C++ data types define the type of data a variable can hold, with common categories including integer, floating-point, character, and boolean types.
Here's a quick example demonstrating different C++ data types:
#include <iostream>
int main() {
int age = 25; // Integer type
float height = 5.9; // Floating-point type
char initial = 'J'; // Character type
bool isStudent = true; // Boolean type
std::cout << "Age: " << age << ", Height: " << height << ", Initial: " << initial << ", Is Student: " << isStudent << std::endl;
return 0;
}
Understanding Data Types in C++
What Are Data Types?
Data types are fundamental concepts in programming languages, including C++, that define the type of data a variable can hold. This includes integers, floating-point numbers, characters, and more. Choosing the right data type is crucial because it determines how much memory the data will occupy and how the data can be manipulated. In essence, data types help the compiler understand what operations can be performed on the data and ensure data integrity.
Importance of Using the Right Data Types
Using appropriate data types impacts both the efficiency and readability of code. For instance, using a `float` type for a variable that only needs integer values not only wastes memory but can also lead to unnecessary complications in logic. Conversely, using an `int` instead of a `long long` for large numbers can result in overflow errors. Hence, making informed decisions about data types enhances code clarity and reduces errors.
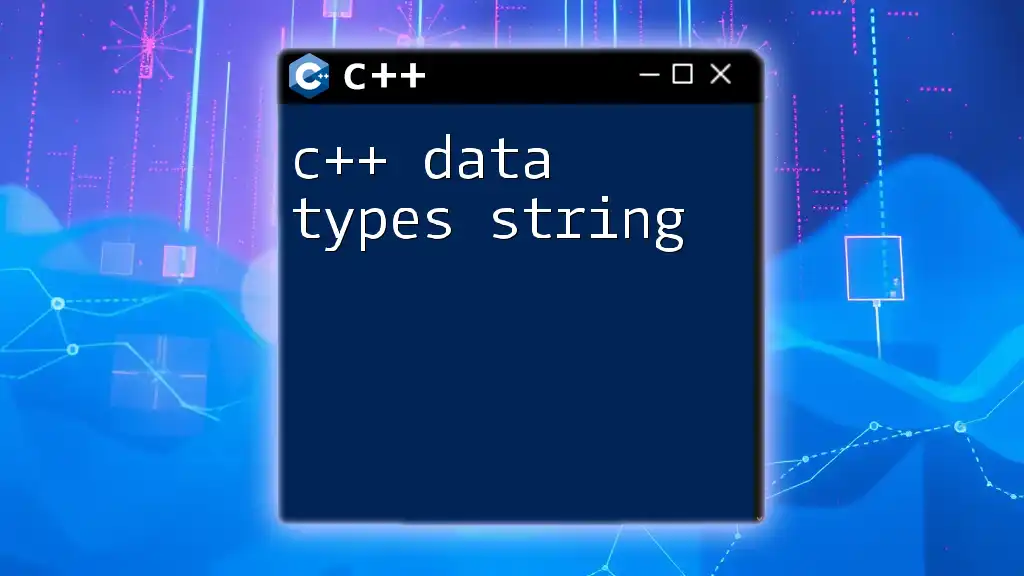
Overview of C++ Data Types
Built-in Data Types in C++
C++ provides a rich set of built-in data types that fall under two primary categories: fundamental and derived data types.
- Fundamental data types include integers, floating points, and characters.
- Derived data types include arrays, pointers, references, and functions.
Explicit vs. Implicit Data Types
In C++, the data type of a variable can be explicitly defined or inferred implicitly by the compiler. Explicit typing ensures clarity and leaves no room for confusion. Implicit typing, often used with `auto`, allows for flexibility but can reduce readability.
Examples:
int x = 10; // Explicit typing
auto y = 10.5; // Implicit typing (float)
By understanding when to apply explicit or implicit typing, programmers can write cleaner and more maintainable code.
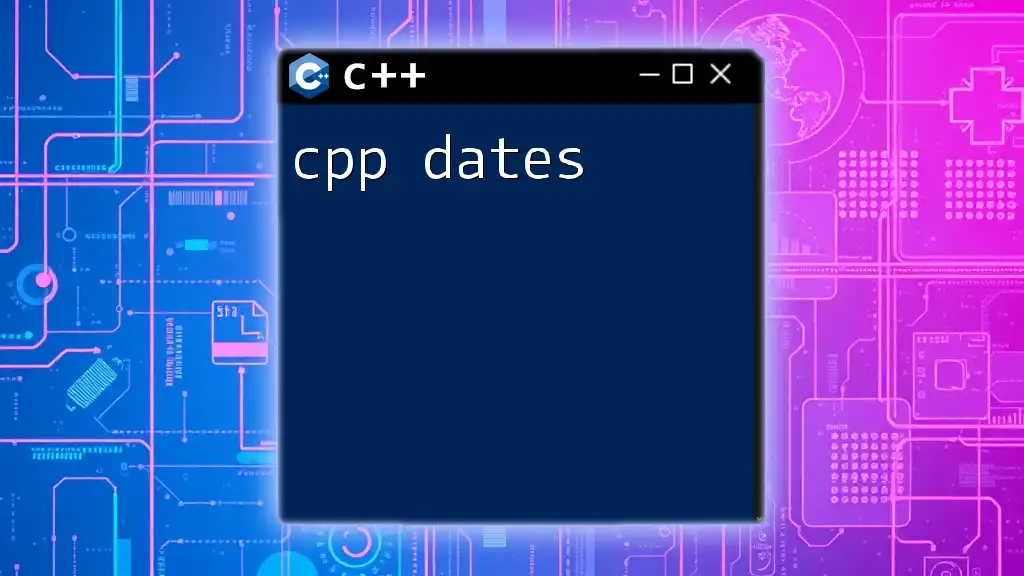
Fundamental Data Types in C++
Integer Types: `int`, `short`, `long`, and `long long`
Integers are basic numeric types that represent whole numbers. In C++, various integer types exist, each optimized for different ranges of values:
- `int`: The most commonly used integer type. Typically, it occupies 4 bytes.
- `short`: Uses less memory (2 bytes), holding values from -32,768 to 32,767.
- `long`: Typically occupies 4 bytes or 8 bytes, depending on the architecture. Use it for larger values.
- `long long`: Ensures at least 8 bytes of storage. Ideal for very large integers.
Example:
int a = 10;
short b = 5;
long c = 100000;
long long d = 10000000000;
Use cases: When to opt for each integer type is largely based on the expected range of values, with `short` and `long` being preferable for memory-efficient applications.
Floating-Point Types: `float`, `double`, and `long double`
Floating-point types are used for decimal values. They differ mainly in precision and memory size:
- `float`: Typically 4 bytes, it can handle 6-7 decimal digits.
- `double`: At 8 bytes, it holds 15-16 decimal digits, thus being the preferred choice for most calculations.
- `long double`: Offers even more precision, usually around 10 bytes.
Example:
float e = 5.5f;
double f = 15.99;
long double g = 20.123456789;
Choosing the appropriate floating-point type is essential when precision is critical; for most applications, `double` suffices.
Character Type: `char`
The `char` data type is utilized to represent single characters and occupies 1 byte of memory.
Example:
char h = 'A';
Use cases: While individual characters are stored as `char`, strings (a sequence of characters) are often represented using arrays or the C++ `string` class for better manipulation and readability.
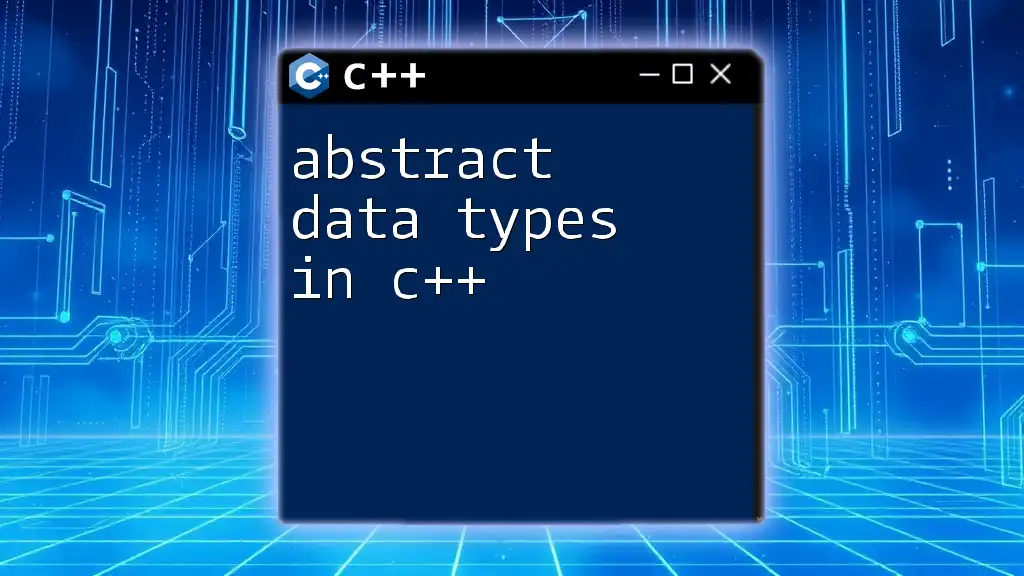
User-Defined Data Types in C++
Structures: `struct`
Structures (or `structs`) are user-defined data types that group related data under one name. This encapsulation of data makes code cleaner and more understandable.
Example:
struct Person {
string name;
int age;
};
Benefits of using structures: They enhance code organization, especially when managing more complex data relations, such as a database of people or cars.
Classes: `class`
Classes are a cornerstone of object-oriented programming in C++. They encapsulate data and functions that operate on that data, promoting the principles of encapsulation, inheritance, and polymorphism.
Example:
class Car {
public:
string model;
int year;
};
Encapsulation in classes: Classes allow you to combine both data and behavior, providing a powerful framework for building applications.
Unions: `union`
A union is another user-defined data type, allowing different data types to occupy the same memory location. This is particularly useful for memory-constrained applications.
Example:
union Data {
int intValue;
float floatValue;
};
Use cases for unions: They are perfect for scenarios where multiple types are needed but only one is accessed at a time, enhancing memory efficiency.
Enums: `enum`
Enums define a set of named integral constants, improving code clarity by giving descriptive names to variables.
Example:
enum Colors { RED, GREEN, BLUE };
When to use enums: They simplify code logic and improve maintainability, especially when dealing with a fixed set of related constants.
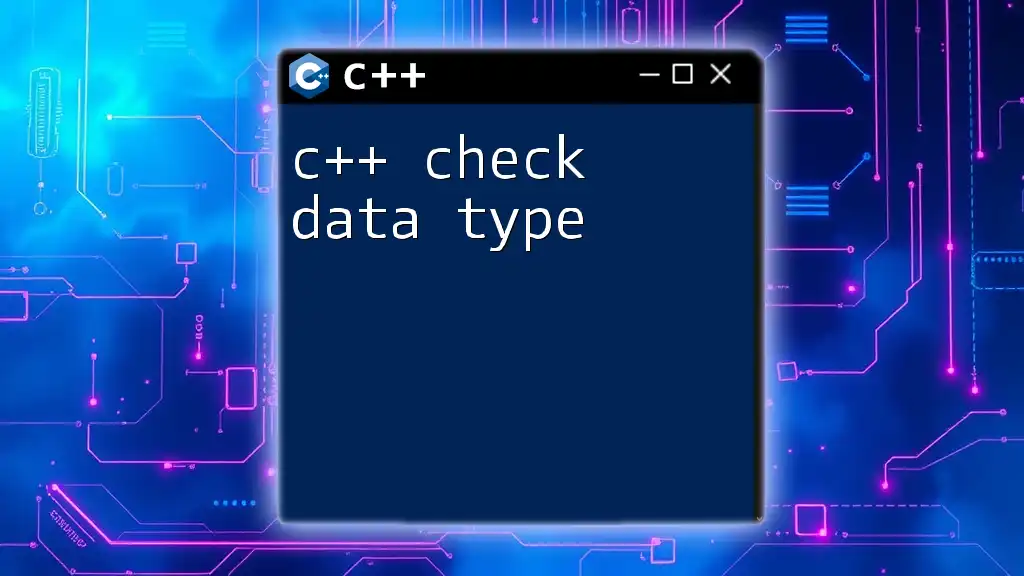
Modifiers and Type Qualifiers
Type Modifiers: `signed`, `unsigned`, `short`, and `long`
Type modifiers allow fine-tuning of the basic data types, affecting their range and characteristics.
Example:
unsigned int x = 10; // No negative numbers
signed char y = -5; // Can hold both positive and negative
Choosing the right modifier: Knowing whether to use signed or unsigned types can prevent overflow and enhance data integrity.
Type Qualifiers: `const` and `volatile`
Type qualifiers like `const` and `volatile` alter the behavior of data types. A `const` variable cannot be modified after initialization, while `volatile` indicates that a variable can change at any time outside the scope of the program.
Example:
const int z = 20; // Unmodifiable variable
volatile int v = 30; // Value can change unexpectedly
Implications of `const` and `volatile`: They are crucial in multithreading environments, ensuring that a program handles shared resources correctly.
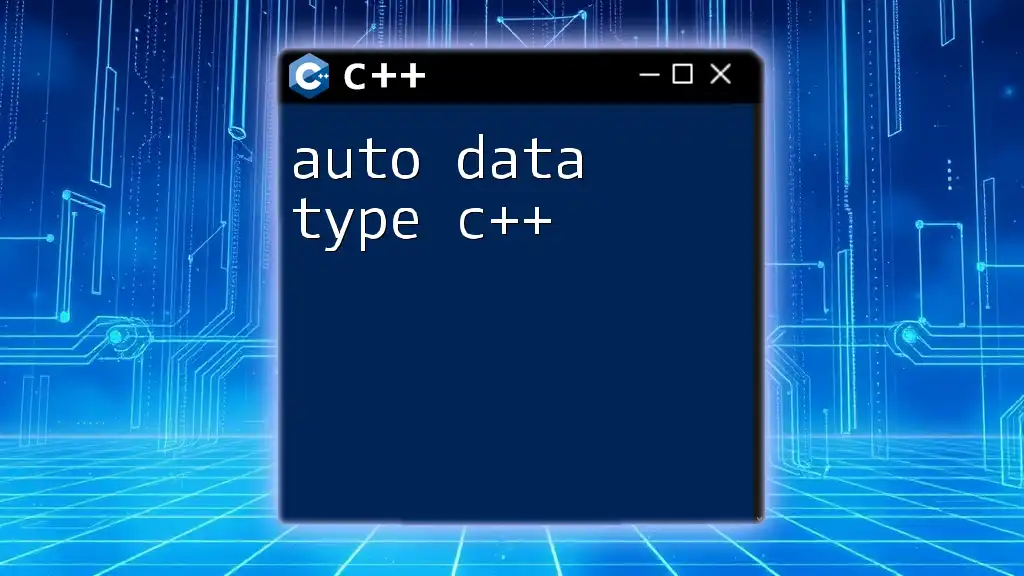
Special Data Types
The `void` Type
The `void` type signifies that a function does not return any value. This is often used for functions that perform actions but don’t produce a result.
Example:
void displayMessage() {
cout << "Hello World";
}
This allows for cleaner syntax in functions that serve a purpose beyond returning data.
The `nullptr` Type
In modern C++, `nullptr` replaces the traditional `NULL`, providing a type-safe null pointer constant.
Example:
int* ptr = nullptr;
Comparison with NULL: Using `nullptr` improves code safety and clarity, distinguishing between null pointers and integers.
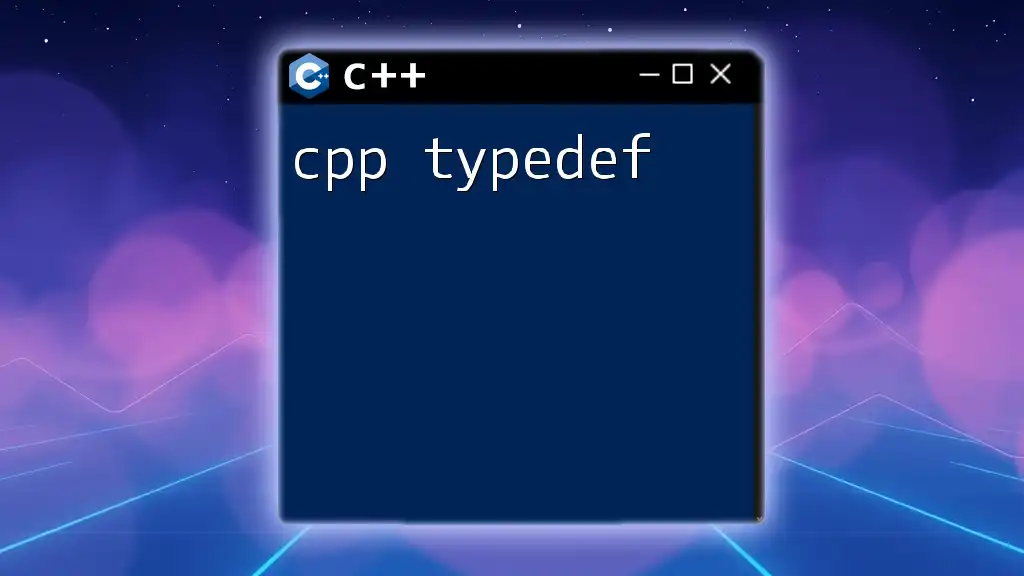
Conclusion
Understanding cpp data types is vital for efficient programming in C++. Choosing the right type enhances performance, memory usage, and code readability. As you delve deeper into C++, keep practicing these concepts, implementing them in various contexts to strengthen your programming skills. With these fundamental principles in mind, you can navigate the world of C++ data types with confidence.
Additional Resources
For further learning, consider exploring various tutorials, documentation, and community resources that cover advanced topics in C++ data types and programming best practices.