The `auto_ptr` class template in C++ is a smart pointer that provides automatic memory management by transferring ownership of a dynamically allocated object, which was deprecated in C++11 in favor of `unique_ptr` and `shared_ptr`.
Here's a code snippet demonstrating the use of `auto_ptr`:
#include <iostream>
#include <memory>
int main() {
std::auto_ptr<int> ptr1(new int(10)); // Create an auto_ptr
std::auto_ptr<int> ptr2 = ptr1; // Ownership transferred to ptr2
std::cout << *ptr2 << std::endl; // Outputs: 10
// ptr1 is now empty, accessing it leads to undefined behavior
return 0;
}
Understanding `auto_ptr`
What is `auto_ptr`?
`auto_ptr` is a smart pointer type introduced in C++98 that manages dynamically allocated objects. Its primary purpose is to automate memory management, making it easier for developers to handle resource allocation and deallocation without manual intervention. When an `auto_ptr` is created, it takes ownership of a dynamically allocated object, ensuring that the object is automatically deleted when the `auto_ptr` goes out of scope.
Historical Context
`auto_ptr` was one of the first smart pointers available in C++. However, as C++ evolved, significant issues with its semantics became apparent, leading to its deprecation in C++11. Understanding these historical contexts is crucial for modern programming practices, as developers are encouraged to utilize more robust alternatives.
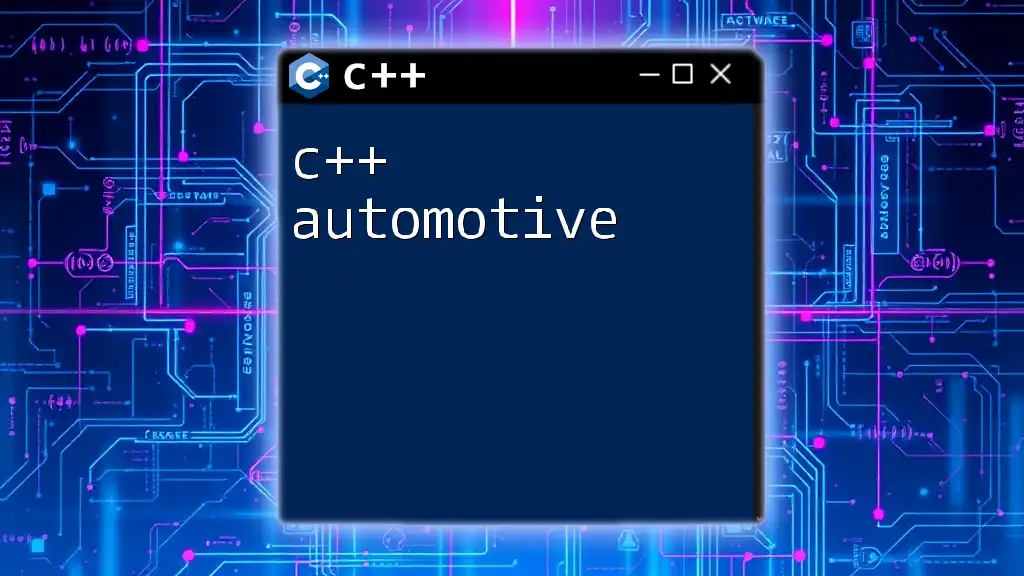
How `auto_ptr` Works
Ownership Semantics
In C++, ownership semantics refer to the management of resources, specifically who is responsible for cleaning up an object. With `auto_ptr`, ownership of an object can be transferred from one smart pointer to another. This transfer means that only one `auto_ptr` can own a particular object at any time.
Basic Syntax
Creating an `auto_ptr` is straightforward:
#include <iostream>
#include <memory>
int main() {
std::auto_ptr<int> ptr1(new int(10));
std::cout << *ptr1 << std::endl; // Output: 10
return 0;
}
In this example, we create an `auto_ptr` named `ptr1` that points to an integer with a value of 10. When `ptr1` goes out of scope, it automatically deletes the allocated memory.
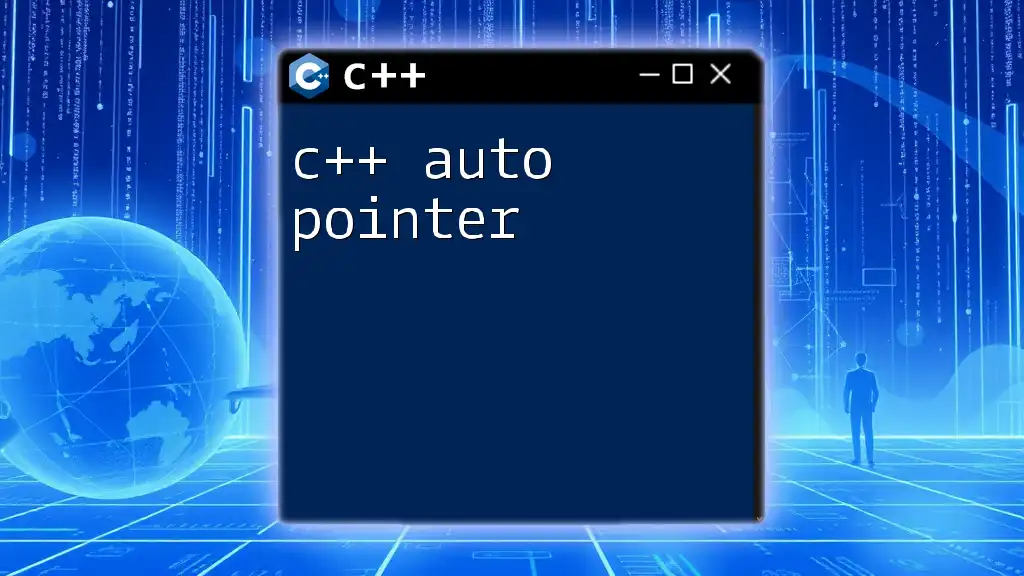
Limitations of `auto_ptr`
Single Ownership
A major limitation of `auto_ptr` is its single ownership model. Unlike some other smart pointers, `auto_ptr` does not support sharing ownership of an object. When an `auto_ptr` is copied, the copy will take ownership and the original pointer will be set to `nullptr`. This behavior can lead to unexpected bugs and must be handled with caution.
Problematic Behavior
Copying `auto_ptr` can lead to a situation where the original pointer becomes invalid, which can potentially cause dangling pointers:
std::auto_ptr<int> ptr1(new int(20));
std::auto_ptr<int> ptr2 = ptr1; // ptr1 is now null
std::cout << (ptr1.get() == nullptr) << std::endl; // Output: 1 (true)
In the above example, after the assignment, `ptr1` is now null, making it impossible to access the original dynamically allocated memory.
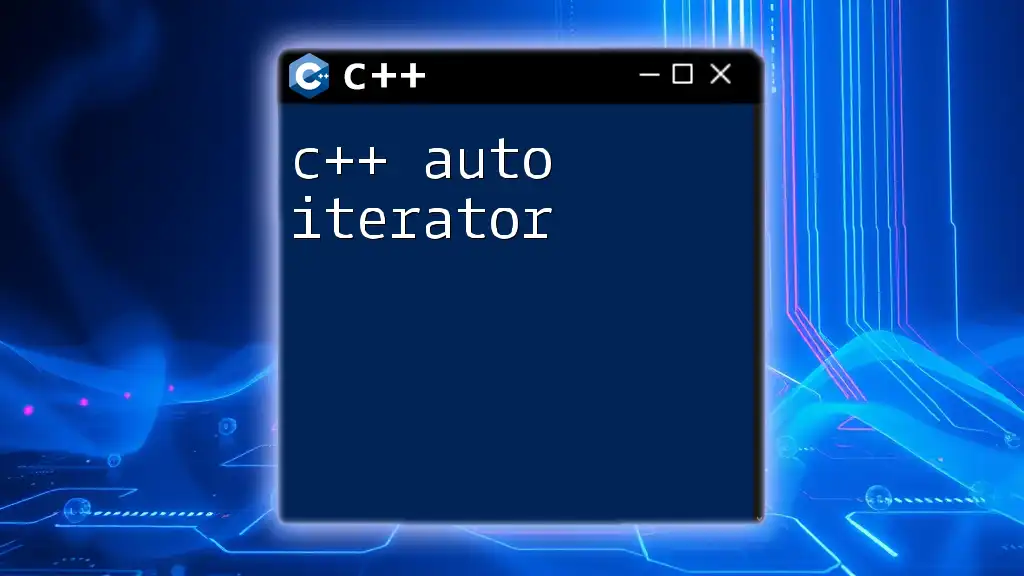
Alternatives to `auto_ptr`
Introduction to Smart Pointers
The introduction of C++11 brought stronger alternatives to `auto_ptr`. Modern C++ emphasizes the importance of smart pointers and introduces two new types: `unique_ptr` and `shared_ptr`. These types offer better management of resource ownership, making them the preferred choices for developers today.
Using `unique_ptr`
`unique_ptr` is a smart pointer that retains sole ownership of an object through a pointer and destroys that object when the `unique_ptr` goes out of scope.
#include <iostream>
#include <memory>
int main() {
std::unique_ptr<int> ptr1(new int(30));
std::cout << *ptr1 << std::endl; // Output: 30
return 0;
}
In contrast to `auto_ptr`, `unique_ptr` cannot be copied; it can only be moved, preventing unintentional copying and dangling pointers.
Comparison with `shared_ptr`
While `unique_ptr` enforces unique ownership, `shared_ptr` allows multiple pointers to share ownership of a single object. This is achieved through reference counting, where the object is only destroyed when the last accompanying pointer to it is released.
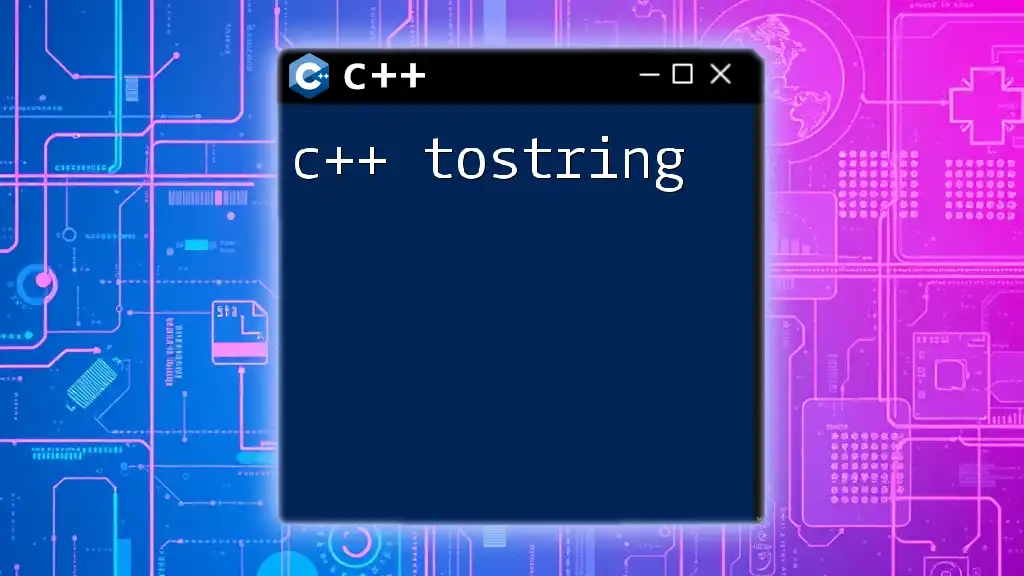
Practical Examples with `auto_ptr`
Simplistic Example
Consider the following example where `auto_ptr` is used to manage the memory of a simple integer:
#include <iostream>
#include <memory>
int main() {
std::auto_ptr<int> myPtr(new int(100));
std::cout << "Value: " << *myPtr << std::endl; // Output: Value: 100
return 0; // Implicitly deletes allocated memory
}
This demonstrates the basic functionality of `auto_ptr`, ensuring that memory management is automated.
Complex Example
In a more complex scenario, `auto_ptr` can be used within classes:
#include <iostream>
#include <memory>
class Test {
public:
Test() { std::cout << "Constructor" << std::endl; }
~Test() { std::cout << "Destructor" << std::endl; }
};
void createObject() {
std::auto_ptr<Test> t(new Test());
// Do some work...
} // Destructor is called automatically here
int main() {
createObject(); // Constructor and Destructor are called
return 0;
}
Here, the constructor and destructor of the `Test` class are automatically called when the `auto_ptr` goes out of scope, showcasing the convenience of automatic resource management.
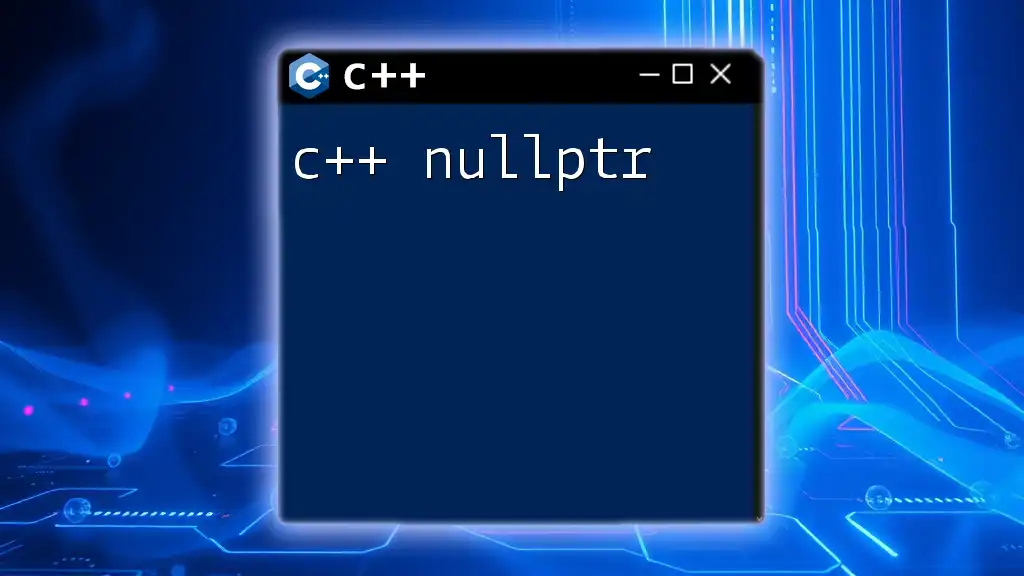
Best Practices in Modern C++
Transitioning from `auto_ptr`
If you encounter `auto_ptr` in legacy code, consider refactoring to modern smart pointers like `unique_ptr` or `shared_ptr`. This transition not only enhances code safety but also ensures maintainability. Evaluate your usage of object ownership and choose the correct smart pointer that fits your needs.
Embracing RAII
Resource Acquisition Is Initialization (RAII) is a programming paradigm where resources are tied to the lifetime of objects. When used correctly, smart pointers align with RAII principles, ensuring that resources are properly released without memory leaks or dangling pointers.
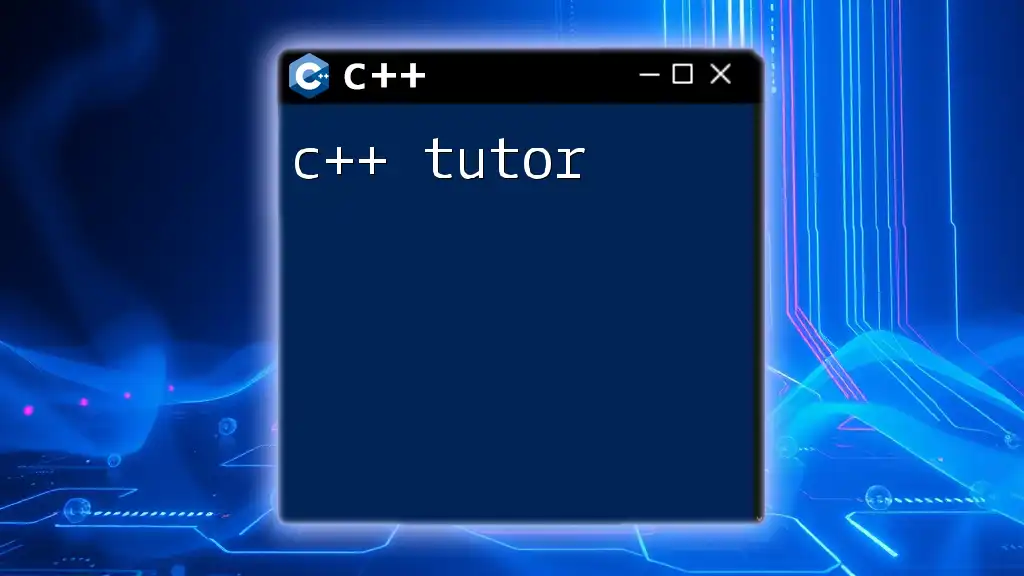
Conclusion
Summary of Key Points
In conclusion, while `c++ auto_ptr` served as an early attempt at smart pointers, its limitations prompted the need for more effective memory management techniques. Both `unique_ptr` and `shared_ptr` offer flexibility and safety that `auto_ptr` lacks.
Final Thoughts
As modern C++ continues to evolve, embracing smart pointers offers significant advantages for developing stable, efficient, and maintainable applications. Transitioning from `auto_ptr` to modern alternatives is essential for any developer focused on best practices in C++. Always remember to choose the appropriate smart pointer based on your specific ownership semantics to allow your code to thrive.
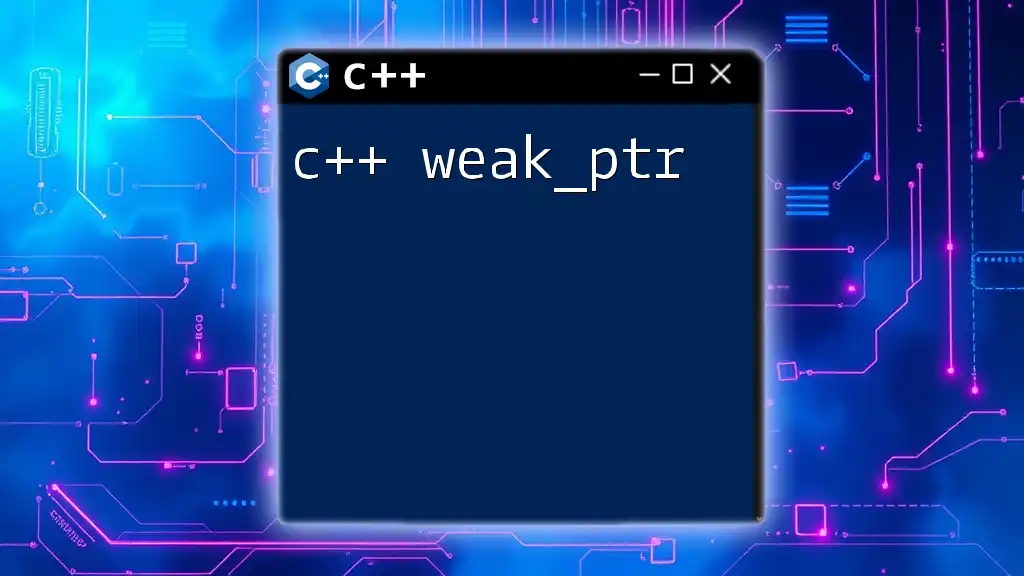
FAQs
Is `auto_ptr` still usable in C++?
Although `auto_ptr` remains in the C++ standards for backward compatibility, its use is highly discouraged in favor of newer alternatives like `unique_ptr` and `shared_ptr`.
What should I use instead of `auto_ptr`?
Developers should utilize `unique_ptr` for exclusive ownership and `shared_ptr` for shared ownership of dynamically allocated objects in order to ensure better safety and resource management in modern C++.