To install a C++ compiler, you can use the following command based on your operating system, such as using `g++` from the GNU Compiler Collection on Ubuntu.
sudo apt install g++
Understanding C++ Compilers
What is a C++ Compiler?
A C++ compiler is a specialized program that translates C++ code, which is human-readable, into machine code that a computer can execute. This translation process involves several key steps: preprocessing, compiling, assembling, and linking. Understanding this procedure is crucial for anyone engaged in C++ programming. Without a properly installed C++ compiler, writing code is futile as the system won't be able to understand and execute it.
Types of C++ Compilers
C++ compilers come in various types, broadly categorized into open-source and commercial compilers.
-
Open Source Compilers: These are freely available and include the likes of GCC (GNU Compiler Collection) and Clang. They are typically community-supported and regularly updated with new features and bug fixes, making them suitable for many projects.
-
Commercial Compilers: These include options like Microsoft Visual C++ and Intel C++ Compiler. They often come packed with additional features such as optimized performance and better debugging tools, but usually require a purchase.
Selecting the right compiler is pivotal based on your specific needs; beginners might prefer open-source options due to the availability of extensive documentation and community support.
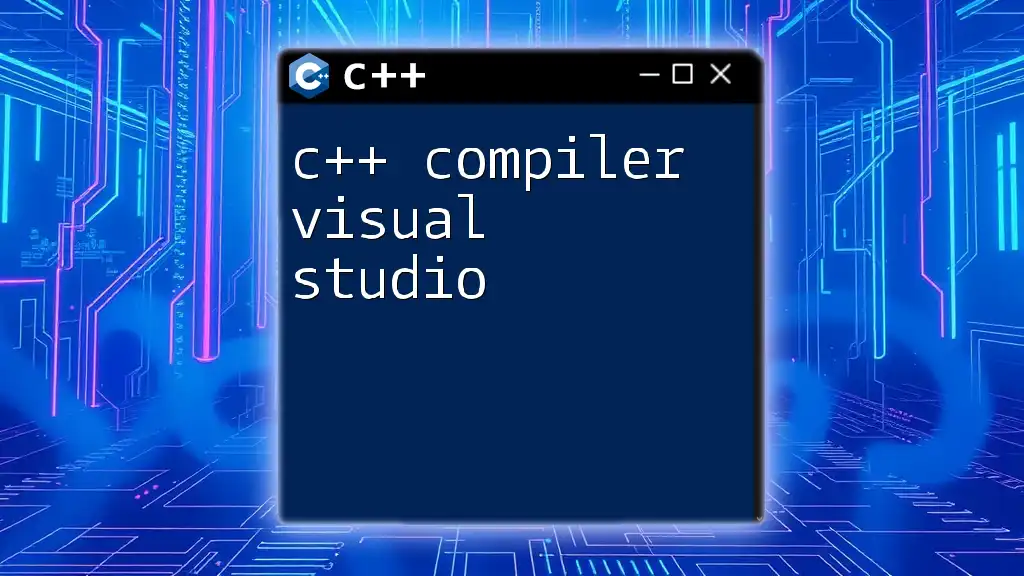
Preparing for Installation
System Requirements
Before diving into C++ compiler installation, it's essential to ensure your system meets the necessary requirements. Most compilers support multiple operating systems, including Windows, macOS, and Linux. Here are some common requirements:
- Operating Systems Supported: Windows 10 or later, macOS Sierra or later, various distributions of Linux (like Ubuntu, Fedora, etc.).
- Hardware Requirements: Generally, you will need at least 2GB of RAM and sufficient disk space to accommodate the compiler and tools, ideally around 1GB for installation.
Choosing a Compiler
Selecting a compiler can impact your development experience. Here’s a brief overview of popular choices:
- GCC (GNU Compiler Collection): Highly versatile and widely used in open-source environments.
- Clang: Known for its fast compilation and superior error messages, making it a favorite among developers.
- Visual Studio: Recommended for Windows users, it integrates both the compiler and the IDE, providing a comprehensive development experience.
Consider your programming goals and existing setup when choosing a compiler, as ease of installation and usability will vary.
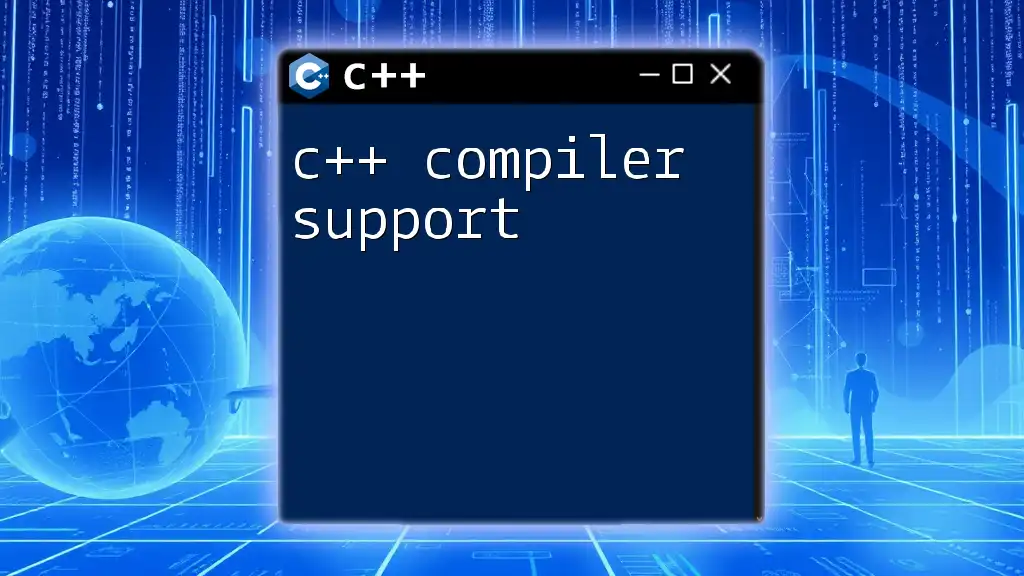
C++ Compiler Installation on Windows
Installing Microsoft Visual C++
Microsoft Visual C++ is part of the Visual Studio suite and provides one of the most robust environments for C++ development on Windows. Here’s how to install it:
-
Download the Installer: Visit the official Visual Studio website and download the installer for the Community version, which is free for individual developers and students.
-
Selecting the Workload: Run the installer. In the setup screen, select “Desktop development with C++.” This option includes all necessary components to start working with C++.
-
Complete the Installation: Click on “Install” and allow the process to complete. Depending on your internet speed, this may take a while.
-
Configuration After Installation: Following installation, you may need to set up environment variables. You might also run a simple program to ensure everything is in order.
Example Code Snippet
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Once you compile and run this code, you should see "Hello, World!" printed in your console. If you do, congratulations—your Visual C++ compiler is successfully installed!
Installing GCC using MinGW
If you prefer to use GCC on Windows, MinGW (Minimalist GNU for Windows) is an excellent option. Here's how to install it:
-
Downloading the Installer: Go to the MinGW official website, and download the MinGW installer.
-
Installing Components: Run the installer. During the installation, ensure that you select essential components, such as `g++` (for C++) and `mingw32-make`.
-
Setting Up the PATH Environment Variable: After the installation, you’ll need to add the MinGW `bin` directory to the PATH environment variable so that you can run commands from any command prompt.
-
Testing the Installation: To ensure that MinGW is installed correctly, create a simple C++ program and compile it.
Example Code Snippet
#include <iostream>
int main() {
std::cout << "Hello from MinGW." << std::endl;
return 0;
}
Compile this using the command `g++ hello.cpp -o hello.exe`, then run it with `./hello.exe`.
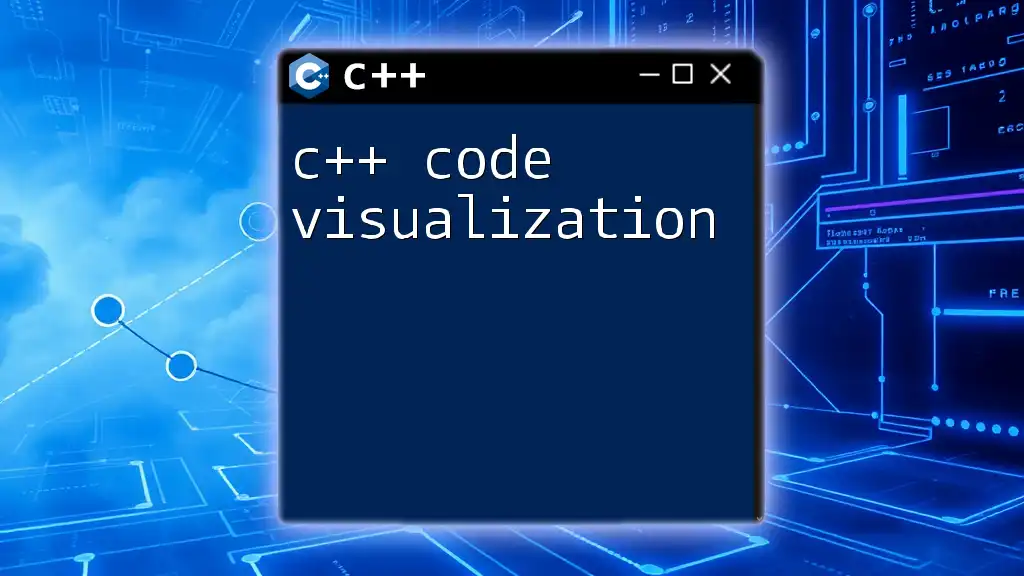
C++ Compiler Installation on macOS
Installing Xcode Command Line Tools
For macOS users, installing the Xcode Command Line Tools is the most straightforward way to get a C++ compiler. Here's how to do it:
-
What is Xcode?: Xcode is Apple's suite of software development tools, which includes compilers.
-
Installation Steps: Open the terminal and enter the command:
xcode-select --install
-
Prompt for Installation: A dialog box will appear to confirm the installation. Follow the instructions to complete the process.
-
Configuration Overview: After installation, test if GCC has been successfully installed by running:
gcc --version
Example Code Snippet
#include <iostream>
int main() {
std::cout << "Welcome to Xcode!" << std::endl;
return 0;
}
Compile and run this program to verify that everything is working as expected.
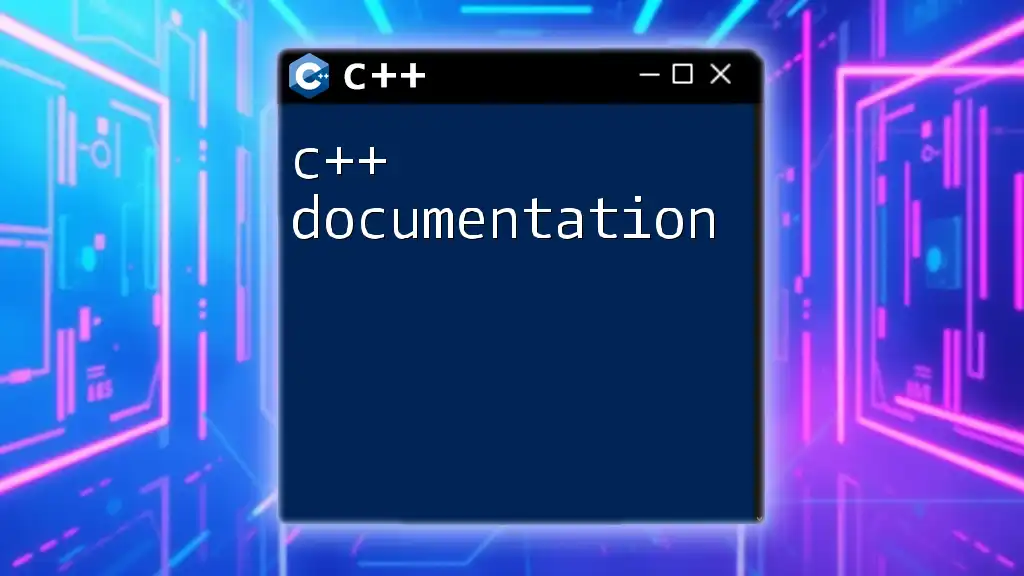
C++ Compiler Installation on Linux
Installing GCC
On Linux systems, installing GCC is often as simple as using a package manager. Different distributions use different package managers, but here’s a general approach:
-
Using Package Managers: Depending on the Linux distribution you’re using, run one of the following commands:
- For Ubuntu or Debian-based systems:
sudo apt-get install build-essential
- For Fedora:
sudo dnf install gcc gcc-c++
- For CentOS:
sudo yum install gcc gcc-c++
-
Verifying the Installation: After installation, verify it by running:
gcc --version
-
Configuration Tips: For enhanced usability, ensure you have a text editor or IDE installed to write your C++ code easily.
Example Code Snippet
#include <iostream>
int main() {
std::cout << "Running GCC on Linux" << std::endl;
return 0;
}
Compile it with `g++ hello.cpp -o hello`, and run it using `./hello`.
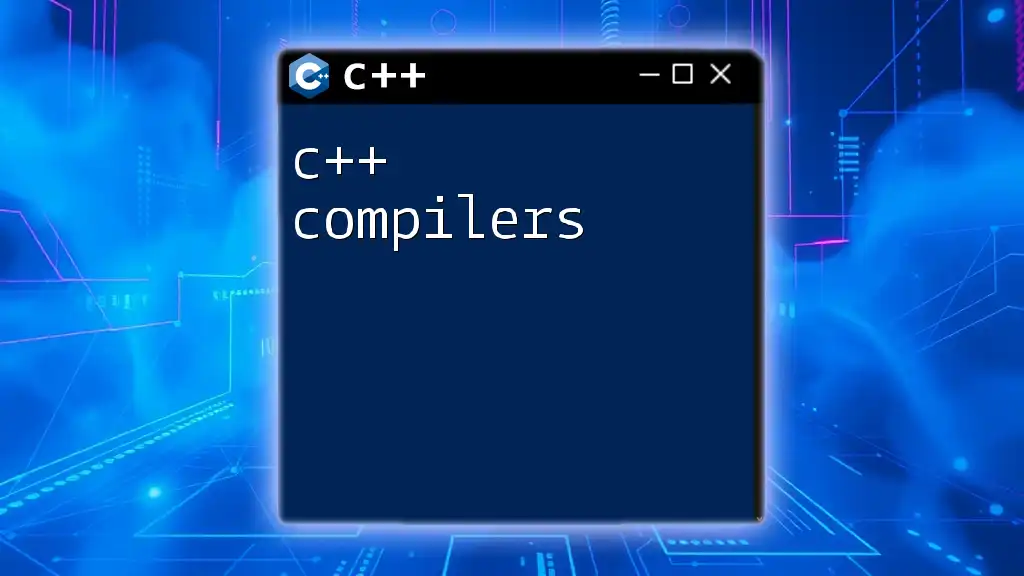
Common Installation Issues
Troubleshooting Installation Problems
Errors can occur during the installation process. Here are some common issues and how to resolve them:
-
Compiler Not Found: If you encounter "command not found" errors after installation, this usually indicates that the PATH environment variable isn’t set correctly. Check the PATH settings and ensure it includes your compiler's binary directory.
-
Compilation Errors: If you see messages when trying to compile code, closely examine the error messages. Common issues might include syntax errors in your code or missing libraries. Google specific error messages for targeted solutions.
FAQs
-
What is the best C++ compiler for beginners? Many beginners find GCC or Clang to be the most accessible due to their extensive documentation and community support.
-
How to update an existing compiler installation? Keep an eye on the official websites of your chosen compiler for updates and follow the specific update instructions provided.
-
Can I use multiple compilers on the same system? Yes, it is entirely possible to install multiple C++ compilers on one system. Just ensure each one is properly configured with its PATH settings.
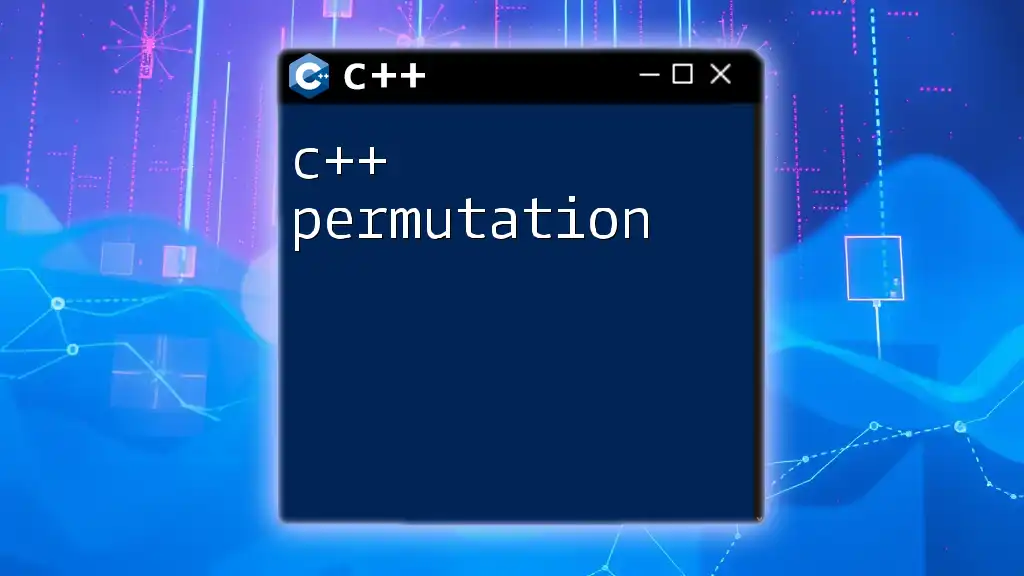
Conclusion
Installing a C++ compiler is the first step towards entering the exciting world of C++ programming. With everything set up, you can now start coding, debugging, and developing your software. Your choice of compiler can significantly affect your coding experience, so choose wisely based on your needs.
Explore more tutorials and resources available to strengthen your understanding of C++. Dive deep, experiment, and most importantly, enjoy coding in C++!
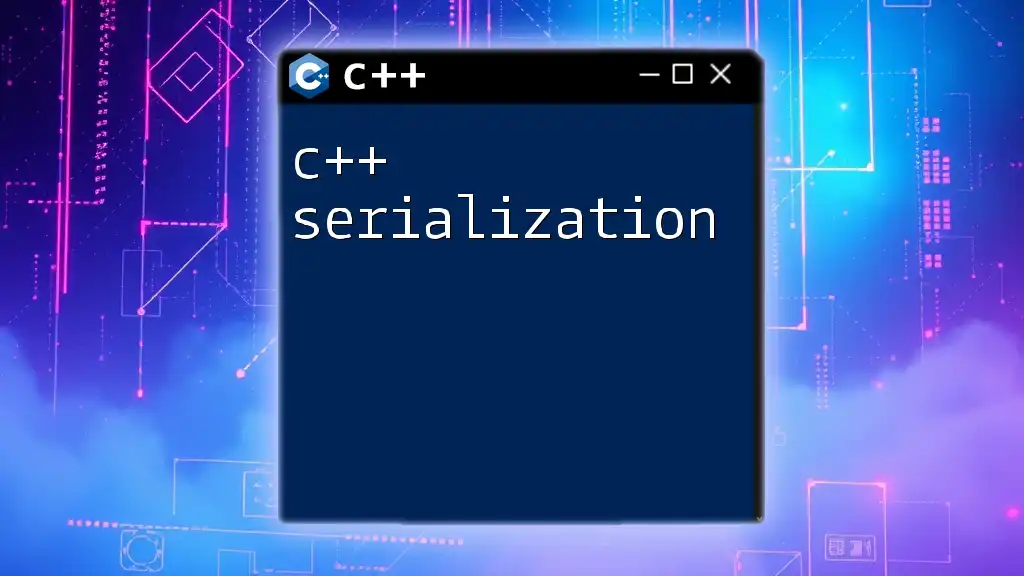
Additional Resources
For further information, refer to the official documentation of the compilers discussed above and consider investing some time in books or online courses to deepen your C++ knowledge.
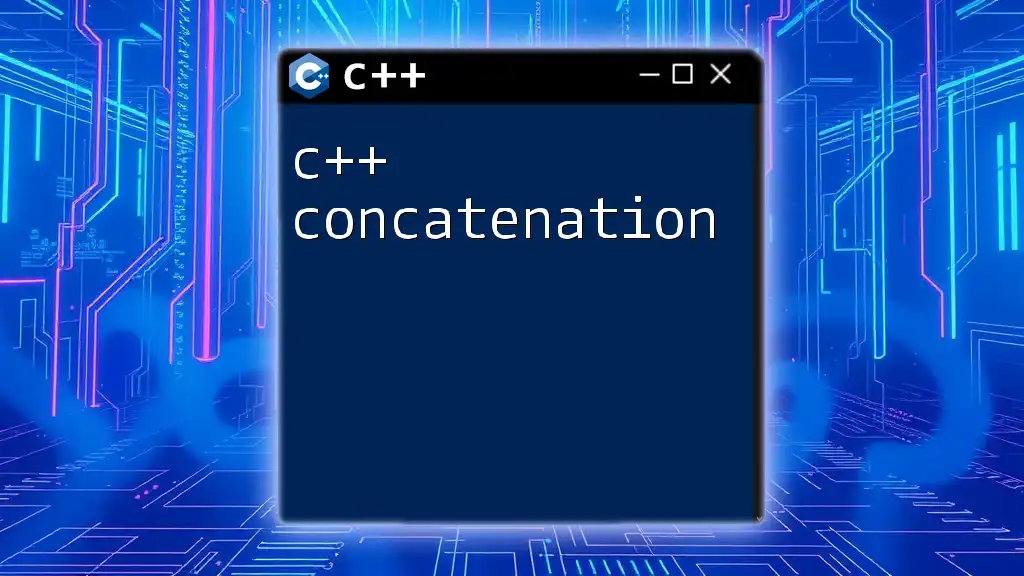
Call to Action
Sign up for our upcoming courses on C++ programming and compiler usage! Stay connected and enhance your coding skills with concise and effective learning.