In C++, a class is initialized by defining a constructor method that is called when an object of the class is created.
Here's a code snippet illustrating how to initialize a class in C++:
#include <iostream>
class MyClass {
public:
MyClass() {
std::cout << "Class Initialized!" << std::endl;
}
};
int main() {
MyClass obj; // This will call the constructor
return 0;
}
Understanding Classes in C++
What is a Class in C++?
A class in C++ serves as a blueprint for creating objects, encapsulating data for the object and methods to manipulate that data. At its core, a class allows programmers to implement object-oriented programming (OOP) practices, focusing on how real-world entities can be modeled.
Components of a Class
A class usually consists of two main components:
- Attributes (data members): These represent the properties or characteristics of an object. For example, a class `Dog` may have attributes like `breed`, `age`, and `weight`.
- Methods (member functions): These are functions declared within a class that define the behavior of the class. Using the previous example, a `Dog` class could have methods like `bark()` or `fetch()`.

Methods of Class Initialization in C++
Default Constructor
What is a Default Constructor?
A default constructor is a special type of constructor that does not take any parameters. Its primary role is to initialize objects with default values, setting the stage for object usage even before explicit values are provided.
Example of Default Constructor
class MyClass {
public:
MyClass() {
// Initialization code
}
};
In this example, when an object of `MyClass` is instantiated, the default constructor is called. The code within the constructor can be used to initialize member variables to default values, thus ensuring the object is in a valid state.
Parameterized Constructor
Overview of Parameterized Constructors
A parameterized constructor, on the other hand, allows constructors to accept values when creating an object, providing flexibility to initialize attributes based on specific user input.
Example of Parameterized Constructor
class MyClass {
public:
MyClass(int x) {
// Use 'x' to initialize data members
}
};
Here, the parameterized constructor accepts an integer `x`, which can be used to set a member variable. This form of initialization is particularly useful for classes where values vary widely between instances.
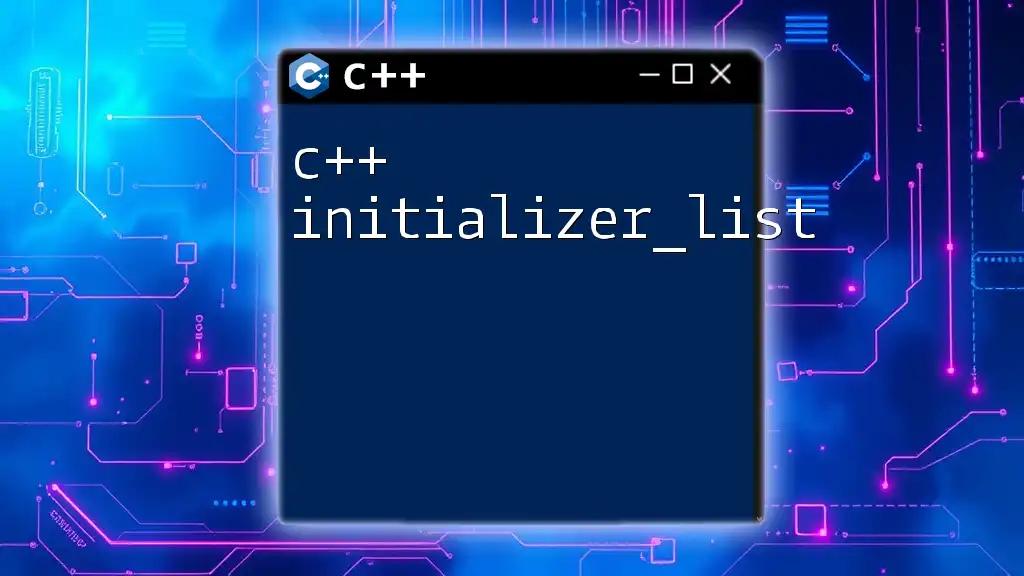
Initializing Class Members Directly
In-Class Member Initializer
What is an In-Class Member Initializer?
An in-class member initializer allows you to initialize members at the point of declaration inside a class. This leads to clearer code by keeping the initialization logic close to the member definitions.
Example of In-Class Member Initializer
class MyClass {
private:
int x = 0; // In-class initialization
};
In this example, `x` is initialized right when it's declared. This technique ensures that `x` always starts with a value, eliminating possible uninitialized usage later on.
Constructor Member Initializer List
Understanding Constructor Member Initializer Lists
An initializer list allows initialization of member variables directly and is a preferred method when dealing with const or reference types, or when better performance is required.
Example of Using Initializer Lists
class MyClass {
private:
int x;
public:
MyClass(int val) : x(val) {} // Initializer list usage
};
In this example, `x` is initialized using the value `val`. This technique is beneficial as it avoids potential extra default construction before the actual construction with value assignment.
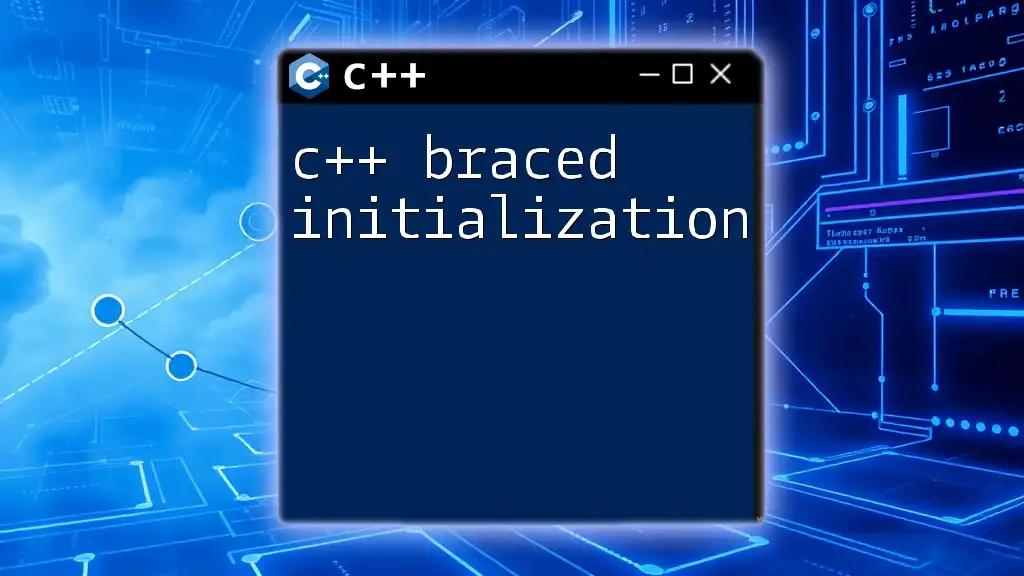
Using `new` and Dynamic Memory Allocation
What is Dynamic Memory Allocation in C++?
Dynamic memory allocation is a powerful feature that allows developers to allocate and deallocate memory at runtime. This is particularly useful for managing resources effectively, especially in programs that require flexibility in memory management.
Example using `new` Operator
MyClass* obj = new MyClass(); // Dynamically initializing an object
With the `new` operator, an object of `MyClass` is created on the heap. This method enables the creation of objects whose lifetime extends beyond the function stack, but it also requires careful management to avoid memory leaks.

Tips and Best Practices for Class Initialization
Optimal Use of Constructors
When deciding between default and parameterized constructors, consider the needs of your class and how you expect it to be used. Choose default constructors for general-purpose classes and parameterized constructors for those that require specific initialization.
Leveraging Member Initializers
In-class initializers are a great shortcut for clarity, while constructor initializer lists offer the most control and efficiency. Understanding when to leverage each will enhance code safety and maintainability.
Avoiding Common Pitfalls
One common mistake during class initialization is forgetting the order of member initialization in constructors, especially in base class scenarios. It's crucial to remember that in derived classes, base class members are initialized before derived class members, which can have implications for resource management.
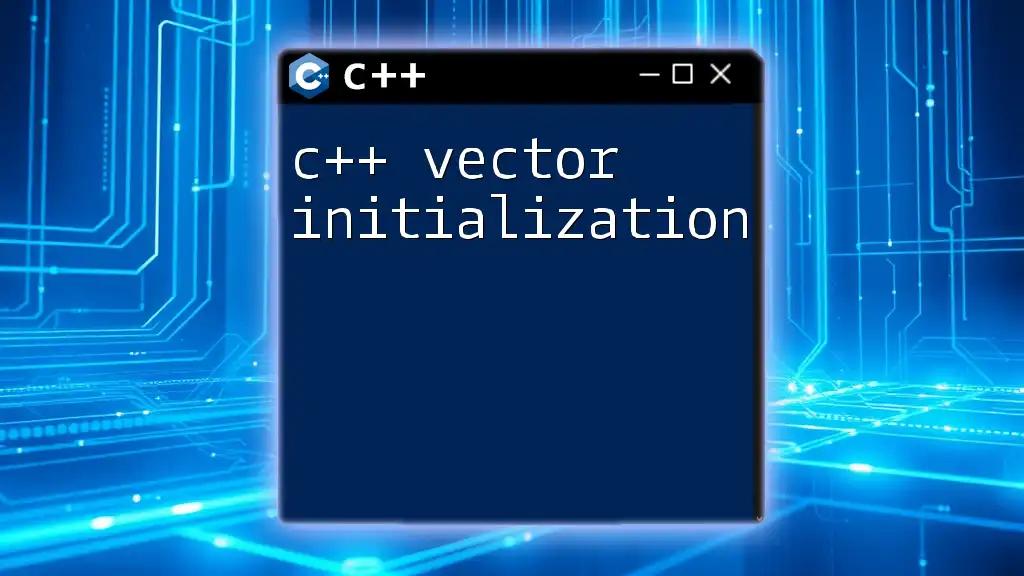
Advanced Topics in Class Initialization
Move Constructors and Move Semantics
Understanding Move Constructors
Move constructors enable the transfer of resources from one object to another, enhancing performance by eliminating unnecessary deep copies.
Example of Move Constructor
MyClass(MyClass&& other) noexcept {
// Transfer resources
}
In this example, resources from `other` are "moved" to the new instance, which can significantly improve program efficiency, especially with large objects or expensive resource handling.
Using `std::optional` for Class Initialization
What is `std::optional`?
`std::optional` is a utility in C++ that represents an object that may or may not hold a value. It simplifies class designs that deal with uncertain values, providing safer access patterns.
Example of `std::optional` in Class Initialization
class MyClass {
private:
std::optional<int> value;
public:
MyClass(std::optional<int> val) : value(val) {}
};
This example shows how `std::optional` can take an optional integer during initialization. This conveys the possibility of absence in a clean and expressive way, enhancing code clarity and robustness.

Conclusion
Understanding how to c++ class initialize is essential for creating robust, efficient, and maintainable C++ applications. By using constructors effectively, leveraging modern features like `std::optional`, and mastering dynamic memory management, programmers can ensure their classes are initialized correctly and intuitively. I encourage you to experiment with these initialization techniques and integrate them into your coding practices for better object-oriented design.

Additional Resources
For those wishing to dive deeper into the intricacies of C++ class design, consider exploring recommended texts on C++ programming or engaging with online communities. Practicing different initialization techniques will solidify your understanding and proficiency in C++.