The `while` loop in C++ repeatedly executes a block of code as long as a specified condition is true. Here's an example:
#include <iostream>
int main() {
int count = 0;
while (count < 5) {
std::cout << "Count is: " << count << std::endl;
count++;
}
return 0;
}
Understanding the Basics of C++ Loops
What Are Loops?
Loops are fundamental constructs in programming that allow you to execute a block of code multiple times based on a specific condition. They help automate repetitive tasks, thus reducing redundancy in code. In C++, there are several types of loops, including `for` loops, `while` loops, and `do while` loops. Each type has its use cases, but the commonality lies in their capability to iterate until a certain condition is met.
Why Use a While Loop?
The `while` loop is particularly useful when the number of iterations is not known beforehand and depends on a condition. It checks the condition before executing the loop body, making it ideal for scenarios where you need to continue executing code until a specific condition stops being true. This makes `while` loops preferable for tasks like reading user input until a valid value is provided.
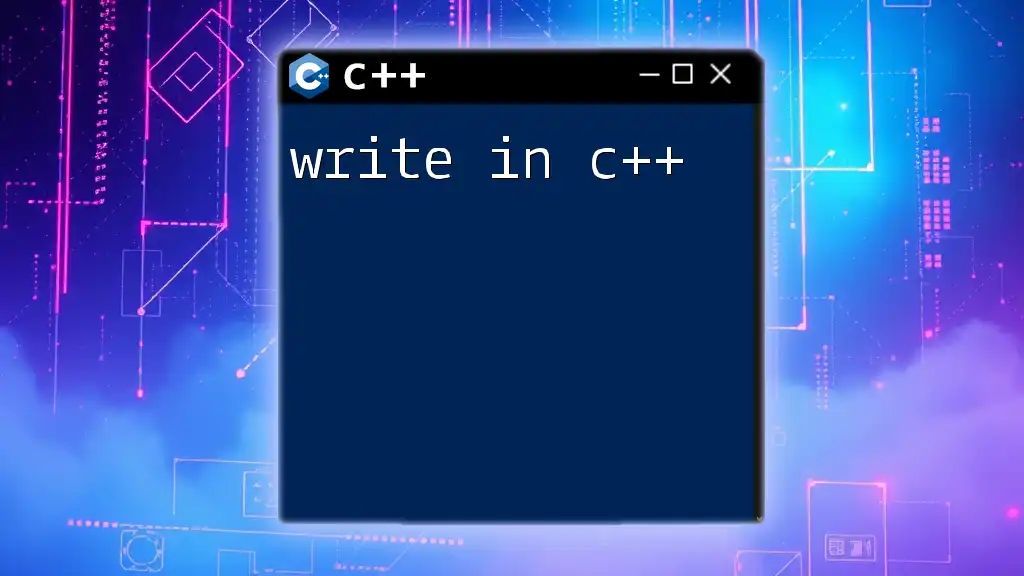
While Loop in C++
Syntax of While Loop in C++
The syntax for a `while` loop in C++ is straightforward. It consists of the `while` keyword followed by a condition in parentheses, and the code block to execute enclosed in braces. Here’s the general format:
while (condition) {
// code to be executed
}
- Condition: A Boolean expression that is evaluated before each iteration. If true, the loop continues; if false, the loop terminates.
- Code Block: The set of statements that will be executed repeatedly as long as the condition is true.
Working of While Loop
When the `while` loop is executed, the program first evaluates the condition. If it resolves to true, the code block is executed. After executing the code, the program jumps back to evaluate the condition once again. This process continues until the condition evaluates to false. The flow of control in a `while` loop can be visualized as:
- Evaluate condition
- If true, execute code block
- Go back to step 1
This continual checking makes the `while` loop a powerful structure for controlling repetitive tasks.
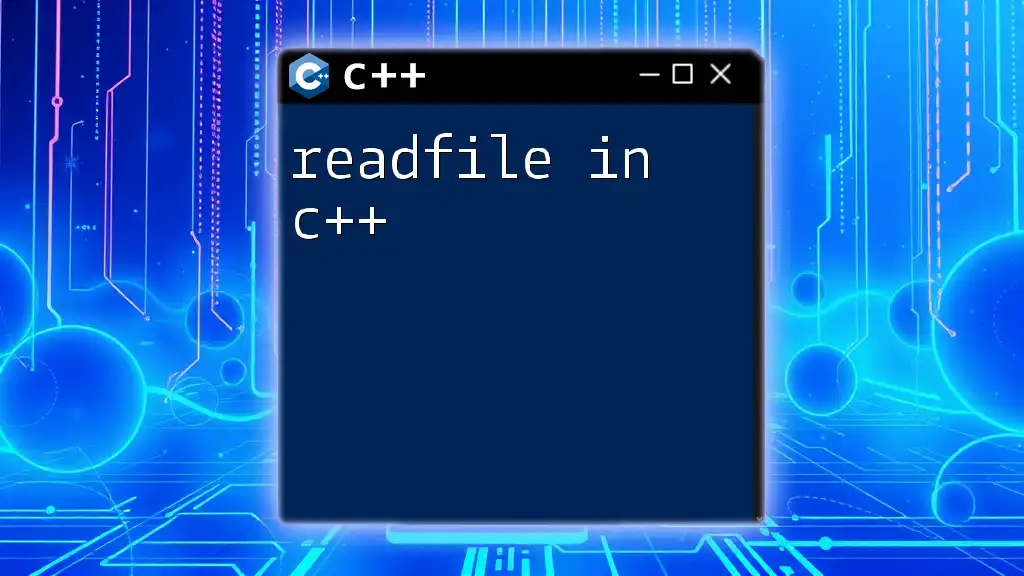
Examples of While Loop in C++
Simple While Loop Example
To illustrate how a `while` loop operates, consider the following simple example that counts from 0 to 4:
#include <iostream>
using namespace std;
int main() {
int i = 0;
while (i < 5) {
cout << "i is: " << i << endl;
i++;
}
return 0;
}
In this code:
- We initialize an integer variable `i` to 0.
- The loop checks if `i` is less than 5. If true, it prints the value of `i` and increments it by 1.
- This will continue until `i` reaches 5, after which the condition will be false, and the loop will terminate.
Using a While Loop with User Input
Sometimes the condition for continuing a loop is based on user input. Here’s an example that keeps asking for input until the user enters zero:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a positive number (0 to end): ";
cin >> number;
while (number != 0) {
cout << "You entered: " << number << endl;
cout << "Enter another number (0 to end): ";
cin >> number;
}
return 0;
}
In this example:
- The loop continues to prompt the user for input as long as the `number` entered is not zero.
- This demonstrates a real-world application of a `while` loop, making it user-interactive and demonstrating its power in handling conditions based on runtime data.
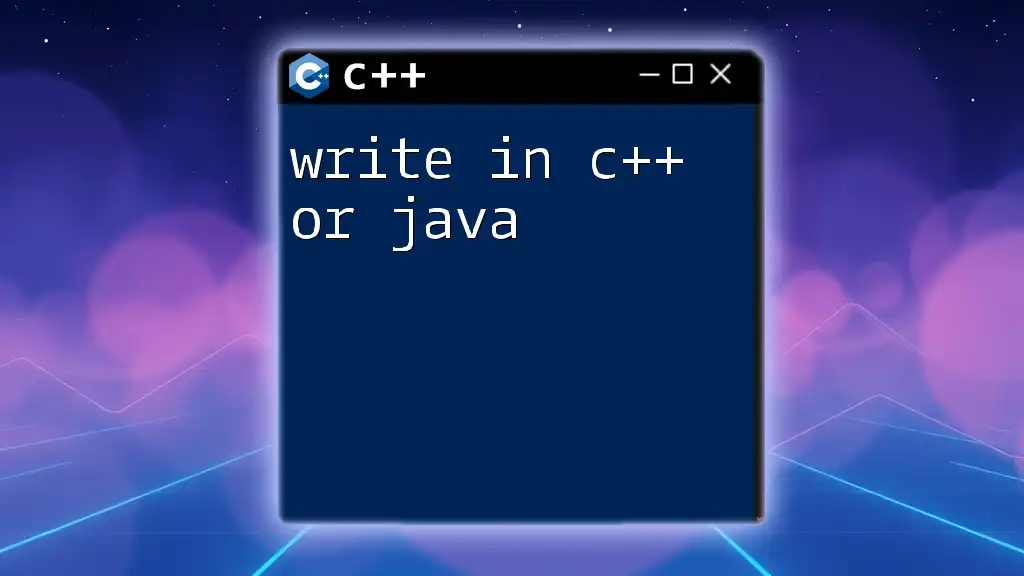
Do While Loop in C++
Understanding the Do While Loop
The `do while` loop is similar to a `while` loop but with a significant difference: the code block within a `do while` loop is executed at least once before the condition is evaluated. This makes it useful when you want to ensure at least a single execution of the block.
Syntax of Do While Loop in C++
The syntax for a `do while` loop is as follows:
do {
// code to be executed
} while (condition);
Here, the code inside the `do` block runs first, and then the condition checks whether to execute it again. If the condition evaluates to true, the loop runs once more; if false, the loop terminates.
Example of a Do While Loop
To illustrate, consider a `do while` loop that prints "Hello, World!" a specified number of times:
#include <iostream>
using namespace std;
int main() {
int i = 0;
do {
cout << "Hello, World!" << endl;
i++;
} while (i < 5);
return 0;
}
In this code:
- The loop will print "Hello, World!" five times.
- Regardless of the value of `i`, the statement will be executed at least once because the condition is only checked after the loop body runs.
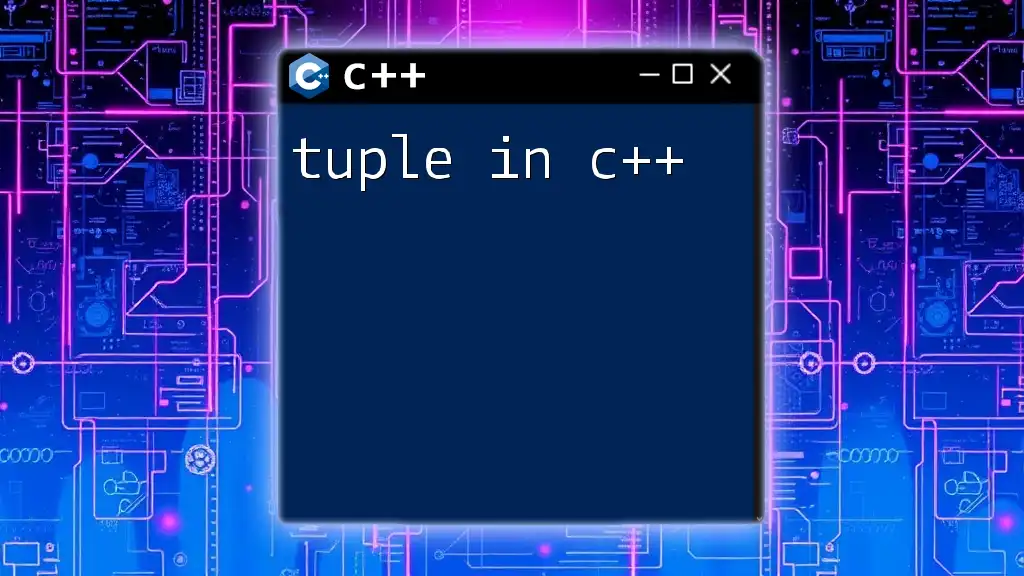
Common Patterns with While Loops
Infinite Loops
An infinite loop occurs when the condition of a loop always evaluates to true. While these loops might have legitimate uses in some cases, they often indicate a coding error. For example:
#include <iostream>
using namespace std;
int main() {
while (true) {
cout << "This will run forever!" << endl;
// break or return statement to exit the loop would go here
}
return 0;
}
In this snippet, the loop will output the string indefinitely unless interrupted or terminated through an external force. Infinite loops can cause programs to crash or become unresponsive, so it's important to use them judiciously.
Nested While Loops
Nested loops occur when one loop runs inside another. This is useful for handling multidimensional data structures, such as matrices. Here’s an example:
#include <iostream>
using namespace std;
int main() {
int i = 1;
while (i <= 3) {
int j = 1;
while (j <= 2) {
cout << "i: " << i << ", j: " << j << endl;
j++;
}
i++;
}
return 0;
}
This example illustrates how the outer `while` loop iterates three times while the inner loop iterates twice for each outer loop iteration. This coupling notably increases the total number of iterations, making it an effective pattern for exploring complex data relationships.
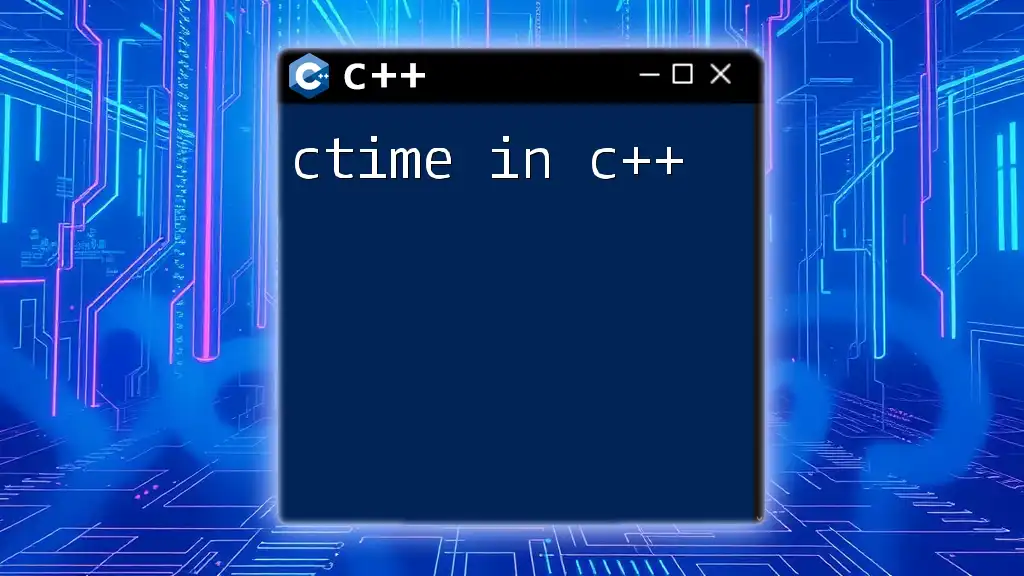
Best Practices for While Loops in C++
Avoiding Infinite Loops
To prevent infinite loops, it’s crucial to ensure that the condition will eventually resolve to false. This often involves properly updating the variable checked in the condition within the loop body. Regularly auditing your code will help identify potential issues that may lead to unwanted infinite iterations.
Performance Considerations
When deciding whether to use a `while` loop, it's essential to consider your specific needs:
- Use `while` loops when the number of iterations is unknown and depends on dynamic conditions.
- Opt for `for` loops when the number of iterations is known ahead of time.
- Resort to `do while` loops when at least one iteration is mandatory, regardless of the condition.
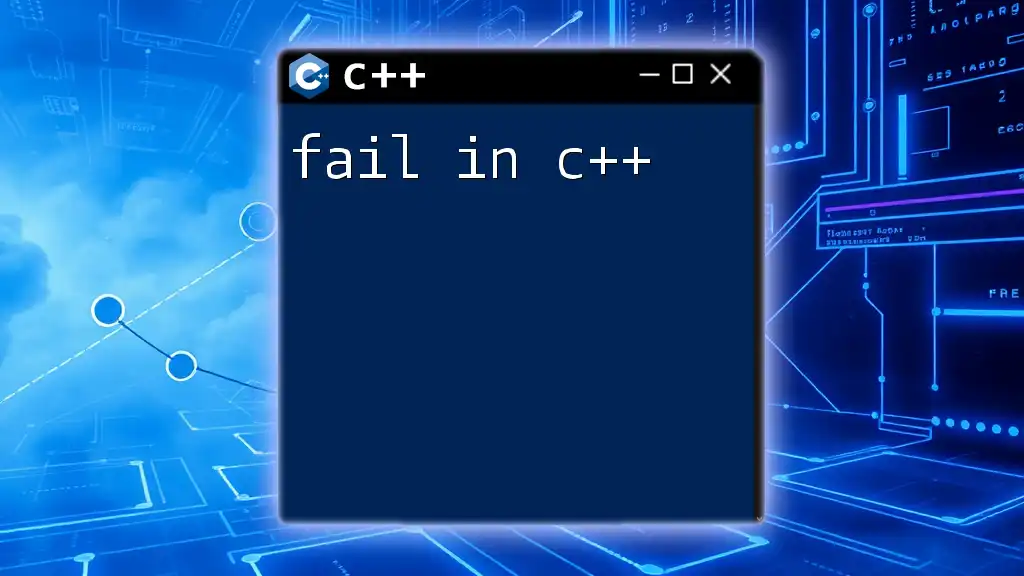
Conclusion
In summary, understanding the while in C++—including its syntax, functionality, and variations like the `do while` loop—empowers you to effectively handle repetitive tasks in programming. Mastering these loops is fundamental as they form the backbone of many algorithms and operations within C++. By practicing through examples and applying best practices, you can enhance your coding skills and write efficient C++ programs.