C++ braced initialization allows you to initialize variables, containers, and objects using curly braces to create a more uniform and consistent syntax, minimizing issues like narrowing conversions.
Here’s a code snippet demonstrating braced initialization:
#include <iostream>
#include <vector>
int main() {
// Braced initialization of a variable
int a{5};
// Braced initialization of a vector
std::vector<int> vec{1, 2, 3, 4, 5};
std::cout << "Variable a: " << a << std::endl;
std::cout << "Vector elements: ";
for (const auto& element : vec) {
std::cout << element << " ";
}
return 0;
}
The Evolution of Initialization Methods
C++ has a rich history of initialization techniques. Historically, developers often relied on traditional methods, such as assignment and constructor calls. For instance:
int value = 10; // Assignment initialization
With the advent of C++11, braced initialization was introduced as a more robust and consistent approach, aiming to simplify the initialization process while avoiding common programming pitfalls.

Understanding C++ Brace Initializer
Syntax and Usage
The syntax of C++ braced initialization is straightforward and intuitive. It utilizes curly braces `{}` to denote the values being assigned during initialization:
int value{10}; // Basic braced initialization
std::vector<int> vec{1, 2, 3, 4}; // Initializing a vector
Types of Braced Initialization
Direct Initialization
Braced initialization can be applied directly when creating objects, offering a clear and concise way to set values:
struct Point { int x, y; };
Point p{1, 2}; // Direct initialization of Point
Copy Initialization
This method also supports copy initialization, where the objects are created using the assignment operator:
Point p = {3, 4}; // Copy initialization
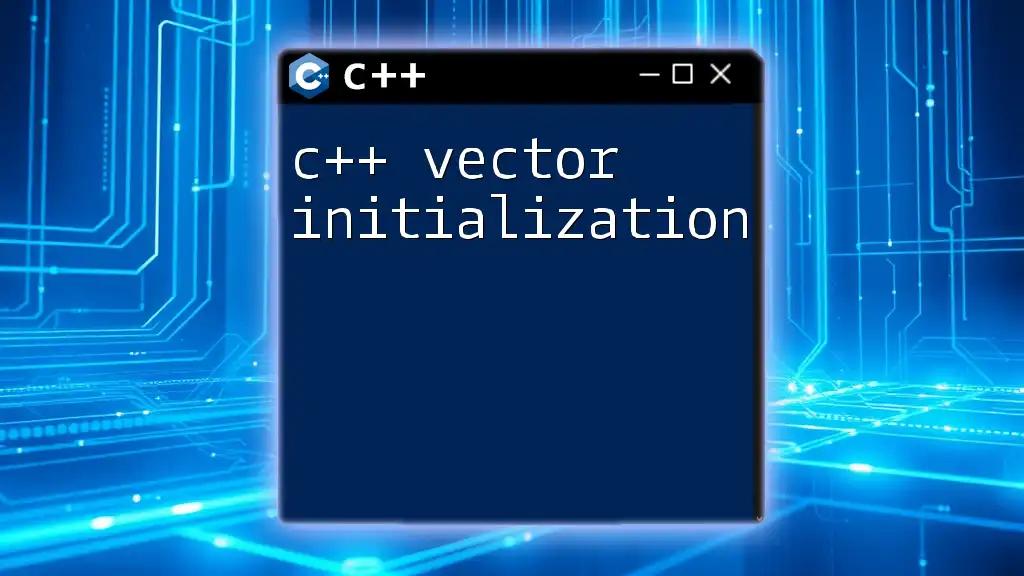
Advantages of C++ Brace Initialization
Avoiding Narrowing Conversions
One of the standout features of C++ braced initialization is its capacity to prevent narrowing conversions. Narrowing conversions occur when data is converted from a wider type to a narrower type, potentially leading to data loss. This is exemplified in the following code snippet:
int a{3.5}; // Error: narrowing conversion
Here, attempting to initialize an integer with a floating-point number results in a compilation error, alerting the programmer to potential issues before runtime.
Consistency and Readability
Braced initialization contributes to a more uniform style of writing initialization code, enhancing both consistency and readability. By using a standardized format, developers can quickly understand the variable's intent without the ambiguity that sometimes accompanies traditional initialization methods.
Aggregate Initialization
Braced initialization simplifies the initialization of aggregates such as arrays and structs. Consider the following example:
struct Point {
int x;
int y;
};
Point pt{1, 2}; // Aggregate initialization
In this case, the struct `Point` is initialized seamlessly using braces, making the code cleaner and more expressive.

C++ Brace Initialization with Containers
Using Braced Initialization with STL Containers
The power of braced initialization extends beyond basic types, making it particularly useful with STL containers. Here are a few examples showcasing this utility:
std::array<int, 3> arr{1, 2, 3}; // Array initialization
std::set<int> s{5, 6, 7}; // Set initialization
These constructs offer an elegant way to set up container data structures without the need for additional boilerplate code.

Understanding List Initialization and its Variants
List Initialization Explained
Under the umbrella of braced initialization lies the concept of list initialization. This method is especially practical when initializing arrays and aggregates with compatibility and type constructors being automatically resolved.
Uniform Initialization
Uniform initialization further consolidates the purpose of braced initialization by providing a consistent syntax that applies across multiple types of variable declarations. This is a hallmark of modern C++.
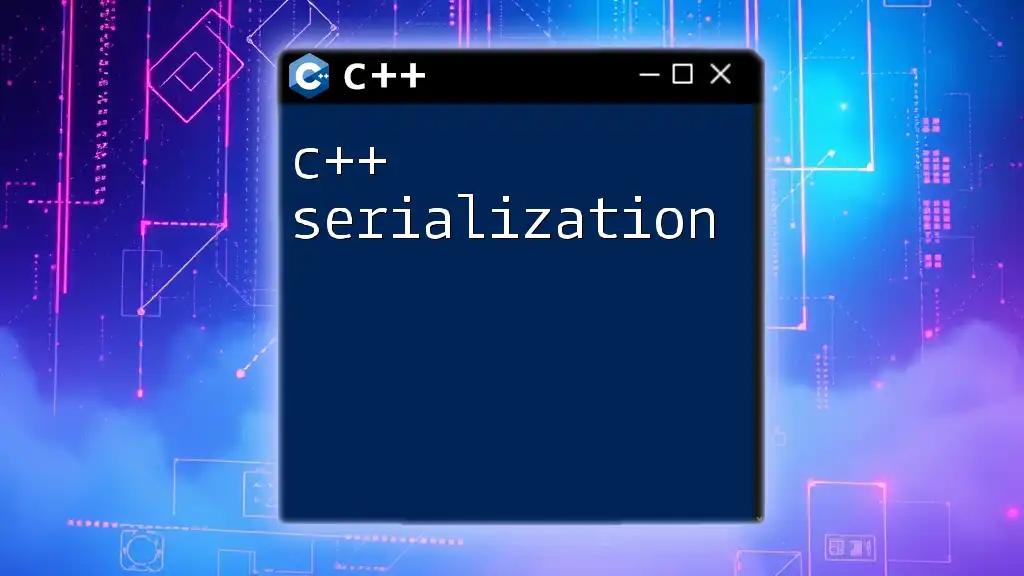
Common Pitfalls with Braced Initialization
While C++ braced initialization has numerous advantages, it is essential to recognize potential pitfalls.
Ambiguity in Overloaded Constructors
One common source of confusion occurs when using braced initialization with overloaded constructors. Depending on how constructors are defined, it may lead to ambiguity that can be difficult to debug.
Important Rules to Remember
Keep in mind the following rules when utilizing braced initialization:
- Use braces to avoid narrowing conversions explicitly.
- Ensure your constructors are well-defined to prevent ambiguity.

Best Practices for Using C++ Brace Initialization
When to Use Braced Initialization
Braced initialization is particularly beneficial when dealing with aggregates, containers, or when your code demands clarity. Its ability to simplify initialization and prevent errors makes it a vital tool in a C++ programmer's repertoire.
Leveraging for Modern C++ Features
Braced initialization interacts harmoniously with several modern C++ features, such as `auto`, where it can reduce type ambiguity:
auto myValue{42}; // Automatically deduces type as int
This is an elegant and efficient way to declare and initialize variables.
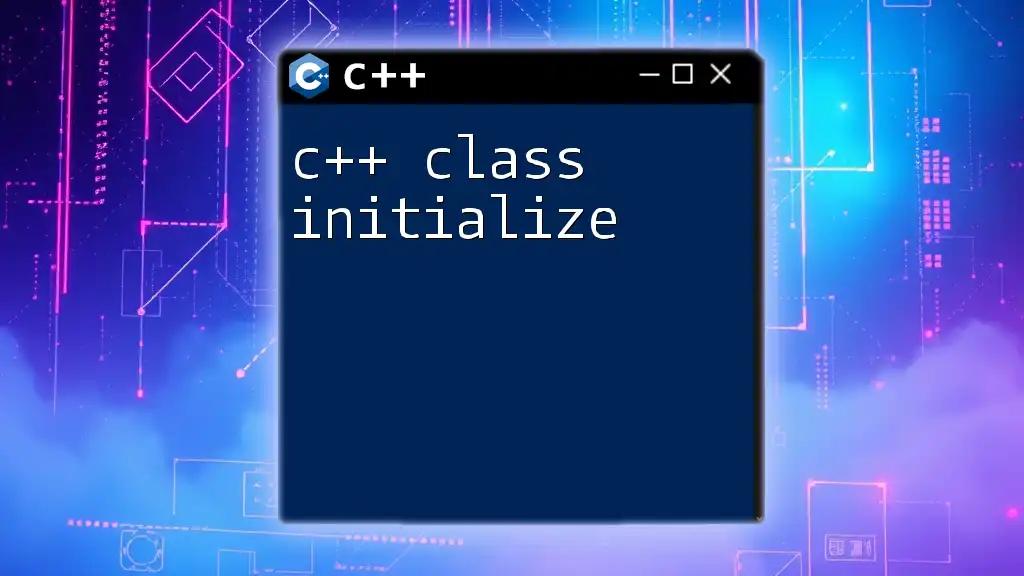
Conclusion
In summary, C++ braced initialization is not just a syntax trick but a significant enhancement to the language that promotes safer and more readable code. By embracing this initialization method, programmers can significantly reduce common pitfalls associated with variable initialization.
Additional Resources
For further exploration of C++ initialization techniques, consider diving into the official C++ standard documentation or reputable C++ programming textbooks. Online coding platforms are also excellent resources to practice and solidify your understanding of braced initialization.
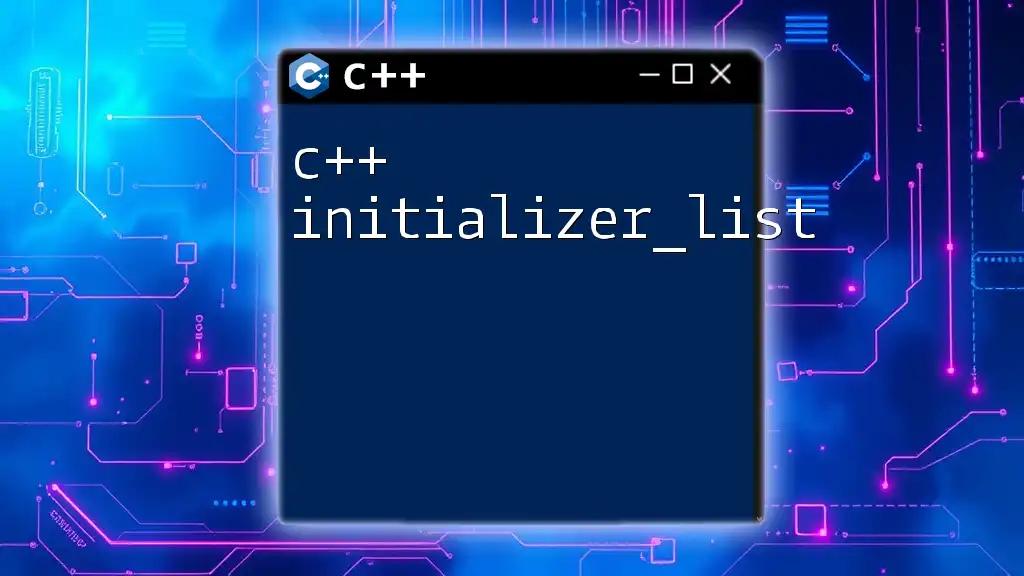
Frequently Asked Questions (FAQ)
What is the difference between braced initialization and traditional initialization?
Braced initialization distinctly prevents narrowing conversions and offers a more uniform syntax compared to traditional methods that don't guarantee type safety.
Can braced initialization be used with user-defined types?
Absolutely! Braced initialization can be applied to user-defined types, making it a versatile feature in C++. For example:
class MyClass {
public:
int a;
MyClass(int value) : a(value) {}
};
MyClass obj{10}; // Braced initialization for user-defined type
By following this guide, you should be well-equipped to make the most of C++ braced initialization in your programming endeavors.