In C++, perfect forwarding allows you to pass arguments to functions while preserving their value category (lvalue or rvalue), enabling efficient and flexible function templates.
Here's a simple example using `std::forward` to achieve perfect forwarding:
#include <iostream>
#include <utility>
template<typename T>
void wrapper(T&& arg) {
process(std::forward<T>(arg));
}
void process(int& x) {
std::cout << "Lvalue reference: " << x << std::endl;
}
void process(int&& x) {
std::cout << "Rvalue reference: " << x << std::endl;
}
int main() {
int a = 10;
wrapper(a); // Calls the lvalue reference overload
wrapper(20); // Calls the rvalue reference overload
}
What is Perfect Forwarding?
C++ perfect forwarding is a technique that allows you to forward arguments to another function while retaining their value category (lvalue or rvalue). This ensures that objects are moved or copied appropriately without unnecessary overhead, thereby enhancing performance and efficiency.
In modern C++ programming, perfect forwarding is crucial. It minimizes code duplication and lets you write more generic and efficient code. By leveraging templates and the `std::forward` function, developers can create versatile functions that handle a variety of types seamlessly.
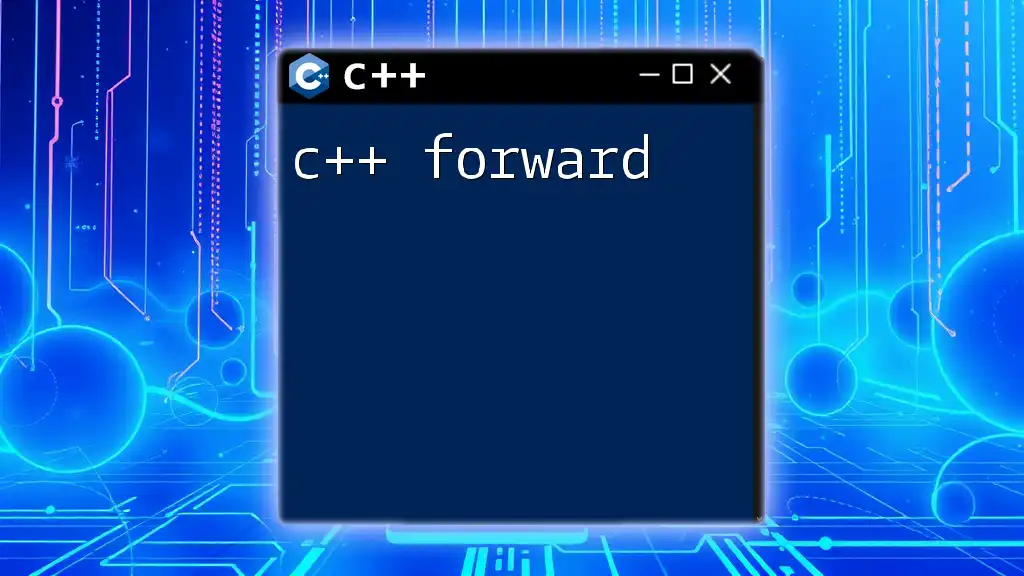
Understanding rvalue and lvalue References
What are rvalue and lvalue References?
In C++, references can be categorized mainly into lvalue references and rvalue references:
- Lvalues are named objects that have an identifiable location in memory (e.g., a variable).
- Rvalues are temporary objects that do not have a specific memory location (e.g., the result of a computation).
Lvalues vs Rvalues: A Deep Dive
- Lvalue Example:
int x = 10; // x is an lvalue
- Rvalue Example:
int y = x + 5; // x + 5 is an rvalue
Understanding these types is essential for effectively utilizing perfect forwarding since the value category determines how values can be manipulated.
The Role of Reference Collapsing
Reference collapsing simplifies the types of references when used in templates. The rules are as follows:
- `T& &` becomes `T&`
- `T& &&` becomes `T&`
- `T&& &` becomes `T&`
- `T&& &&` becomes `T&&`
Here’s a simple example demonstrating how reference collapsing works:
template<typename T>
void showType(T&& arg) {
// This resolves to either T& or T&& based on the input
}
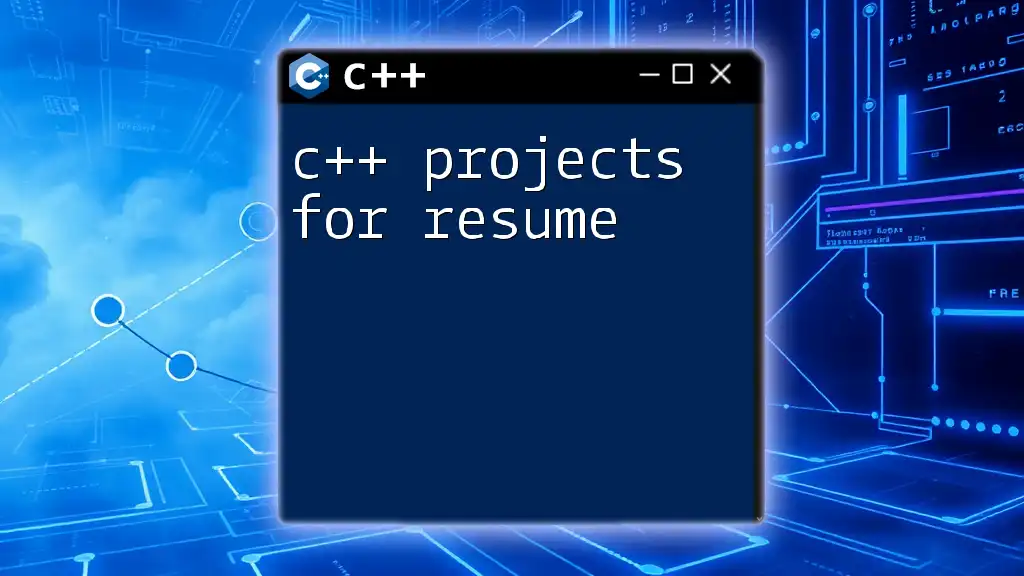
The Role of `std::forward`
What is `std::forward`?
`std::forward` is a utility function in C++ that enables the perfect forwarding of arguments. It is used within template functions to preserve the value category of the argument being forwarded.
Using `std::forward` in Practice
To demonstrate `std::forward`, consider the following implementation:
template<typename T>
void wrapper(T&& arg) {
func(std::forward<T>(arg));
}
In this example, `arg` is forwarded to `func` while preserving its original type, making it either an lvalue or an rvalue.
This is particularly beneficial when working with functions accepting both lvalues and rvalues, as it avoids unnecessary copies and moves.
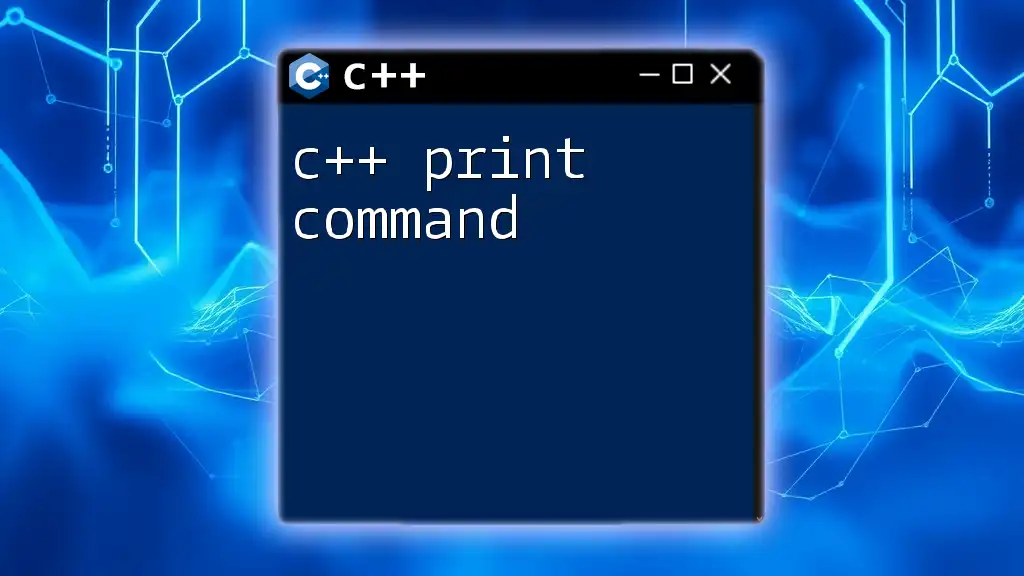
Implementing Perfect Forwarding
Creating a Function Template with Perfect Forwarding
To implement perfect forwarding effectively, create a function template as follows:
template<typename T>
void process(T&& arg) {
// Some processing logic here
}
Here, the `process` function can accept both lvalues and rvalues without any loss of performance due to copying.
Ensuring Correct Value Categories
When implementing perfect forwarding, it’s vital to preserve the original value categories to prevent unintended copies or moves. Consider this example:
void function(int& x) {
// Handle lvalue
}
void function(int&& y) {
// Handle rvalue
}
template<typename T>
void forwardFunc(T&& arg) {
function(std::forward<T>(arg));
}
When calling `forwardFunc`, the right overload of `function` will be invoked based on whether the argument is an lvalue or rvalue.
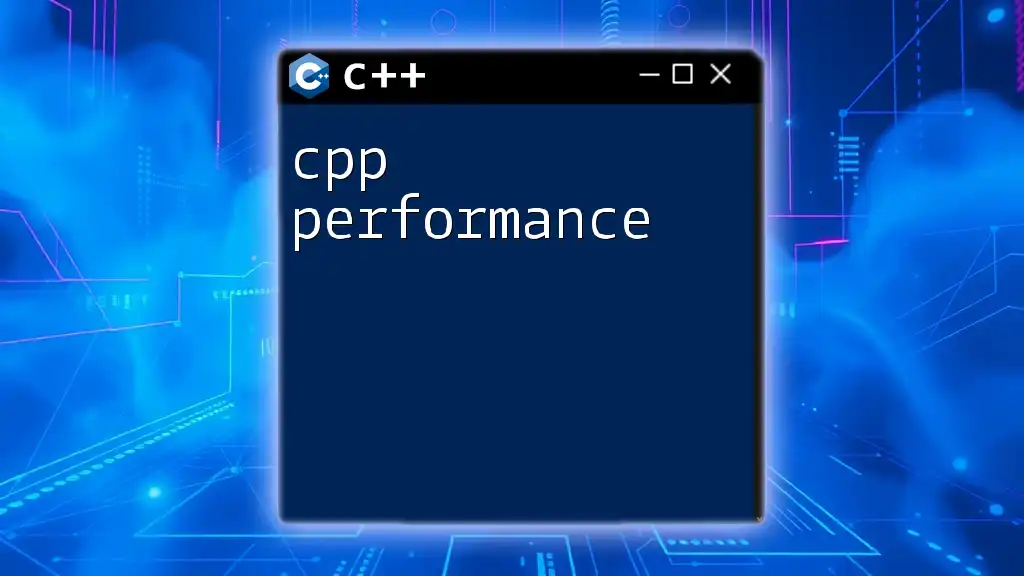
Common Pitfalls and How to Avoid Them
Overusing Perfect Forwarding
While perfect forwarding is powerful, it can lead to overly complex code. Use this technique judiciously. If a function does not need to forward parameters, consider using simpler approaches.
Confusion Between `std::move` and `std::forward`
Though they seem similar, `std::move` and `std::forward` serve different purposes. `std::move` casts an object to an rvalue, forcing a move, while `std::forward` preserves the original value category. Here’s a common mistake:
template<typename T>
void incorrectForward(T&& arg) {
// This will always convert arg to an rvalue
func(std::move(arg)); // Too aggressive
}
Using Perfect Forwarding on Non-Forwardable Types
Perfect forwarding also fails when applied to types that cannot be moved or copied (e.g., `std::unique_ptr`). It's critical to ensure that the types being forwarded support these operations.
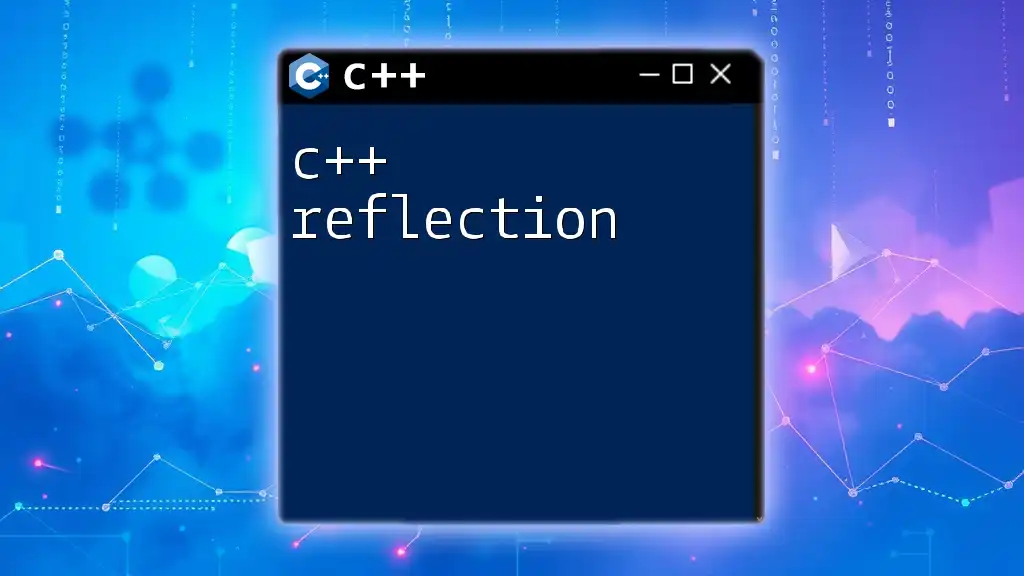
Best Practices for Perfect Forwarding
When to Implement Perfect Forwarding in Your Code
Implement perfect forwarding primarily in scenarios where:
- You are writing library functions or generic code.
- You want to preserve the value category for optimization.
- Function templates need to accommodate both lvalues and rvalues.
Writing Clean and Readable Code with Perfect Forwarding
Ensure your code remains clean and maintainable:
- Use clear and concise naming for your template and function parameters.
- Organize code logically and include relevant comments to clarify complex implementations.
Performance Considerations
While perfect forwarding can enhance efficiency, it’s essential to analyze its impact. Use benchmarking tools to evaluate performance differences in various contexts before integrating it into critical code paths.
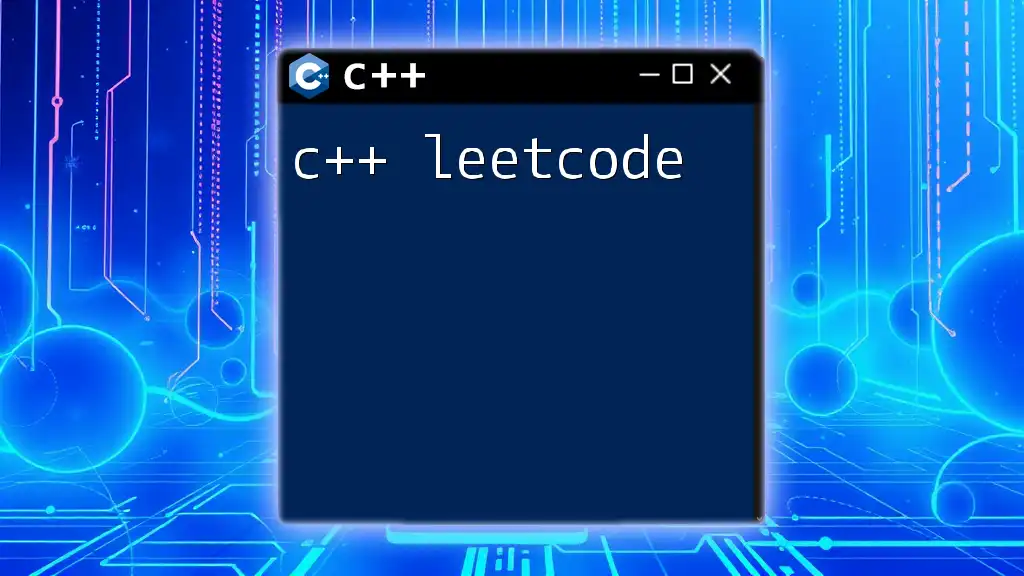
Real-World Applications of Perfect Forwarding
Using Perfect Forwarding in C++ Libraries
Consider how C++ standard libraries often utilize perfect forwarding:
template<typename T>
void push_back(std::vector<T>& vec, T&& element) {
vec.emplace_back(std::forward<T>(element));
}
This function allows users to add elements to a vector without unnecessary copies.
Case Study Analysis
In a recent project, a developer used perfect forwarding to create a logging utility that efficiently handled various data types. The performance gain was notable, especially when processing large datasets, showcasing the strength of this technique when applied in practice.
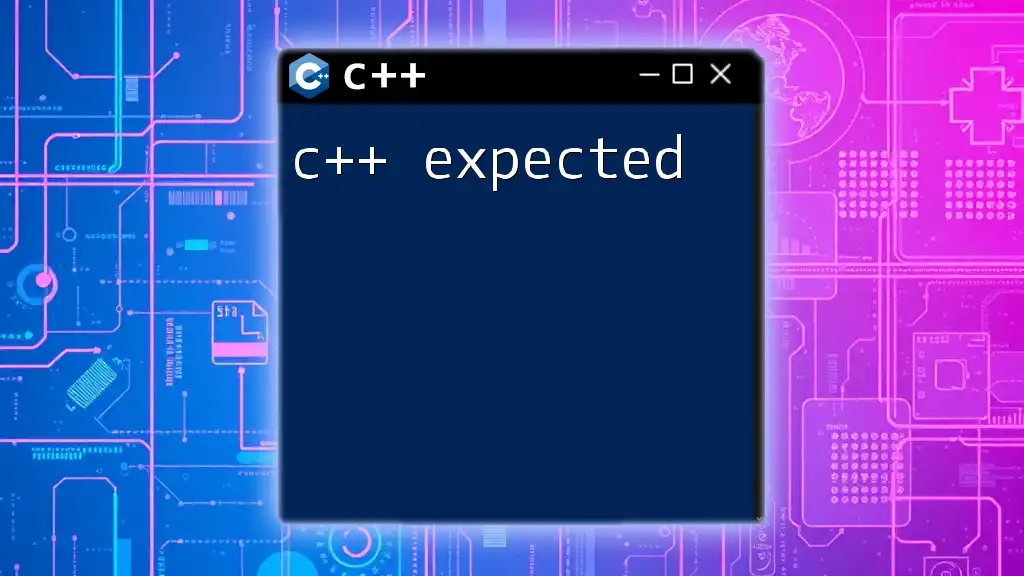
Conclusion
In summary, C++ perfect forwarding is a sophisticated technique that optimizes function argument handling. By ensuring that the value categories of arguments remain intact, developers can craft more efficient and flexible code.
As you explore this topic, practice implementing perfect forwarding in your projects, and consider the benefits it could bring to your C++ applications. For further mastery, engage with community resources, documentation, and real-world projects to enhance your understanding and skills in modern C++ programming.