A C++ vector of objects allows you to create a dynamic array that can store multiple instances of a user-defined class, enhancing memory management and providing flexibility for handling collections of data.
#include <iostream>
#include <vector>
class MyObject {
public:
MyObject(int value) : value(value) {}
void display() const { std::cout << "Value: " << value << std::endl; }
private:
int value;
};
int main() {
std::vector<MyObject> objects; // Vector of MyObject instances
objects.emplace_back(1); // Adding objects
objects.emplace_back(2);
for (const auto& obj : objects) {
obj.display(); // Display each object's value
}
return 0;
}
Understanding C++ Vectors
What is a Vector?
In C++, a vector is a dynamic array that can grow and shrink in size as needed, providing the flexibility that static arrays lack. Vectors manage their own storage and automatically handle memory allocation and deallocation, allowing for a more efficient and error-free coding experience.
Why Use Vectors?
Vectors offer several advantages over traditional arrays:
- Dynamic Size: Vectors can change size at runtime, adapting to the amount of data they store.
- Ease of Use: Built-in functions like `push_back()`, `pop_back()`, and `insert()` simplify data manipulation.
- Memory Management: Vectors automate memory allocation and deallocation, reducing the risk of memory leaks.
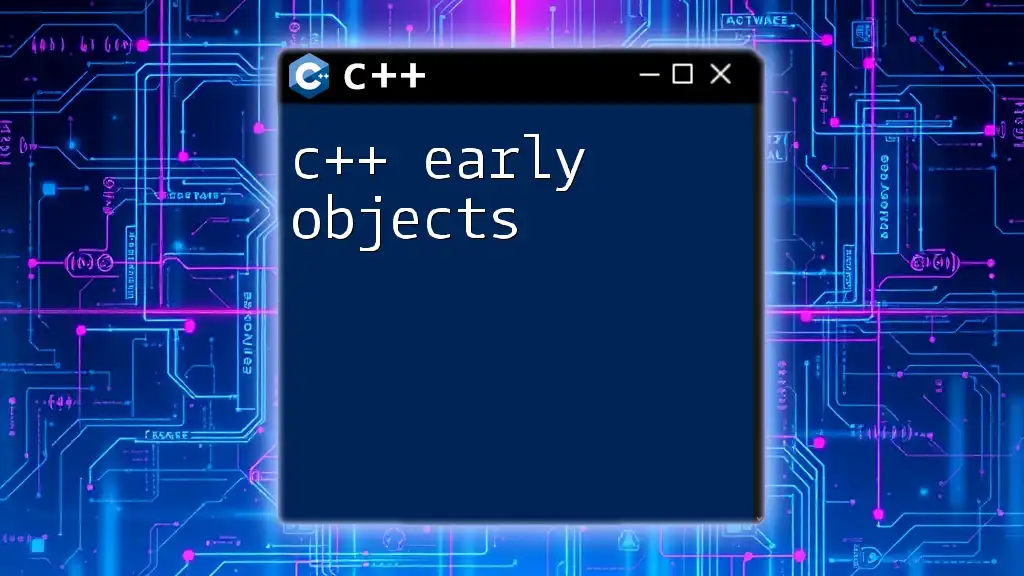
Understanding Objects in C++
What is a Class?
A class in C++ is a user-defined data type that represents real-world entities. It encapsulates data (attributes) and functions (methods) that operate on that data, promoting modular programming and data abstraction.
Creating an Object
To create an object, you first define a class and then instantiate it. Here is how you can define a simple class and create an object:
class Student {
public:
std::string name;
int age;
Student(std::string n, int a) : name(n), age(a) {} // Constructor
};
In this example, we have defined a `Student` class with a constructor that initializes the name and age attributes.
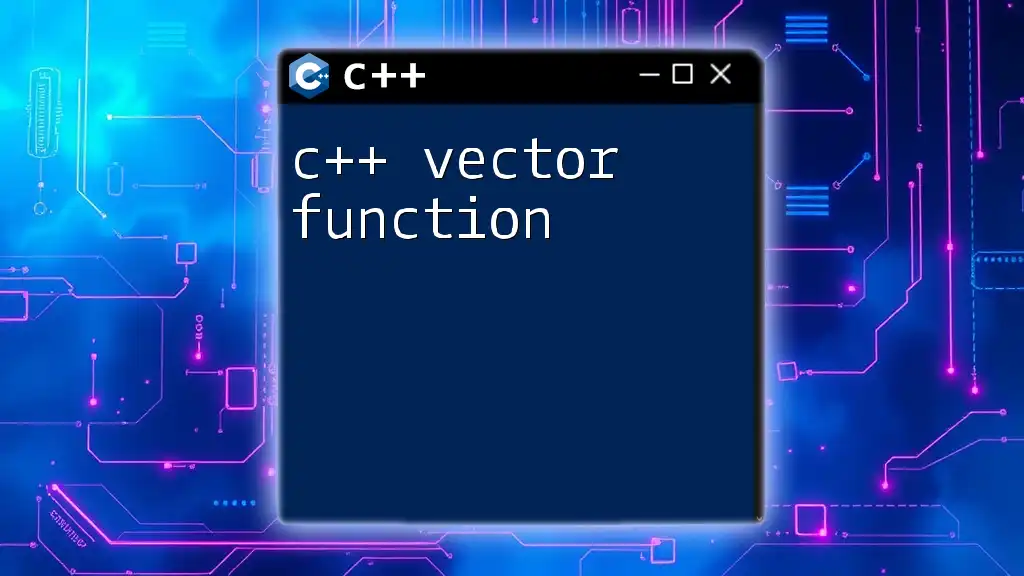
C++ Vector of Objects
What is a Vector of Objects?
A vector of objects is a vector that holds instances of a user-defined class. This concept allows for the storage and manipulation of multiple objects in a single, manageable structure. Unlike vectors of primitive types, vectors of objects involve more complexity due to object construction, copying, and destruction.
Declaring a Vector of Objects
To declare a vector of objects, you need to specify the class type. Here's an example:
#include <iostream>
#include <vector>
class Student {
public:
std::string name;
int age;
Student(std::string n, int a) : name(n), age(a) {}
};
int main() {
std::vector<Student> students; // Declare a vector of Student objects
return 0;
}
Adding Objects to a Vector
You can add objects to a vector using the `push_back()` method, which appends a new item to the end of the vector. Here’s how it’s done:
Student s1("John", 20);
Student s2("Alice", 22);
students.push_back(s1); // Adding John
students.push_back(s2); // Adding Alice
Accessing Objects in a Vector
To access objects in a vector, you can use both indexing and iterators. Here's an example using a range-based for loop to access and print student details:
for (const auto& student : students) {
std::cout << student.name << " is " << student.age << " years old.\n";
}
This method succinctly iterates through each object, allowing you to access their properties with ease.
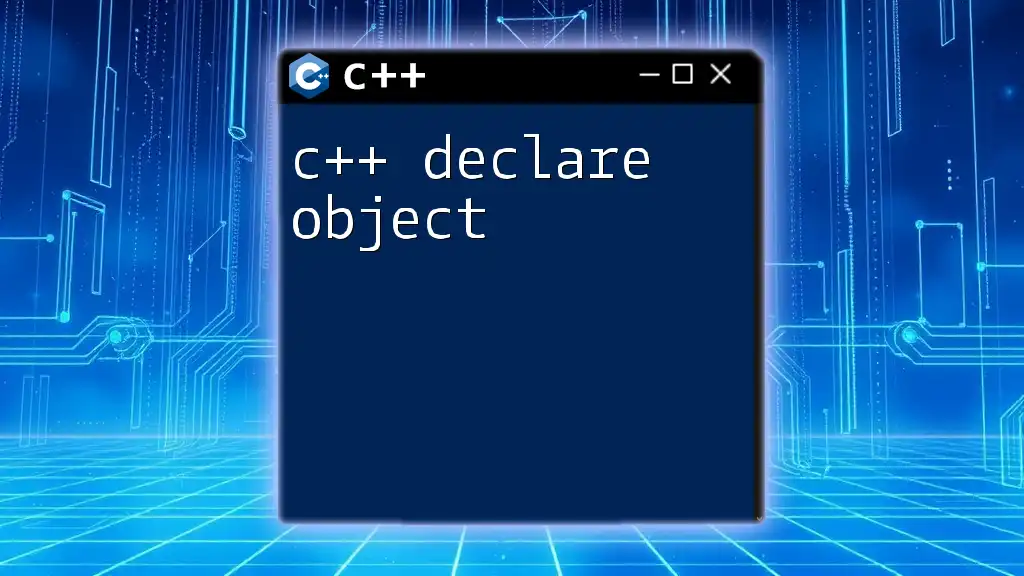
Iterating Over a Vector of Objects
Using Range-Based For Loop
The range-based for loop is convenient for iterating over all elements in a vector. It improves code readability and reduces the possibility of errors during iteration. For instance:
for (const Student& student : students) {
std::cout << student.name << " - " << student.age << std::endl;
}
By using this syntax, you avoid manual index management while iterating.
Using Standard Iterators
Another method to access objects in your vector is through standard iterators. This allows more control over the iteration process. Here’s an example:
for (auto it = students.begin(); it != students.end(); ++it) {
std::cout << it->name << std::endl; // Using arrow operator to access members
}
Using iterators can be particularly useful when you need to manipulate the vector during iteration.
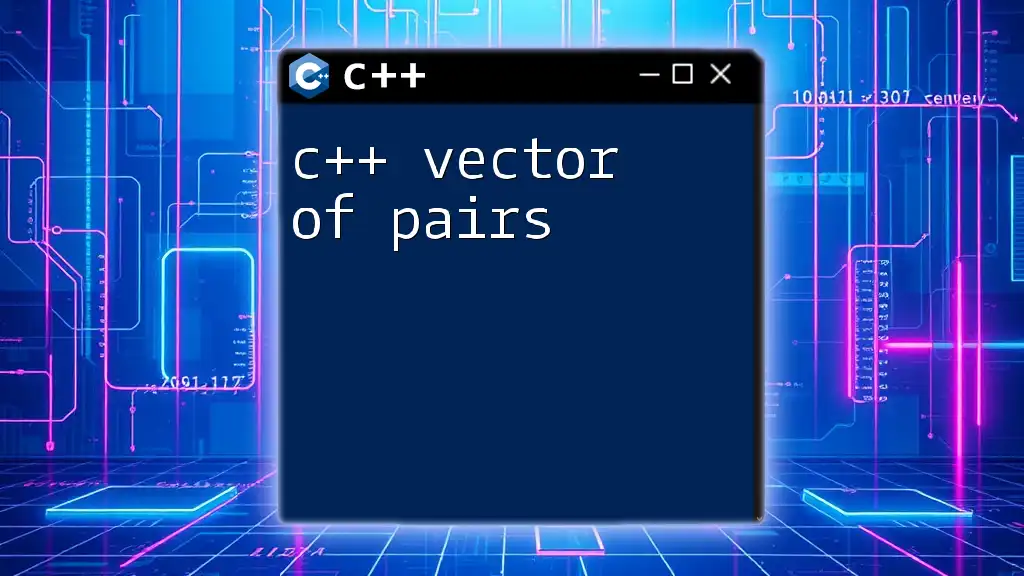
Modifying Objects in a Vector
Updating Objects
You can easily modify the properties of objects stored within a vector. For example, if you want to change the age of the first student, you can do this:
students[0].age = 21; // Update age of the first student
This direct access ensures that any changes are immediately reflected within the vector.
Removing Objects from a Vector
To remove objects from a vector, you can use the `erase()` method along with the element's position or the `remove_if()` function for conditions. For example, to remove the second student:
students.erase(students.begin() + 1); // Removes the second student
Keep in mind that using `erase()` shifts elements, which can impact performance if done repeatedly inside a loop.
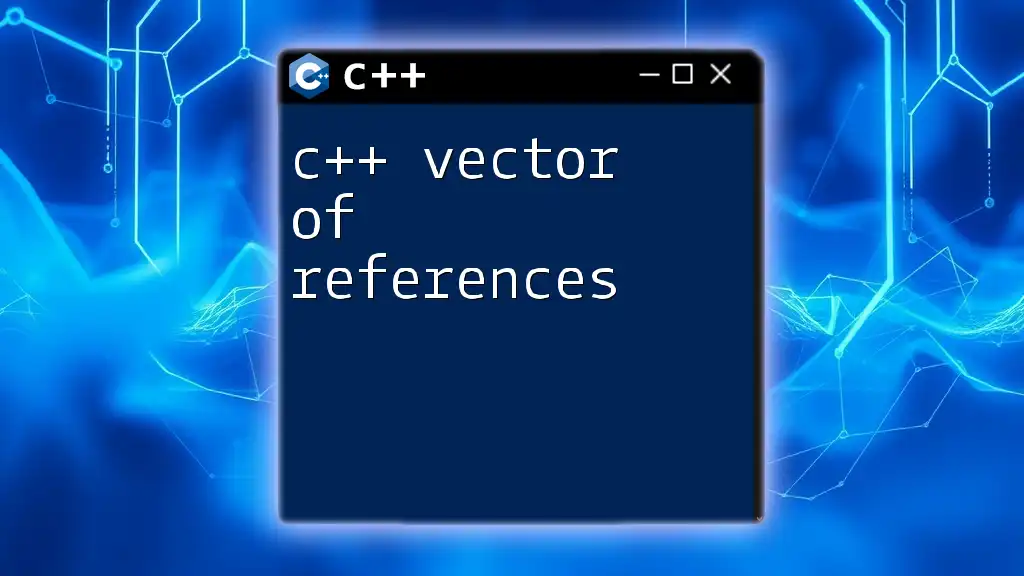
Best Practices when Using Vectors of Objects
Memory Management Considerations
When working with vectors of objects, it’s crucial to ensure proper memory management. Constructors and destructors automatically handle memory allocation and deallocation. Always ensure that the resources allocated by the object are released properly to avoid memory leaks.
Performance Considerations
When dealing with large vectors, consider reserving the space in advance using the `reserve()` function. This minimizes reallocation costs and improves performance. For instance:
students.reserve(100); // Reserve space for 100 students
This strategy helps avoid costly reallocations as the vector grows.
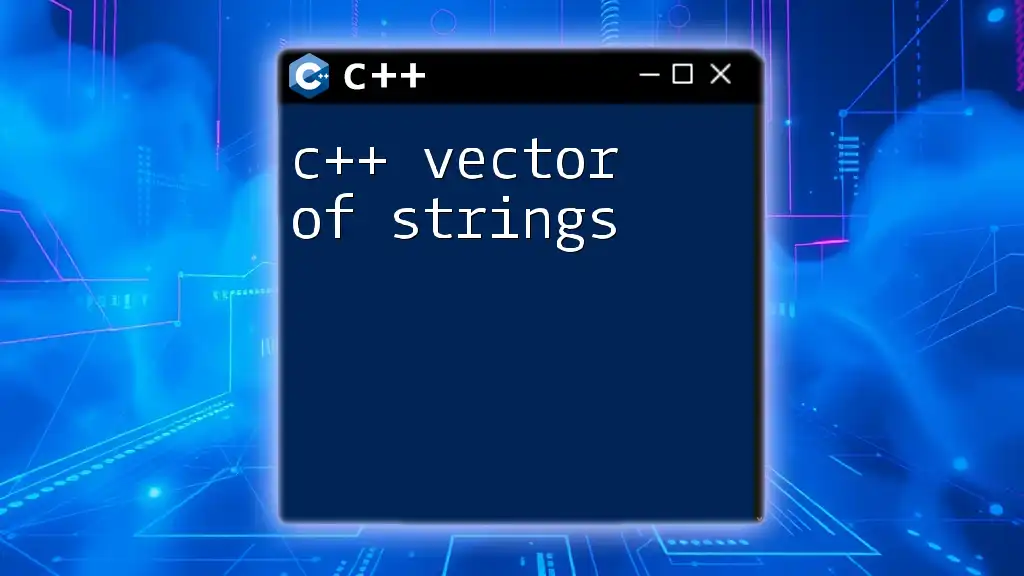
Conclusion
C++ vectors of objects are an essential element of modern C++ programming, providing flexibility and powerful data management capabilities. Understanding how to effectively declare, manipulate, and iterate over these objects is vital for developing efficient and robust applications. Practice with these concepts will enhance your coding skills and prepare you for more complex programming challenges.
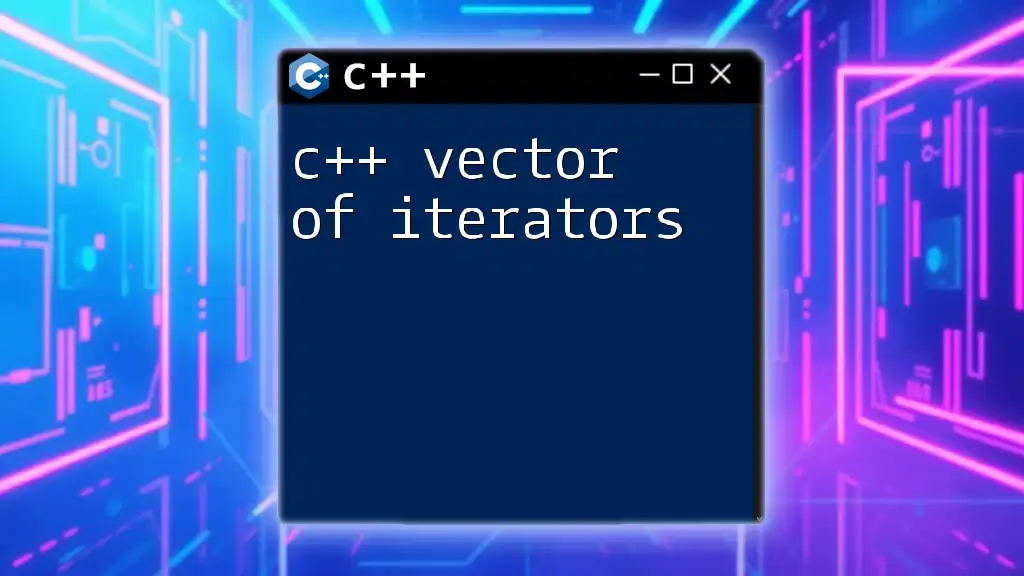
Additional Resources
For those eager to delve deeper into C++ vectors and object-oriented programming, the official C++ documentation and recommended books provide excellent information and examples to enhance your learning experience. Happy coding!