The C++ Standard Template Library (STL) vector is a dynamic array that can resize itself automatically when elements are added or removed, making it a versatile container.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
numbers.push_back(6); // Adding an element
for(int num : numbers) {
std::cout << num << " "; // Output: 1 2 3 4 5 6
}
return 0;
}
What is a Vector?
A C++ STL vector is a sequence container that encapsulates dynamic size arrays. Unlike regular arrays that have a fixed size, vectors can resize themselves automatically when elements are added or removed. This flexibility offers significant advantages in memory management and ease of use.
Advantages of Using Vectors
- Dynamic Sizing: Vectors can grow and shrink based on the needs of an application, making it easy to manage collections of data without worrying about size limitations.
- Efficiency: Vectors utilize contiguous memory allocation, which leads to better cache performance and provides the ability to access elements quickly.
- Convenient Functions: C++ STL vectors come with a variety of built-in functions that simplify operations such as adding, removing, and accessing elements.
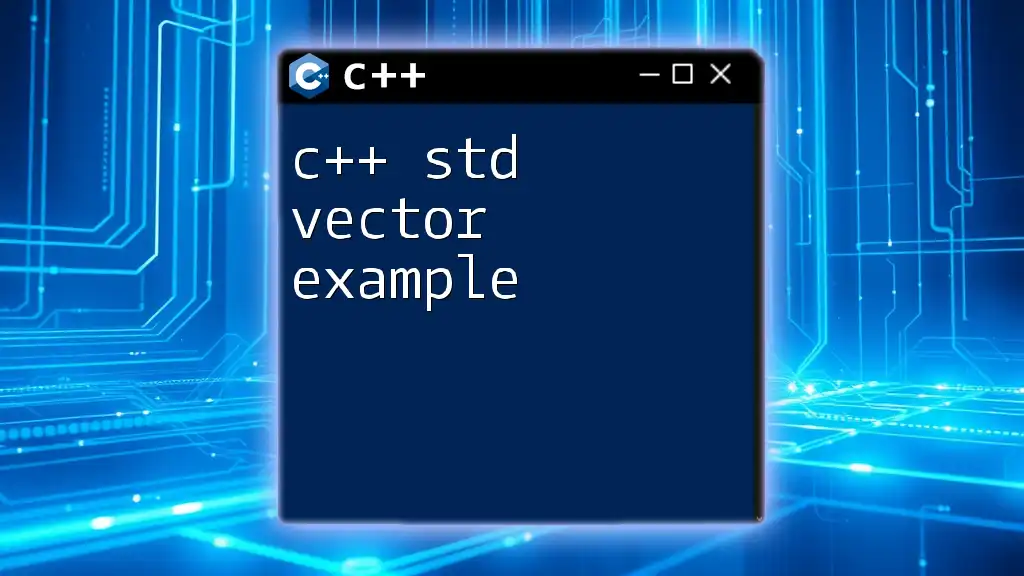
Basic Operations on Vectors
Declaring and Initializing Vectors
To use a vector in C++, you must include the `<vector>` header file. Here are some common ways to declare and initialize vectors:
#include <iostream>
#include <vector>
std::vector<int> vec1; // Empty vector
std::vector<int> vec2(5); // Vector of size 5, initialized with default values (0)
std::vector<int> vec3{1, 2, 3}; // Initializer list with values 1, 2, and 3
Adding Elements
Using `push_back()`
To add elements to the end of a vector, you can use the `push_back()` method. This method allows you to append new elements efficiently.
vec1.push_back(10); // Adds 10 to the end of vec1
Using `insert()`
If you need to insert elements at specific positions, the `insert()` function can be used. It requires an iterator pointing to the position where you want to add the new data.
vec3.insert(vec3.begin() + 1, 5); // Inserts 5 at index 1, shifting subsequent elements
Accessing Elements
Using `[]` Operator
You can access elements using the index operator `[]`. This provides direct access, but it does not perform bounds-checking, so ensure that the index is valid.
std::cout << vec3[0]; // Outputs the first element: 1
Using `at()`
For safer access, the `at()` function can be used. This method includes bounds-checking and throws an exception if the index is out of range.
std::cout << vec3.at(1); // Outputs the second element: 5
Removing Elements
Using `pop_back()`
To remove the last element from a vector, you can use the `pop_back()` method. This operation does not return the removed element.
vec1.pop_back(); // Removes the last element from vec1
Using `erase()`
To remove specific elements from a vector, `erase()` is the appropriate method. You must specify the iterator pointing to the element to be removed.
vec3.erase(vec3.begin() + 1); // Removes the element at index 1 (which is 5)
Other Important Vector Functions
`size()`
To find out how many elements are in a vector, you can call the `size()` function.
std::cout << "Size: " << vec1.size(); // Displays the current size of vec1
`clear()`
If you want to remove all elements from a vector, you can use the `clear()` function, which effectively resets the vector.
vec1.clear(); // Clears all elements from vec1
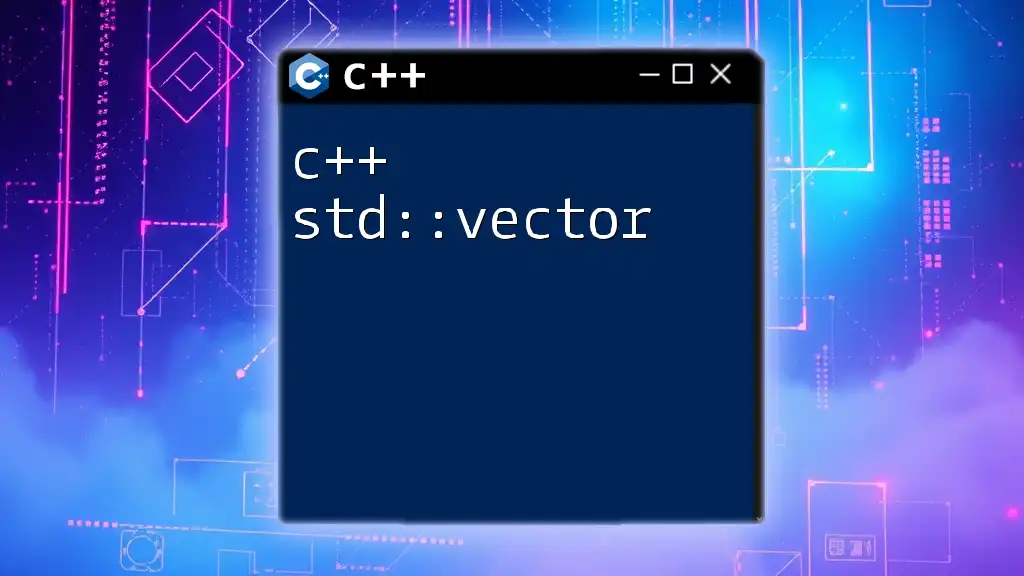
Iterating Over Vectors
Range-based For Loop
One of the simplest ways to iterate over a vector is by using a range-based for loop. This approach is clean and concise.
for (auto& element : vec3) {
std::cout << element; // Prints each element in vec3
}
Traditional For Loop
Although range-based for loops are convenient, you can also use a traditional for loop if you need the index for other operations.
for (int i = 0; i < vec3.size(); ++i) {
std::cout << vec3[i]; // Accessing each element by index
}
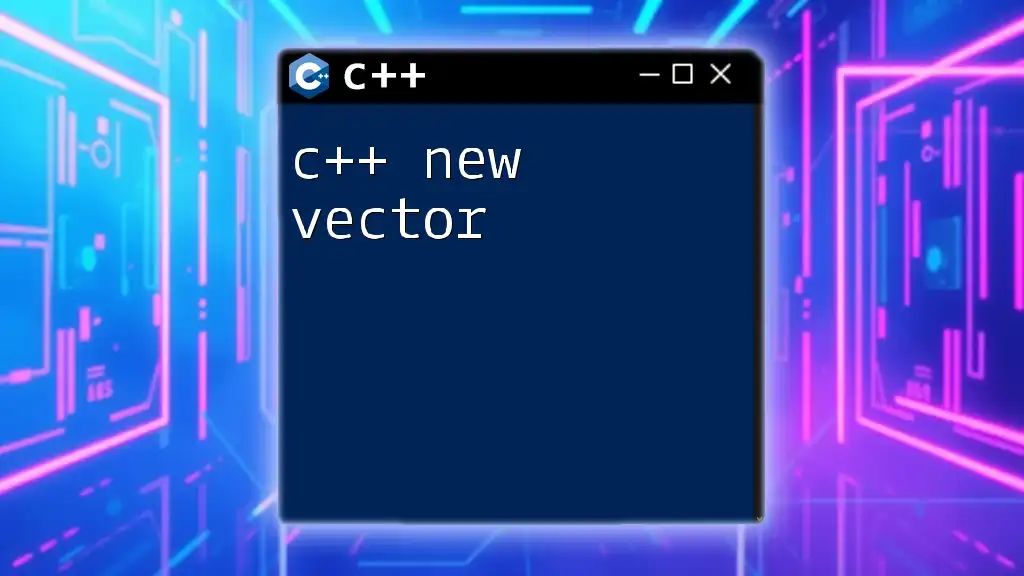
Memory Management and Performance
Internal Representation
Vectors in C++ manage memory through contiguous allocation, which means all elements are stored in a single block of memory. This can lead to better cache performance and speed, especially when accessing elements sequentially.
Capacity Management
Understanding the difference between the capacity and size of a vector is crucial for memory management. The `capacity()` function gives you the amount of allocated memory, while `size()` returns the number of actual elements:
- Capacity: The total number of elements that can be stored without reallocating.
- Size: The current number of elements in the vector.
You can also control the growth of your vectors by using `reserve()` to pre-allocate memory. This can improve performance by reducing the number of reallocations.
vec1.reserve(100); // Reserves space for 100 elements without changing the size
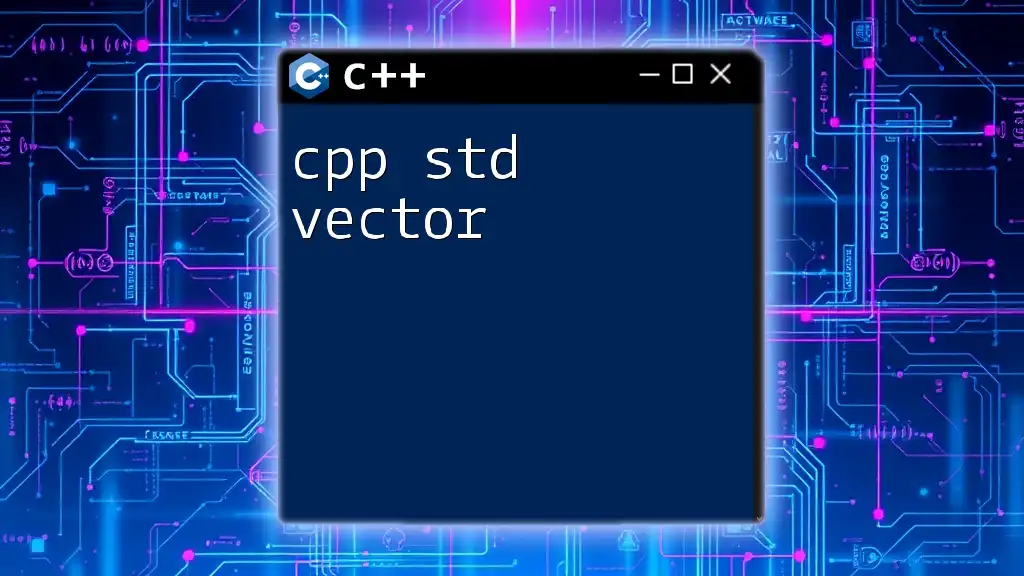
Common Use Cases for Vectors
Storing Collections of Data
Vectors are ideal for storing dynamic collections where the size can change frequently, such as lists of user inputs or items in a shopping cart.
Implementing Algorithms
Vectors are particularly advantageous when implementing algorithms, such as sorting and searching, due to their flexibility and ease of element access.
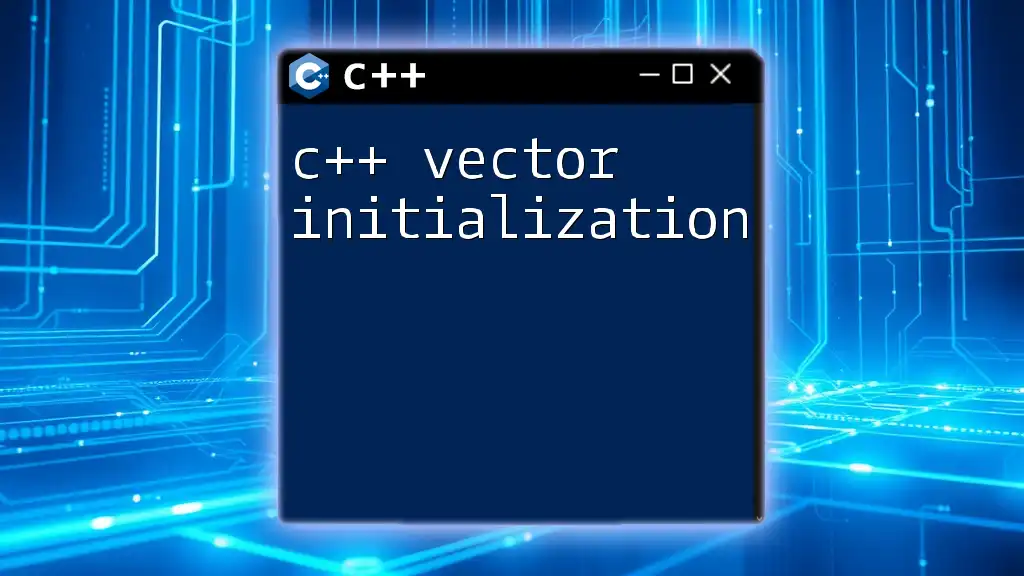
Best Practices for Using Vectors
Performance Considerations
When to choose vectors over other STL containers depends on the situation. For frequently changing sizes or when the benefit of cache performance is critical, vectors are highly recommended.
Avoiding Pitfalls
Common mistakes with vectors include accessing elements out-of-bounds. Always use `.at()` for safer access, or ensure your indices are within valid ranges.
// Avoid this!
vec3.at(100); // This throws an out_of_range exception
Using these guidelines, you can leverage the C++ STL vector effectively in your applications, enhancing both functionality and performance. With a wealth of dynamic capabilities and built-in support from the Standard Template Library, vectors remain a fundamental tool for C++ programmers.