To initialize an empty vector in C++, you can use the following syntax:
#include <vector>
std::vector<int> myVector; // Initializes an empty vector of integers
What is a Vector in C++?
A vector in C++ is a part of the Standard Template Library (STL) that represents a dynamic array. Unlike regular arrays, vectors can grow and shrink in size dynamically during runtime. This flexibility makes them an essential data structure in modern C++ programming.
Vectors are characterized by:
- Dynamic Sizing: Unlike arrays, vectors allow for dynamic memory allocation. You can add or remove elements without needing to know the total size in advance.
- Contiguous Memory Storage: Elements in a vector are stored in contiguous memory locations, making access to elements efficient.
- Versatile Data Types: Vectors can hold multiple data types, including built-in types or user-defined types.
Understanding vectors is crucial for anyone looking to write advanced C++ code, particularly when managing collections of data.

Why Use an Empty Vector?
Initializing an empty vector is a common practice for developers. The benefits include:
Scalability and Dynamic Sizing: An empty vector provides the foundation for an adaptable data structure. You can start with nothing and add elements based on your application's needs.
Memory Management: Since an empty vector occupies minimal memory, you can optimize your program by only allocating memory when necessary.
Performance Considerations: Empty vectors ensure efficiency. They allow you to avoid unnecessary initializations, keeping your program responsive.

Creating an Empty Vector in C++
How to Create an Empty Vector in C++
The simplest way to c++ initialize an empty vector is by using the default constructor provided by the STL. Here’s the basic syntax:
#include <vector>
int main() {
std::vector<int> emptyVector; // Initializing an empty vector of integers
return 0;
}
In this example, `emptyVector` is created but has no elements.
Alternative Methods to Initialize an Empty Vector
You can also create an empty vector using an initializer list. This method enables you to initialize an empty vector explicitly:
std::vector<int> anotherEmptyVector({});
Using curly braces `{}` signifies that no elements are initially added.
Including Specific Data Types
Vectors are versatile and can hold any data type, including user-defined types. For instance, if you have a struct or a class, you can initialize an empty vector of that type:
struct Person {
std::string name;
int age;
};
std::vector<Person> emptyPersonVector; // Empty vector of Person objects
This allows you to manage collections of complex data structures effortlessly.
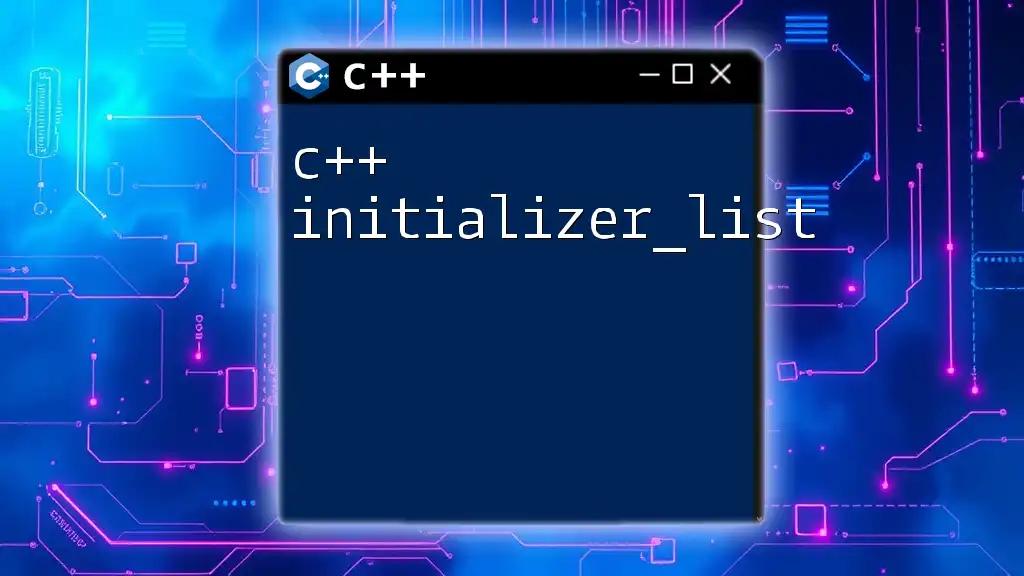
Memory Management of Empty Vectors
Memory Footprint of an Empty Vector
When you c++ initialize an empty vector, it occupies minimal memory—often just enough to store some internal bookkeeping information. In practice, this means that even if you have a large number of vectors created but empty, memory usage remains low.
Growing Vectors Dynamically
One of the key features of vectors is their ability to dynamically allocate memory as you add elements. When an element is pushed into an empty vector, the vector allocates memory automatically:
emptyVector.push_back(10); // Adds 10 to the empty vector
This method handles memory internally, resizing as needed, ensuring efficient memory usage as your data set grows.

Accessing Elements in a Vector
Safety Considerations
When working with vectors, always check if they are empty before accessing elements. Accessing an element in an empty vector can lead to undefined behavior:
if (!emptyVector.empty()) {
std::cout << emptyVector[0]; // Access first element safely
}
This simple check prevents program crashes and logical errors.
Best Practices for Using Empty Vectors
When initializing an empty vector, consider the following:
- Analyze when and why you need to initialize an empty vector. Use it when you anticipate that the data collection will need to grow.
- Reserve space when you know the approximate number of elements needed. This can improve performance by avoiding multiple reallocations. For example:
emptyVector.reserve(100); // Reserve space for 100 elements upfront
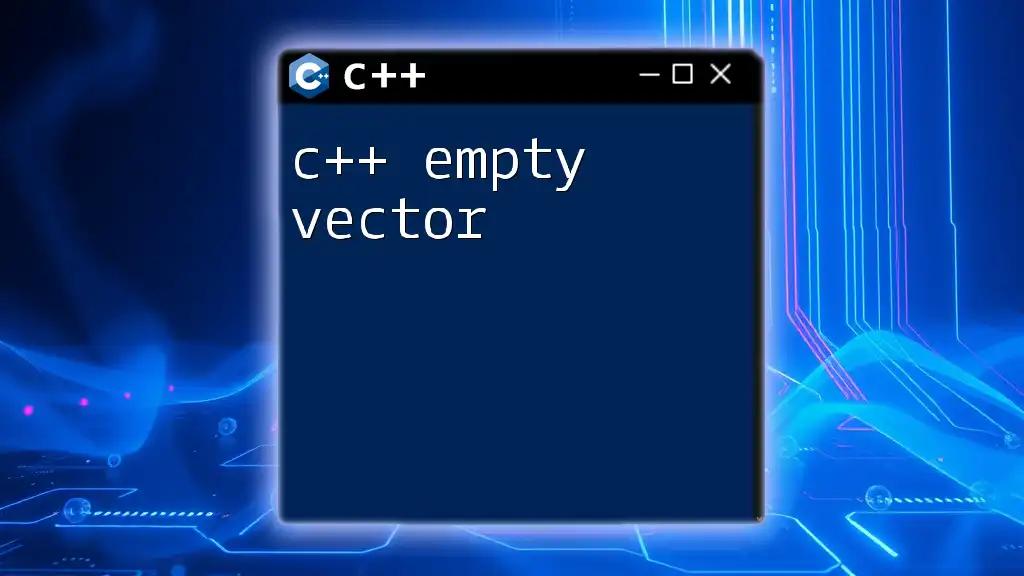
Common Mistakes When Working with Empty Vectors
One common mistake is forgetting to check for emptiness before accessing an element. Always remember to use the `.empty()` method to ensure that you are interacting with valid data.
Another error is misunderstanding the concept of vector capacity vs. size. The size refers to the number of elements currently stored in the vector, while capacity indicates the amount of space that has been allocated for future elements. An empty vector has a size of 0 but might have a positive capacity if memory has been reserved:
std::cout << "Size: " << emptyVector.size() << ", Capacity: " << emptyVector.capacity();
Understanding the difference can significantly impact performance and memory utilization.

Conclusion
Mastering how to c++ initialize an empty vector is a crucial skill for any C++ programmer. Vectors provide flexibility, dynamic sizing, and efficient memory management. By understanding the fundamental operations of establishing and managing empty vectors, you can enhance the performance and reliability of your applications.
Experiment with vectors in your own coding projects to reinforce these concepts, and explore more advanced functionalities that vectors offer in the Standard Template Library (STL).
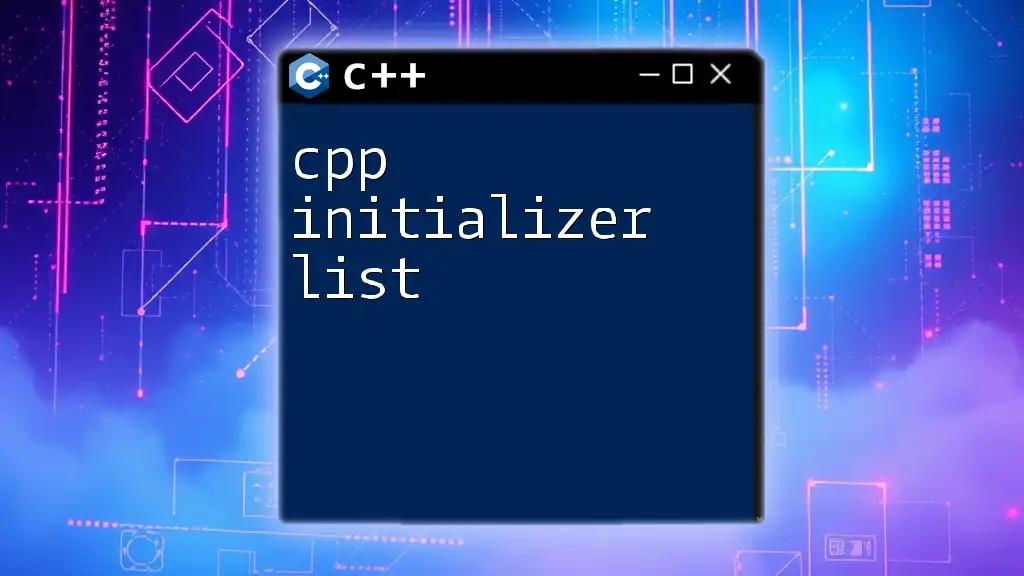
Additional Resources
For further exploration, consult the official C++ documentation and tutorials that cover vectors and the STL comprehensively. There are numerous online resources, books, and courses available to help enhance your understanding of C++ vectors.

FAQs
Is an empty vector really empty? Yes, an empty vector has a size of 0 and contains no elements, but it can still be utilized to store items by adding them dynamically.
How do I know how much space an empty vector has? Use the `.capacity()` method. An empty vector typically has a capacity of 0, but this may change as you add elements.
Can I initialize a vector without specifying its data type? No, C++ requires you to specify the data type for vectors. If you need a generic-like structure, consider using `std::variant` or pointers.
What’s the difference between `std::vector::clear()` and initializing a new empty vector? `std::vector::clear()` removes all elements from the vector while maintaining its allocated capacity. Initializing a new empty vector resets its size and capacity to zero.