In C++, the `sizeof` operator can be used to determine the size in bytes of a vector, which returns the size of the vector's internal storage rather than the number of elements it contains.
Here's a code snippet demonstrating this:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::cout << "Size of vector in bytes: " << sizeof(vec) << std::endl;
return 0;
}
Understanding C++ Vectors
What is a Vector?
A vector in C++ is a dynamic array that can change in size during program execution. Unlike traditional arrays, which have a fixed size, vectors can grow or shrink as needed. This flexibility makes them an essential part of the C++ Standard Library, especially when the required size of a dataset is unknown at compile time.
Vectors provide several benefits over arrays:
- Dynamic sizing: You do not need to specify the size upfront.
- Convenient methods: Vectors come with built-in functions for insertion, deletion, and traversal.
- Memory management: C++ handles memory allocation and deallocation for vectors, which reduces the risk of memory leaks.
Basic Operations on Vectors
Performing basic operations with vectors is intuitive. You can easily add, remove, and access elements. For example, consider the following code snippet:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec; // Declare a vector of integers
vec.push_back(10); // Add an element
vec.push_back(20);
vec.push_back(30);
for (int i : vec) {
std::cout << i << " "; // Accessing elements
}
return 0;
}
In this code, `vec` starts empty, and elements are added using `push_back()`. The loop prints the contents of the vector.
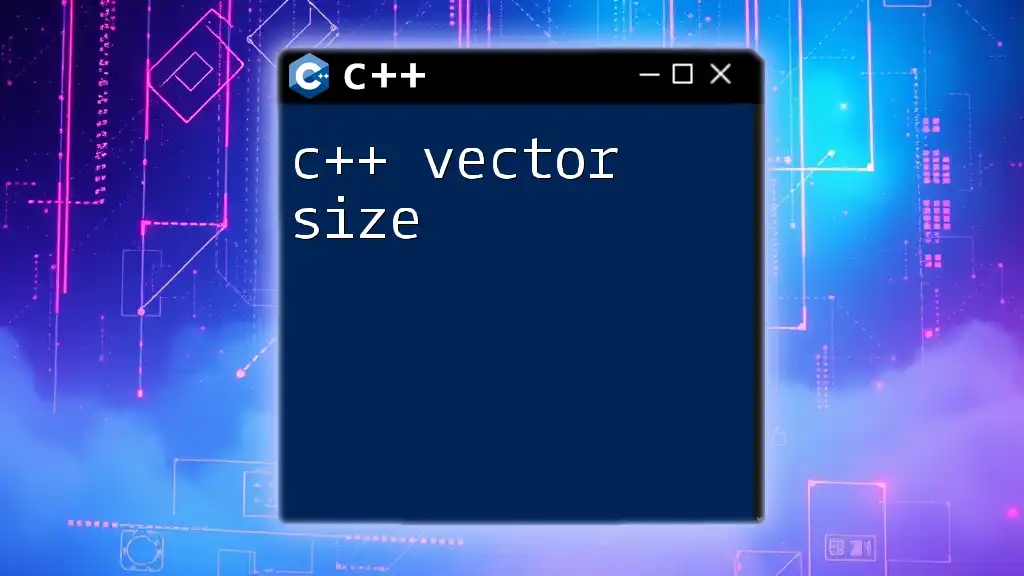
The `sizeof` Operator in C++
What Does `sizeof` Do?
The `sizeof` operator is a compile-time operator in C++ that returns the size, in bytes, of a type or an object. It is crucial for understanding memory requirements in your application.
Using `sizeof`, you can determine how much memory an object will occupy, which is particularly important in performance-sensitive applications where memory usage can impact efficiency.
Using `sizeof` with Basic Data Types
Let's examine how `sizeof` works with standard data types:
#include <iostream>
int main() {
std::cout << "Size of int: " << sizeof(int) << " bytes." << std::endl;
std::cout << "Size of char: " << sizeof(char) << " bytes." << std::endl;
std::cout << "Size of float: " << sizeof(float) << " bytes." << std::endl;
return 0;
}
The output will vary based on the compiler and system architecture, but typically:
- `sizeof(int)` may return 4 bytes
- `sizeof(char)` will return 1 byte
- `sizeof(float)` may return 4 bytes
These results show how `sizeof` helps determine memory usage for basic types.
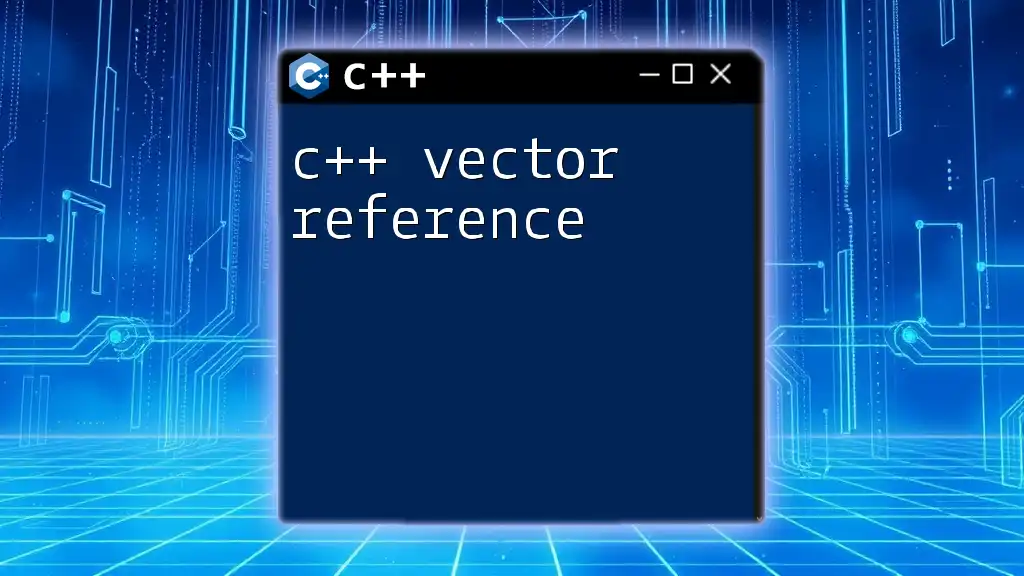
Using `sizeof` with Vectors
Getting the Size of a Vector
When you use `sizeof` on a vector, it returns the size of the vector object itself, not the size of the elements. For example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4};
std::cout << "Size of vec: " << sizeof(vec) << " bytes." << std::endl;
return 0;
}
Here, `sizeof(vec)` gives you the size of the vector's metadata (like pointers to the data, size, and capacity), not a count of the individual elements. This is typically much less than you might expect.
Memory Size of Vector Elements
To calculate the total memory size of all elements in a vector, you should multiply the number of elements by the size of each element type. For an integer vector:
std::cout << "Total size of elements: " << vec.size() * sizeof(int) << " bytes." << std::endl;
This code snippet correctly computes the total memory used by all integer elements, emphasizing that `sizeof` on the vector does not account for the dynamically allocated array behind it.
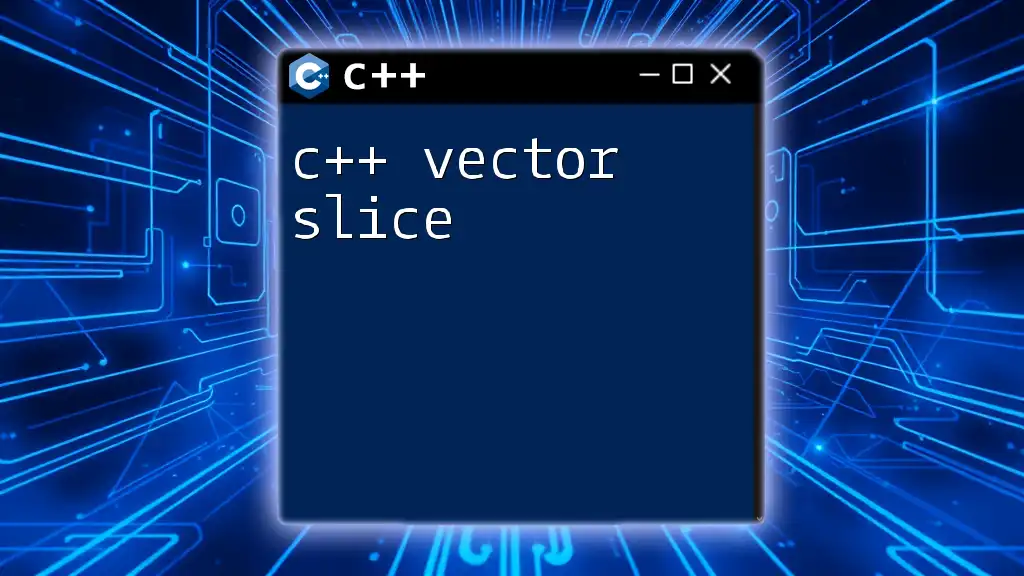
Considerations When Using `sizeof`
Important Details to Note
It is critical to note that the size obtained with `sizeof` on vector instances does not include the size of the actual data they may point to. Given that a vector allocates memory dynamically, its overhead (size and capacity management) is separate from the size of the elements.
When to Use `sizeof` with Vectors
`sizeof` can inform decisions in performance-sensitive applications, such as:
- Memory allocation: Understanding how much memory is used by the vector metadata.
- Benchmarking: Measuring overhead when performing operations on large datasets.
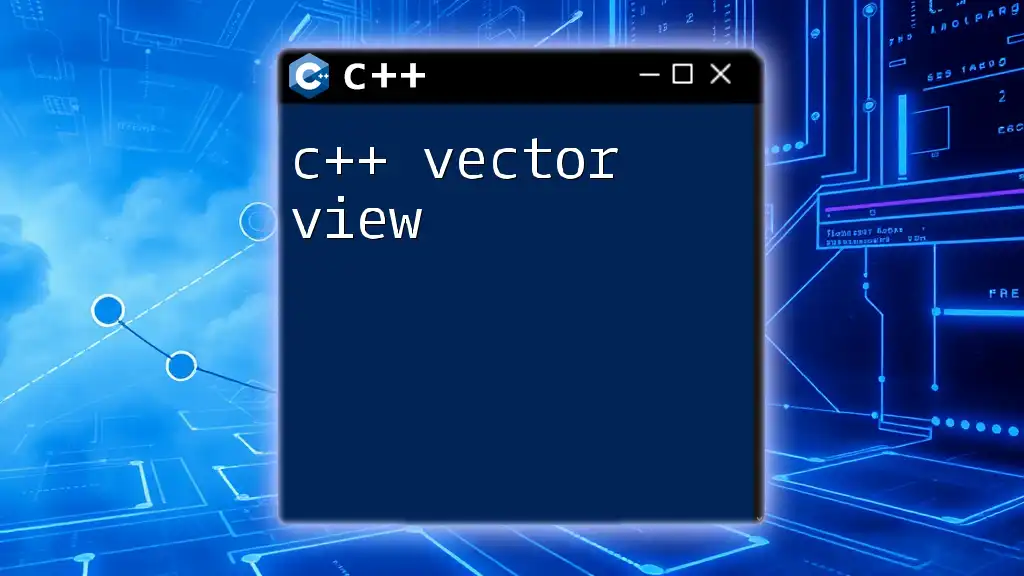
Comparing `sizeof` and `std::vector::size()`
What’s the Difference?
`std::vector::size()` returns the number of elements currently stored in the vector. Contrastingly, `sizeof` tells you the size of the vector object itself. An example comparison is shown below:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4};
std::cout << "Size of vector (element count): " << vec.size() << std::endl;
std::cout << "Size in bytes using sizeof: " << sizeof(vec) << " bytes." << std::endl;
return 0;
}
Here, `vec.size()` reflects how many integers are stored in the vector (4), while `sizeof(vec)` returns the size of the vector's internal structure. It's important to understand this distinction to avoid underestimating memory use.
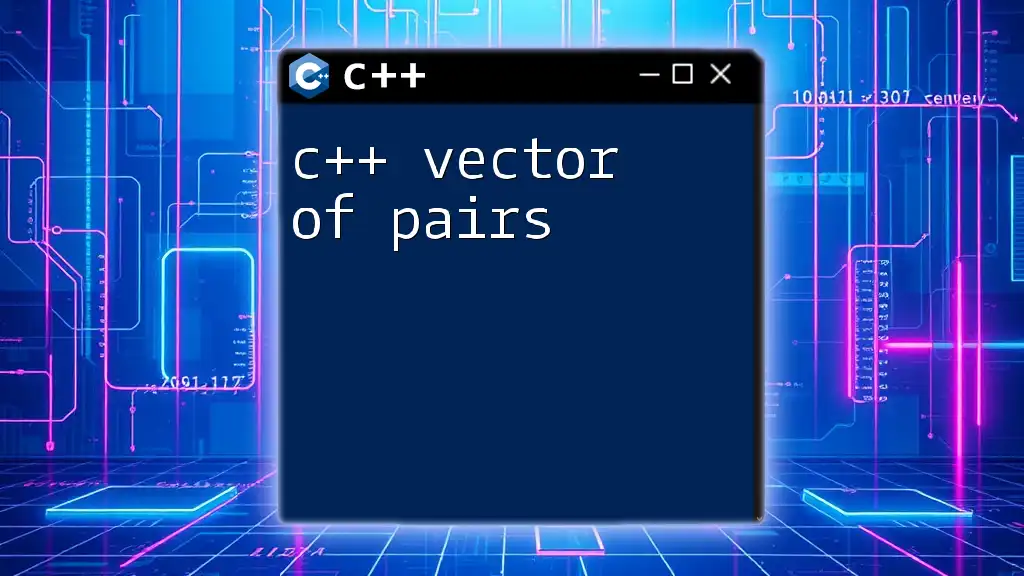
Practical Applications
Case Scenarios
Understanding memory sizes through `sizeof` helps in various scenarios, such as:
- Memory profiling: When working with large datasets, efficiently measuring memory can guide optimizations.
- Data structures: Knowing the vector's size versus capacity can enhance performance in applications that rely heavily on dynamic data.
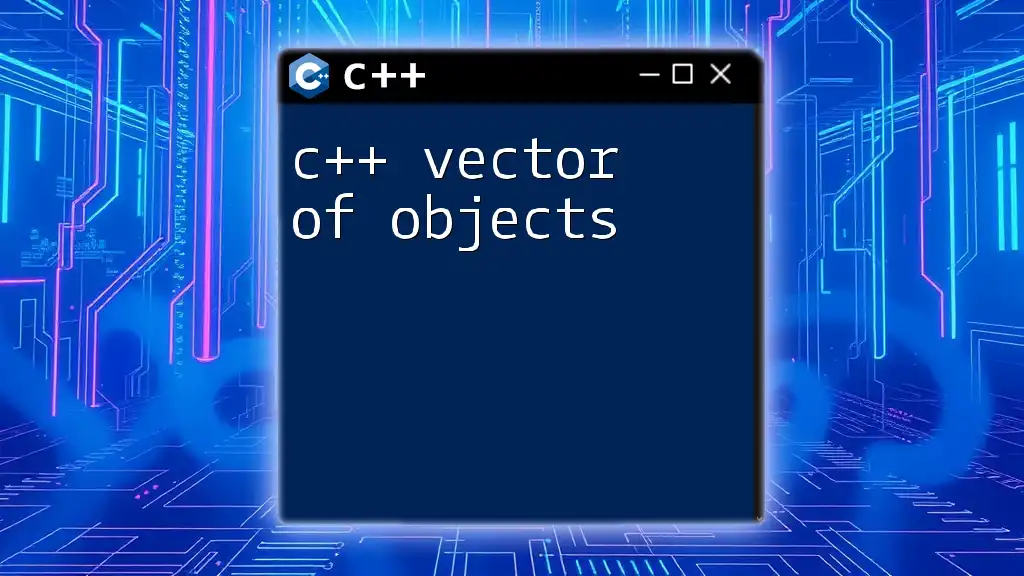
Conclusion
In summary, mastering the concept of C++ vector sizeof is vital for efficient memory management and optimizing performance in C++ applications. The `sizeof` operator provides valuable insights into object sizes and memory layouts but should be used judiciously alongside `std::vector::size()`, which gives the count of elements. Gaining proficiency with these concepts will empower you to write more efficient, robust C++ code.
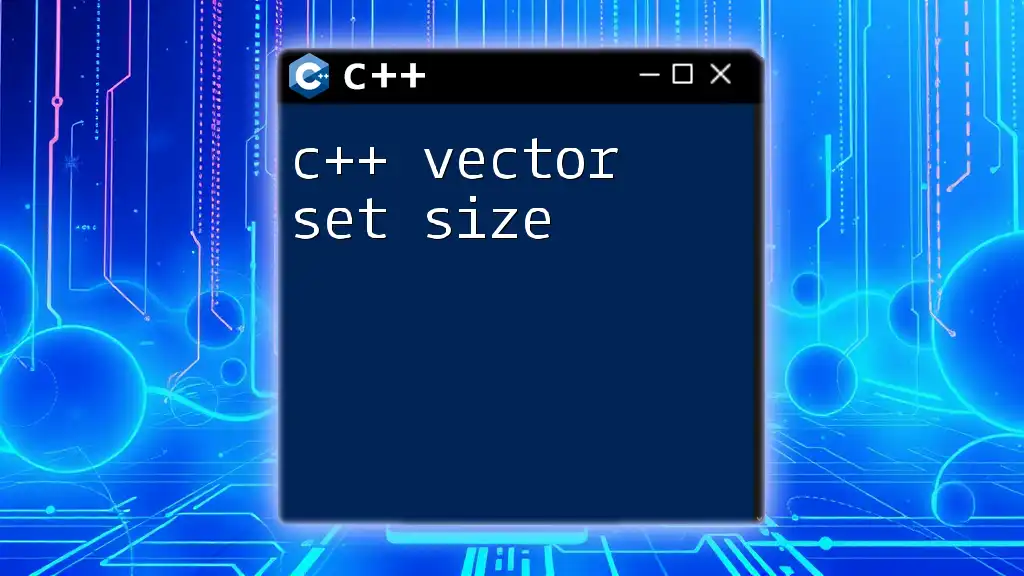
Additional Resources
For continued learning, refer to the official C++ documentation on vectors and the `sizeof` operator to explore further ways to optimize your understanding and application of C++.