In C++, parsing command-line arguments allows you to retrieve user-provided input when executing a program, typically accessed via the `argc` and `argv` parameters in the `main` function.
Here’s a simple example of how to parse command-line arguments in C++:
#include <iostream>
int main(int argc, char* argv[]) {
for (int i = 0; i < argc; ++i) {
std::cout << "Argument " << i << ": " << argv[i] << std::endl;
}
return 0;
}
What Are Command Line Arguments?
Command line arguments are inputs provided to a program at the time it is started. They enable users to customize the behavior of a program without modifying its code. When a C++ program is executed, it receives these inputs through two main components: `argc` and `argv`.
- `argc`: This integer represents the number of command line arguments passed, including the program name.
- `argv`: This is an array of C-strings, each representing an individual argument passed to the program.
For instance, if a user executes `./myProgram arg1 arg2`, `argc` will be `3` and `argv` will contain `["./myProgram", "arg1", "arg2"]`.
Let’s review a simple C++ program that utilizes command line arguments to illustrate how they work:
#include <iostream>
int main(int argc, char* argv[]) {
for (int i = 0; i < argc; ++i) {
std::cout << "Argument " << i << ": " << argv[i] << std::endl;
}
return 0;
}
When executed, this program will print all command line arguments passed to it.

C++ Parsing Command Line Arguments
Basics of Argument Parsing
When it comes to C++ parsing command line arguments, understanding how to utilize `argc` and `argv` effectively is crucial. Proper parsing is essential for building robust command line applications. Often, command line arguments are used to specify input files, output files, or various options that modify the program’s behavior.
Step-by-Step Guide to Parse Command Line Arguments
Setting Up Your Development Environment
To get started with your C++ argument parser, set up an Integrated Development Environment (IDE) such as Visual Studio, Code::Blocks, or CLion. Make sure a compatible C++ compiler (like GCC or Clang) is installed. Your project structure might look like this:
/my_cpp_project
│
├── main.cpp
└── CMakeLists.txt
Writing the Command Line Argument Parser
Creating a simple command line argument parser involves writing a function that reads and processes each argument. Here’s an example of how to structure your parser:
#include <iostream>
#include <string>
void parseArguments(int argc, char** argv) {
for (int i = 1; i < argc; ++i) { // Start from index 1, index 0 is the program name
std::string arg = argv[i];
std::cout << "Parsed argument: " << arg << std::endl;
}
}
int main(int argc, char** argv) {
parseArguments(argc, argv);
return 0;
}
When executed with arguments, this program outputs each argument passed to it, demonstrating a fundamental parsing mechanism.
Advanced C++ Argument Parsing Techniques
Using Libraries for Argument Parsing
While writing a parser from scratch is valuable for understanding, leveraging existing libraries can streamline development. Popular libraries for C++ argument parsing include:
- Boost.ProgramOptions: This powerful library enables users to define options and parse command line arguments easily.
- cxxopts: A lightweight, easy-to-use library.
Using libraries offers significant advantages, such as cleaner code and built-in error handling.
Example: Using Boost.ProgramOptions
To use the Boost.ProgramOptions library, first ensure it is properly installed in your project. Here’s how to implement a parser using this library:
#include <boost/program_options.hpp>
#include <iostream>
namespace po = boost::program_options;
int main(int argc, char* argv[]) {
po::options_description desc("Allowed options");
desc.add_options()
("help,h", "produce help message")
("input,i", po::value<std::string>(), "input file")
("output,o", po::value<std::string>(), "output file");
po::variables_map vm;
po::store(po::parse_command_line(argc, argv, desc), vm);
po::notify(vm);
if (vm.count("help")) {
std::cout << desc << "\n";
return 0;
}
if (vm.count("input")) {
std::cout << "Input file: " << vm["input"].as<std::string>() << "\n";
}
return 0;
}
This code snippet illustrates how to set up command line options, handle help requests, and parse input file arguments seamlessly.

Best Practices for C++ Argument Parsing
Keep It User-Friendly
When developing a command line application, clarity is key. Make sure to provide informative help messages. Users should easily understand the expected inputs and the available options.
Error Handling in Argument Parsing
Proper error handling is integral to creating a robust parser. Check the validity of the provided arguments and ensure they meet the expected criteria. For example:
if (!vm.count("input")) {
std::cerr << "Input file must be specified!\n";
return 1;
}
This snippet validates that an input file has been provided, improving the user experience by preventing unclear error states.

Debugging Your Command Line Argument Parser
Debugging argument parsing can be challenging. Utilize print debugging by displaying parsed arguments throughout the parsing sequence. This will help ensure that expected inputs appear as intended. Additionally, integrating logging frameworks can provide more systematic tracking of command line arguments.
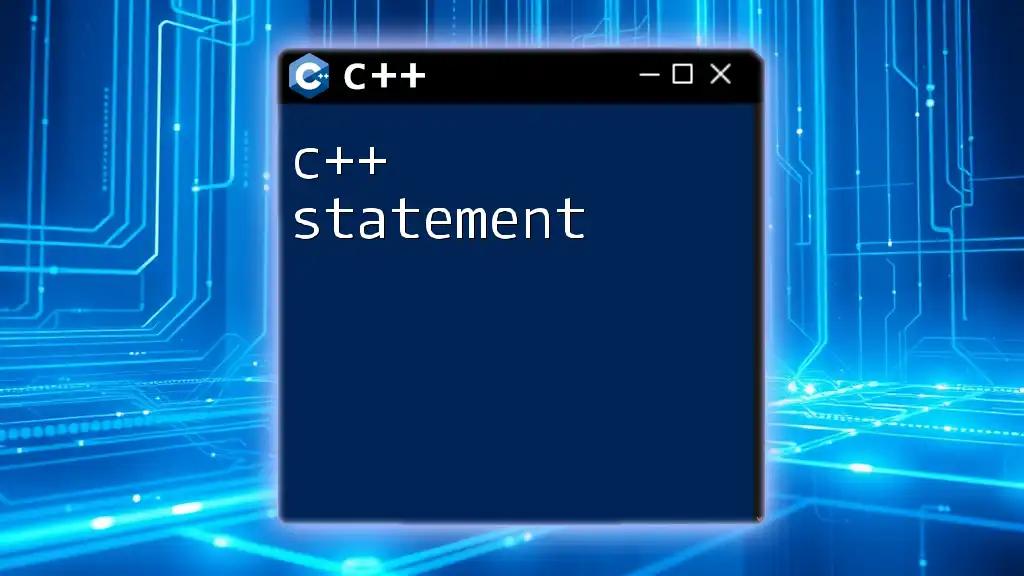
Conclusion
Mastering how to C++ parse arguments is crucial for creating powerful command line applications. By understanding the mechanics of `argc` and `argv`, implementing simple parsing techniques, and leveraging libraries like Boost.ProgramOptions, developers can enhance the usability of their programs.
A solid grasp of argument parsing will not only facilitate robust applications but also allow developers to focus on delivering greater functionality. Practice with various examples and engage with the community to share experiences and improvements in your command line argument handling skills.
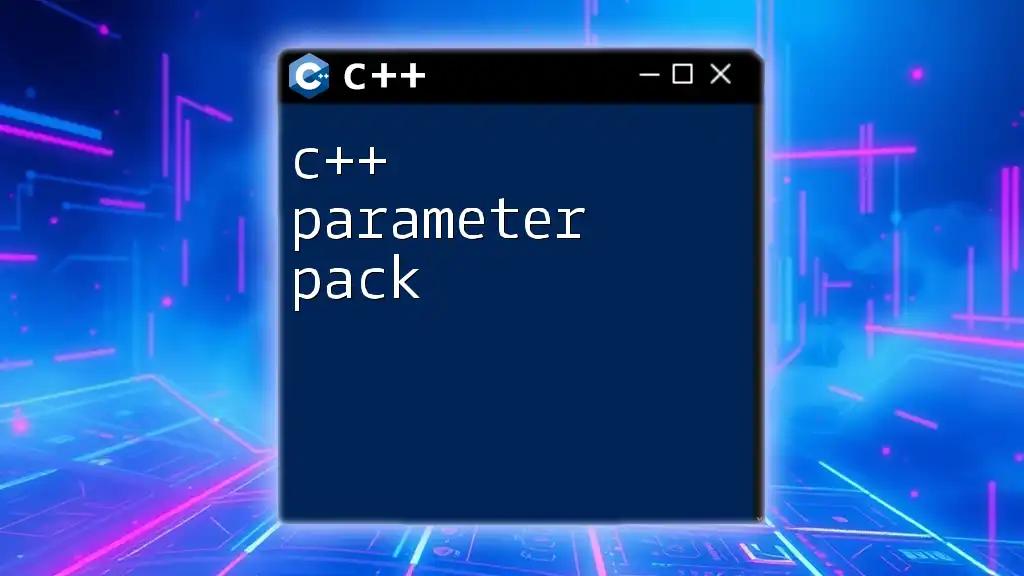
Additional Resources
For further exploration, check out the official documentation for Boost.ProgramOptions and other relevant libraries. Books and articles dedicated to advanced C++ programming will also deepen your understanding and enhance your skills. Embrace the challenges of argument parsing and push the boundaries of your command line application!