In C++, the `string` data type is part of the Standard Library and is used to represent sequences of characters, allowing for dynamic string manipulation.
Here's a simple example of how to use the `string` data type in C++:
#include <iostream>
#include <string>
int main() {
std::string greeting = "Hello, World!";
std::cout << greeting << std::endl;
return 0;
}
Understanding the C++ String Data Type
The C++ string data type is a fundamental part of the language that allows developers to manipulate and handle sequences of characters easily. Unlike traditional character arrays (char arrays), the `std::string` class provides a more powerful and flexible interface for working with strings. It abstracts many complexities associated with memory management and includes a rich set of functionalities, making it easier to perform common tasks related to text processing.
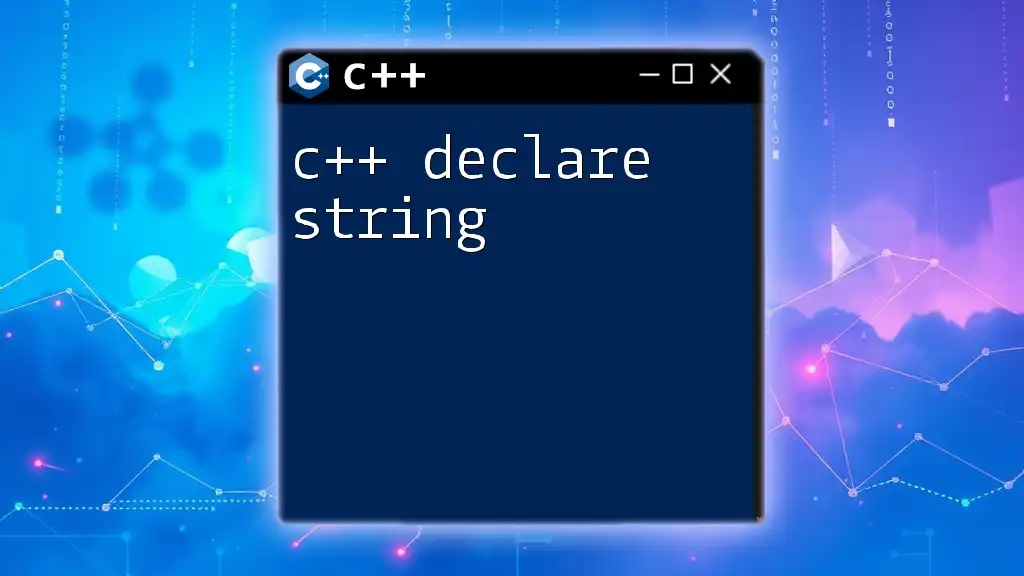
Creating and Initializing C++ Strings
Declaration and Initialization of Strings
In C++, you can declare a string using the `std::string` type. This can be done in various ways:
std::string greeting = "Hello, World!";
std::string name("Alice");
std::string emptyString; // Default initialization
Each of these methods initializes a string variable with specific content or leaves it empty. The ability to initialize strings flexibly is one of the advantages of using the C++ string data type over basic char arrays.
String Assignment
To assign new values to strings, you can use both copy and move assignment techniques. Here are a few examples:
std::string str1 = "Hi";
std::string str2;
str2 = str1; // Copy assignment
str2 = std::move(str1); // Move assignment
Copy assignment creates a new copy, while move assignment transfers the contents of `str1` to `str2`, leaving `str1` in a valid but unspecified state.
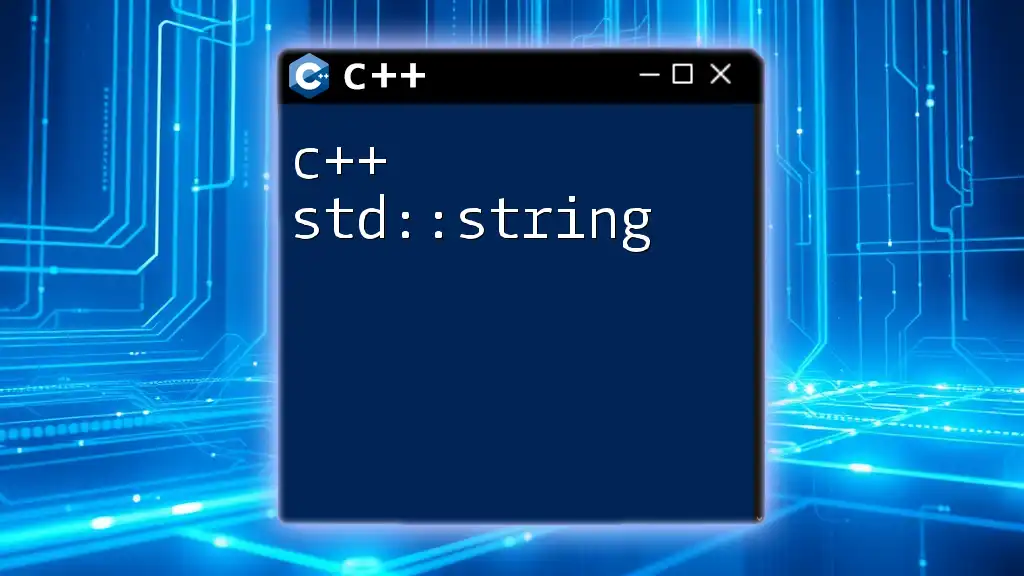
Common String Operations in C++
Concatenation of Strings
Combining strings is a common operation, easily performed with the `+` operator:
std::string result = "Hello" + std::string(" World");
This statement concatenates the two strings together, allowing you to build complex strings without hassle.
String Length
You can find the length of a string using either the `.length()` or `.size()` member functions. Both yield the same result:
std::string text = "Hello";
std::cout << "Length: " << text.length(); // Outputs: Length: 5
Understanding the length of a string is essential for various tasks, including validation and iteration.
Accessing Characters in Strings
Accessing individual characters in a string can be done using `[]` or `.at()`. The advantage of `.at()` is that it performs bounds checking, which is crucial for preventing runtime errors:
char firstChar = text[0]; // Accessing 'H'
char secondChar = text.at(1); // Accessing 'e'
Using `.at()` is generally safer but slightly slower due to the additional safety checks.
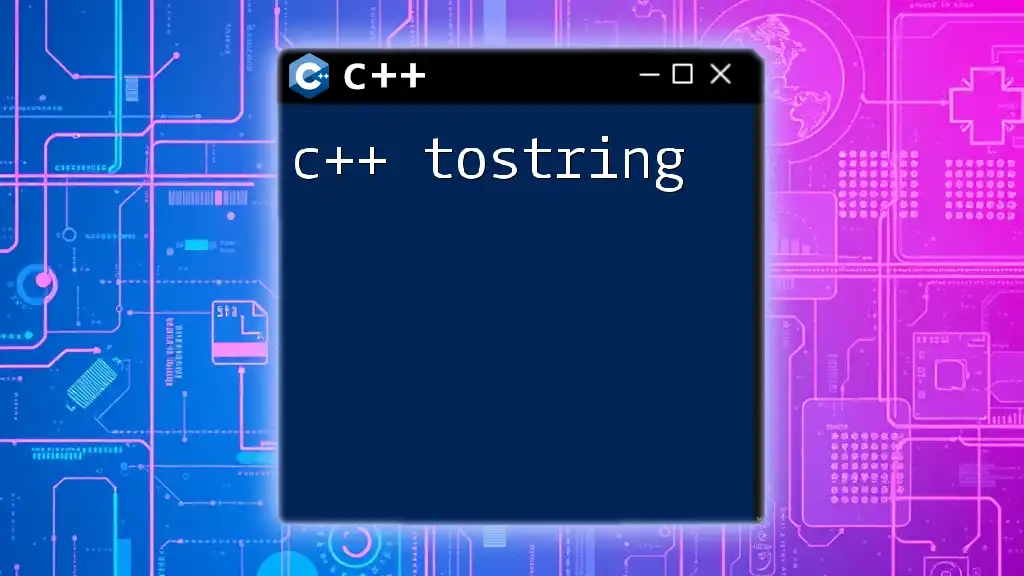
Modifying C++ Strings
Inserting and Appending Strings
Strings can be dynamically modified using the `append()` or `insert()` methods:
std::string str = "abc";
str.append("def"); // str becomes "abcdef"
str.insert(0, "XYZ"); // str becomes "XYZabcdef"
These methods allow you to seamlessly enhance string content at any point.
Removing Characters from Strings
To remove characters, `erase()` can be beneficial:
str.erase(0, 3); // str becomes "abcdef" after removing "XYZ"
This command effectively allows you to specify which part of the string to remove.
Replacing Substrings
You can also manipulate strings by replacing parts of them with the `replace()` method:
str.replace(1, 3, "XYZ"); // Replaces "cde" with "XYZ"
Being able to replace substrings effectively enhances your string manipulation capabilities.
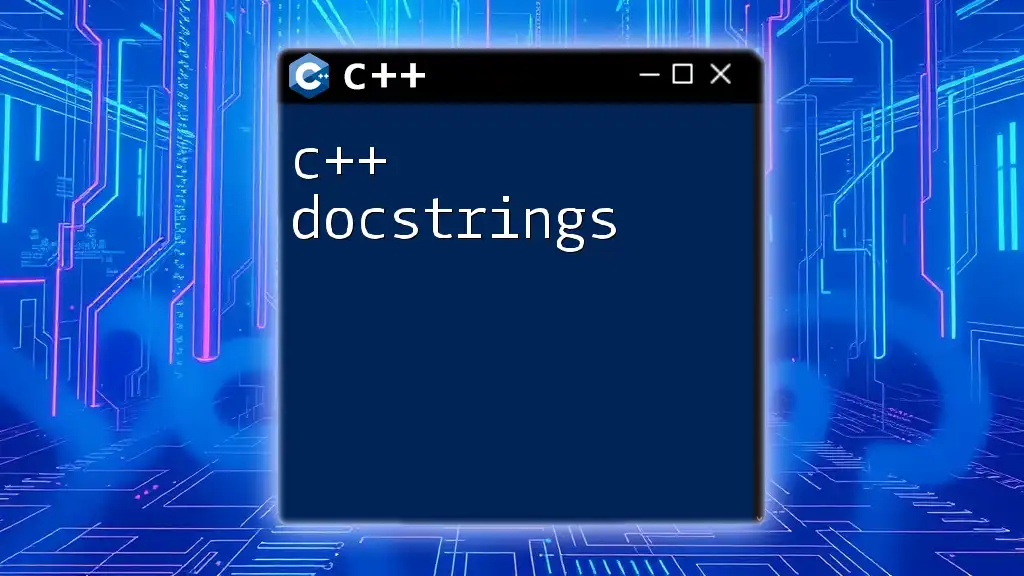
Comparing Strings
C++ provides built-in comparison operators for strings, making it easy to determine the order of two strings:
std::string str1 = "apple";
std::string str2 = "banana";
if (str1 < str2) {
std::cout << str1 << " is less than " << str2;
}
These relational comparisons compare strings lexicographically, similar to how words are ordered in a dictionary.
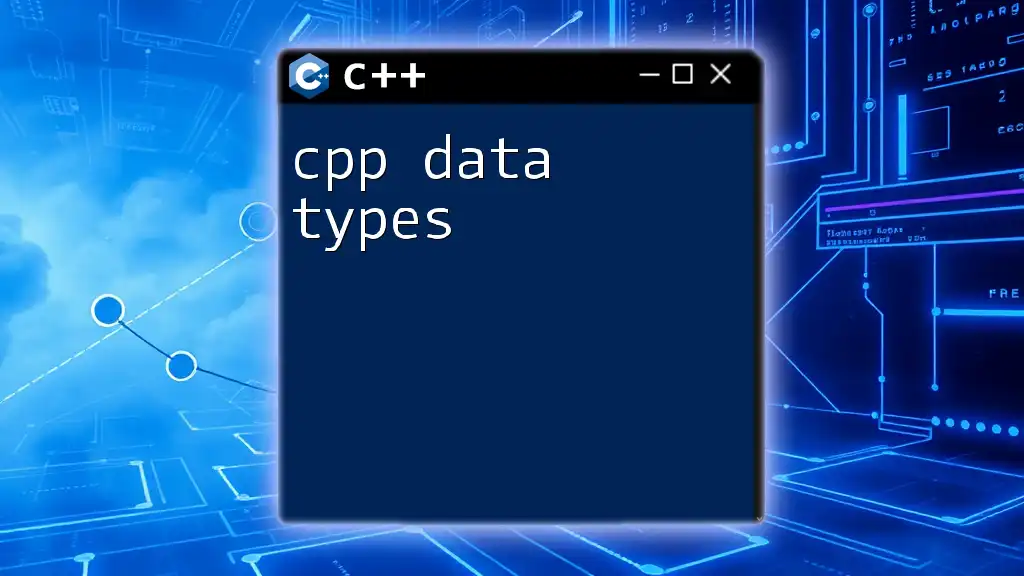
String Conversion in C++
Converting Strings to Other Data Types
When you need to convert strings to numeric types, C++ provides helper functions like `std::stoi()`, `std::stof()`, etc. Here’s a simple example:
std::string numericString = "12345";
int number = std::stoi(numericString);
Such conversions are handy when reading numerical data as strings that need to be processed as integers or floating-point numbers.
Converting Other Data Types to Strings
Conversely, for converting other data types to strings, you can utilize `std::to_string()`:
int num = 100;
std::string numStr = std::to_string(num); // Converts int to string
This function is particularly useful when preparing data for output or concatenation.
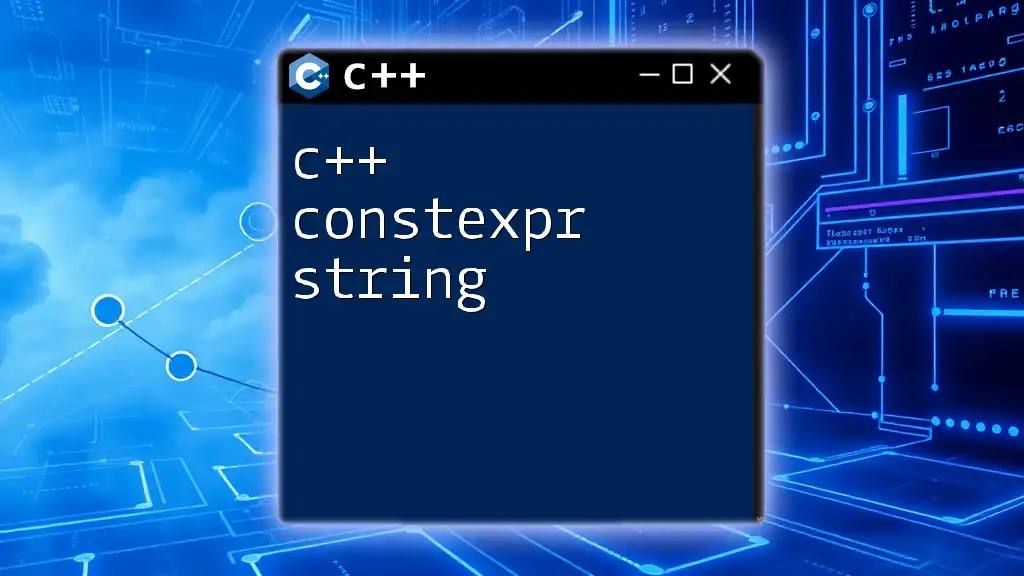
Advanced Topics in C++ Strings
String Literals and Raw String Literals
C++ enables the use of string literals along with raw string literals for better readability. A raw string literal allows you to include special characters without escaping:
std::string rawString = R"(This is a raw string)";
This approach is beneficial when you need to include backslashes or quotes without the overhead of escaping them.
String View and Performance
For performance-focused applications, consider `std::string_view`, introduced in C++17. This type provides a lightweight view into a string without owning the underlying memory, which can reduce copy overhead in multiple scenarios where the string doesn’t need modification. It is essential to understand when to use `string_view` to optimize performance in applications that require frequent read access without modification.
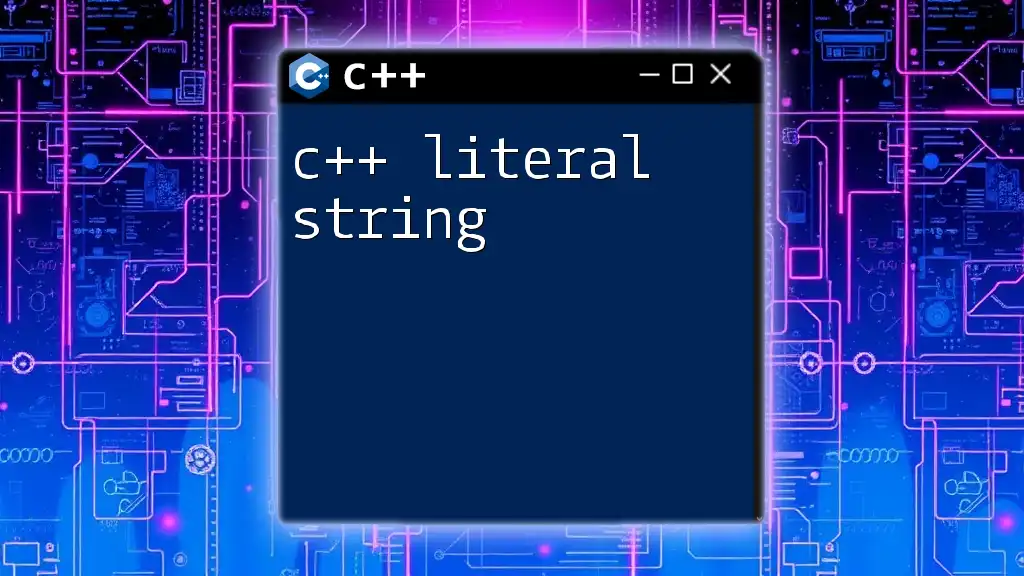
Conclusion
In summary, understanding C++ data types string is essential for anyone looking to master the language. By using `std::string`, developers can easily handle textual data without the complications often associated with lower-level character arrays. With its robust functionality and flexibility, the string data type in C++ is indispensable for handling text operations efficiently.
Continuously practicing string operations will enhance your ability to manipulate text in C++, paving the way for more complex programming tasks. For those eager to learn more, many resources are available to deepen your knowledge of C++ strings and their extensive capabilities.