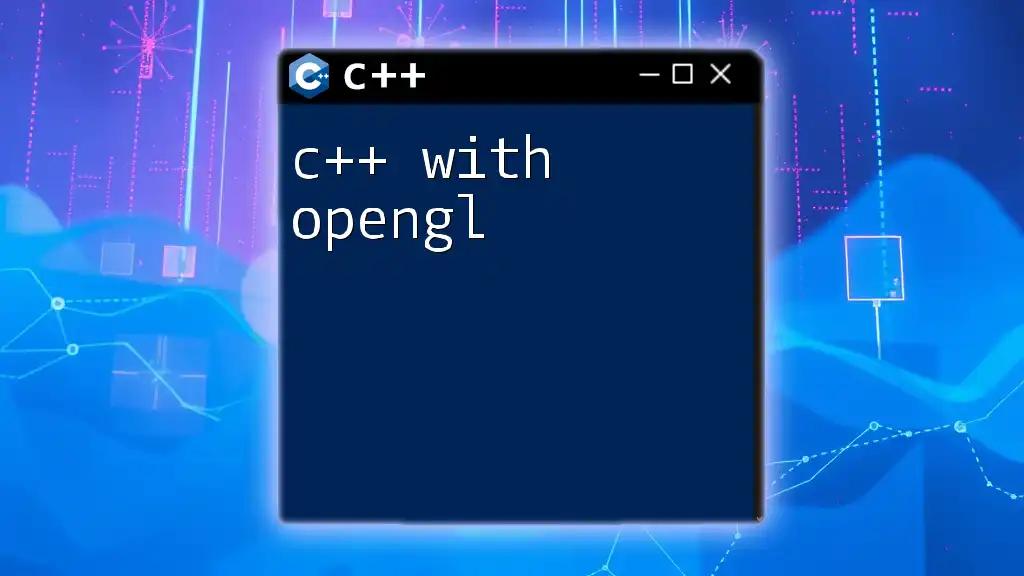
Advanced Features of C++ in Eclipse
Utilizing Plugins for Enhanced Functionality
Essential Plugins for C++ Development
There are several plugins that can enhance your C++ development experience in Eclipse:
- CDT (C/C++ Development Tooling): This is essential as it provides core IDE functionality for C++.
- Graphic Debugger: Helpful for debugging graphical applications with visual feedback.
Integration with Version Control Systems
Using Git with Eclipse
Version control is crucial for managing changes and collaborating with others. Eclipse integrates well with Git. To begin using Git:
- Install the EGit plugin.
- Create or clone a Git repository through `File -> Import -> Git -> Projects from Git`.
- After making changes, right-click your project and select `Team -> Commit` to save changes to your repository.
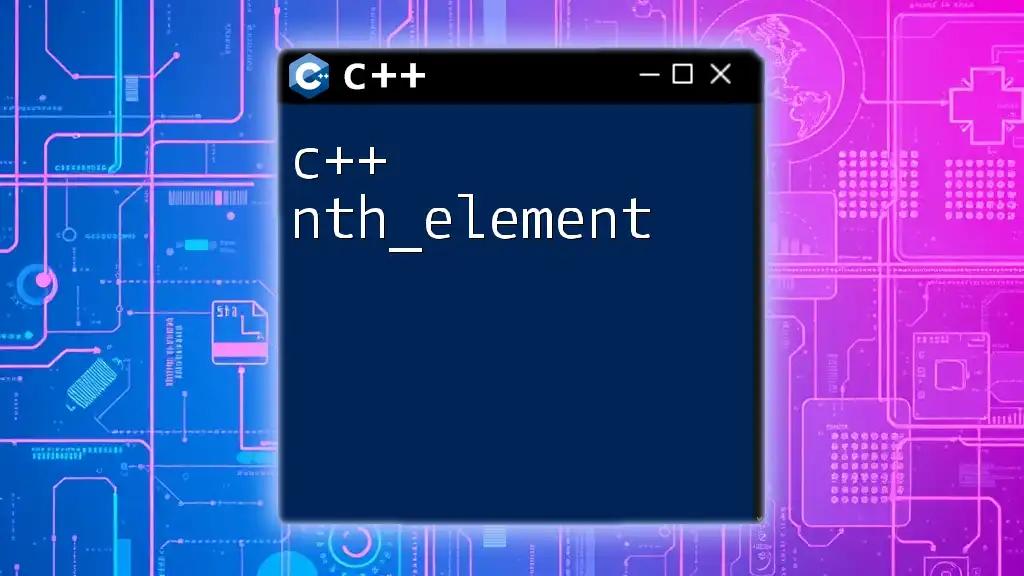
Tips and Best Practices for C++ Development with Eclipse
Effective Project Organization
Structuring Your Projects
Establish a solid directory structure for your files. This approach makes it easier to manage larger projects and locate files quickly. Consider separating your source code, headers, resources, and documentation into distinct folders within your project.
Commenting and Documentation
Importance of Comments
Effective commenting enhances code readability, making it easier for yourself and others to understand the logic behind your implementation. For instance:
// Function to calculate factorial of a number
int factorial(int n) {
return (n <= 1) ? 1 : n * factorial(n - 1);
}
Performance Optimization Techniques
Code Efficiency
Enhancing the efficiency of C++ code can significantly improve application performance. Prioritize algorithms with better time complexity and use data structures that minimize overhead.
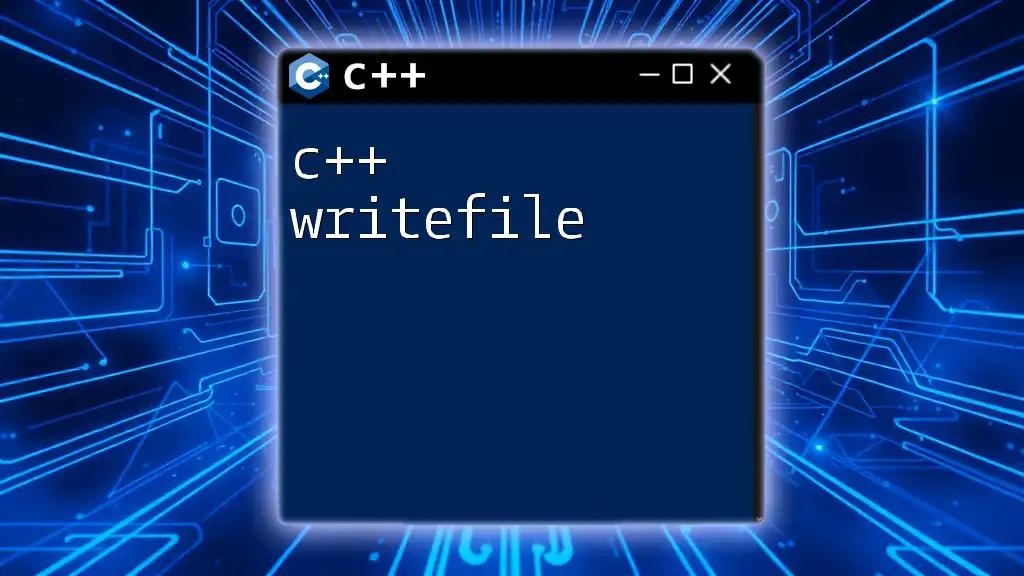
Conclusion
Summary of Benefits
Utilizing C++ with Eclipse offers a powerful IDE equipped with various features aimed at streamlining the development process. From code completion to debugging tools, Eclipse makes writing, testing, and managing C++ code more efficient.
Encouragement to Explore Further
The world of C++ programming is vast and continually evolving. With your newfound knowledge of C++ with Eclipse, you're encouraged to dive deeper into both the language itself and the myriad tools available within the Eclipse ecosystem.
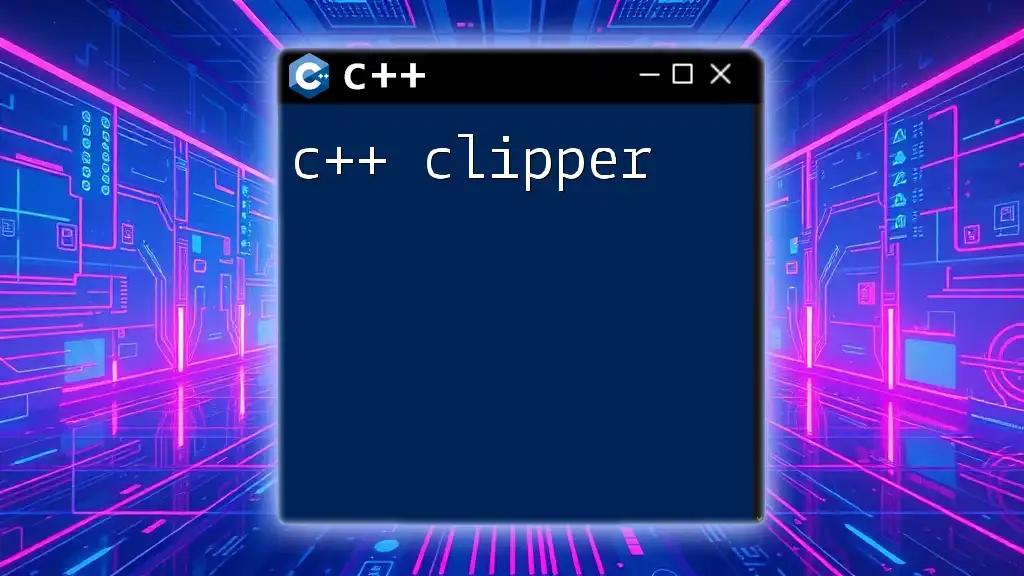
Additional Resources
For further growth and learning in C++ and Eclipse, consider exploring the official [Eclipse CDT documentation](https://www.eclipse.org/cdt/documentation.php) and seeking out online forums or C++ programming tutorials that can guide you on your journey.