In C++, the `#include` directive is used to include the contents of a specified file or library into your program, allowing you to utilize functions, classes, and variables defined in those files.
#include <iostream> // This includes the standard input-output stream library
Understanding the `#include` Directive
What is `#include`?
The `#include` directive is a preprocessor command in C++ that instructs the compiler to include the contents of a specified file into the program. This is crucial for utilizing external code, such as libraries or header files, allowing greater modularity and code reuse. By using `#include`, you can easily leverage existing functionalities without having to write every function from scratch.
Types of Includes
Standard Includes
In C++, including standard libraries is a common practice. Standard includes refer to the pre-defined header files provided by the C++ Standard Library, which facilitate a wide range of operations, from input/output to complex mathematical computations.
For instance, to utilize the input and output stream capabilities, you would write:
#include <iostream>
Other commonly used standard headers include:
- `<cmath>`: for mathematical functions.
- `<cstring>`: for string manipulation.
- `<vector>`: for working with dynamic arrays.
Including these files allows you to use their built-in functions seamlessly.
User-Defined Includes
Besides standard libraries, you may find the need to create your own header files, especially for larger projects. User-defined includes help you organize code into manageable sections.
To create a simple header file named `myheader.h`, you would define it as follows:
// myheader.h
#ifndef MYHEADER_H
#define MYHEADER_H
void greet();
#endif
In this example, `greet()` can be a function you implement in a corresponding source file. When you include this header in your main C++ program, you can call the `greet()` function without rewriting its definition.
How to Use `#include`
Syntax
The syntax of the `#include` directive varies depending on whether you are including standard libraries or user-defined headers.
To include a standard library, use angle brackets:
#include <header> // For standard libraries
For user-defined headers, double quotes are used:
#include "header" // For user-defined headers
This distinction clarifies the location the compiler searches for the files — standard libraries in the system directories and user-defined headers in the current directory.
Best Practices
It is important to understand when to use each type of include. Angle brackets (`< >`) tell the compiler to look for the header in system directories, while double quotes (`" "`) prompt it to consider the local directory first. Prioritizing local files helps avoid unnecessary dependency on system libraries.
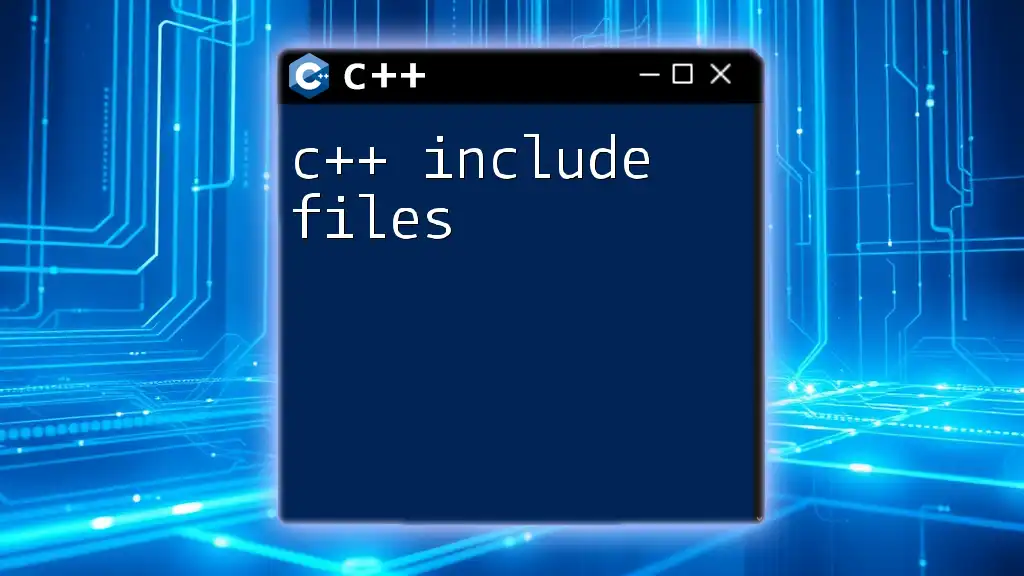
The Preprocessor and Its Role
Understanding the Preprocessor
The C++ preprocessor operates before the actual compilation of code begins. When you use `#include`, the preprocessor replaces the directive with the entire content of the included file. This means that by the time the compiler processes your code, the contents of the included files are available as if they had been typed out directly in your program.
How `#include` Affects Compilation
When the preprocessor encounters an `#include` statement, it effectively merges the contents of the file into the current file. This process can lead to complications such as multiple inclusions, which occurs if the same header file is included multiple times unintentionally.
To mitigate this issue, developers employ include guards or the `#pragma once` directive.
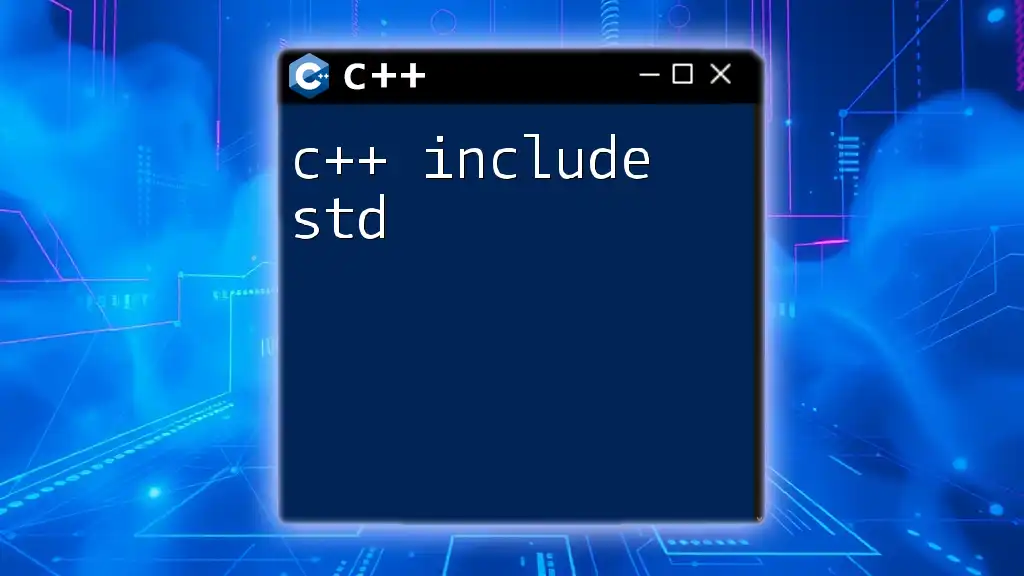
Guards and Best Practices
What Are Include Guards?
Include guards are a programming practice used to prevent multiple inclusions of a header file. They help to maintain cleaner code and ensure that definitions are only included once per compilation unit.
Implementation of Include Guards
Creating an include guard involves using conditional macros around the header file's contents. Here’s an example:
// myheader.h
#ifndef MYHEADER_H
#define MYHEADER_H
void greet();
#endif
This pattern ensures that if `myheader.h` is included more than once, the compiler will ignore subsequent inclusions.
Best Practices for Includes
Organizing your includes is essential for maintaining clear and efficient code. Limit the number of include statements by removing unnecessary headers and favoring forward declarations when possible. This practice can drastically reduce compilation time, particularly in larger projects.
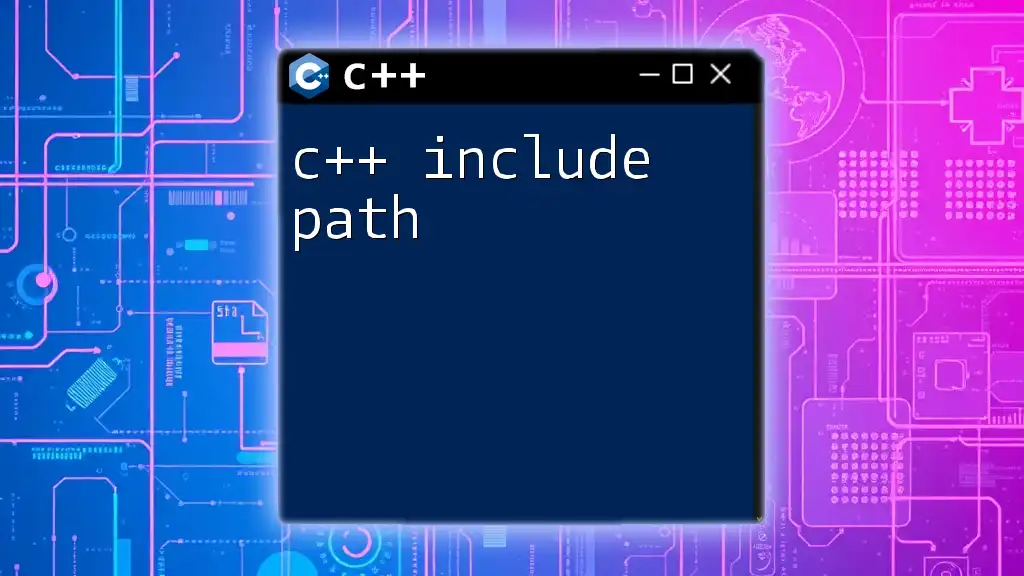
Common Mistakes with `#include`
Forgetting Include Guards
Neglecting to add include guards can lead to various issues, such as redefinition errors and unexpected behaviors as multiple headers are pulled into the same source file. This often results in the compilation failing.
Including Unnecessary Headers
Including headers that your code doesn’t actually use can bloat your project, slowing down compile times and making it harder to read. Always review your include statements and retain only those that are necessary for the project.
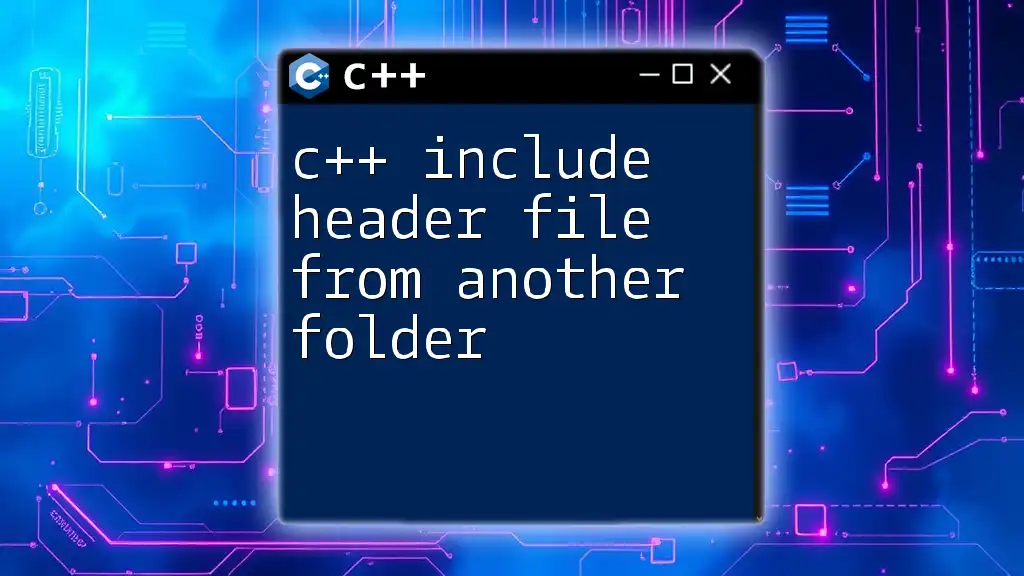
The Future of Include Management
Alternatives to Traditional Includes
While traditional include directives are still widely used, the `#pragma once` directive serves as an alternative to include guards. When you include a file using `#pragma once`, the compiler ensures that it is included only once per compilation unit, simplifying the management of includes.
Here’s how you would use it:
#pragma once
void greet();
Using Modules in Modern C++
With the introduction of C++20, modules present a modern alternative to traditional header files and include directives. Modules allow for better organization, encapsulation, and can significantly enhance compile-time performance by reducing dependencies.
Despite their benefits, modules come with a learning curve and are not yet universally supported across all compilers.
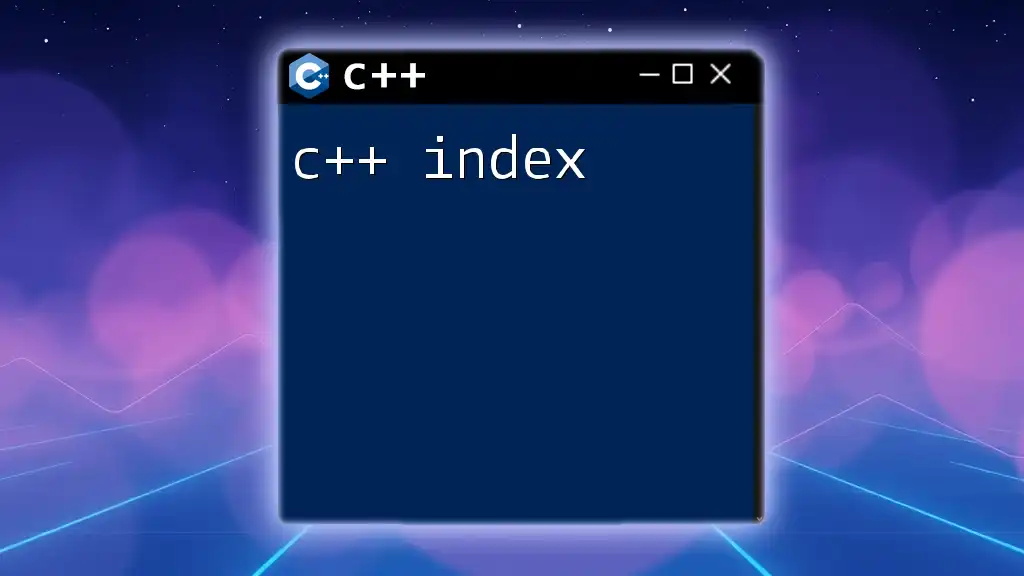
Conclusion
Understanding the `c++ include` directive is critical for any programmer looking to write effective, modular, and maintainable code. Through mastering libraries, include guards, and keeping an eye on future developments like modules, you can significantly improve your coding practices. Always strive for clean, efficient, and well-structured code, and remember that effective use of the `#include` directive is foundational to achieving that goal.
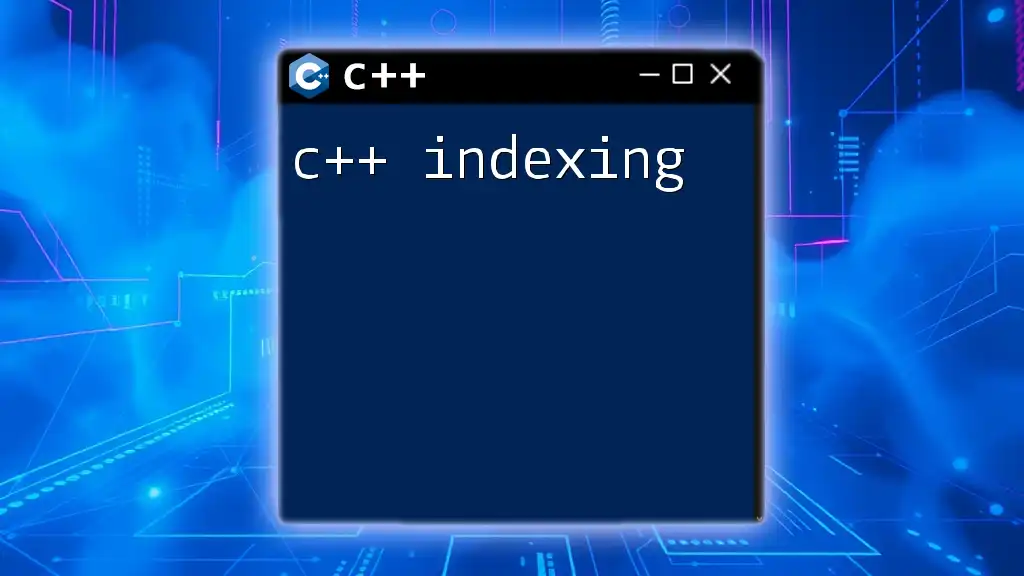
Additional Resources
For those interested in deepening their understanding of C++ includes and further honing their skills, exploring official documentation, reputable programming books, and online educational platforms can be invaluable.