`std::unique_ptr` is a smart pointer in C++ that ensures exclusive ownership of a dynamically allocated object, automatically releasing the object when the `unique_ptr` goes out of scope.
Here’s a simple code snippet demonstrating its usage:
#include <iostream>
#include <memory>
int main() {
std::unique_ptr<int> ptr(new int(42));
std::cout << *ptr << std::endl; // Outputs: 42
return 0;
}
Overview of Smart Pointers
What are Smart Pointers?
In C++, smart pointers are objects that help manage the memory of dynamically allocated objects. Unlike traditional pointers, smart pointers automatically handle memory deallocation, which helps prevent memory leaks and dangling pointers in your code.
Using smart pointers simplifies memory management in C++, leading to safer and more robust applications.
Types of Smart Pointers
There are three primary types of smart pointers in C++: `shared_ptr`, `weak_ptr`, and `unique_ptr`. While `shared_ptr` allows multiple pointers to own the same resource, and `weak_ptr` provides a non-owning reference to a resource, our focus here will be on `unique_ptr`.
Understanding the significance of `unique_ptr` is crucial, as it enforces unique ownership of resources, ensuring that no two pointers manage the same resource, thus avoiding conflicts and undefined behavior.

Understanding `unique_ptr`
Definition of `unique_ptr`
`unique_ptr` is a smart pointer introduced in C++11 that provides a mechanism to manage dynamic memory in a way that ensures exclusive ownership and automatic cleanup. When a `unique_ptr` goes out of scope, it automatically deallocates the memory it owns. This characteristic is essential for resource management, making it a preferred choice for robust C++ applications.
How `unique_ptr` Works
When you allocate memory using a `unique_ptr`, it encapsulates the raw pointer, ensuring that it is deleted when the `unique_ptr` object is destroyed. This automatic resource management prevents memory leaks, which can occur with raw pointers if they are not explicitly deallocated.
Syntax and Structure of `unique_ptr`
The general syntax for declaring a `unique_ptr` is as follows:
std::unique_ptr<Type> ptr(new Type);
However, the recommended way to create a `unique_ptr` is by using `std::make_unique`:
std::unique_ptr<Type> ptr = std::make_unique<Type>();
This approach simplifies memory management and enhances code safety.
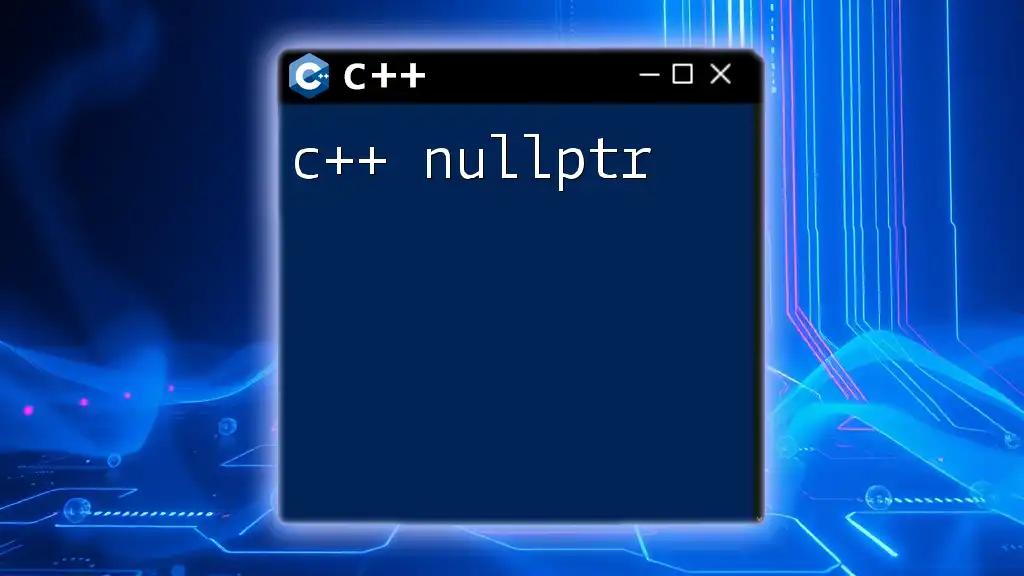
Creating and Using `unique_ptr`
Initializing `unique_ptr`
To create a `unique_ptr`, you typically use `std::make_unique`, which makes writing code cleaner and safer. For example:
std::unique_ptr<int> ptr = std::make_unique<int>(10);
In the above snippet, an integer is allocated dynamically, initialized to 10, and managed by `ptr`.
Accessing and Modifying Values
Once you have a `unique_ptr`, you can access or modify the value it points to by dereferencing it. Consider this example:
std::unique_ptr<int> numPtr = std::make_unique<int>(5);
*numPtr = 20; // Modifying value
std::cout << *numPtr; // Accessing value
Here, you can see that you can modify the held value directly through the pointer, showcasing how straightforward usage can be with `unique_ptr`.
Transferring Ownership
One of the key features of `unique_ptr` is its exclusive ownership. You can transfer ownership from one `unique_ptr` to another using `std::move`:
std::unique_ptr<int> ptr1 = std::make_unique<int>(5);
std::unique_ptr<int> ptr2 = std::move(ptr1); // Ownership transferred
After the move, `ptr1` no longer owns the memory, while `ptr2` assumes ownership. This transfer mechanism allows for flexible memory management without copying.
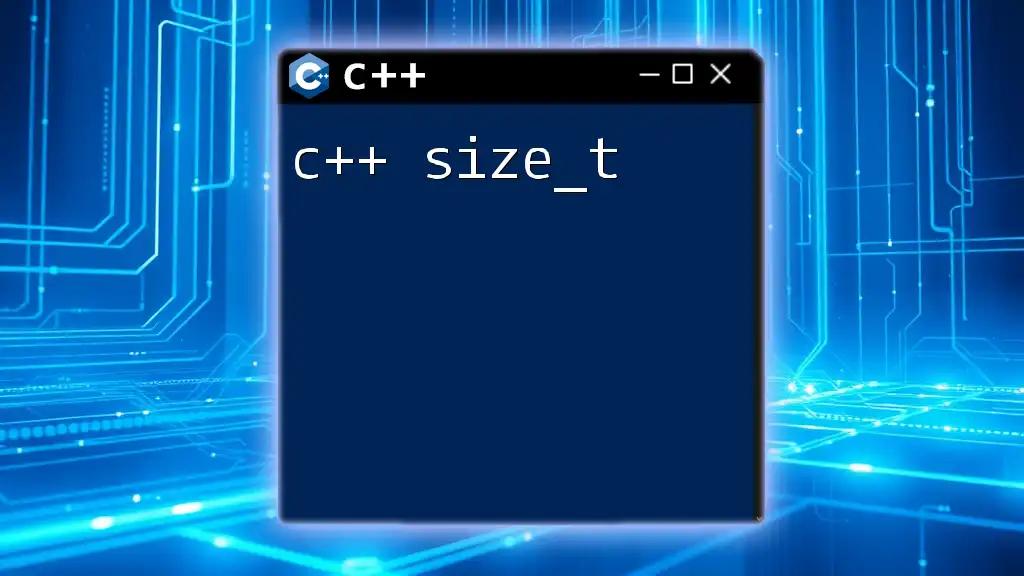
Best Practices with `unique_ptr`
When to Use `unique_ptr`
You should consider using `unique_ptr` whenever you need a dynamically allocated object that has a single owner throughout its lifetime. They are particularly useful in scenarios like managing resources in container objects and implementing factory patterns.
Using `unique_ptr` ensures that the resource is automatically freed when it goes out of scope, preventing possible memory leaks.
Avoiding Common Pitfalls
While `unique_ptr` simplifies memory management, it's vital to be aware of potential pitfalls. For instance, unnecessarily transferring ownership may result in dangling pointers:
std::unique_ptr<int> ptr = std::make_unique<int>(10);
std::unique_ptr<int> ptr2 = std::move(ptr);
// ptr is now invalid
std::cout << *ptr; // Undefined behavior
To avoid such issues, ensure you're aware of ownership transfer, and consider using `make_unique` in most cases for safety.
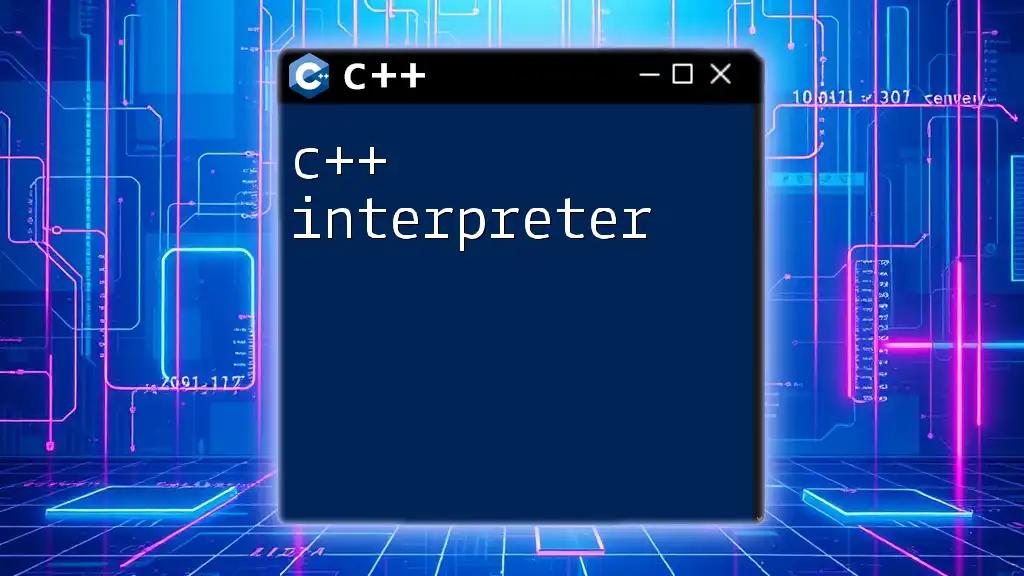
`unique_ptr` in Containers and Standard Library
Using `unique_ptr` with STL Containers
`unique_ptr` can also be stored within Standard Template Library (STL) containers like `std::vector`, enabling efficient and safe resource management:
std::vector<std::unique_ptr<MyClass>> myObjects;
myObjects.push_back(std::make_unique<MyClass>());
This usage pattern helps manage collections of dynamically allocated objects effectively, harnessing the safety features of `unique_ptr`.
Combining `unique_ptr` with Algorithms
Another valuable feature of `unique_ptr` is its compatibility with STL algorithms, allowing for powerful abstractions. For example, you can utilize `std::for_each` with `unique_ptr` in a lambda:
std::for_each(myObjects.begin(), myObjects.end(), [](const std::unique_ptr<MyClass>& obj) {
obj->someMethod(); // Calling a method on MyClass instances
});
This approach combines the safety of smart pointers with the versatility of C++ algorithms, creating clear and readable code.

Advanced Features of `unique_ptr`
Custom Deleters
In some cases, you may need to provide a custom deletion mechanism when the `unique_ptr` is destroyed. This can be achieved with custom deleters, which are functions that define how the resource should be released:
auto customDeleter = [](MyClass* p) { /* custom deletion logic */ delete p; };
std::unique_ptr<MyClass, decltype(customDeleter)> myPtr(new MyClass(), customDeleter);
Using custom deleters allows you to tailor resource management to suit specific requirements, enhancing the flexibility of `unique_ptr`.

Common Use Cases for `unique_ptr`
Resource Management in C++
The primary purpose of `unique_ptr` is to manage resources, particularly in scenarios where one object should control a dynamically allocated resource. For instance, when developing game engines or in scenarios involving file handling, utilizing `unique_ptr` ensures that resources are freed correctly when they are no longer needed.
Implementing RAII with `unique_ptr`
RAII, or Resource Acquisition Is Initialization, is a key paradigm in C++ for resource management. By using `unique_ptr`, you can easily implement RAII principles, ensuring resources are acquired during the object's lifetime and released when the object is destroyed.
For example, if you design a class that manages a resource, you can use `unique_ptr` internally to handle the resource automatically:
class ResourceManager {
private:
std::unique_ptr<ResourceType> resource;
public:
ResourceManager() : resource(std::make_unique<ResourceType>()) {}
// ResourceType will be automatically cleaned up when ResourceManager is destroyed
};

Conclusion
Recap of `unique_ptr` Importance
In summary, `unique_ptr` is a powerful tool in C++ that provides exclusive ownership and automatic resource management. It simplifies complex memory management tasks and prevents memory leaks, making it an indispensable part of modern C++ programming.
Future Considerations
As C++ continues to evolve, staying updated on best practices regarding smart pointers and other memory management techniques is essential. Consider delving deeper into features introduced in C++11 and beyond to enhance your programming skills and create effective C++ applications.

Additional Resources
Recommended Reading and Documentation
For further learning, consult the official C++ documentation and resources available on smart pointers. Books by experts or online courses can provide deeper insights into resource management and advanced C++ techniques.
Examples and Practice Problems
To solidify your understanding, practice writing your own examples that utilize `unique_ptr` and solve various memory management challenges. Engaging in hands-on coding will help reinforce concepts and promote best practices in using `unique_ptr`.