In C++, optional arguments (also known as default parameters) allow you to specify default values for function parameters that can be omitted when calling the function.
Here's an example:
#include <iostream>
void greet(const std::string& name = "Guest") {
std::cout << "Hello, " << name << "!" << std::endl;
}
int main() {
greet(); // Outputs: Hello, Guest!
greet("Alice"); // Outputs: Hello, Alice!
return 0;
}
What are Optional Parameters in C++?
Definition of Optional Parameters
In C++, optional parameters allow you to define default values for function arguments. This means you can call a function without specifying all of its arguments, as the omitted arguments will take on predefined default values. Mandatory parameters, in contrast, must be provided during the function call. Optional parameters provide flexibility, enabling developers to create more versatile functions without repeating code through overloads.
Syntax of Optional Parameters
To define optional parameters, simply assign a value to the parameter within the function declaration. The syntax is straightforward and looks like this:
void func(int a, int b = 10) {
// function implementation
}
In this example, the function `func` takes two integer parameters: `a`, which is mandatory, and `b`, which is optional and defaults to 10 if not provided when the function is called.
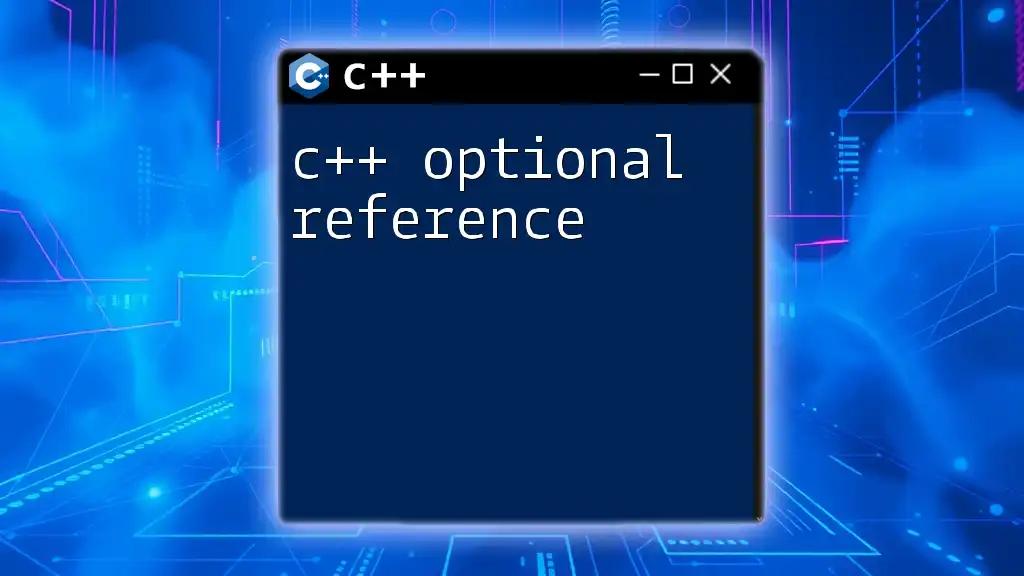
Benefits of Using C++ Optional Arguments
Enhanced Code Readability
Utilizing optional parameters can significantly enhance code readability by simplifying the function signature. For instance, instead of having multiple versions of a function that accept different numbers of parameters, you can define one function with optional arguments. This makes understanding the function's purpose easier.
Default Behavior with Optional Parameters
Optional parameters provide default values, simplifying function behavior by reducing the amount of code necessary to handle different cases. Consider this example:
void display(int x, int y = 5) {
std::cout << "X: " << x << ", Y: " << y << std::endl;
}
In this case, `y` defaults to 5 if not provided during the function call. Thus, calling `display(10)` outputs `X: 10, Y: 5`, while `display(10, 20)` outputs `X: 10, Y: 20`.
Fewer Function Overloads
Another significant advantage of optional parameters is that they reduce the need for multiple function overloads. For instance, consider an overloaded setup that looks like this:
// Overloaded version
void print(int x);
void print(int x, int y);
// Optional parameter version
void print(int x, int y = 0);
By using optional parameters, the code is simplified and more maintainable since fewer distinct functions need to be managed.

How to Define C++ Optional Arguments
Setting Default Values
When defining optional parameters, it’s crucial to set default values that are meaningful and appropriate for the function's context. Default values can either be literals or expressions. For example:
void configure(int timeout = 30, bool verbose = true) {
// function implementation
}
In this `configure` function, if a caller does not specify `timeout` or `verbose`, they will default to 30 seconds and `true`, respectively.
Order of Parameters
In C++, the order of parameters is critical. Mandatory parameters must precede optional parameters in the function definition. Failing to adhere to this rule results in compilation errors. This incorrect definition illustrates the point:
// Incorrect: must place required parameters before optional ones
void incorrectFunc(int a = 0, int b) {
// function implementation
}
Instead, the correct way would be:
void correctFunc(int a, int b = 0) {
// function implementation
}

C++ Optional Arguments in Practice
Common Use Cases of Optional Arguments
Optional parameters find broad application in numerous scenarios. For example, they’re often used for functions that configure settings or behaviors, such as logging or initialization functions. A common use case can be seen in libraries where configurations might have sensible defaults but still allow the user to specify their options.
Real-World Code Example
Here is a practical example showcasing how optional parameters can be utilized in a typical function scenario:
void calculateFee(double amount, double discount = 0.0) {
double finalAmount = amount - discount;
std::cout << "Final Amount: " << finalAmount << std::endl;
}
Calling `calculateFee(100.0)` would yield a final amount of 100.0, while `calculateFee(100.0, 20.0)` would give a final amount of 80.0.

Best Practices for Using Optional Parameters
Considerations for Performance
When deciding to use optional parameters, consider performance impacts. Although minor, the performance can be affected by the overhead of default values, especially in performance-critical applications. Therefore, weigh the trade-offs between functionality and performance based on your specific use case.
Clarity and Maintainability
While optional parameters can simplify function calls, it is vital not to overuse them. Aim for clarity and maintainability in your code. Documenting your functions with optional parameters is essential, as it helps other developers understand the parameters' purposes and defaults.
Avoiding Ambiguity
A common pitfall when using optional parameters is creating ambiguity in function calls. For instance, if multiple parameters are optional but have similar default values, this may lead to confusion. Avoid such situations by being explicit and ensuring the default values chosen are distinct and clear. Consider this example, which highlights potential confusion:
void func(int x, int y = 0, int z = 10);
A call to `func(1)` could be interpreted in multiple ways. Resolving ambiguity often requires thoughtful parameter naming or altering the structure of the function.
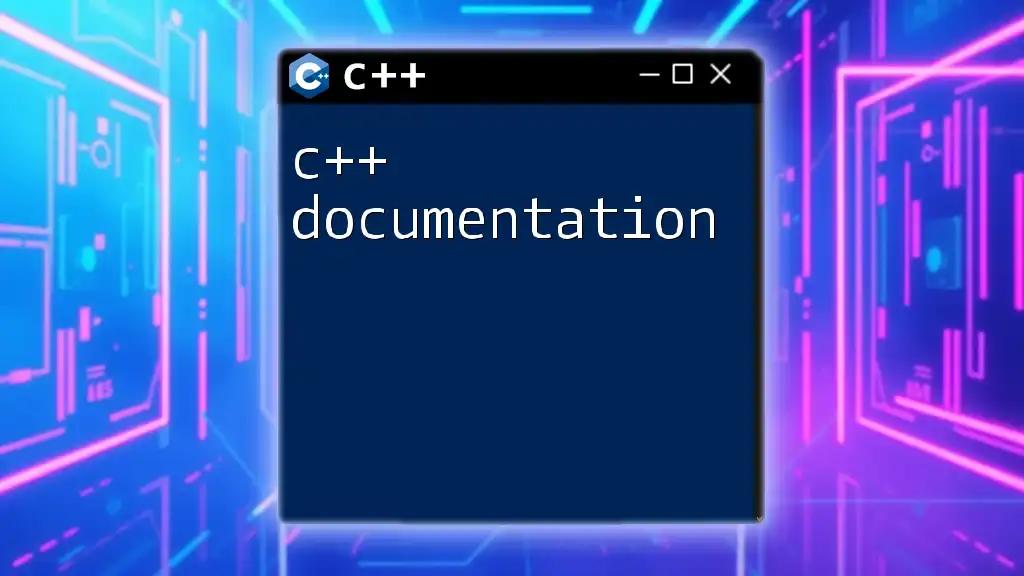
Conclusion
Optional arguments in C++ are a powerful feature that allows for greater flexibility and simplicity in function design. By leveraging optional parameters, developers can create cleaner, more readable code while maintaining functionality. Remember to balance the use of these parameters with best practices to ensure clarity and maintainability in your projects.
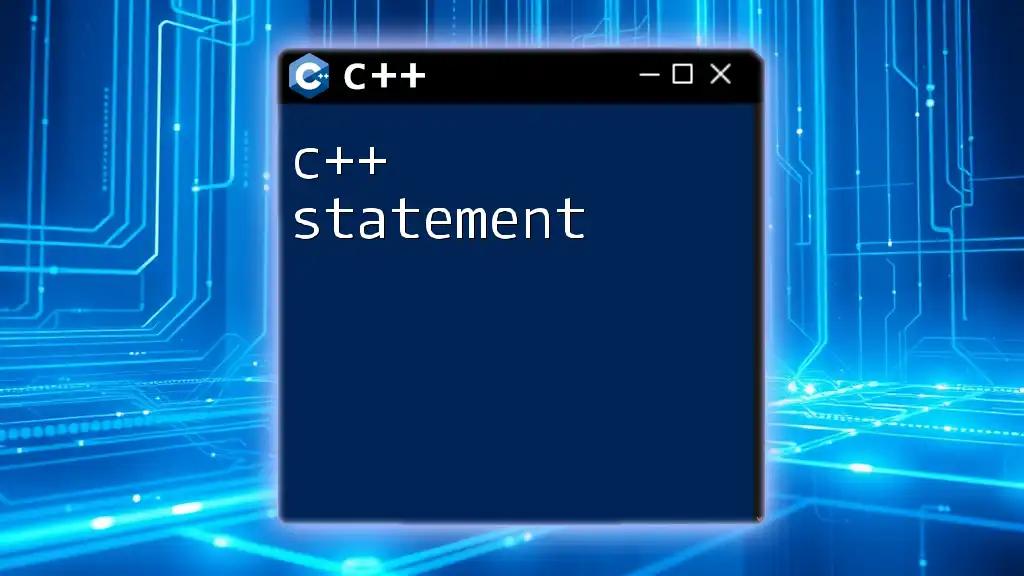
Additional Resources
For those looking to delve deeper into the topic of C++ optional parameters, numerous resources can provide further insight. books, websites, and online courses can offer more advanced knowledge and practical applications of optional parameters in C++. Expand your understanding and experiment with the capabilities afforded by this powerful aspect of the C++ language.