C++ projects are practical applications that utilize the C++ programming language to create software solutions, allowing learners to apply their knowledge through hands-on experience.
Here’s a simple C++ code snippet that demonstrates a basic "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Projects
What is a C++ Project?
A C++ project is a structured collection of code, libraries, and resources designed to accomplish a specific task using the C++ programming language. These projects can range from simple console applications to complex software systems. Engaging in C++ projects is crucial for anyone learning the language as it enables practical application of theoretical concepts. Moreover, C++ finds its use in a myriad of real-world applications, including game development, system software, and high-performance servers.
Setting Up Your C++ Development Environment
Before diving into C++ projects, setting up your development environment is essential for a seamless coding experience.
Choosing an Integrated Development Environment (IDE) is the first step. Popular options include Visual Studio, a robust choice for Windows users; Code::Blocks, which is platform-independent; and CLion, a powerful IDE with excellent features for modern C++ development.
Installing the necessary compilers is also crucial to compile and run your C++ code. You can use GCC for Linux users, Clang for macOS, and MSVC for Windows environments. Each of these compilers has its own setup procedures, which you can find on their official documentation sites.
To create a simple “Hello, World!” project, choose an IDE, create a new project, and enter the following code:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This code snippet is a fundamental building block in learning C++. It introduces you to output streams, which is vital for interacting with users.
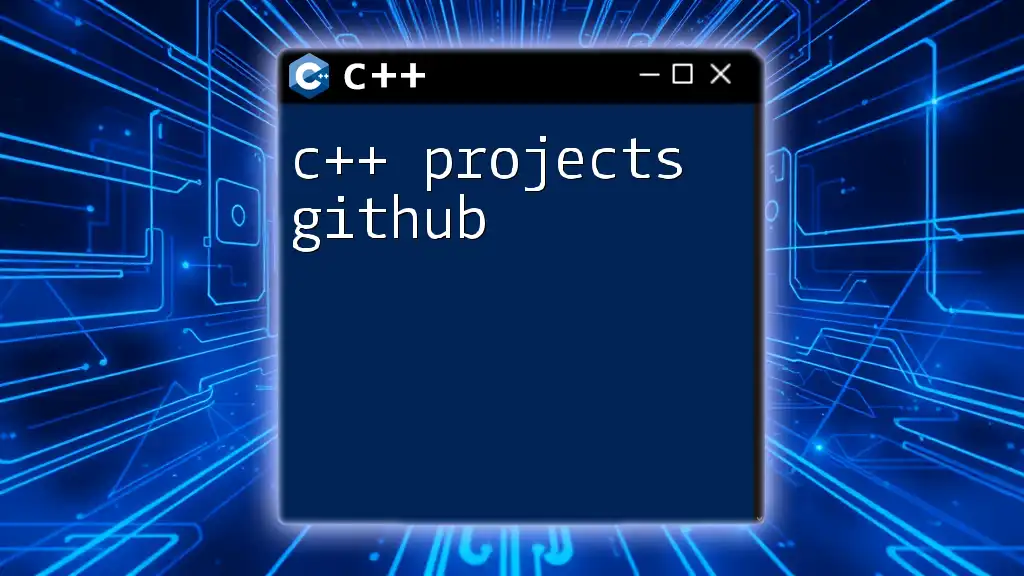
Types of C++ Projects
Beginner Level Projects
Simple Calculator
A simple calculator is a great starting project that helps reinforce the fundamentals of C++. Its basic purpose is to perform arithmetic operations like addition, subtraction, multiplication, and division. Here’s a code snippet to illustrate this:
#include <iostream>
int main() {
double num1, num2;
char operation;
std::cout << "Enter first number: ";
std::cin >> num1;
std::cout << "Enter second number: ";
std::cin >> num2;
std::cout << "Enter operation (+, -, *, /): ";
std::cin >> operation;
switch (operation) {
case '+':
std::cout << "Result: " << num1 + num2 << std::endl;
break;
case '-':
std::cout << "Result: " << num1 - num2 << std::endl;
break;
case '*':
std::cout << "Result: " << num1 * num2 << std::endl;
break;
case '/':
std::cout << "Result: " << num1 / num2 << std::endl;
break;
default:
std::cout << "Invalid operation!" << std::endl;
}
return 0;
}
This project teaches you how to handle user input and use conditionals effectively.
Tic-Tac-Toe
Developing a Tic-Tac-Toe game gains deeper insights into logic and user interaction. The game consists of two players alternately marking spaces in a 3x3 grid, attempting to achieve three markers in a row.
Here’s a simplified version of the game’s core logic:
#include <iostream>
char board[3][3] = { {' ', ' ', ' '}, {' ', ' ', ' '}, {' ', ' ', ' '} };
void printBoard() {
// Function to print the current state of the board
for (int i = 0; i < 3; i++) {
std::cout << " " << board[i][0] << " | " << board[i][1] << " | " << board[i][2] << std::endl;
if (i < 2) std::cout << "---|---|---" << std::endl;
}
}
int main() {
// Game loop and logic would go here
return 0;
}
This project introduces basic array usage and function creation, which are integral to more complex programming tasks.
Intermediate Level Projects
Text-based Adventure Game
Creating a text-based adventure game allows you to explore branching narratives. Players make choices that influence the story's direction. Here is an example of code showcasing a branching decision:
#include <iostream>
void startGame() {
std::cout << "You are in a dark room. Do you go left or right? (l/r) ";
char choice;
std::cin >> choice;
if (choice == 'l') {
std::cout << "You encounter a dragon! Game over." << std::endl;
} else {
std::cout << "You find a treasure chest! You win!" << std::endl;
}
}
int main() {
startGame();
return 0;
}
This example demonstrates user input handling and conditional statements, which are crucial in many programming scenarios.
File Management System
A file management system project introduces you to data handling capabilities. The project could involve functions to create, read, and update text files. Here’s how a simple read operation might look:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("example.txt");
std::string line;
if (file.is_open()) {
while (getline(file, line)) {
std::cout << line << std::endl;
}
file.close();
} else {
std::cout << "Unable to open file." << std::endl;
}
return 0;
}
Working with files enhances your understanding of data persistence and error handling.
Advanced Level Projects
Personal Finance Tracker
This project aims to help users track expenses and set budgets. Using STL containers can enhance the functionality. Here’s an example of how you might store and display expenses:
#include <iostream>
#include <vector>
struct Expense {
std::string name;
double amount;
};
int main() {
std::vector<Expense> expenses;
expenses.push_back({"Coffee", 2.5});
expenses.push_back({"Groceries", 50.0});
double total = 0;
for (const auto& exp : expenses) {
std::cout << exp.name << ": $" << exp.amount << std::endl;
total += exp.amount;
}
std::cout << "Total Expenses: $" << total << std::endl;
return 0;
}
This code snippet illustrates the use of structs and vectors, fundamental C++ concepts that are widely applicable.
Chat Application
A chat application can introduce you to more complex concepts, such as client-server architecture and real-time communication. Implementing socket programming is crucial here. Here’s a basic launch setup for a server:
#include <iostream>
#include <cstring>
#include <sys/socket.h>
#include <netinet/in.h>
int main() {
int server_fd = socket(AF_INET, SOCK_STREAM, 0);
struct sockaddr_in address;
int opt = 1;
int addrlen = sizeof(address);
setsockopt(server_fd, SOL_SOCKET, SO_REUSEADDR, &opt, sizeof(opt));
address.sin_family = AF_INET;
address.sin_addr.s_addr = INADDR_ANY;
address.sin_port = htons(8080);
bind(server_fd, (struct sockaddr *)&address, sizeof(address));
listen(server_fd, 3);
std::cout << "Server is listening on port 8080" << std::endl;
return 0;
}
This snippet sets up a basic server that listens for incoming connections, representing a foundation for real-time communication in a chat application.
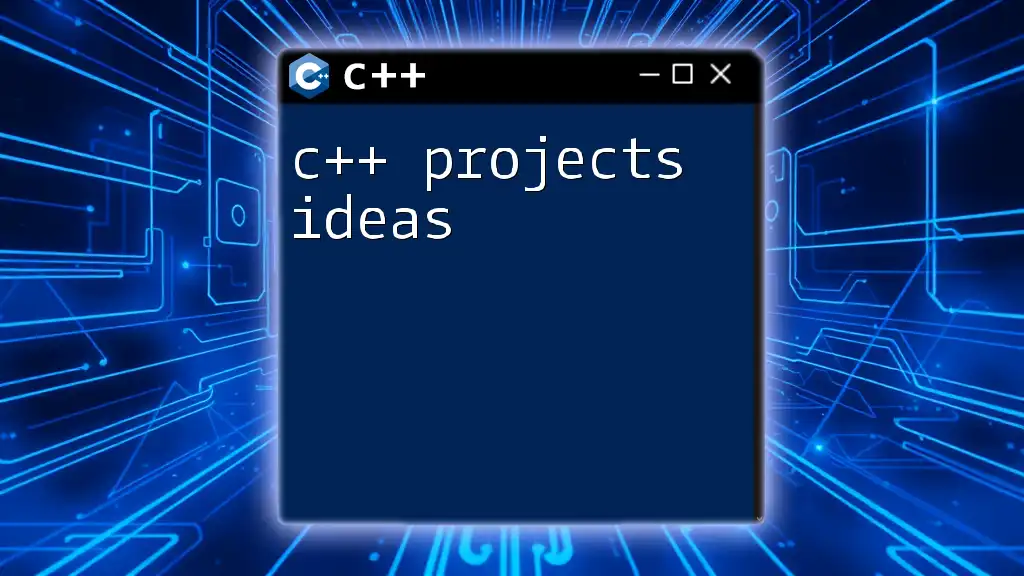
Best Practices for C++ Projects
Code Organization and Structure
Effectively organizing your code is crucial in larger C++ projects. Modular programming enhances readability and reusability. A well-structured directory might contain separate folders for source files, header files, and resources.
Using namespaces and classes can help avoid name clashes and encapsulate functionality, respectively, making your codebase more manageable.
Version Control
Incorporating version control, especially with Git, is vital for any C++ project. Begin by creating a new repository using:
git init
You can track changes to your files through commands like `git add`, `git commit`, and `git push`. Understanding version control fosters collaboration and minimizes potential issues.
Testing and Debugging
Testing should be an integral part of your C++ projects. Using frameworks like Google Test can help automate your testing processes and ensure that your code remains robust against future changes.
Debugging is equally important. Tools such as gdb offer advanced functionality to pinpoint issues within your code. Learning effective debugging techniques will pay off significantly as your projects grow in complexity.
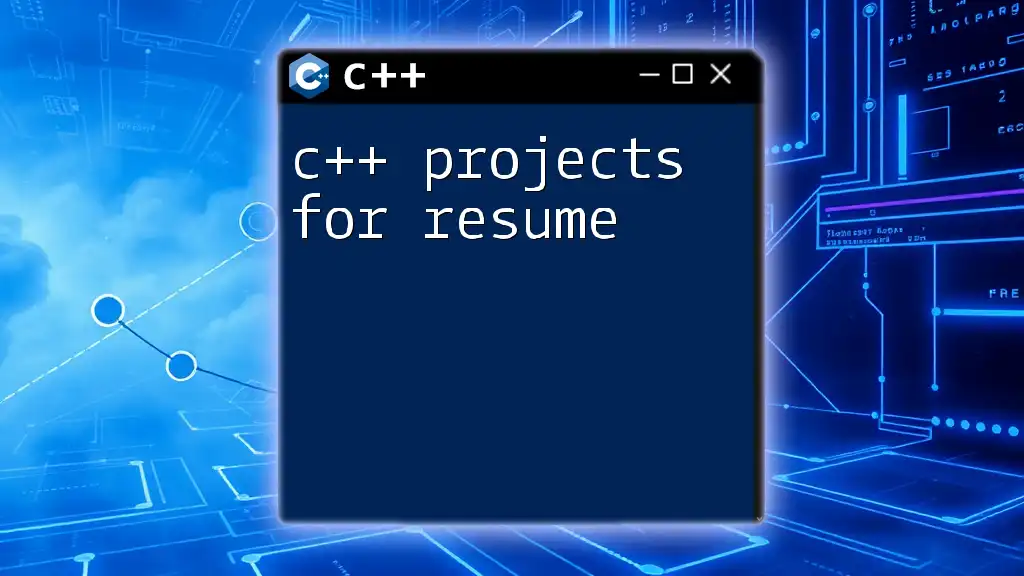
Conclusion
Engaging with C++ projects is not merely about coding but is a pathway to mastering the intricacies of programming. Each project type, from simple calculators to chat applications, builds upon essential concepts that are fundamental to C++. By consistently working on projects, following best practices, and employing version control and debugging tools, you pave the way for continuous growth and skill enhancement in the world of C++. Embrace the challenge, explore new ideas, and keep learning as you progress in your C++ journey.