The GDB (GNU Debugger) is a powerful tool that helps developers debug C++ programs by allowing them to inspect the state of a running program, track down bugs, and control program execution.
Here’s a basic example of how to compile a C++ program and use GDB to run it:
g++ -g my_program.cpp -o my_program # Compile with debugging information
gdb ./my_program # Start GDB with the compiled program
What is GDB?
GDB, or GNU Debugger, is a powerful tool for debugging programs written in C and C++. It allows developers to see what is occurring inside a program while it executes, or what it was doing at the moment it crashed. Understanding how to use GDB can be a crucial skill for any C++ programmer, as it provides insights that are integral to the software development process.
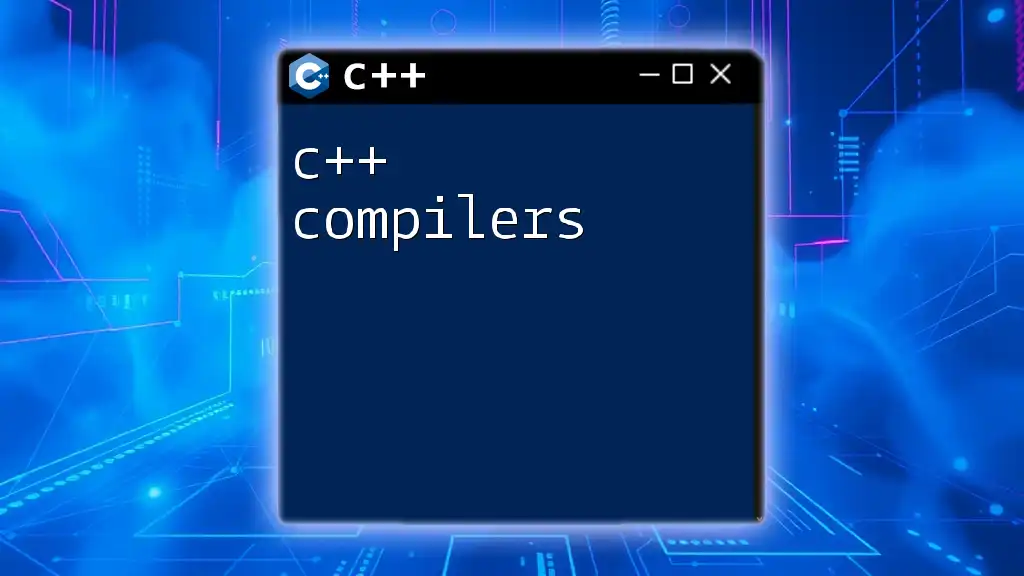
Why Use GDB for C++?
Using GDB comes with several advantages, especially when working with C++. Notably, GDB allows you to:
- Debug C++ programs at runtime: You can inspect variables, modify program execution, and even manipulate memory.
- Handle complex scenarios: The debugger can manage multithreaded applications, enabling you to trace issues across concurrent processes.
- Gain visibility: GDB offers detailed information regarding the program's state, especially when dealing with crashes or unexpected behaviors.
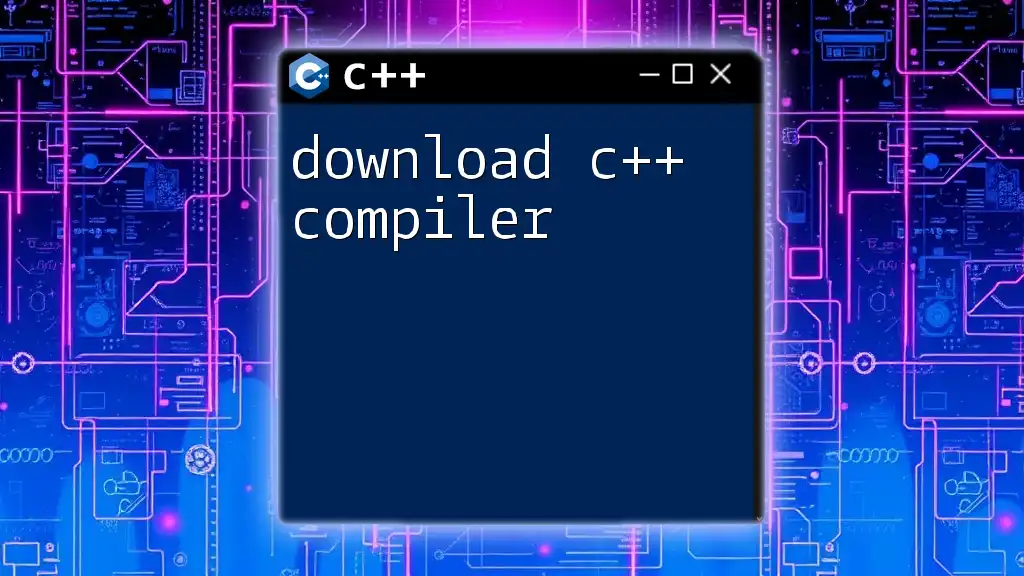
Setting Up GDB with C++
Installing GDB
Installing GDB is the first step to harnessing its power. The installation process varies depending on the operating system:
- Linux: Usually, GDB can be installed from the package manager. For Debian-based distributions, you can use:
sudo apt-get install gdb
- Windows: GDB can be installed as part of MinGW or Cygwin environments. Follow the installation instructions for either package.
- macOS: If you have Homebrew installed, run:
brew install gdb
To verify the installation, you can check the version of GDB by running:
gdb --version
Basic Configuration
Configuring GDB for an optimal debugging experience is essential. After installation, you can configure preferences called `.gdbinit`. This file can be placed in your home directory and can contain custom settings to enhance your debugging workflow, such as disabling pagination or setting up shorthand for commands.
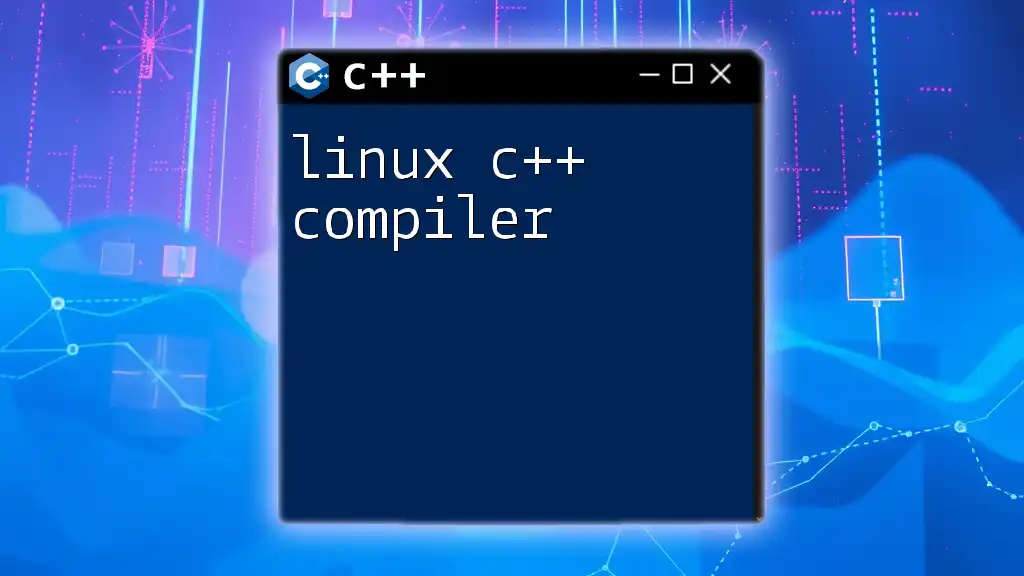
Compiling C++ Code with GDB
Pre-requisites for Using GDB
Before you can use GDB to debug your programs, you need to ensure that your C++ code is compiled with debugging information. This is accomplished by using the `-g` option when invoking the g++ compiler.
Example Compilation Command
g++ -g -o myprogram myprogram.cpp
Using the `-g` flag means GDB can provide more detailed information and allows for a more effective debugging session.
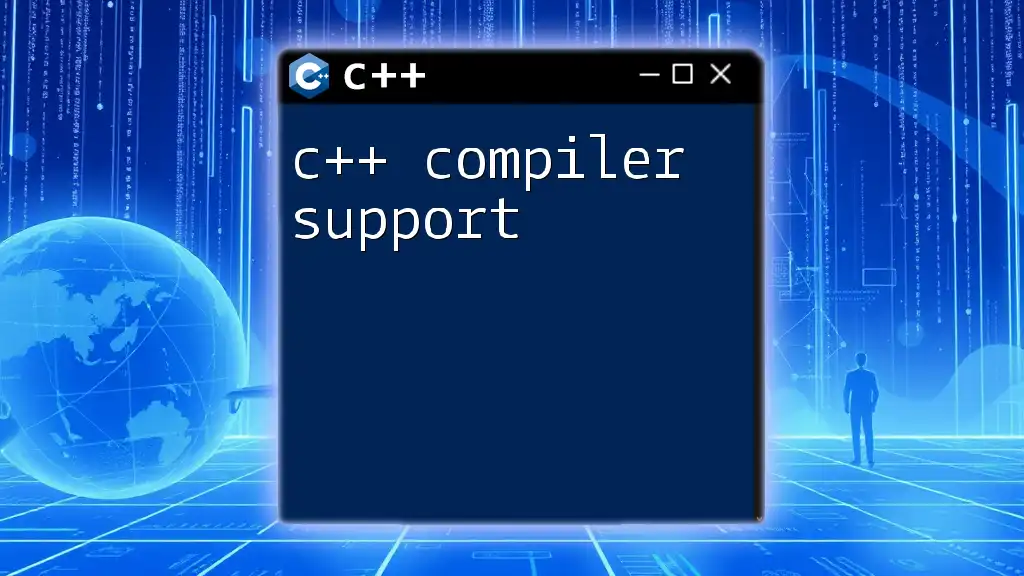
Starting GDB
Launching GDB
Once your program is compiled, you can start GDB. The command is straightforward. Navigate to the directory containing your compiled binary and run:
gdb ./myprogram
Understanding the GDB Prompt
After launching GDB, you will encounter a prompt that signifies GDB is ready to accept commands. The prompt typically looks like this:
(gdb)
Familiarizing yourself with the prompt and basic commands is an essential first step.
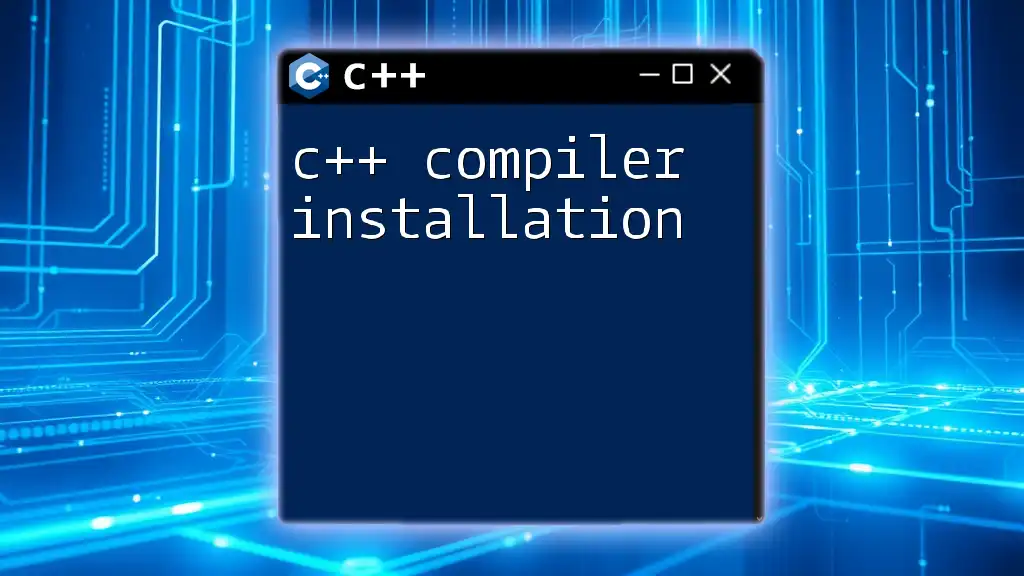
Essential GDB Commands for C++ Debugging
Setting Breakpoints
Breakpoints are fundamental for debugging, allowing you to pause execution at specified points in your program. This enables you to investigate the state of your program at critical junctures.
To set a breakpoint at the start of the `main` function, use:
break main
You can also set breakpoints at specific lines or functions, providing flexibility in your debugging process.
Running the Program
To execute your program within GDB, you use the `run` command. If your program takes command-line arguments, you can pass them directly:
run arg1 arg2
Navigating through Code
Step Commands
- `step`: This command lets you step into functions, providing a thorough look at each function's execution line by line:
step
- `next`: Differently, this command will execute the next line of code but will not step into any functions:
next
Continuing Execution
If you have paused execution and want to resume, the `continue` command is used:
continue
This will continue executing until the next breakpoint or the program finishes.
Inspecting Variables
One of the powerful features of GDB is the ability to inspect and manipulate variables. The `print` command allows you to check the current state of variables:
print myVariable
You can also modify variables on the fly, which can be invaluable for testing different scenarios without recompiling.
Examining the Call Stack
Understanding the call stack is critical for tracking the flow of execution through functions. The command used to view the call stack is:
backtrace
This will show you the function calls that led to the current point in execution, helping identify where issues may arise.
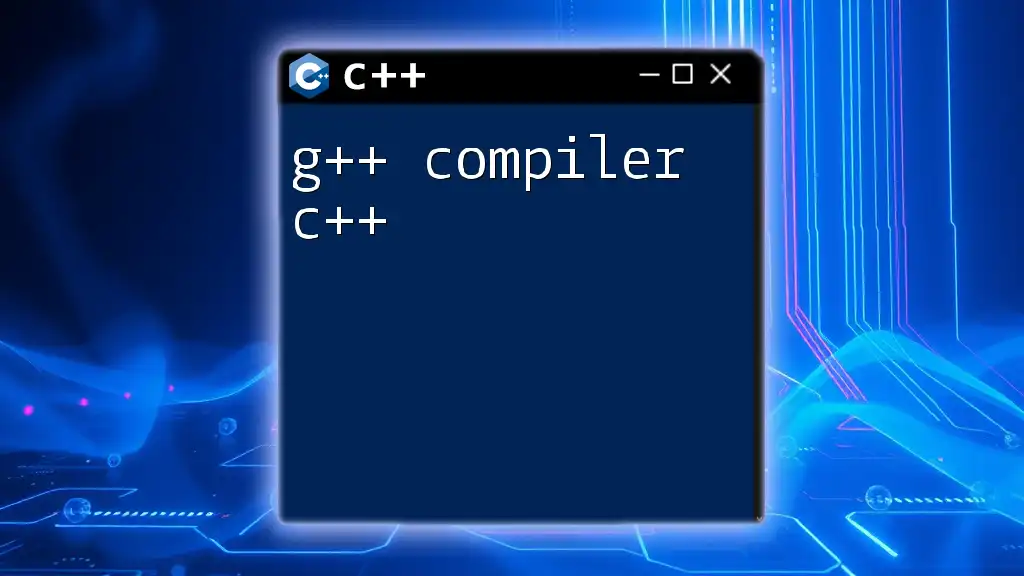
Advanced GDB Features
Debugging Multithreaded Programs
When dealing with multithreaded applications, GDB offers commands to manage and switch between threads, making it easier to pinpoint issues specific to concurrency. You can list threads using:
info threads
Conditional Breakpoints
Conditional breakpoints allow you to stop program execution only when certain conditions are met. This is particularly useful for isolating issues that might not occur on every run:
break myFunction if myVariable == 5
Watchpoints
Watchpoints are unique and allow GDB to stop execution when the value of a variable changes. This is tremendously helpful when trying to locate bugs associated with variable modifications:
watch myVariable
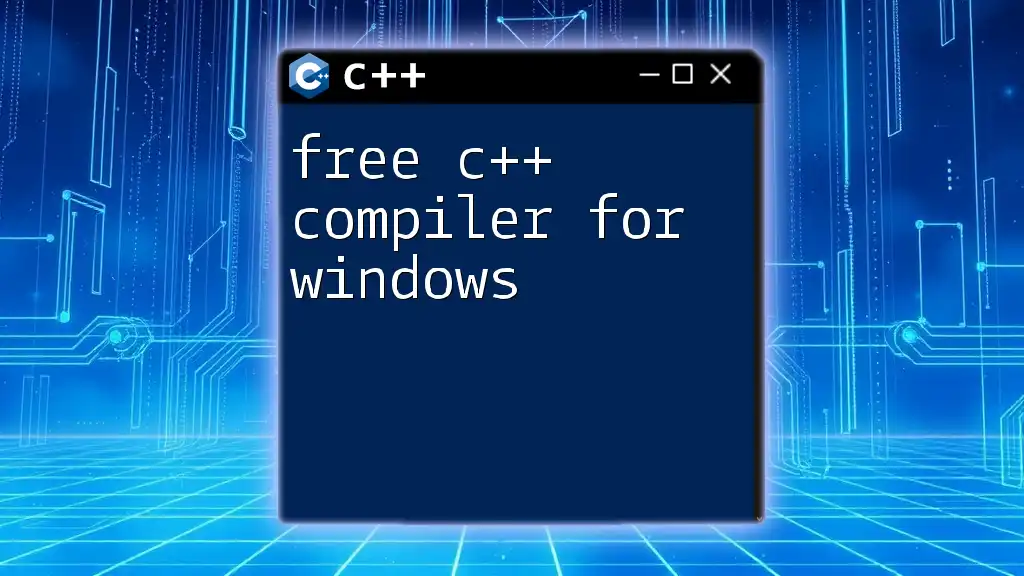
Best Practices When Using GDB
Efficient Debugging Tips
Maximizing your GDB experience often involves combining commands and understanding your code's structure. Leveraging commands like `info locals` can be beneficial to quickly view all local variables.
Using GDB with Integrated Development Environments (IDEs)
Many IDEs, including Visual Studio Code and Eclipse, integrate GDB for a more visual debugging experience. Configuring your IDE to use GDB allows you to set breakpoints, inspect variables, and manage program execution directly from the development environment.
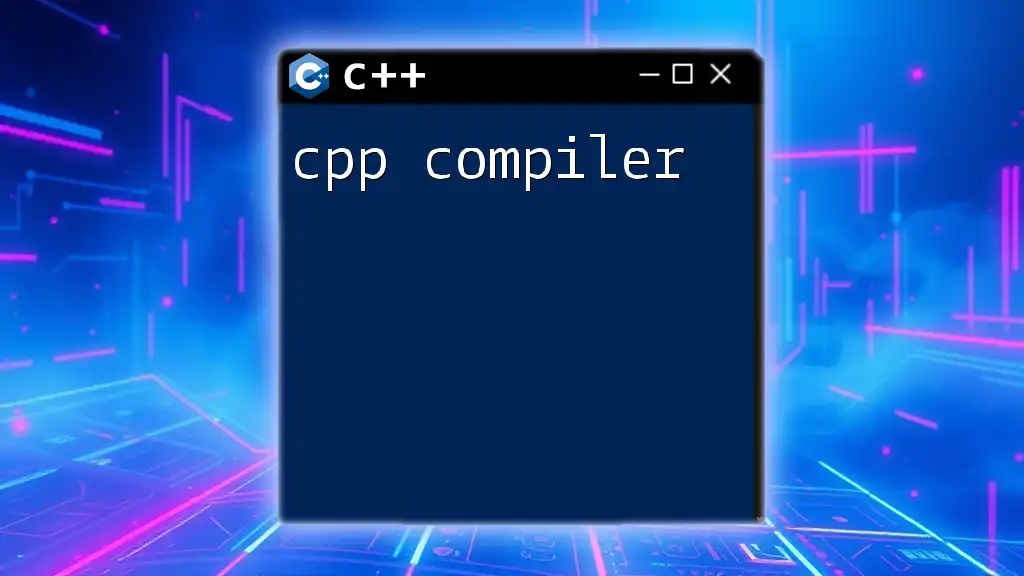
Troubleshooting Common GDB Issues
Common Errors and Resolutions
Debugging is rarely straightforward, and users may encounter common issues such as:
- Program not compiling with `-g` flag: Ensure your build command includes the `-g` option.
- GDB crashes on certain commands: Update GDB or check your source for known compatibility issues.
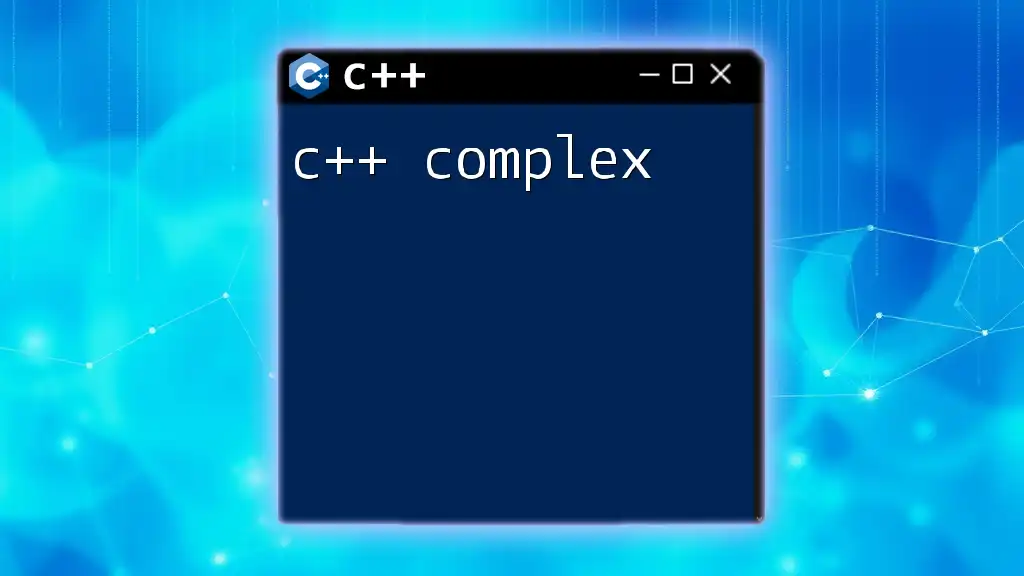
Conclusion
GDB is an essential tool for C++ developers, enhancing the debugging process and providing deep visibility into program execution. Familiarizing yourself with its command set, including breakpoints, variable inspection, and advanced features, can significantly improve your productivity and effectiveness in debugging.
Encouragement to Practice
Mastering GDB takes time and practice, but the reward is an increased understanding of your program's behavior. Experiment with the various commands in your debugging sessions to become proficient and confident in resolving complex issues.
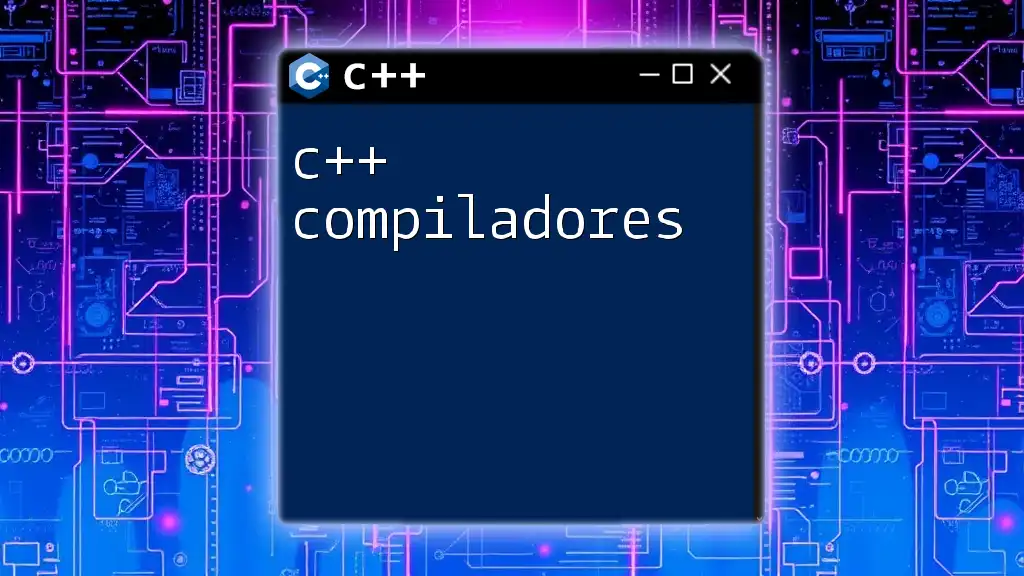
Additional Resources
For further learning, consider exploring recommended books and online courses that delve deeper into GDB and debugging techniques in C++. These resources can provide structured insights and advanced strategies for effective programming in C++.