A C++ interpreter allows you to execute C++ code directly without the need for a separate compilation step, enabling quick testing and experimentation with C++ commands.
Here's a simple example of using an interpreter-like environment (such as C++ shell) to execute a basic "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Introduction to C++ Interpreters
What is an Interpreter?
An interpreter is a program that reads and executes code directly, translating high-level programming languages into machine code line-by-line. This contrasts sharply with compilers, which translate the entire source code into machine code before execution.
Why Use an Interpreter for C++?
Using a C++ interpreter affords several advantages, particularly for developers and learners. One of the most significant benefits is the speed with which one can test and debug code. You can write a command and see the result instantly, allowing for quick iteration and learning. This immediate feedback loop is crucial when you are starting to understand the nuances of the C++ language.
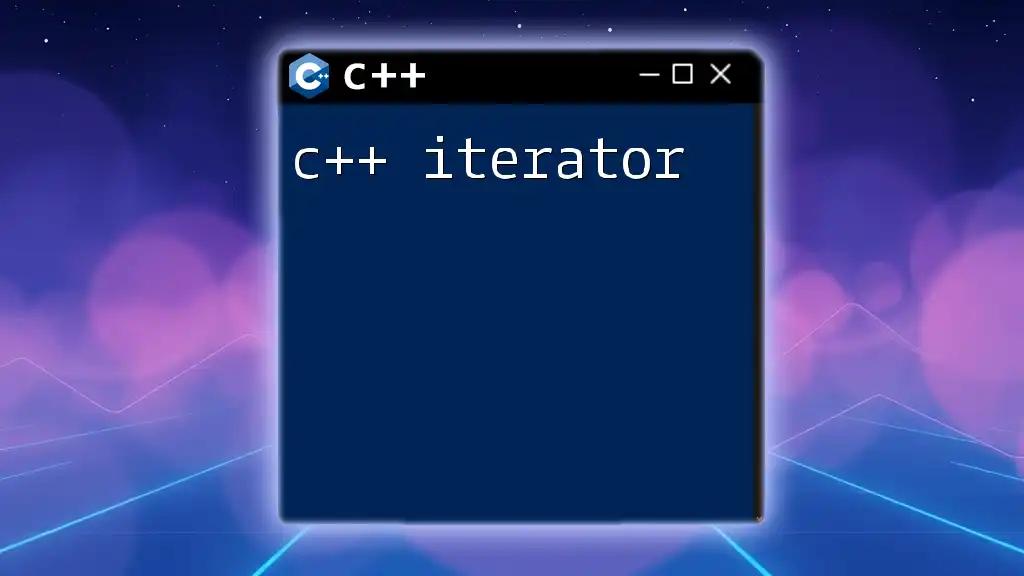
Understanding C++ Interpreters
How C++ Interpreters Work
C++ interpreters function by performing the translation of code during runtime rather than beforehand. This means that each line of code is executed as it is parsed. The flow can be illustrated in simplicity: the interpreter reads a line, compiles it, runs it, and then proceeds to the next line. For beginners, this model makes it easier to understand how one line of code can affect the execution of subsequent lines.
Types of C++ Interpreters
There are various types of C++ interpreters, each serving different needs.
Standalone Interpreters
Standalone interpreters are dedicated programs designed specifically to interpret C++ code without the need for an external Integrated Development Environment (IDE). Examples include CINT and Cling, both of which offer flexibility in experimenting with C++ code snippets without extensive setup.
Integrated Development Environment (IDE) Interpreters
IDE interpreters are built into development environments, providing additional tools like code completion and debugging. This integration enhances the user experience, particularly for beginners catching on to syntax and other nuances.
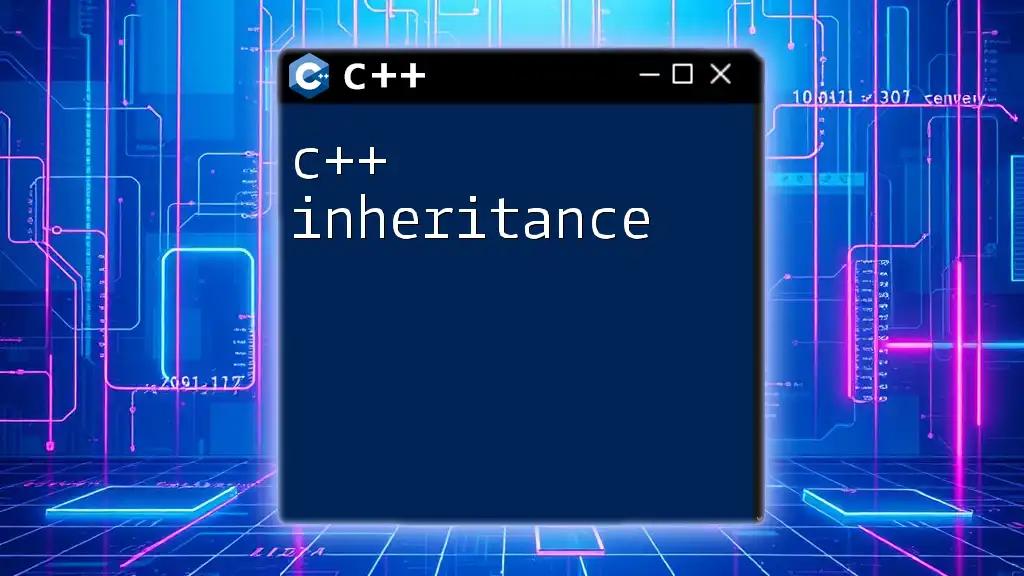
Getting Started with a C++ Interpreter
Setting Up Your Environment
Before you can start coding, you need to set up your environment. Here’s a straightforward approach:
- Choose an interpreter based on your needs (Cling is highly recommended for its features).
- Download the interpreter from its official website.
- Follow the installation instructions provided to set up your environment correctly.
Now that you are set up, let's discuss some basic commands and syntax.
Basic Commands and Syntax
C++ syntax can be intimidating at first, but the basics are relatively easy to grasp. Here’s a simple program to get started:
int main() {
std::cout << "Hello, C++ Interpreter!" << std::endl;
return 0;
}
In this code snippet, we declare the `main` function, which serves as the entry point for execution. The `std::cout` command outputs the string to the console, while `std::endl` ensures the cursor moves to the next line after the output, providing clear separation for multiple outputs.

Working with C++ Code in an Interpreter
Input and Output Handling
Being able to interact with users through input and output is fundamental in programming. Here’s how to take input and provide output using an interpreter:
#include <iostream>
int main() {
int num;
std::cout << "Enter a number: ";
std::cin >> num;
std::cout << "You entered: " << num << std::endl;
return 0;
}
In this code, we:
- Include the header `<iostream>` to utilize standard input and output.
- Declare an integer variable `num`.
- Prompt the user for input and read the value using `std::cin`.
- Finally, we print the value back, demonstrating a basic input-output cycle.
Data Types and Variable Declaration
Understanding data types is essential for effective programming. C++ includes basic data types such as `int`, `float`, `char`, and `bool`. Here’s a simple example demonstrating variable declaration:
float pi = 3.14;
std::cout << "Value of Pi: " << pi << std::endl;
In this snippet, we declare a floating-point variable `pi` and display its value. Variables in C++ must be declared with their respective types, which tells the compiler what kind of data they will hold.

Advanced Uses of C++ Interpreters
Using Libraries and Header Files
C++ allows us to include libraries and header files to extend functionality. For example, to use the Standard Template Library (STL), you might include the following:
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
In this example, we created a vector to hold integers and utilized a range-based for loop to print each element. Using libraries is crucial for modern C++ programming as they greatly expand what you can achieve.
Creating Functions and Structs
Modular programming is a pillar of efficient C++ code. Defining functions allows code reuse:
int add(int a, int b) {
return a + b;
}
std::cout << "Sum: " << add(5, 3) << std::endl;
Here, we define a function `add` that takes two integers as parameters and returns their sum. We then call this function and display the result. This modularity not only keeps the code cleaner but also makes debugging easier since you can isolate problems to specific functions.

Common Pitfalls and Troubleshooting
Debugging Common Errors in C++ Interpreters
As with any programming language, beginners may encounter various errors. Common errors include:
- Syntax Errors: Mistakes in the code structure.
- Runtime Errors: Errors that occur when the code is executed.
For example, leaving out a semicolon can lead to syntax errors, while dividing by zero may cause runtime errors. It’s essential to read error messages carefully and consult documentation for explanations.
Optimizing Performance
While interpreters excel in speed for testing code, understanding best practices can help optimize performance further. Avoid unnecessary calculations within tight loops, and prefer using built-in functions instead of creating your own unless necessary.
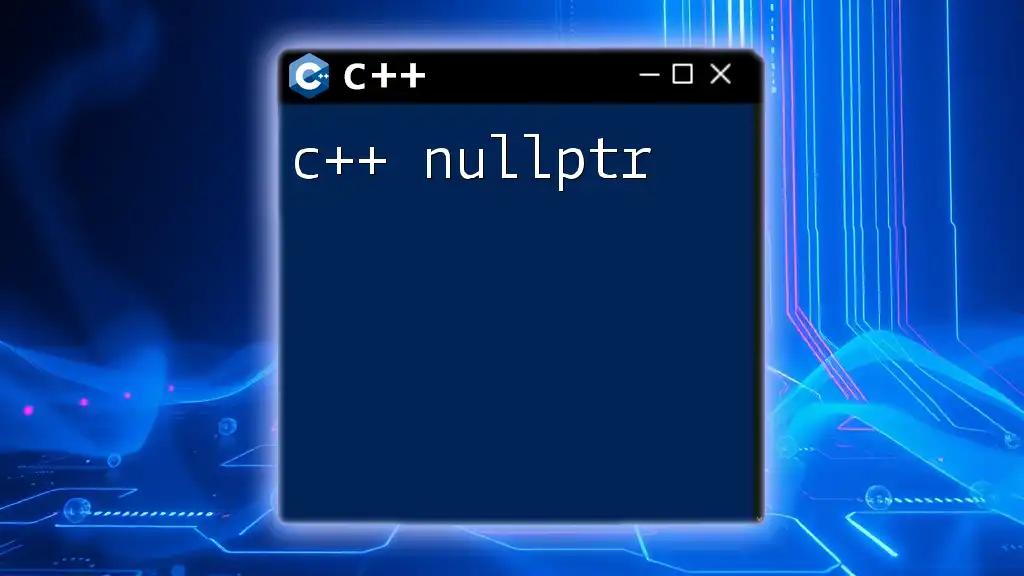
Conclusion
In this guide, we delved deeply into the concept of a C++ interpreter. We explored its function, benefits, and how to effectively execute C++ code with it. Mastering an interpreter will empower you in your C++ programming journey, allowing you to test, debug, and learn more efficiently.
As you continue your exploration of C++, remember that hands-on experimentation with interpreters can significantly enhance your understanding. Keep experimenting with code snippets, explore different interpreter features, and join communities to share your experiences. The future of C++ programming is bright, and mastering the use of interpreters is a vital skill for any programmer!
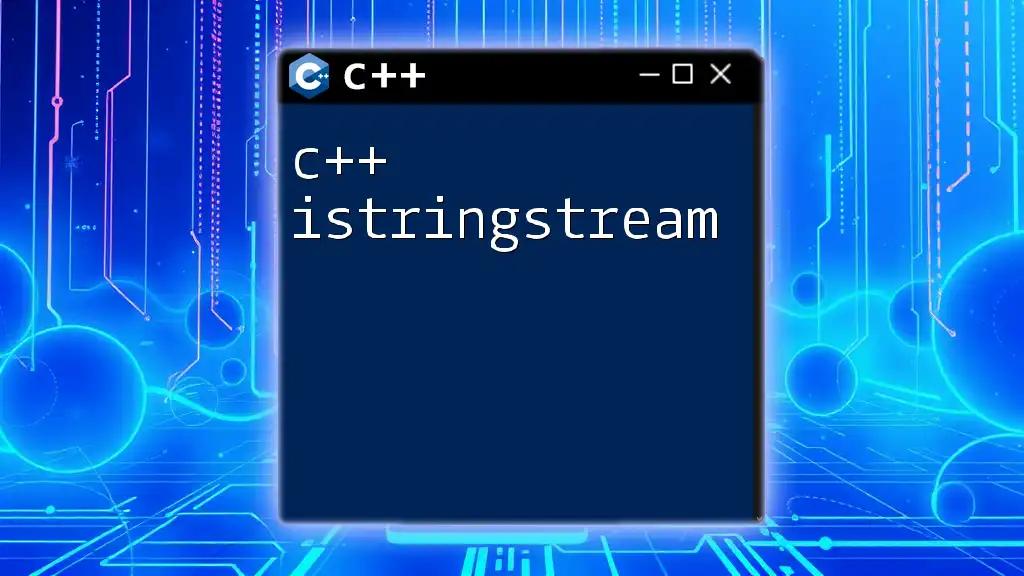
Additional Resources
If you’re eager to delve deeper into C++ and its interpreters, consider checking out the official C++ documentation, which frequently updates with new standards and functions. Additionally, various reputable tutorials and books can aid in your learning process, offering structured content and exercises to reinforce your skills. Happy coding!