`enable_if` is a C++ template metaprogramming utility that selectively enables functions or class templates based on compile-time conditions, allowing for type-safe function overloading or template specialization.
#include <type_traits>
#include <iostream>
template<typename T>
typename std::enable_if<std::is_integral<T>::value, void>::type
process(T value) {
std::cout << "Processing integer: " << value << std::endl;
}
int main() {
process(10); // This works
// process(10.5); // This would cause a compile-time error
return 0;
}
Understanding enable_if
What is enable_if?
`enable_if` is a powerful utility in C++ that allows you to enable or disable template instantiations based on certain conditions. This feature is rooted in a broader concept known as SFINAE (Substitution Failure Is Not An Error), which means that if a substitution of a template parameter fails, it is not treated as an error, allowing the compiler to continue searching for valid template overloads.
The Syntax of enable_if
The basic syntax of `enable_if` is as follows:
std::enable_if<condition, type>::type
- condition: A compile-time boolean expression that determines whether the associated type is defined.
- type: The type that will be defined if the condition is true.
Here’s a simple example demonstrating the syntax:
template<typename T>
typename std::enable_if<std::is_integral<T>::value, void>::type myFunction(T arg);
In this case, `myFunction` will only be defined for integral types.
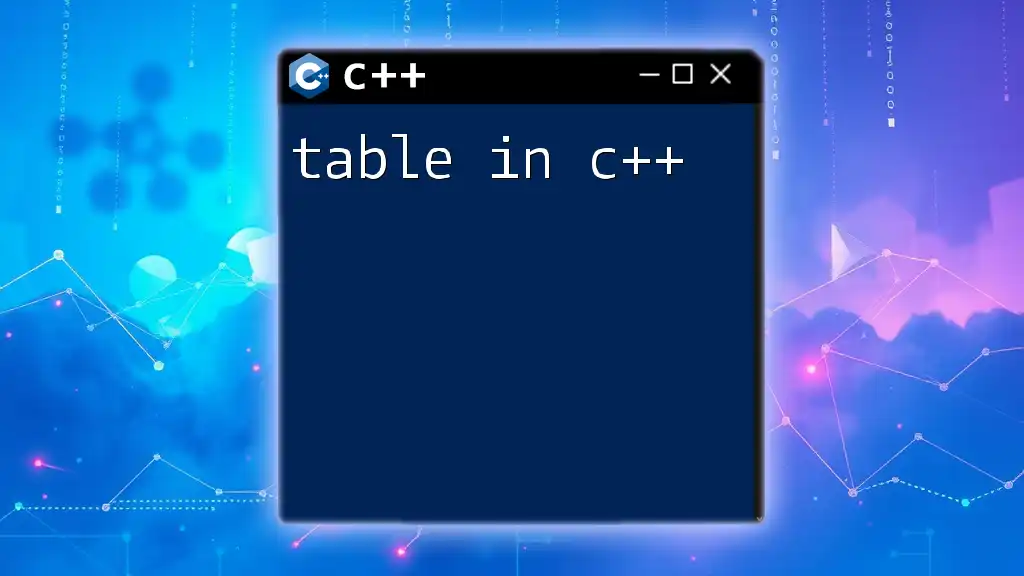
Practical Applications of enable_if
Enforcing Type Constraints in Templates
One of the practical applications of `enable_if` is enforcing type constraints in templates. This means you can restrict your function or class to only work with certain types.
Here’s an example that shows how to use `enable_if` to create a function that only accepts integral types:
template<typename T>
typename std::enable_if<std::is_integral<T>::value, void>::type
foo(T arg) {
// Implementation for integral types
std::cout << "Integral type!" << std::endl;
}
In this example, if you attempt to call `foo` with a non-integral type, the function will not be instantiated, effectively preventing compilation errors.
Conditional Function Overloading
Another great use case for `enable_if` is function overloading. You can provide different implementations of the same function based on the type of the argument.
Here’s an example demonstrating this:
template<typename T>
typename std::enable_if<!std::is_integral<T>::value, void>::type
foo(T arg) {
// Implementation for non-integral types
std::cout << "Non-integral type!" << std::endl;
}
In this function, if `T` is not an integral type, the provided implementation will be used, while integral types will be ignored without recompilation errors. This is extremely useful for writing clear and semantic code.
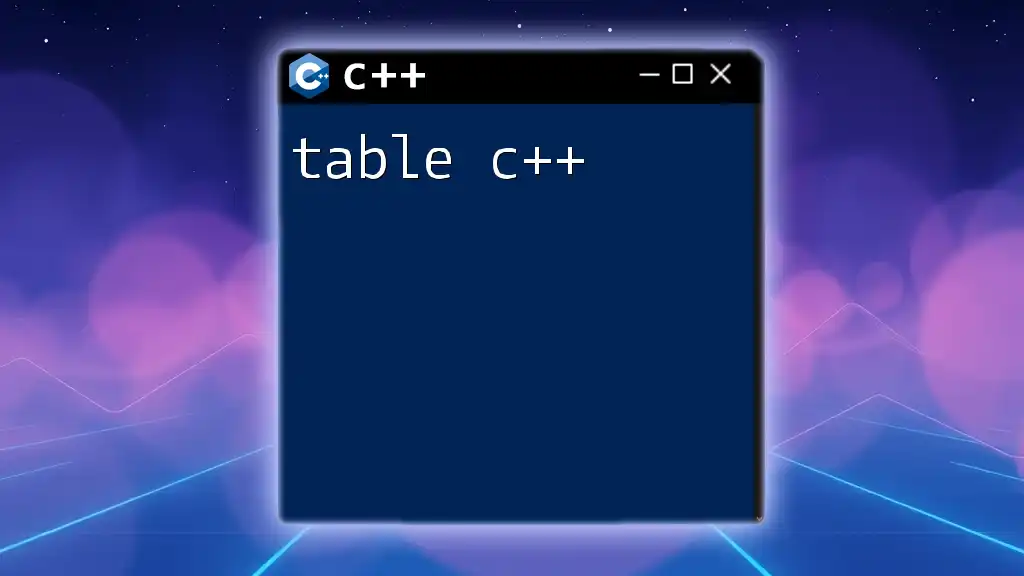
Using enable_if with Classes
Enabling Class Templates with enable_if
`enable_if` can be used not just with functions but also with class templates. This enables you to conditionally define a class based on some type traits.
Here’s how you can do this:
template<typename T, typename = typename std::enable_if<std::is_floating_point<T>::value>::type>
class MyClass {
public:
void display() {
std::cout << "Floating-point type!" << std::endl;
}
};
In this example, `MyClass` can only be instantiated if `T` is a floating-point type. If you attempt to create an instance of `MyClass<int>`, it will result in a compilation error.
Combining enable_if with Inheritance
You can also use `enable_if` to create derived classes that are constrained to certain types. This is especially useful in template design patterns.
Consider the following example:
template <typename T>
class Base {};
template <typename T>
class Derived : public Base<T> {
static_assert(std::is_arithmetic<T>::value, "T must be an arithmetic type");
};
In this case, the `Derived` class will only inherit from `Base` if `T` is an arithmetic type, ensuring that inappropriate types cannot be used.
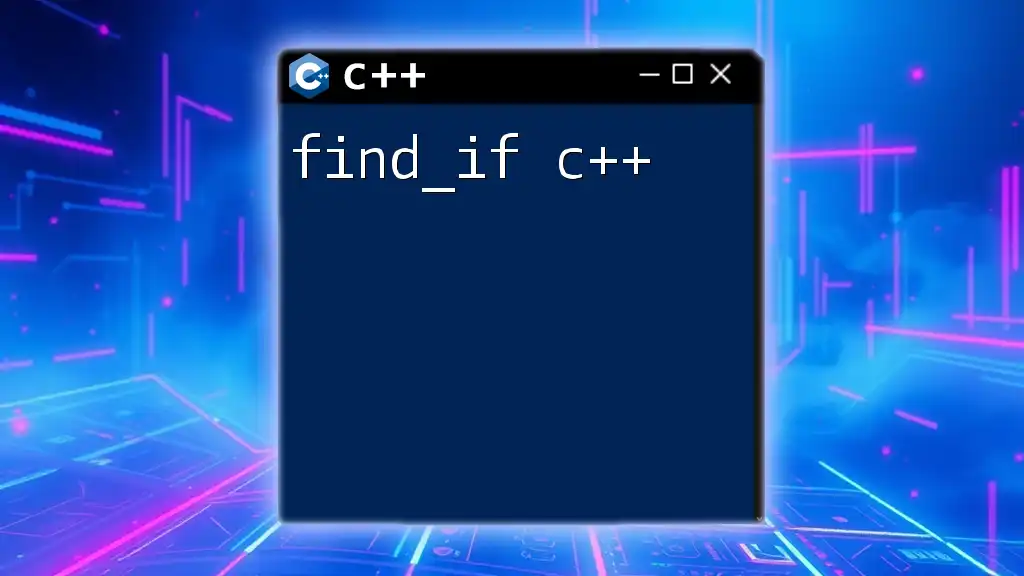
Advanced Usage of enable_if
Custom Type Traits
`enable_if` can also work hand-in-hand with custom type traits that you create. This means that you can define more complex conditions for when certain operations should be valid.
Here is an example:
template <typename T>
struct is_special_type {
static const bool value = /* some condition */;
};
template <typename T>
typename std::enable_if<is_special_type<T>::value, void>::type
specialFunction() {
// Function implementation
}
In this snippet, `specialFunction` will only be defined if `T` meets the criteria defined in `is_special_type`. This flexibility allows for robust type-checking and handling in your templates.
Compounding enable_if with std::conditional
You can also combine `enable_if` with `std::conditional` to create more complex template logic depending on types.
For instance:
template<typename T>
using ResultType = typename std::conditional<std::is_integral<T>::value, int, double>::type;
// Usage
ResultType<float> myVariable; // This will be double.
In this scenario, `ResultType` will evaluate to `int` if `T` is an integral type; otherwise, it results in `double`. This allows for dynamic type selection based on conditions.
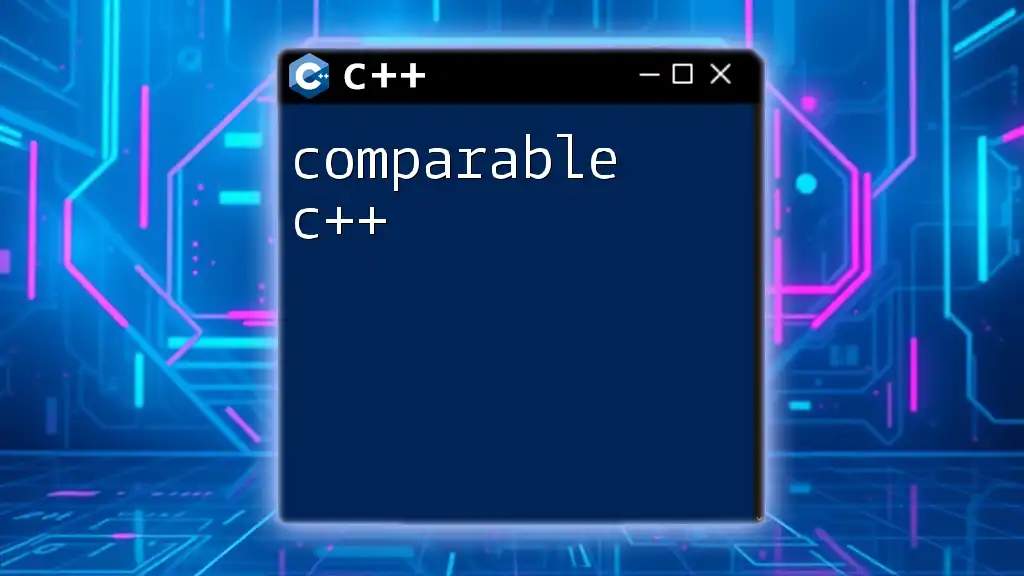
Common Mistakes and Pitfalls
Overusing enable_if
While `enable_if` is incredibly useful, overusing it can lead to code complexity and decreased readability. It's important to strike a balance and only use it when necessary to maintain clarity and avoid excessive template metaprogramming complications.
Confusing enable_if with Other Techniques
Many newcomers might confuse `enable_if` with other C++ features, such as `static_assert`. While both are compile-time checks, `enable_if` is often used for conditional template instantiation, while `static_assert` checks conditions at the point of function or class definition. Choosing the right tool for your needs is crucial.
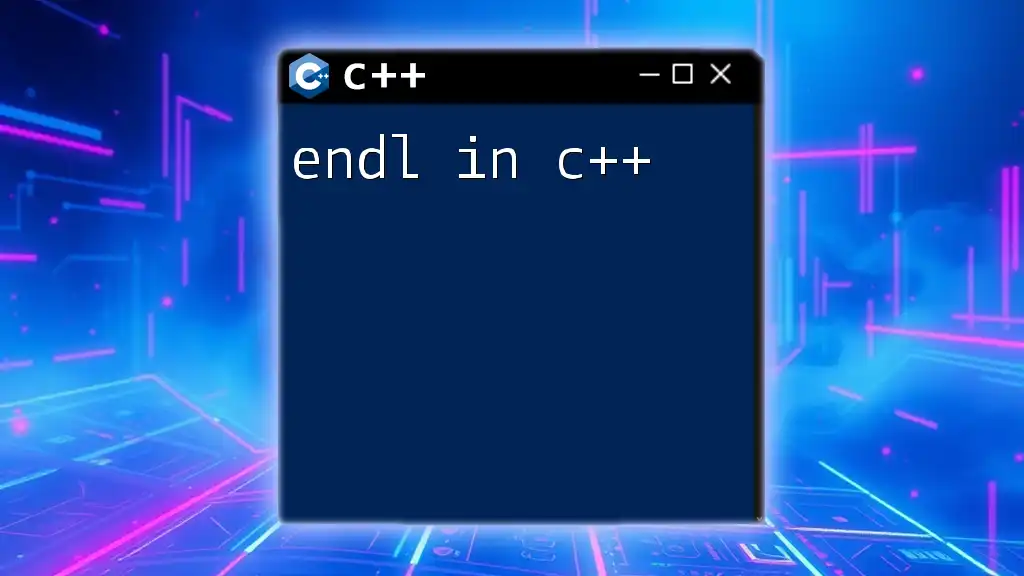
Conclusion
In summary, `enable_if` in C++ is an invaluable tool that enables you to write safer, more generic code by conditionalizing template parameters. With its help, you can enforce type constraints, facilitate function overloading, and create robust class templates. Leveraging `enable_if` will lead to cleaner and more maintainable C++ code.
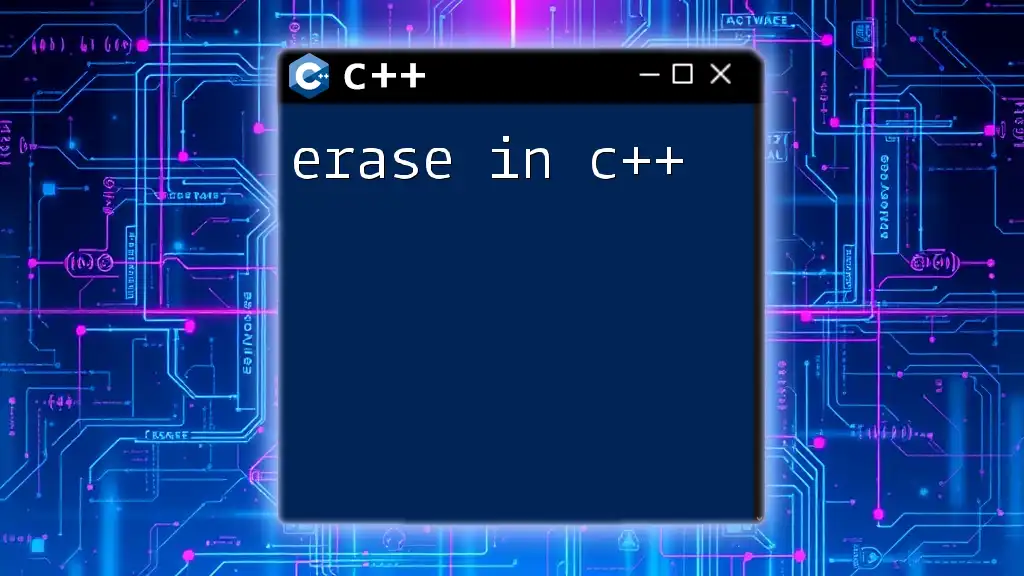
Additional Resources
For those looking to expand their knowledge on this topic, consult the official [C++ standard library documentation](https://en.cppreference.com/w/cpp/types/enable_if) on `std::enable_if`, explore tutorials on advanced C++ techniques, and consider taking online courses dedicated to template metaprogramming.
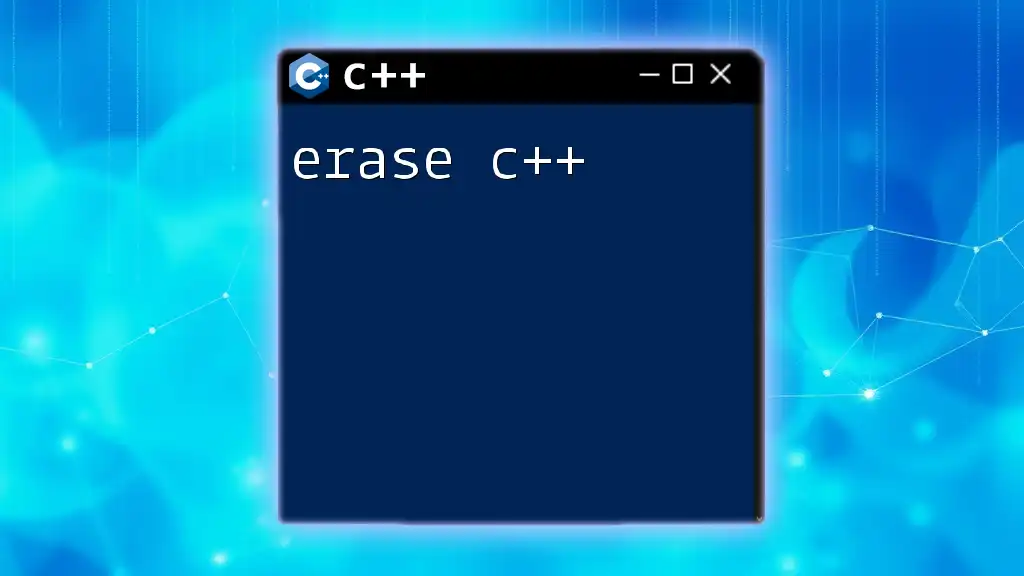
Call to Action
Have you used `enable_if` in your projects? Share your experiences or any questions you might have in the comments below. Don't forget to subscribe for more insights into C++ programming and advanced concepts!