C++ integer types are fundamental data types that represent whole numbers and can be categorized based on their size and whether they are signed or unsigned, influencing the range of values they can store.
Here's a code snippet demonstrating the declaration and initialization of different integer types in C++:
#include <iostream>
int main() {
int a = 5; // signed integer
unsigned int b = 10; // unsigned integer
short c = 100; // short signed integer
long d = 10000; // long signed integer
long long e = 100000; // long long signed integer
std::cout << "a: " << a << ", b: " << b << ", c: " << c << ", d: " << d << ", e: " << e << std::endl;
return 0;
}
Understanding C++ Integer Types
What are Integer Types?
In programming, integer types are data types that represent whole numbers without any fractional component. In C++, these types are crucial for various operations, such as mathematical calculations, indexing, and loop control. Integers can be either positive, negative, or zero, making them versatile for a wide range of applications.
Why Use Integer Types in C++?
Using integer types in C++ has significant advantages. Efficiency is a primary reason; integers typically consume less memory and require less processing power compared to floating-point numbers. This makes them particularly suitable for performance-critical applications, such as game development or real-time systems, where every bit of performance counts. Moreover, integers play a vital role in systems programming, algorithms, and data structures, enabling precise control over data representation and manipulation.
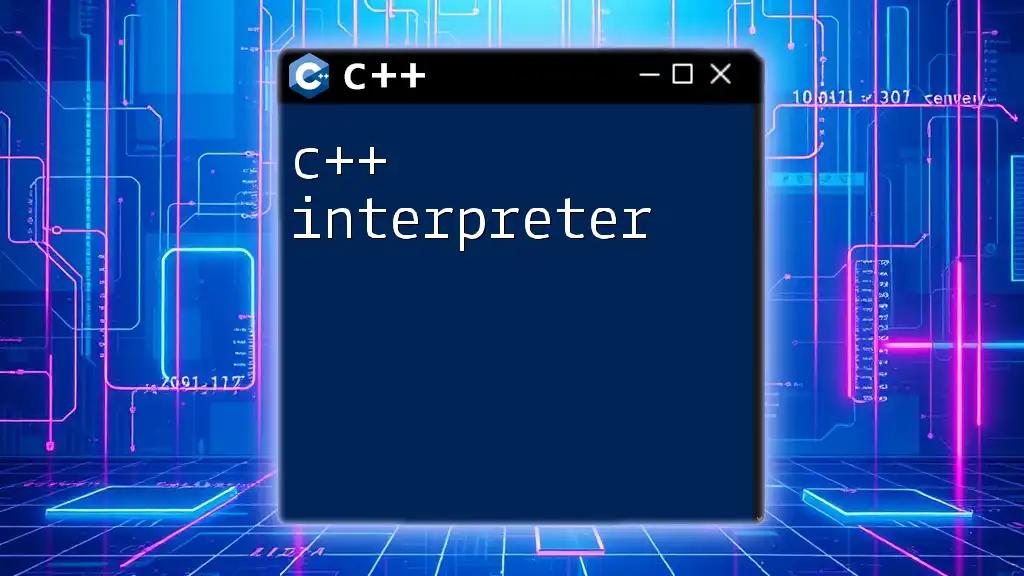
C++ Integer Data Types Overview
Built-in Integer Types
C++ provides several built-in integer types that cater to various needs:
- `int`: The most commonly used type, representing integers. Its size can vary, but it typically uses 32 bits.
- `short`: A smaller integer type that usually uses 16 bits. It is useful when memory conservation is essential.
- `long`: This type typically uses 32 or 64 bits, making it effective for storing larger integers.
- `long long`: Designed for even larger integer values, this type typically uses 64 bits.
Understanding these types is vital for choosing the right one based on the needs of your application.
Size and Range of Integer Types
Each integer type has a specific size and range of values it can represent, which you can retrieve using the `<limits>` header in C++.
For example:
#include <iostream>
#include <limits>
int main() {
std::cout << "int: " << sizeof(int) << " bytes, range: "
<< std::numeric_limits<int>::min() << " to "
<< std::numeric_limits<int>::max() << std::endl;
return 0;
}
This snippet demonstrates how to find the size in bytes as well as the minimum and maximum values for the `int` type. Each integer type has its limits, which influence how you choose and use them in your applications.
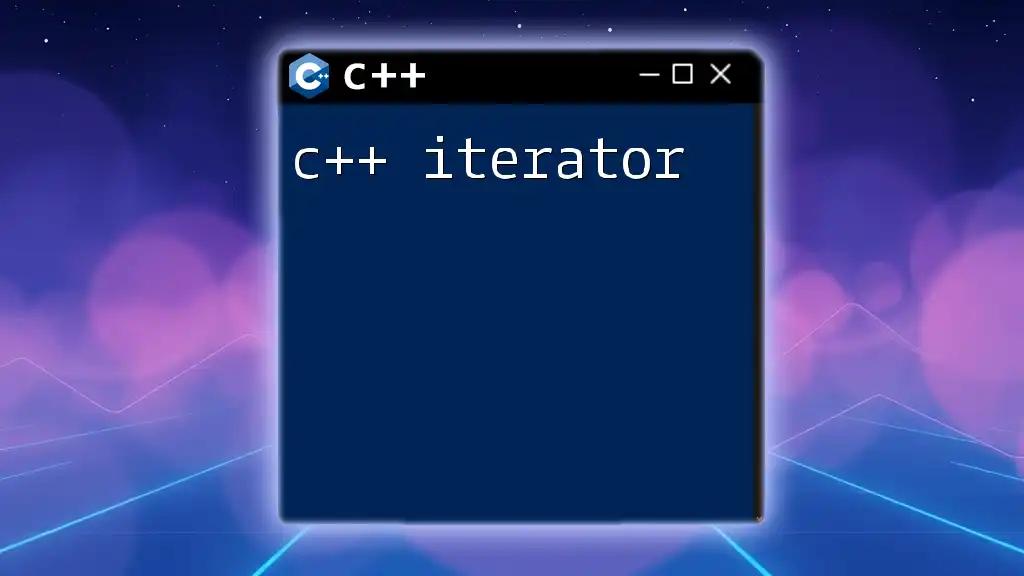
Signed vs Unsigned Integers
Differences Between Signed and Unsigned Integers
In C++, integers can be signed or unsigned.
- Signed integers can represent both positive and negative numbers. For example, a signed `int` can store values ranging from -2,147,483,648 to 2,147,483,647.
- Unsigned integers, on the other hand, can only represent non-negative values, effectively doubling their upper limit. An unsigned `int` ranges from 0 to 4,294,967,295.
Consider the following code snippet:
int signedInt = -10;
unsigned int unsignedInt = 10;
std::cout << signedInt << " is a signed integer." << std::endl;
std::cout << unsignedInt << " is an unsigned integer." << std::endl;
This example illustrates the use of each integer type and highlights their fundamental differences.
When to Use Signed or Unsigned Integers
Choosing between signed and unsigned integers depends on your requirements. If you know your data can only be zero or positive, unsigned types are beneficial as they allow manipulation of a larger range of positive values. However, using unsigned integers can lead to issues when combined with signed integers, sometimes causing unexpected behavior, particularly under conditions of overflow or comparisons.
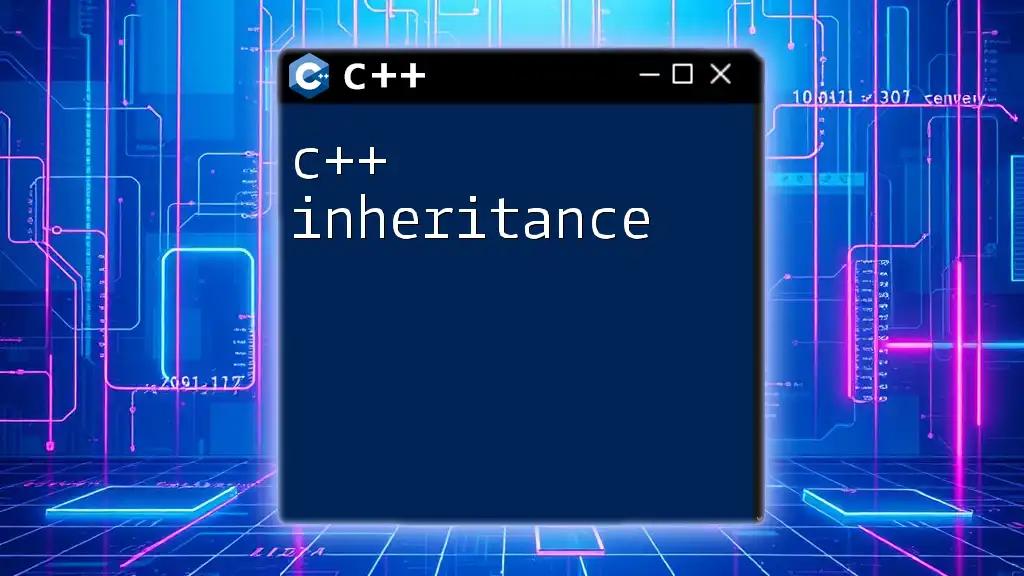
Integer Literals in C++
How to Define Integer Literals
In C++, integer literals can be defined in various numeral systems: decimal, hexadecimal, octal, and binary. This flexibility allows developers to write more readable and meaningful numeric expressions.
For example:
int dec = 42; // Decimal
int hex = 0x2A; // Hexadecimal
int oct = 052; // Octal
int bin = 0b101010; // Binary (C++14 and later)
std::cout << dec << " (decimal), " << hex << " (hex), "
<< oct << " (octal), " << bin << " (binary)" << std::endl;
In this snippet, you can see how different types of literals can be declared, each serving a specific purpose depending on the context.
Specifying Integer Types with Suffixes
C++ lets you specify the type of an integer literal using specific suffixes:
- `u` denotes an unsigned value.
- `l` denotes a long integer.
- `ll` for long long integers.
Here’s how you would declare these:
unsigned long uLong = 3000UL; // 'UL' for unsigned long
long long llValue = 1000000LL; // 'LL' for long long
Using suffixes allows for precise control over the types and helps prevent unintended type conversions.

Type Conversion and Casting
Implicit Type Conversion
C++ automatically converts smaller integer types to larger ones when necessary. For instance, assigning a `short` value to an `int` type is safe and performed without any explicit action. However, this can sometimes lead to loss of data or unexpected results if not handled cautiously.
Explicit Type Conversion (Casting)
When you want to convert types intentionally, you can use casting to ensure correctness. For example, you can use `static_cast`, which is the safest form of cast for most use cases:
int a = 10;
double b = static_cast<double>(a);
In this example, `a` is explicitly converted to a `double`, ensuring that you retain precision in calculations afterward.
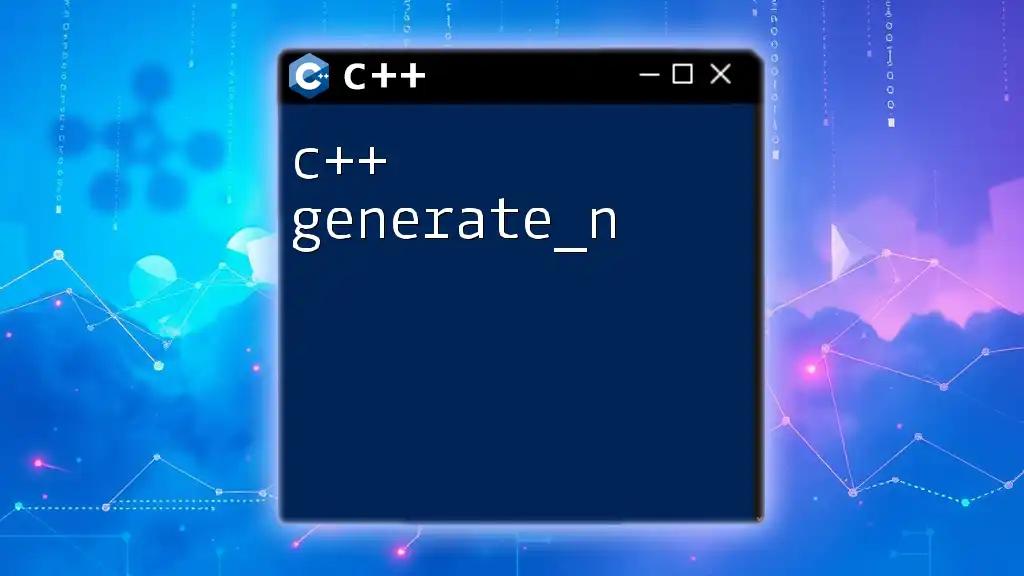
Integer Operations
Common Integer Operations
C++ supports a straightforward set of operations for integer types, including addition, subtraction, multiplication, division, and modulus. These operations are fundamental to many algorithms and applications.
Here’s a simple example demonstrating these operations:
int a = 20, b = 3;
std::cout << "Sum: " << a + b << ", Difference: " << a - b
<< ", Product: " << a * b << ", Quotient: " << a / b
<< ", Modulus: " << a % b << std::endl;
This snippet performs basic calculations and presents the results, which are foundational to manipulating integer types in C++.
Overflow and Underflow Handling
It’s essential to be aware of potential overflow and underflow conditions when performing operations with integers. Overflow occurs when a calculation exceeds the maximum range of an integer type, while underflow happens when it drops below the minimum range.
For instance, adding two large signed integers might push the result beyond their limit, wrapping it around to a negative value. Proper checks or exception handling can mitigate these risks.

Best Practices with Integer Types
Choosing the Right Integer Type
Selecting the most appropriate integer type depends on factors like your application’s needs, memory constraints, and performance analysis. For small values, consider using `short` or `unsigned short`, while `long long` is better for significantly large integers that exceed the standard range.
Common Mistakes to Avoid
One common mistake is implicitly mixing signed and unsigned integers in expressions. This can yield unexpected results, as the signed integer may convert to an unsigned type, leading to erroneous values or logical errors. Always ensure that you maintain type consistency in your expressions.

Conclusion
Recap of C++ Integer Types
C++ integer types offer a variety of options tailored to manage whole numbers effectively. Choosing appropriate types based on size, range, and signedness is crucial in ensuring efficient and error-free programs.
Final Thoughts
Understanding and effectively utilizing C++ integer types is foundational to programming in this language. Experimenting with these different types and operations will enhance your skills and confidence in tackling more complex programming challenges. For further exploration, consider researching advanced topics related to integer types or experimenting with code snippets in your projects.