Creating C++ projects not only enhances your programming skills but also significantly boosts your resume by showcasing practical experience and problem-solving abilities to potential employers.
Here's a simple example of a C++ program that calculates the factorial of a number:
#include <iostream>
using namespace std;
int factorial(int n) {
return (n <= 1) ? 1 : n * factorial(n - 1);
}
int main() {
int num;
cout << "Enter a number: ";
cin >> num;
cout << "Factorial of " << num << " is " << factorial(num) << endl;
return 0;
}
Why C++ Projects Matter for Your Resume
C++ projects are crucial for showcasing your practical experience and technical capabilities. Unlike theoretical knowledge, which may only indicate what you know, completed projects speak volumes about your abilities to apply concepts in real-world scenarios. They give prospective employers a tangible output of your skills, and they highlight your commitment to learning and improvement.
Employers today are inundated with candidates who may possess a similar educational background. However, showcasing significant projects on your resume can set you apart from the competition. These projects can reveal your problem-solving abilities, creativity, and proficiency with C++, which are all traits that employers value immensely.
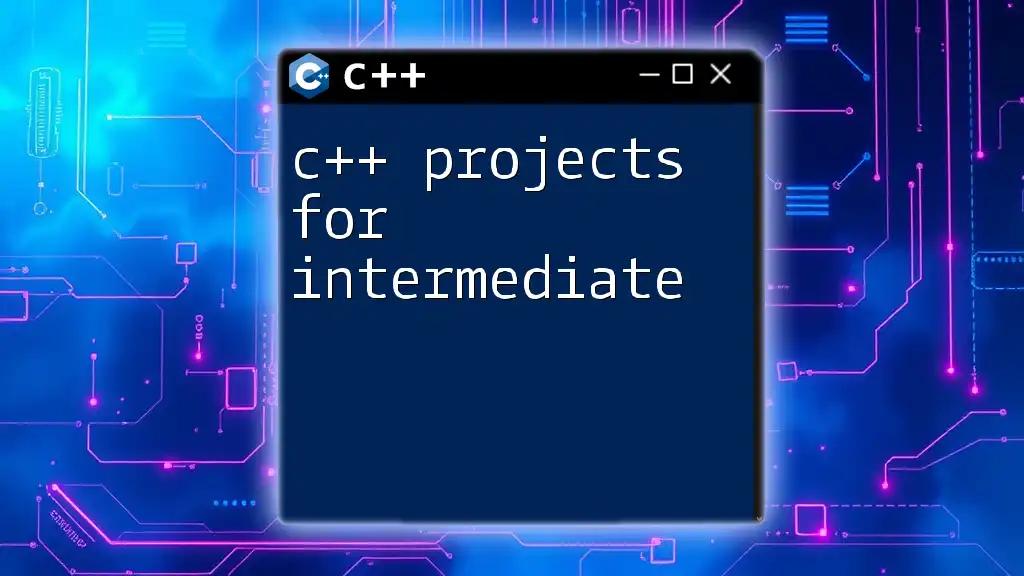
Choosing the Right C++ Projects
When selecting projects, it's essential to consider your skill level and the relevance to your desired job role.
Consider Your Skill Level
Selecting a project that aligns with your current abilities is crucial. If you are a beginner, consider starting with simpler projects that introduce you to C++ basics. As you grow more confident and skilled, you can transition to intermediate and advanced projects.
Relevance to Your Desired Job
Research jobs that pique your interest to discover the C++ skills that are in high demand. By aligning your projects with these market needs, you can enhance your employability in your chosen industry. For instance, if you're interested in game development, working on C++ projects in that space will be more advantageous than unrelated projects.
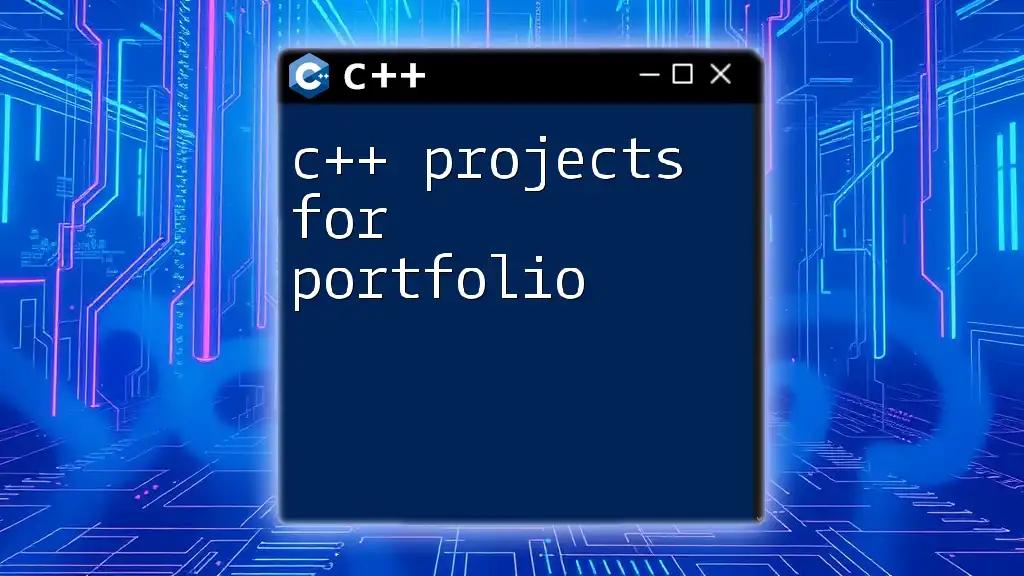
Types of C++ Projects to Consider
Here are some engaging projects across various domains:
Game Development Projects
Overview
C++ is widely used in the gaming industry due to its performance and efficiency. As a result, game development projects can make a striking impression on your resume.
Example Projects
-
Simple Text-Based Game A straightforward way to dip your toes in game development is by creating a text-based adventure or quiz game. The fundamental structure might look like this:
#include <iostream> using namespace std; void startGame() { cout << "Welcome to the Adventure Game!" << endl; // Game logic here } int main() { startGame(); return 0; }
-
2D Game Using Libraries (e.g., SFML) For something more visually stimulating, consider using libraries like SFML to create a simple 2D platformer. Start by setting up a window:
#include <SFML/Graphics.hpp> int main() { sf::RenderWindow window(sf::VideoMode(800, 600), "My first window"); while (window.isOpen()) { sf::Event event; while (window.pollEvent(event)) { if (event.type == sf::Event::Closed) window.close(); } window.clear(); // Draw here window.display(); } return 0; }
Financial Applications
Overview
Financial applications often require complex calculations and data management, making them suitable for demonstrating your skill in C++.
Example Projects
-
Budget Tracker A budget management application can help you practice user input and data storage. Here’s how a simple implementation might begin:
#include <iostream> #include <vector> using namespace std; int main() { vector<double> expenses; double expense; cout << "Enter your expenses (-1 to end): "; while (cin >> expense && expense != -1) { expenses.push_back(expense); } // Logic for tracking expenses goes here }
-
Stock Market Simulator Simulating stock trading offers a complex and rewarding project. You can manage transactions through classes and methods:
class Stock { public: void buy(int shares) { // Code for buying shares } void sell(int shares) { // Code for selling shares } // More methods and properties };
Data Structures and Algorithms
Overview
Understanding and implementing data structures is foundational in computer science. C++ effectively supports these implementations.
Example Projects
-
Custom Data Structure Library Creating your own library for data structures like stacks or queues can be an excellent way to demonstrate technical expertise. Here’s how you might implement a basic stack:
#include <vector> class Stack { vector<int> elements; public: void push(int value) { elements.push_back(value); } void pop() { if (!elements.empty()) elements.pop_back(); } // More stack functionalities };
-
Algorithm Visualizer Visualizing algorithms (such as sorting and searching) can be an engaging project that combines programming and design. For example, you might write a simple representation of the bubble sort algorithm:
void bubbleSort(vector<int>& arr) { int n = arr.size(); for (int i = 0; i < n-1; i++) for (int j = 0; j < n-i-1; j++) if (arr[j] > arr[j+1]) swap(arr[j], arr[j+1]); }
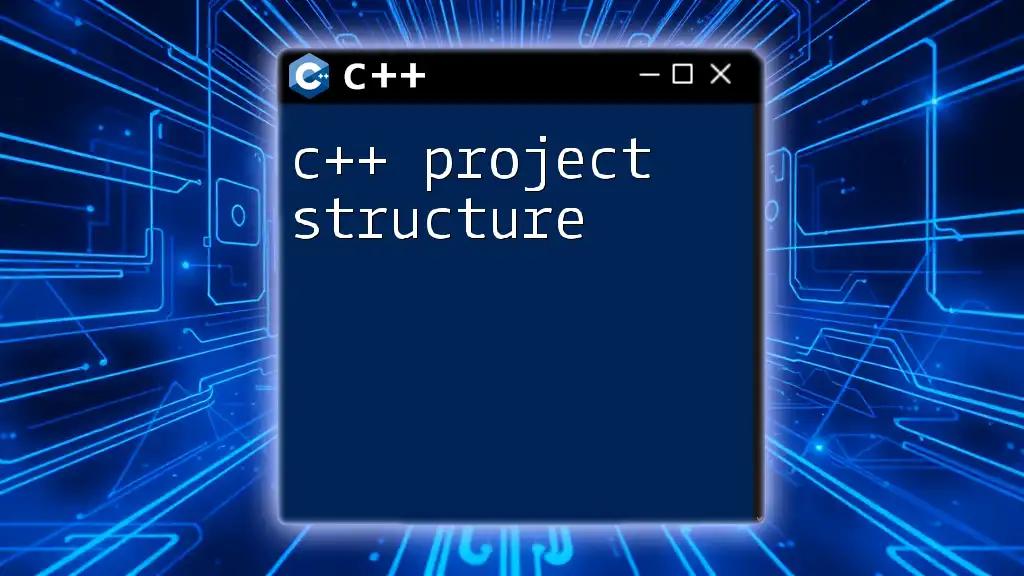
Best Practices for Showcasing Projects on Your Resume
Documenting Your Work
Clean and clear documentation is crucial. Without proper explanations, your code may be difficult to understand, even if the logic is sound. A well-written README file is essential and should include installation instructions, usage notes, and examples.
Using Version Control (e.g., Git)
Using Git for version control can demonstrate your ability to work in collaborative environments. An active GitHub repository showcasing your projects signals organization and professionalism.
Highlighting Key Technologies and Skills
When describing your projects on your resume, make sure to include relevant languages, libraries, and tools. This can give potential employers clearer insights into your skill set.
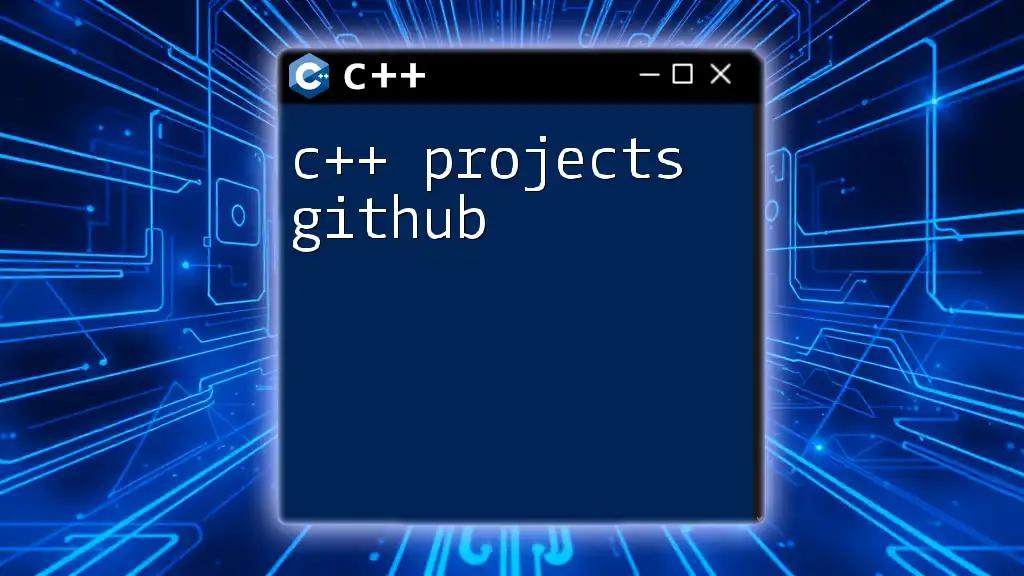
How to Present Your C++ Projects
Creating a Portfolio
An online portfolio is an excellent way to display your projects. Platforms like GitHub and personal websites allow you to present your work visually and textually, making it easier for employers to understand your projects at a glance.
Building a CV/Resume
Include a dedicated section for your projects on your resume. Use action verbs to describe what you accomplished with these projects, mentioning programming concepts, methodologies, and technologies used.
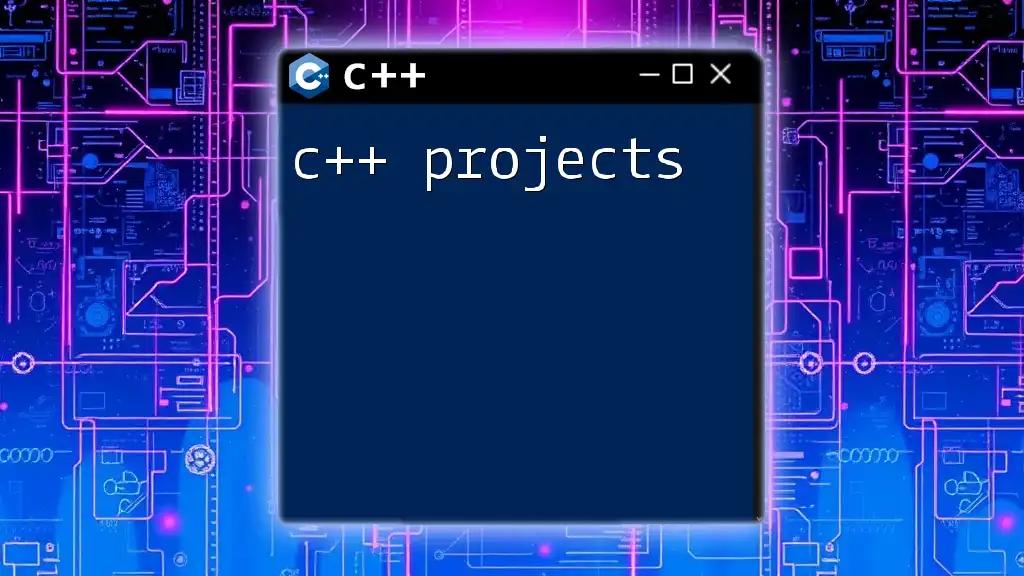
Conclusion
C++ projects can significantly enhance your resume and career prospects. They showcase your practical skills, demonstrate your interests, and align your abilities with what employers are seeking.
By engaging in meaningful projects, you can not only improve your skills but also build a compelling case for your candidacy in the job market. Start building your C++ project portfolio today to set the stage for your professional future.

Call to Action
What projects have you worked on that you’re proud of? Share your experiences or favorite C++ projects in the comments! If you’re eager to learn more, explore our additional resources to continue developing your C++ expertise.