In C++, the `enum` data type is used to define a variable that can hold a set of predefined constant values, enhancing code readability and maintainability.
enum Color { Red, Green, Blue };
Color favoriteColor = Green;
What is Enumeration in C++?
Enumeration, often referred to as enum, is a user-defined data type in C++ that allows you to assign names to integral values. This process improves code readability, making it easier for developers to understand what each value represents. Enums provide a way to define a variable that can hold a set of predefined constants, which can make your code more intuitive and manageable.
The importance of the enumerated type comes from its ability to represent related constants together, thus encapsulating them into a single easily recognizable type. This organization allows developers to work with meaningful names rather than arbitrary values, enhancing overall code quality.

Benefits of Using Enum Data Types
Using enum data types comes with a multitude of benefits:
-
Improved Code Readability: By replacing numeric constant values with meaningful names, the intent behind the code becomes much clearer. For instance, rather than using the number `1` to represent `Red`, you can simply use `Color::Red`, making it evident what the code represents at a glance.
-
Enhanced Type Safety: Enumerations introduce a type-safe way to handle constants. Instead of using integers that might be misinterpreted, enums restrict values to those defined in the enumeration, avoiding potential bugs due to invalid assignments.
-
Simplified Code Maintenance: Changes to the enumerated values can be made in one place. Should you need to add another color, simply update the enum declaration without worrying about every instance where that constant appears in your code.

Syntax of Enum Data Types
Defining an enum in C++ is straightforward. The basic syntax for declaring an enumeration type is as follows:
enum EnumName { Constant1, Constant2, Constant3 };
Example of a Simple Enumeration
Here is an example that defines an enumeration for colors:
enum Color { Red, Green, Blue };
In this example, `Red`, `Green`, and `Blue` are the enumerators. By default, the enumeration values are assigned integer values starting from `0`, so `Red` becomes `0`, `Green` becomes `1`, and `Blue` becomes `2`.
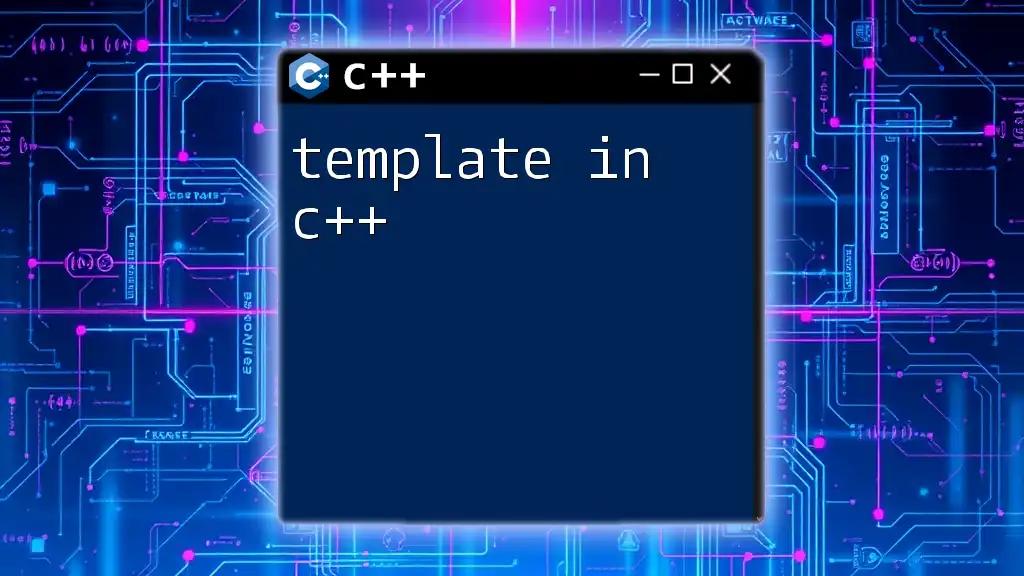
Types of Enumeration in C++
Unscoped Enumerations
Unscoped enumerations are the traditional way to define enumerated types in C++. They are defined without the `class` keyword and their enumerators can be accessed directly. This is beneficial as it does not require any extra scope resolution.
Example of Unscoped Enumeration
enum Animal { Dog, Cat, Bird };
Scoped Enumerations (C++11 and Later)
Scoped enumerations, introduced in C++11, offer better type safety than unscoped enums. To define a scoped enumeration, you use the `enum class` syntax.
Difference between Scoped and Unscoped Enumerations
Scoped enumerations prevent enumerators from conflicting with other identifiers within the same scope. You access their enumerators using the scope resolution operator.
Example of Scoped Enumeration
enum class Direction { North, South, East, West };
To access these enumerators, the syntax would be `Direction::North`, ensuring that the usage is clear and unambiguous.

Assigning Values to Enumerators
By default, enumerators are assigned values starting from `0` and incremented by `1`. However, you can also manually set values for enumerators.
Custom Values in Enumerations
Here’s how you can assign specific integer values:
enum Fruit { Apple = 1, Banana = 2, Cherry = 3 };
In this case, `Apple` is assigned `1`, `Banana` `2`, and `Cherry` `3`, allowing you to set the values explicitly to whatever you need.

Using Enumeration in C++
Using Enum in Switch Statements
Enums are particularly useful in control flow statements like switch cases. They allow cleaner code that handles multiple cases efficiently.
Example of Enum in a Switch Case
Color myColor = Color::Green;
switch (myColor) {
case Color::Red:
// Handle red color
break;
case Color::Green:
// Handle green color
break;
case Color::Blue:
// Handle blue color
break;
}
By using enums in switch statements, you make it clear which values you expect, reducing the risk of errors.
Comparison and Type Checking
One of the advantages of enums is that they can be compared like regular integers. However, with scoped enums, you gain the added benefit of type safety, preventing mixing different enumeration types.

Common Use Cases of Enum Data Types in C++
Enumerations as State Machines
Enums are ideal for implementing state machines, as they can easily represent different states. For example:
enum class State { Idle, Running, Stopped };
State currentState = State::Idle;
// State transition logic
if (/* some condition */) {
currentState = State::Running;
}
Enforcing Valid Values in Function Parameters
Enums also play a crucial role in function parameters. By using an enum as a parameter type, only valid values can be passed to the function.
Example of Function Parameter Using Enum
void setLightColor(Color c) {
// Logic to set the light color based on the enum value
}
This function ensures that only `Color` values are accepted, preventing invalid inputs from creating bugs.
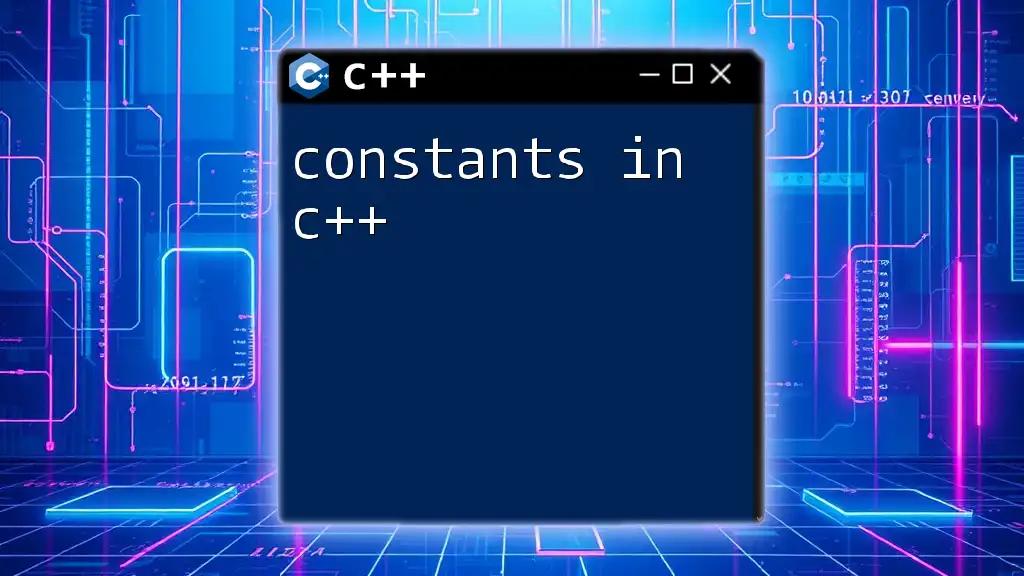
Best Practices for Using Enum in C++
When using enums in your code, consider following these best practices:
-
Naming Conventions for Enum Types: Use meaningful names and maintain a consistent naming strategy. For example, prefix enum types with `E` (e.g., `EColor`) can help distinguish them from other types.
-
Avoiding Magic Numbers with Enum Types: Enums are a great way to eliminate magic numbers in your code, improving maintainability.
-
Ensuring Enum Type Scope: Prefer using scoped enums over unscoped to take advantage of stronger type safety and avoid naming conflicts.

Conclusion
Understanding the enum data type in C++ is crucial for writing clean and maintainable code. The ability to define related constants together, enforce type safety, and improve code readability makes enums a valuable tool in any C++ programmer's toolkit. By mastering enums, you can significantly enhance the quality of your software and streamline your development process.