In C++, the `sizeof` operator is used to determine the size (in bytes) of a data type or variable, and it is often utilized to find the size of an `int` as follows:
#include <iostream>
int main() {
std::cout << "Size of int: " << sizeof(int) << " bytes" << std::endl;
return 0;
}
What is an Integer in C++?
In programming, an integer is a whole number that can be positive, negative, or zero. C++ provides a range of integer types, which can be categorized into signed and unsigned integers. Signed integers can represent both positive and negative values, while unsigned integers only represent non-negative values.
In C++, integers are widely used to store and manipulate numerical data. They are fundamental to various applications, including algorithms, data structures, and mathematical computations. Understanding the characteristics of integers is essential for efficient programming and memory management.
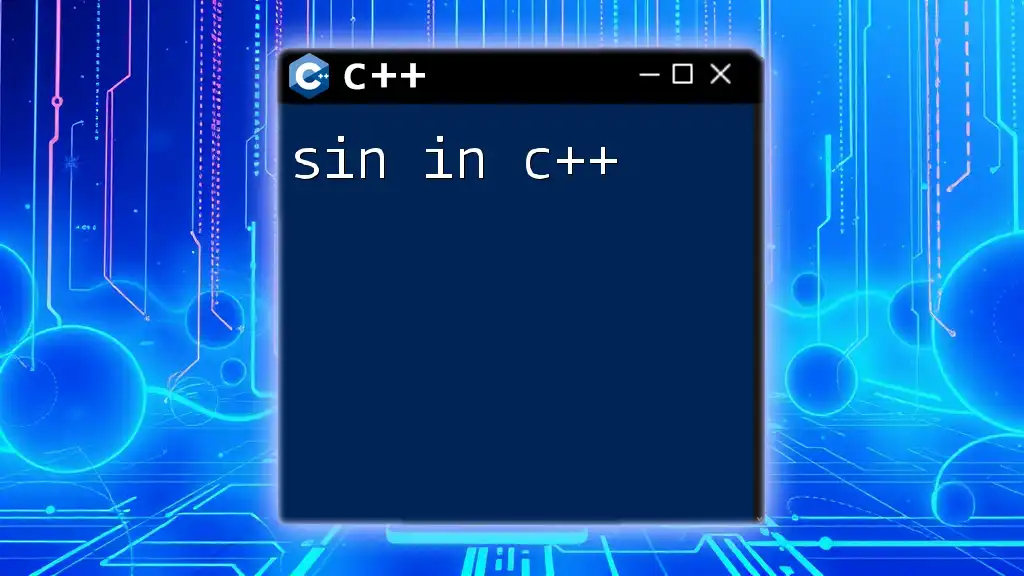
Understanding Data Types in C++
Data types in C++ define the type of data a variable can hold and the operations that can be performed on that variable. The common data types in C++ include:
- Primitive types: such as `int`, `char`, `float`, and `double`.
- Derived types: like arrays and pointers.
- User-defined types: including structs and classes.
Integer types in C++ are specifically designed for storing whole numbers. The most frequently used integer types include `int`, `short`, `long`, and `long long`. Each type offers a different range of values and has a different size in memory.
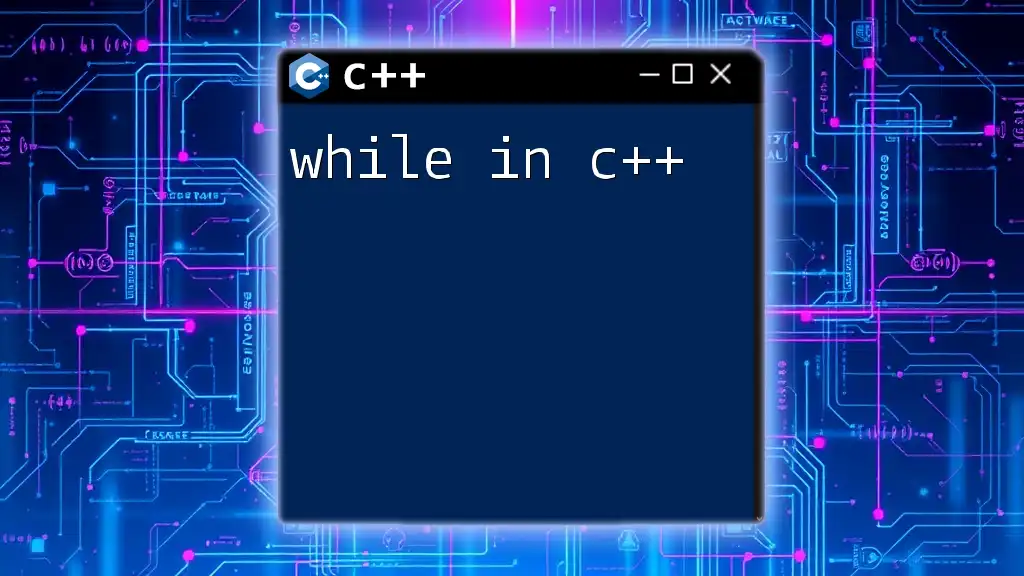
Size of Integer in C++
Fundamentals of Size in C++
Size in programming refers to the amount of memory allocated for a specific data type. Knowing the size of an integer type is crucial for efficient resource management and optimal code performance. Different integer types occupy varying amounts of space in memory, which can impact calculations and performance.
Size of Integer in C++
The size of `int` in C++ is platform-dependent. On most 32-bit systems, the size of `int` is generally 4 bytes (32 bits), while on 64-bit systems, it often remains 4 bytes as well. The most reliable way to determine the size of an `int` is to use the `sizeof` operator.
Here’s a simple code snippet to demonstrate this:
#include <iostream>
using namespace std;
int main() {
cout << "Size of int: " << sizeof(int) << " bytes" << endl;
return 0;
}
When executed, this program outputs the byte size of the `int` type, allowing developers to see how much memory is used.
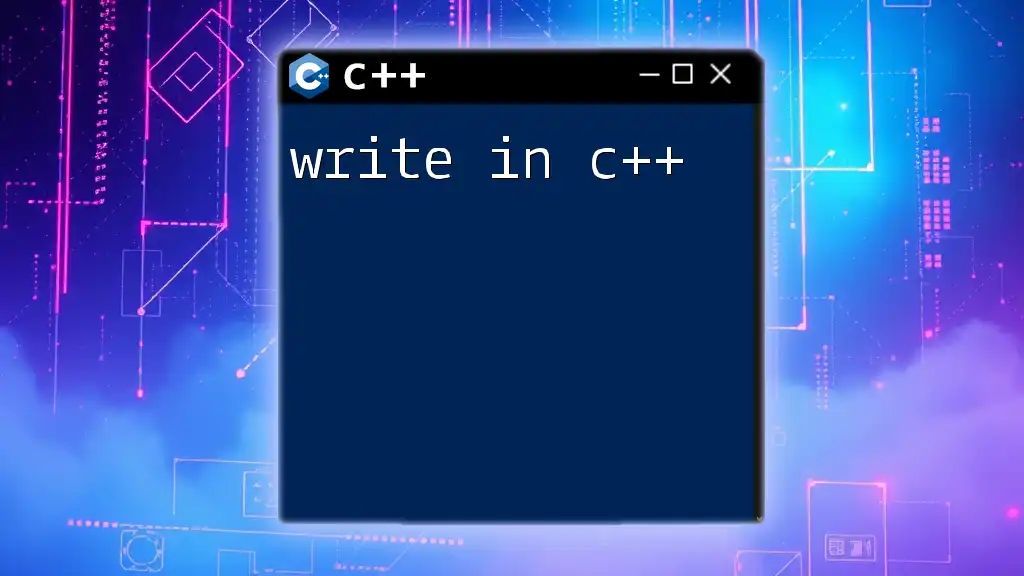
Variants of Integer Sizes
int Size C++
The standard `int` type is designed to handle a wide range of integer values. It is versatile and is the default integer used in most applications. However, the usage of `int` can have limitations based on the context or data range required.
Exploring Other Integer Types
To extend the capabilities of the basic `int` type, C++ provides several variants that can handle different ranges of numerical data.
-
Short Integers: The `short` type typically occupies 2 bytes (16 bits), limiting its range compared to `int` but allowing for more compact storage.
cout << "Size of short: " << sizeof(short) << " bytes" << endl;
-
Long Integers: The `long` type generally allocates 4 bytes on 32-bit systems, and 8 bytes on 64-bit systems, giving it a broader range than `int`.
cout << "Size of long: " << sizeof(long) << " bytes" << endl;
-
Long Long Integers: For applications requiring very large numbers, the `long long` type provides at least 8 bytes (64 bits) of storage.
cout << "Size of long long: " << sizeof(long long) << " bytes" << endl;
Signed vs. Unsigned Integers
The distinction between signed and unsigned integers is crucial in programming.
-
Signed integers can represent both negative and positive values. For example, a signed `int` can range from approximately -2 billion to +2 billion.
-
Unsigned integers, conversely, can only represent non-negative values. This gives them a broader positive range but limits their capability to represent negative numbers.
To illustrate, the code below shows the size of an unsigned integer:
cout << "Size of unsigned int: " << sizeof(unsigned int) << " bytes" << endl;
By understanding the difference between signed and unsigned integers, developers can make informed decisions when choosing the right type for their applications.
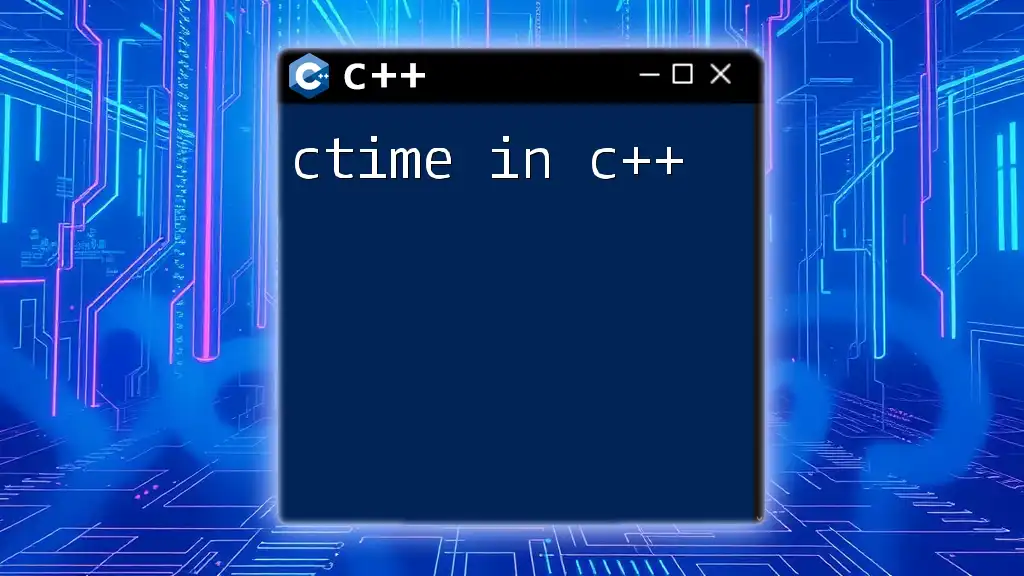
Practical Implications of Integer Sizes
Importance of Choosing the Right Integer Type
Selecting the appropriate integer type has significant implications for memory efficiency and performance. Each type's size affects how much memory is used by variables, which can influence the overall resource consumption of a program. In tightly constrained environments, such as embedded systems or lower-end hardware, choosing a smaller data type can optimize performance and memory usage.
When to Use Specific Integer Types
Understanding when to use different integer types can lead to clearer and more efficient code. Here are some general guidelines:
- Use `int` when the values will generally fall within the typical range of -2,147,483,648 to 2,147,483,647.
- Use `short` for memory-constrained applications where values are consistently small.
- Opt for `long` or `long long` when working with large numerical values or situations that may exceed the range of standard integers.
- Choose unsigned types when you are certain that the values will always be non-negative, which can effectively double the maximum representable value.
For instance, in game development or financial calculations, selecting the correct integer type—whether for representing player scores or monetary amounts—can significantly affect performance and correctness.
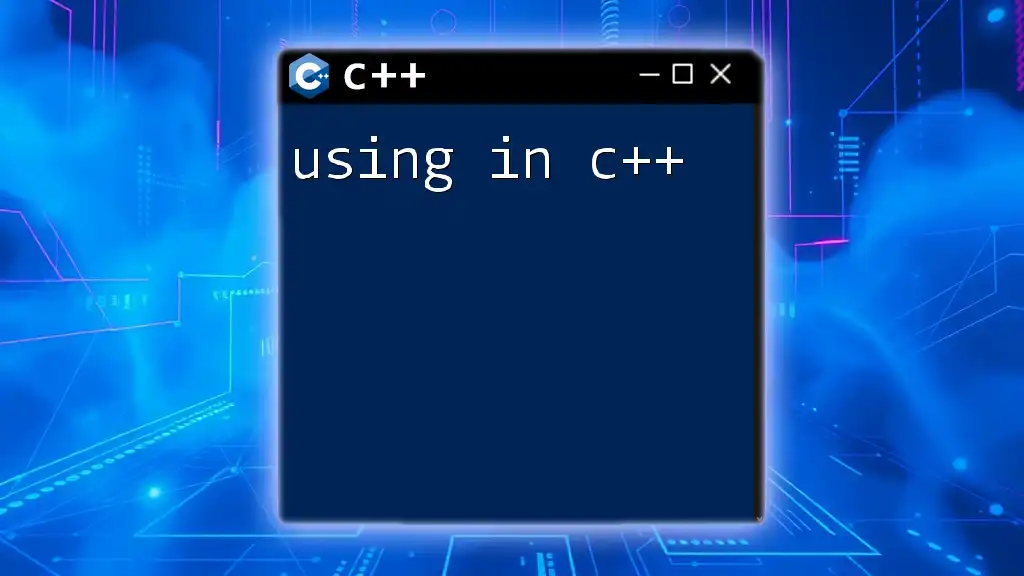
Conclusion
In summary, understanding the size of integers in C++ is fundamental for effective programming practices. The knowledge of the various integer types, their sizes, and their applications allows developers to optimize their code, manage resources effectively, and avoid potential pitfalls that arise from misusing data types.
Final Thoughts on C++ Integer Sizes
Developers are encouraged to explore further and gain a deeper understanding of how to utilize integer types efficiently in their applications. Mastery of integer sizes can lead to improved programming skills and contribute to more robust software solutions.
![Understanding Unsigned Int in C++ [Quick Guide]](/images/posts/u/unsigned-int-cpp.webp)
Additional Resources
For those seeking additional insights into C++ integer types and best practices, consulting recommended books, articles, and interactive tutorials will enhance your understanding and skills in programming with C++.