In C++, the comparison operators allow you to evaluate the relationship between two values, such as determining if one value is greater than, less than, or equal to another.
Here's a code snippet demonstrating basic comparison:
#include <iostream>
int main() {
int a = 5, b = 10;
std::cout << (a < b) << std::endl; // Outputs: 1 (true)
std::cout << (a > b) << std::endl; // Outputs: 0 (false)
std::cout << (a == b) << std::endl; // Outputs: 0 (false)
return 0;
}
Understanding Comparison Operators in C++
Comparison operators are fundamental in C++ programming, enabling developers to perform evaluations and make decisions within their code. These operators allow you to compare values and determine relationships between them, which is essential for control flow statements, loops, and functions.
Types of Comparison Operators
- Equality Operator (`==`): Checks if two values are equal.
- Inequality Operator (`!=`): Checks if two values are not equal.
- Greater Than Operator (`>`): Checks if the left operand is greater than the right operand.
- Less Than Operator (`<`): Checks if the left operand is less than the right operand.
- Greater Than or Equal To Operator (`>=`): Checks if the left operand is greater than or equal to the right operand.
- Less Than or Equal To Operator (`<=`): Checks if the left operand is less than or equal to the right operand.
Code Snippet Example for Comparison Operators
int a = 5, b = 10;
if (a == b) {
// Code for equal case
} else if (a < b) {
// Code for a is less than b
} else {
// Code for a is greater than b
}
This snippet illustrates the use of the equality operator and the less than operator to make decisions based on the values of `a` and `b`. Each operator provides a different avenue for controlling program execution, crucial for writing robust and efficient code.
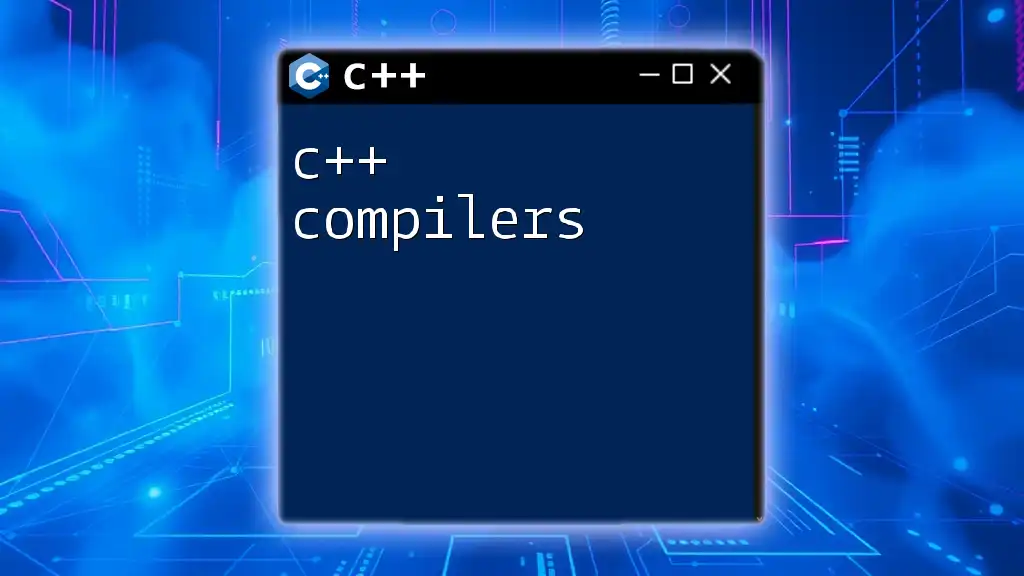
Using Comparison Operators with Different Data Types
In C++, comparison operators can be employed on various types, making them versatile tools in programming.
Comparing Integers
int x = 20, y = 15;
if (x > y) {
// x is greater than y
}
In this case, we simply compare two integers and act accordingly based on their values.
Comparing Floating-Point Numbers
double p = 10.5, q = 10.5;
if (p == q) {
// Code for both values being equal
}
It's crucial to account for precision issues when comparing floating-point numbers. You should often apply a tolerance level rather than direct equality.
Comparing Characters
char char1 = 'a', char2 = 'b';
if (char1 < char2) {
// Code for char1 being less than char2
}
Characters are compared based on their ASCII values, which provides a straightforward method for ordering.
Comparing Strings
Strings in C++ are compared through `std::string`, which allows for lexicographical comparison.
#include <iostream>
#include <string>
std::string str1 = "apple";
std::string str2 = "orange";
if (str1 < str2) {
// Output: "apple is less than orange"
std::cout << str1 << " is less than " << str2 << std::endl;
}
This example illustrates that strings can be compared using relational operators, aiding in sorting and searching operations.
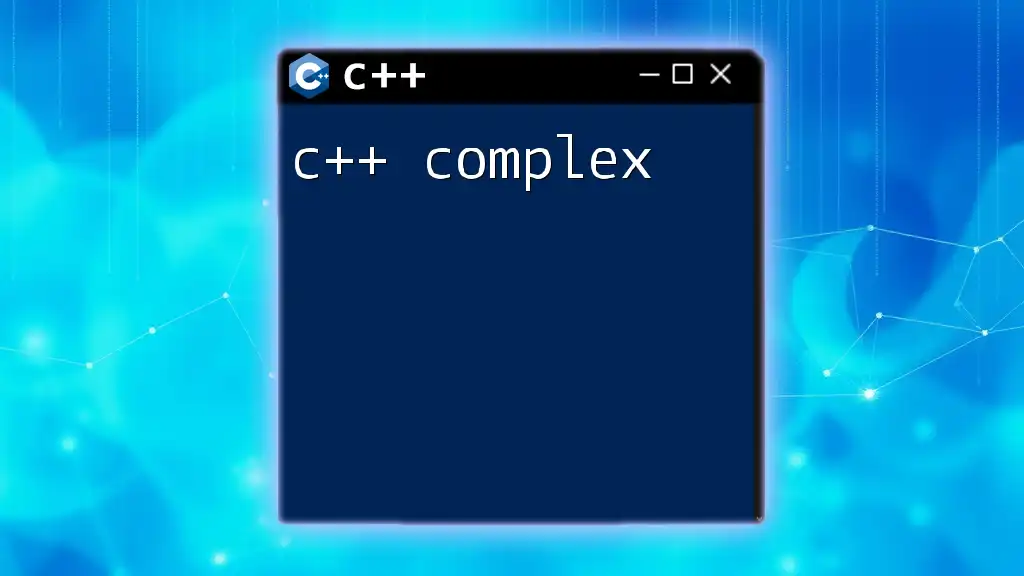
Complex Comparisons: Using Logical Operators
Logical operators enhance the comparison capabilities in C++, allowing for the combination of multiple conditions.
Introduction to Logical Operators
- AND (`&&`): Ensures both conditions are true.
- OR (`||`): Ensures at least one of the conditions is true.
- NOT (`!`): Negates the condition.
Code Snippet Example Using Logical Operators
int a = 5, b = 10;
if (a < b && a != 0) {
// Code for a being less than b and a not being zero
}
In this case, we are ensuring that both conditions are met, which offers a higher level of control in decision-making processes.
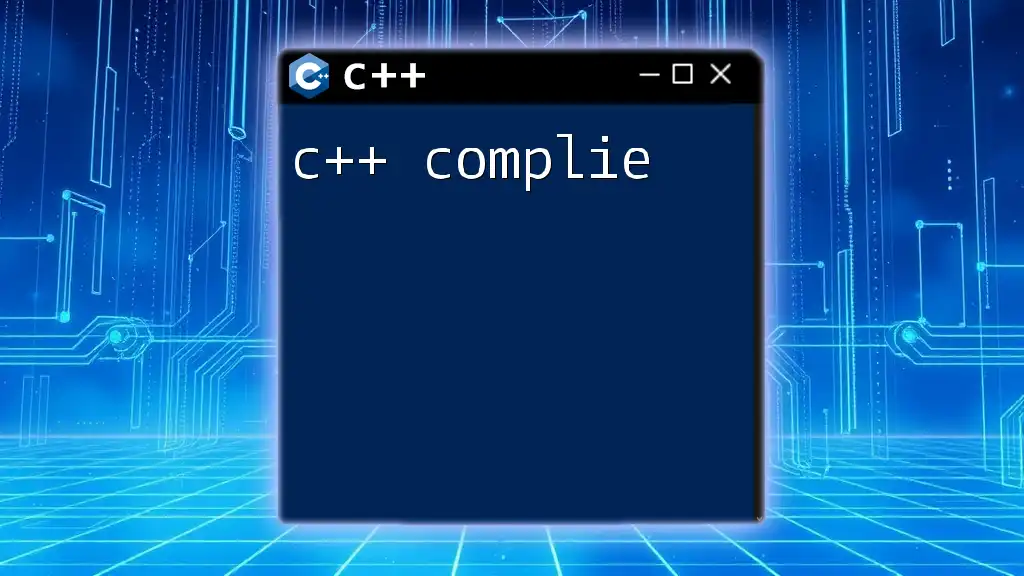
Comparing Pointers and Objects
In C++, pointers and objects can also be compared using overloaded operators.
Pointer Comparisons
You can directly compare pointer values to determine their memory addresses:
int* ptr1 = new int(10);
int* ptr2 = new int(20);
if (ptr1 != ptr2) {
// Pointers point to different addresses
}
However, care must be taken to avoid comparing the values the pointers point to.
Object Comparisons
In many cases, you will want to compare objects. You can achieve this by overloading comparison operators within classes.
Code Snippet Example for Operator Overloading
class Point {
public:
int x, y;
Point(int a, int b) : x(a), y(b) {}
// Overloading the > operator
bool operator>(const Point& p) {
return (x + y) > (p.x + p.y);
}
};
Point p1(3, 4);
Point p2(2, 6);
if (p1 > p2) {
// Code for p1 being 'greater' than p2
}
This code shows how you can define custom behaviors for comparison, enhancing object-oriented design.
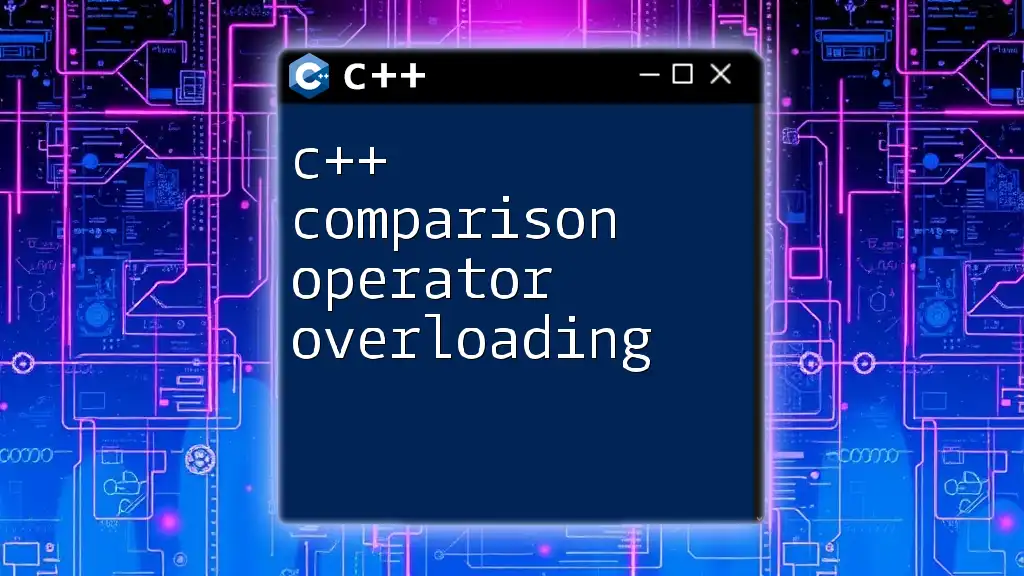
Implementing Comparison in Sorting Algorithms
Comparisons play a crucial role in sorting algorithms, allowing for the organization of data in a desired order. Let's focus on how to implement comparison in standard sorting functions.
Example with Standard Sorting Functions
Using `std::sort`, you can sort a collection based on comparisons.
#include <algorithm>
#include <vector>
bool compare(int a, int b) {
return a < b; // Ascending order
}
int main() {
std::vector<int> vec = {5, 3, 8, 1};
std::sort(vec.begin(), vec.end(), compare);
// Now vec is sorted
}
Here, we define a custom comparison function to control the sorting order. This flexibility allows programmers to sort complex data structures easily.
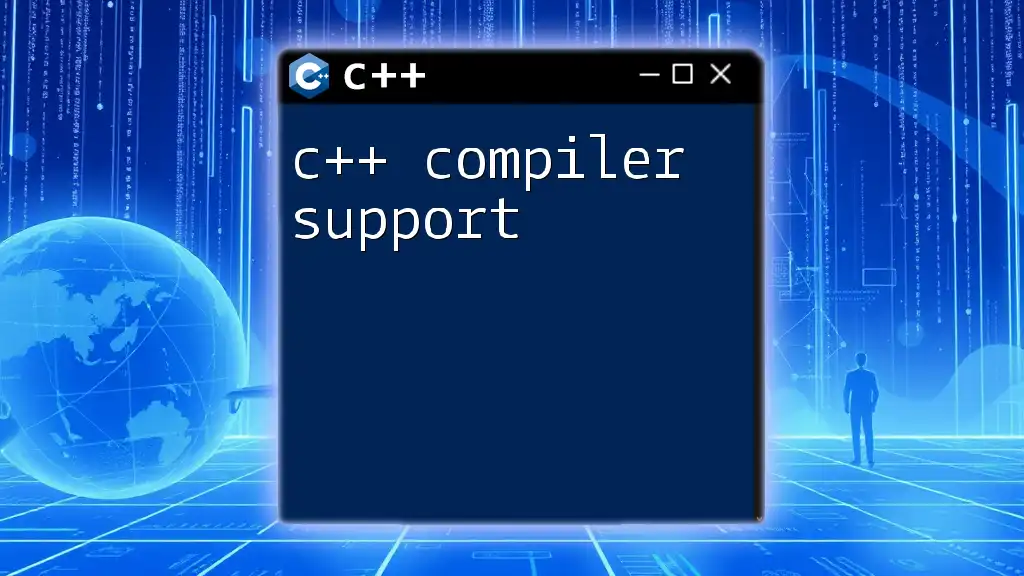
Conclusion: Mastering C++ Comparisons
Understanding and mastering comparisons in C++ is essential for effective programming. By utilizing comparison operators, logical evaluations, and custom overloads, developers can enhance their code's functionality and readability.
The principles discussed in this guide serve as the foundation for more complex programming concepts, so practicing with various examples will solidify your understanding of how to effectively implement comparisons in your C++ projects.
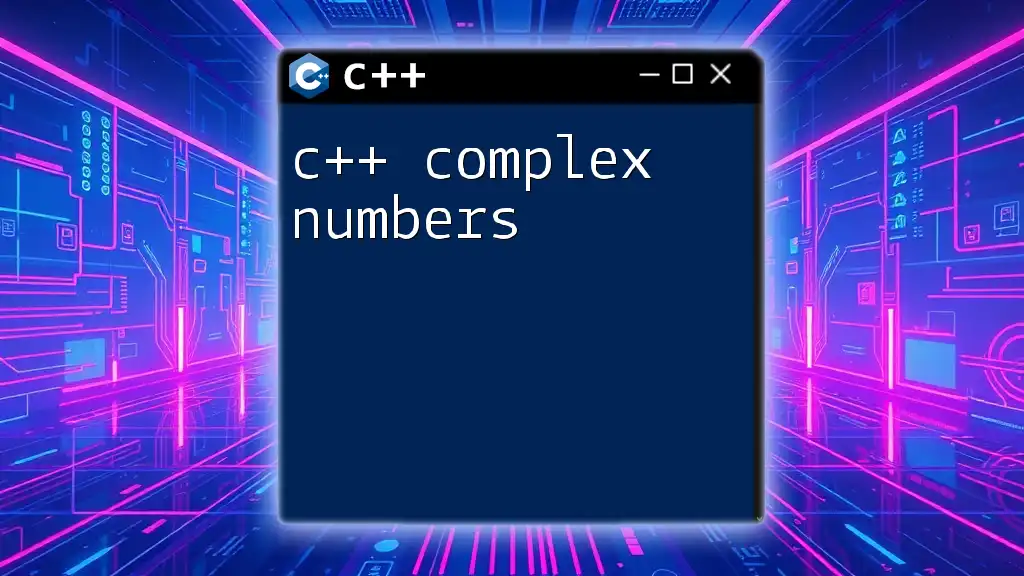
Additional Resources
To further enhance your skills, consider exploring books on C++ programming, online coding platforms, and community forums where you can ask questions and share knowledge. With continuous learning, you'll become adept at using comparisons in a variety of programming contexts.