In C++, a table can be represented using a two-dimensional array, where each element can be accessed through its row and column indices.
Here's a simple example of a 2D table implemented in C++:
#include <iostream>
using namespace std;
int main() {
// Define a 2D array (table) with 3 rows and 4 columns
int table[3][4] = {
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12}
};
// Print the table
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 4; j++) {
cout << table[i][j] << " ";
}
cout << endl;
}
return 0;
}
Understanding Tables in C++
What is a Table in C++?
In C++, a table is a structured arrangement of data, typically organized in rows and columns. The most common implementation of a table is through arrays, which allow for easy access to data elements using an index. Tables serve a critical role in managing and organizing data efficiently, making them indispensable in programming.
Using tables in programming enhances the capability to handle large datasets, aiding in the effective implementation of algorithms that require systematic access to data. With tables, developers can maintain organized structures that simplify data manipulation tasks.
Why Use Tables?
Tables offer several benefits for data organization:
- Efficient Data Representation: Tables allow for a clear and organized presentation of data.
- Ease of Access: Elements within a table can be accessed quickly using an index, making data retrieval fast and straightforward.
- Enhanced Performance: When employing the right form of tables (static vs. dynamic), performance can be significantly improved for specific operations.
- Extensive Use Cases: Tables are commonly utilized in various applications, from databases to game development and beyond.
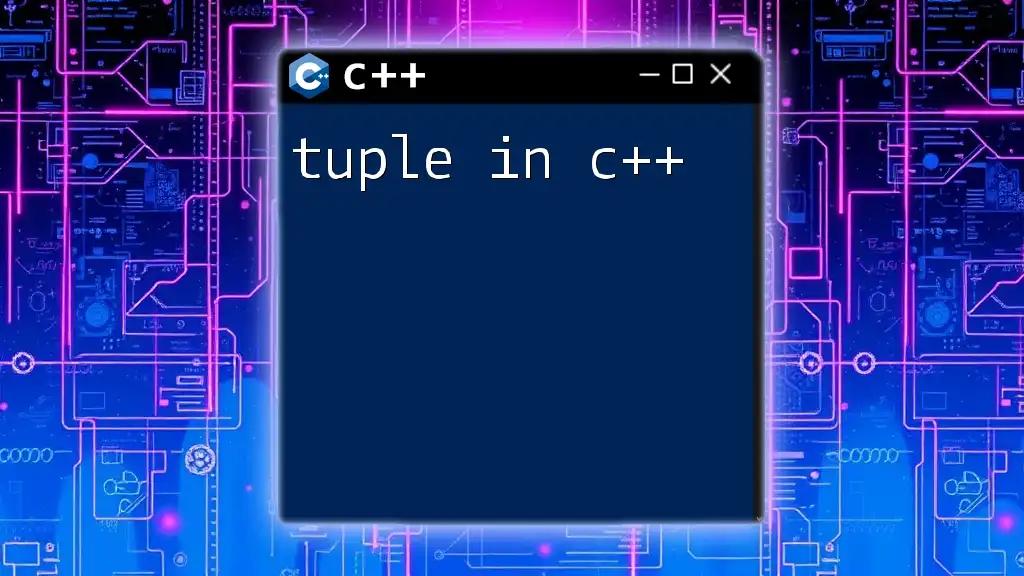
Types of Tables in C++
One-Dimensional Tables (Arrays)
Definition and Syntax
One-dimensional tables, commonly known as arrays, provide a linear structure for storing a collection of elements of the same data type. The syntax for declaring a one-dimensional array is straightforward:
int arr[5] = {10, 20, 30, 40, 50};
This code snippet declares an integer array named `arr` with five elements.
Accessing and Modifying Elements
Accessing elements in a one-dimensional array is achieved through indexing, where the array's index starts at 0. For instance, to access the third element:
int value = arr[2]; // value will be 30
To modify an element, simply assign a new value to the indexed position. For example:
arr[2] = 35; // Modifying the third element to 35
Two-Dimensional Tables (Matrices)
Definition and Syntax
Two-dimensional tables, or matrices, extend the concept of arrays into a grid-like structure, organized in rows and columns. The declaration of a two-dimensional array can be done as follows:
int matrix[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
Here, `matrix` consists of three rows and three columns.
Accessing and Modifying Elements
In a two-dimensional table, elements are accessed using two indices: one for the row and another for the column. For instance, to access the element in the second row and third column:
int value = matrix[1][2]; // value will be 6
You can modify an element in a two-dimensional array in a similar fashion:
matrix[1][2] = 10; // Modifying the element at row 2, column 3

Advanced Table Features
Dynamic Tables
What is a Dynamic Table?
Dynamic tables provide a flexible way of managing memory allocation, allowing developers to create tables that can adjust in size during runtime. This is particularly useful when the required size of the table is not known ahead of time.
Using `new` and `delete`
When working with dynamic tables, memory allocation and deallocation become crucial. Below is an example of how to create and delete a dynamic array:
int* dynamicArray = new int[5]; // Dynamic allocation
delete[] dynamicArray; // Deallocation
Using `new` allocates memory for a specified number of elements, while `delete` frees that memory, preventing memory leaks.
Structure of Tables Using Structs
Creating a Struct
Structs allow for more complex data organization, enabling the grouping of different data types into a single type. For example:
struct Student {
int id;
std::string name;
};
This structure defines a `Student` with an `id` and `name`.
Array of Structs
An array of structs can be utilized to manage multiple instances of these structures effectively:
Student students[2] = {{1, "Alice"}, {2, "Bob"}};
Here, an array named `students` stores details about two students.

Practical Examples of Tables
Example 1: Storing Student Grades
Overview
Consider a scenario where you need to store the grades of students across several subjects. A two-dimensional array is ideal for this task.
Implementation
int grades[3][5] = {
{85, 90, 78, 92, 88},
{75, 80, 70, 79, 81},
{90, 85, 88, 94, 92}
};
Here, `grades` is a matrix that holds three students' grades across five subjects.
Explanation of Code
In this example, each row corresponds to a student, and each column represents a subject. Accessing a specific grade can be easily done through indexing:
int studentOneGrade = grades[0][1]; // Accessing the grade of student 1 in subject 2
Example 2: Creating a Simple Contacts List
Overview
A typical use case for tables is managing a list of contacts. Using structs enables you to combine various attributes (like name and phone number) into a cohesive structure.
Implementation
struct Contact {
std::string name;
std::string phone;
};
Contact contacts[3] = {
{"John", "123-456-7890"},
{"Jane", "987-654-3210"},
{"Doe", "555-555-5555"}
};
This code defines a simple contacts list, where each contact's name and phone number are stored.
Explanation of Code
Here, an array named `contacts` holds three instances of `Contact`. Accessing a contact's information is as simple as:
std::string johnsPhone = contacts[0].phone; // Accessing John's phone number
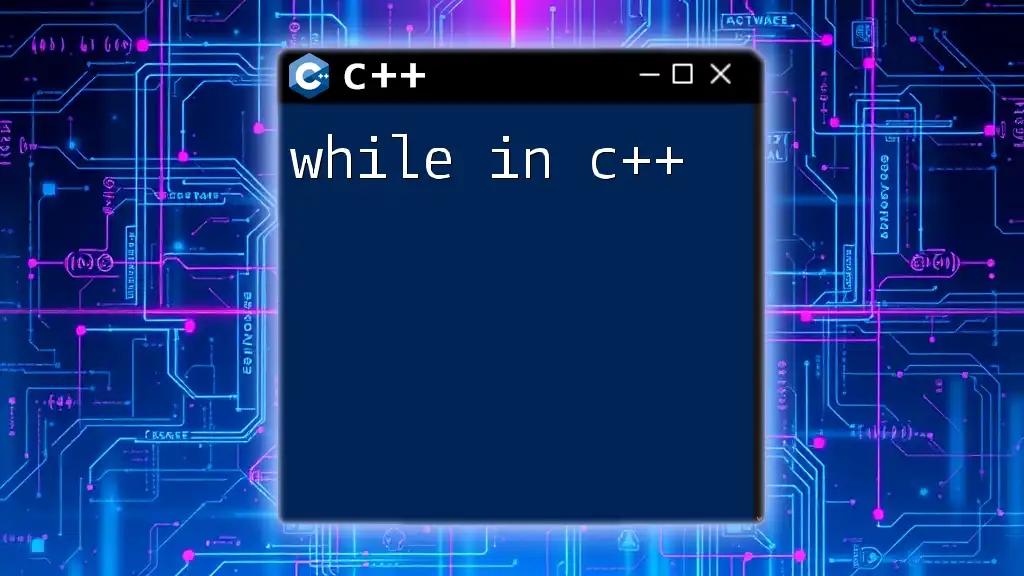
Best Practices and Tips
Efficient Memory Management
When working with dynamic tables, efficient memory management is crucial. Always ensure that every allocation using `new` has a corresponding deallocation with `delete` to avoid memory leaks. This practice increases the robustness of your applications and enhances their performance.
Access Patterns and Performance
Understanding the access patterns of data within tables provides insights into optimizing performance. For instance, accessing elements in a sequential manner is faster due to better cache locality, whereas random access may lead to inefficiencies. Keep the nature of your application in mind when designing tables.
Common Errors and Debugging Tips
Common mistakes when using tables include accessing out-of-bounds elements, which can lead to undefined behavior, and failing to manage memory properly in dynamic scenarios. Always implement checks to ensure indices remain within valid ranges, and use debugging techniques to identify issues effectively.

Conclusion
In summary, mastering the concept of a table in C++ is essential for effective data management and manipulation in programming. From one-dimensional arrays to complex structures, understanding how to implement and utilize tables can lead to significant improvements in code efficiency and organization. Continuing to practice these concepts will undoubtedly enhance your programming proficiency.
For further exploration, consider delving deeper into dynamic memory management, advanced data structures, and algorithm optimizations for tables in C++. Happy coding!