In C++, a list initializer allows you to initialize containers and variables using a braced syntax, making code clearer and more concise.
Here’s a simple example demonstrating the use of a list initializer for a vector:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Understanding List Initialization
What is List Initialization?
C++ list initialization, also known as list initialization, is a syntactical approach that allows the initializing of variables using curly braces `{}`. This method stands out by providing a clear way to assign values to variables while simultaneously ensuring type safety. Unlike traditional initialization techniques that may allow for unintended conversions, list initialization ensures that the types are respected.
How List Initialization Works
In C++, the syntax for list initialization is simple yet powerful. The format utilizes curly braces to encapsulate the values:
Type variableName{value1, value2, value3};
The curly braces not only signify the start and end of the initialization values but also play a crucial role in preventing narrowing conversions—a concept where a value is converted to a type that cannot represent it.
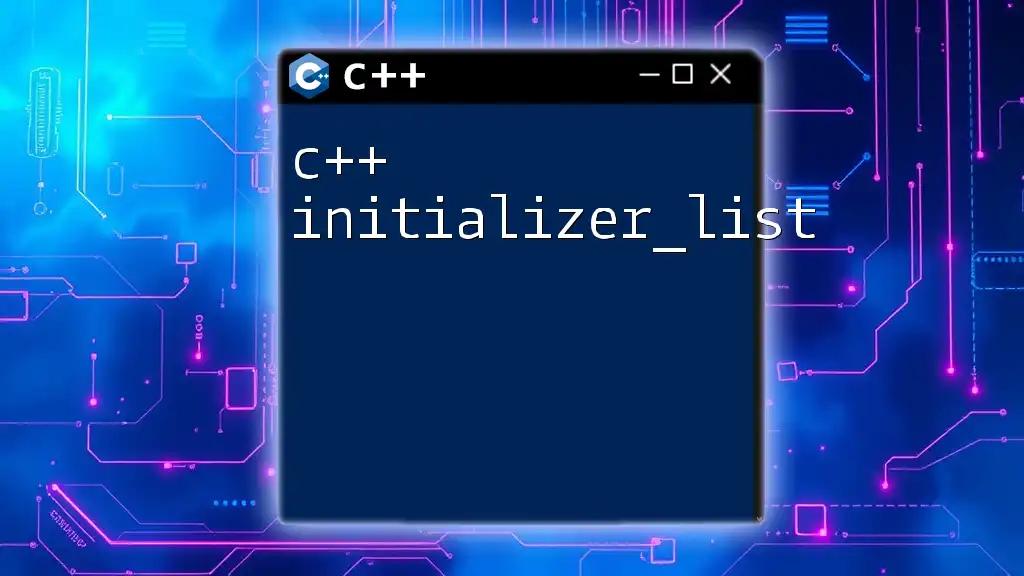
Why Use List Initialization?
Benefits of List Initialization
The advantages of using C++ list initializer are numerous and profound.
-
Clear and Concise Syntax: List initialization helps keep your code neat. It is direct and easy to read, which enhances maintainability.
-
Type Safety: Assigning values using a list initializer ensures that types are checked at compile time, preventing type mismatches that could lead to runtime errors.
-
Narrowing Conversion Prevention: A critical feature of list initialization is its protection against narrowing conversions, which occur when a value of a larger type cannot be accurately represented by a smaller type. For instance:
int x{3.5}; // This will result in an error
-
Code Readability: The structure created by using curly braces adds to the clarity of your code, making it intuitively clear what kind of initialization is taking place.
Common Use Cases
List initialization is versatile and applicable in a variety of scenarios:
-
Initializing Arrays and Containers: You can use list initializers to efficiently initialize collections, such as arrays and STL containers.
-
Zero-Initializing Objects: C++ allows zero-initialization using empty curly braces. For instance, if you want to zero-initialize an array:
int arr[5]{}; // This initializes all elements to 0
-
Use in Constructor Initializations: When defining classes, you may want to ensure that member variables are initialized through class constructors.
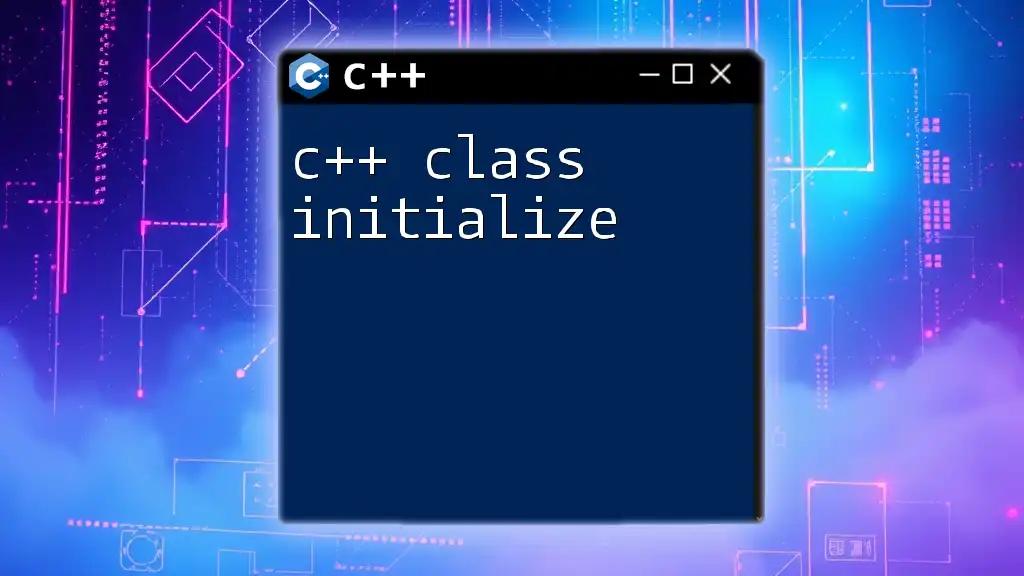
Syntax and Examples
Basic Syntax
To showcase the basic syntax of list initialization:
Type variableName{value1, value2, value3};
List Initialization of Built-in Types
Using list initialization for built-in types is straightforward:
int x{5}; // List initialization for integer
double y{3.14}; // List initialization for double
The compiler checks types closely, which leads to safer code. For instance, attempting to initialize an integer with a floating-point value like so:
int z{3.5}; // Error: narrowing conversion
List Initialization of User-Defined Types
Structs
List initialization can also work seamlessly with user-defined types like structs, making your data structures clearer:
struct Point {
int x;
int y;
};
Point p{1, 2}; // List initializing a struct
By employing this method, we enhance both clarity and the likelihood of correct initialization of the struct.
Classes
Classes handle list initialization gracefully too. Here’s an example of using list initialization in conjunction with class constructors:
class Rectangle {
public:
int width;
int height;
Rectangle(int w, int h) : width{w}, height{h} {}
};
Rectangle rect{10, 20}; // List initialization for class
This showcases how one can easily create objects with pre-defined dimensions, maintaining both clarity and compliance with type checking.
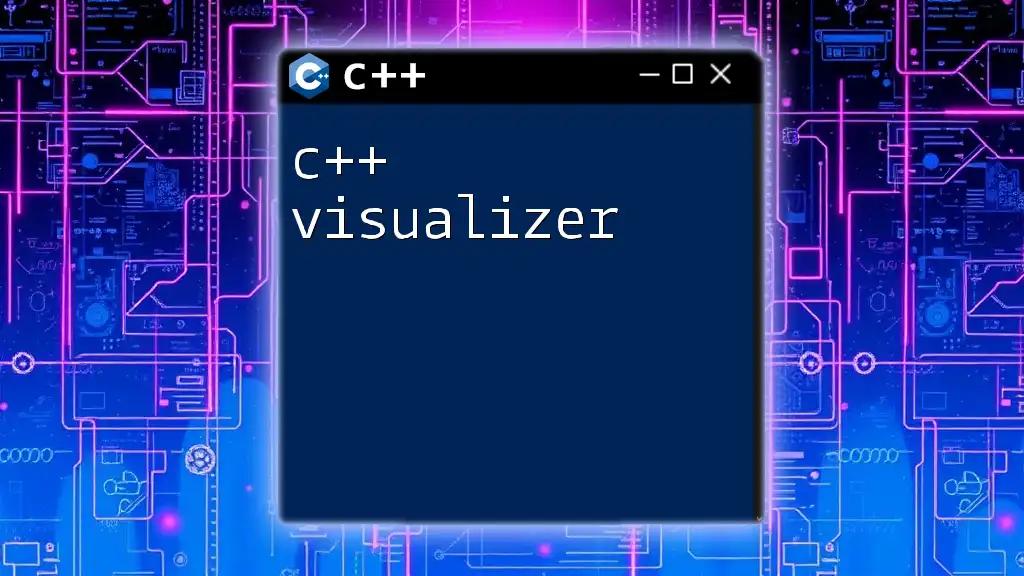
Special Cases in List Initialization
Aggregate Initialization
Aggregate types in C++—such as arrays and structs—can benefit from list initialization. The specifics include:
struct Color {
int r, g, b;
};
Color color{255, 0, 0}; // Using list initialization
This highlights how straightforward it is to initialize compound data types.
Nested Initialization
C++ list initialization allows for powerful nested initializations. For example, you can easily initialize an array of structs with clarity and precision:
Point points[2]{{0, 0}, {1, 1}};
(Optional) Variants of Initialization
It's noteworthy that besides list initialization, C++ allows alternative initialization forms using parentheses `()` or the assignment operator `=`. However, for the purposes of consistency, list initialization is often preferred due to its protective features.
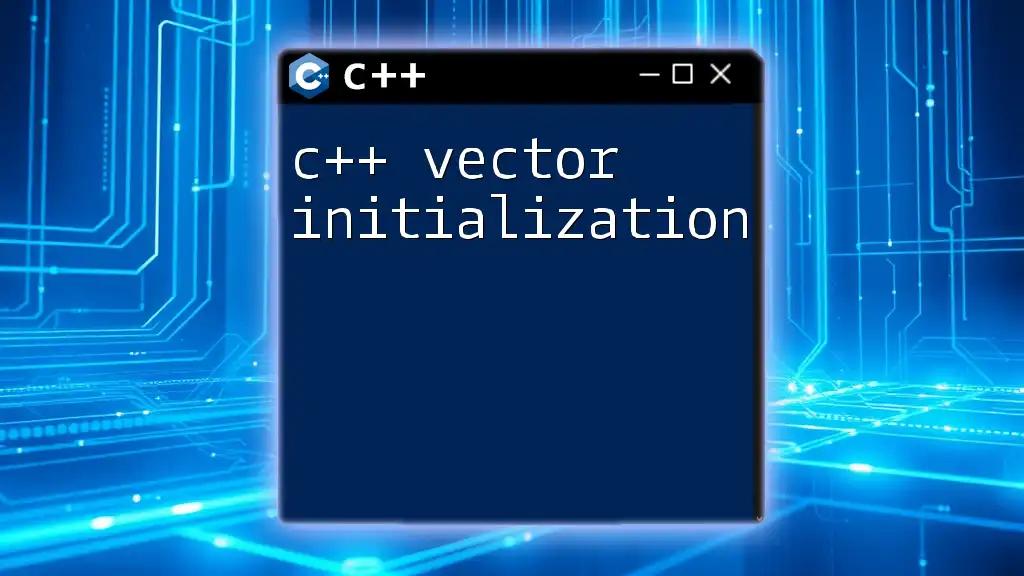
Potential Pitfalls
Common Errors with List Initialization
Despite the benefits, developers can encounter pitfalls. One common issue is narrowing conversions, where values like floats are assigned to integers, resulting in potential data loss and compile-time errors.
Best Practices
To maximize the efficiency of list initialization:
- Prefer List Initialization: Utilize list initialization whenever possible for local variables and member initialization.
- Maintain Type Integrity: Be cautious of the types you are initializing to avoid narrowing conversions.
By adhering to these best practices, programmers can enhance the quality and safety of their C++ code.
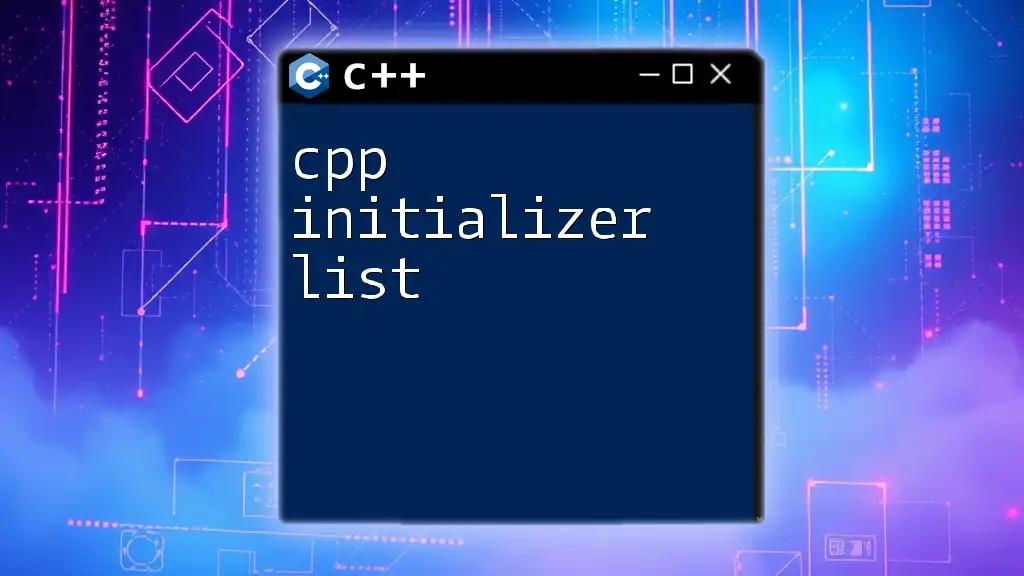
Conclusion
C++ list initializer serves as a powerful tool for initializing variables with clarity and safety. Its emphasis on type integrity and readability makes it a valuable technique in modern C++ programming. By mastering this feature, developers can write cleaner, more reliable code that is easier to maintain.

Additional Resources
For further exploration into C++ list initialization, consider reviewing available online tutorials, thorough documentation, and reputable programming books. Delving deeper into this subject will enhance your proficiency and understanding.
Frequently Asked Questions (FAQs)
What is the difference between list initialization and traditional initialization?
List initialization provides stricter type checking and avoids narrowing conversions, while traditional initialization might allow implicit type conversions with less safety.
Can list initialization be used for all data types?
Most built-in and user-defined types support list initialization, but always check for specific cases in your code.
How does list initialization affect performance?
In terms of performance, the differences are negligible; the primary advantage is in code cleanliness and safety rather than execution speed.