An `unsigned int` in C++ is a data type that represents a non-negative integer, allowing a range of values from 0 to 4,294,967,295 on most systems.
unsigned int num = 42; // Example of declaring and initializing an unsigned integer
Overview of Data Types in C++
Understanding C++ Data Types
In C++, data types are classifications of data items. They specify the type of data a variable can hold, which influences the operations that can be performed on that data. C++ data types can be categorized into three main groups: fundamental types (like `int`, `char`, and `float`), derived types (like arrays and pointers), and user-defined types (like structs and classes). Each type plays a crucial role in programming, allowing for efficient memory usage and data manipulation.
What are Unsigned Integers?
An unsigned integer is a data type that can only represent non-negative whole numbers. As the name suggests, the term unsigned indicates that these integers do not reserve a bit for negative numbers, effectively doubling the maximum value that can be stored compared to a signed integer. This means that if an `int` can hold values from -2,147,483,648 to 2,147,483,647, an `unsigned int` can hold values from 0 to 4,294,967,295.
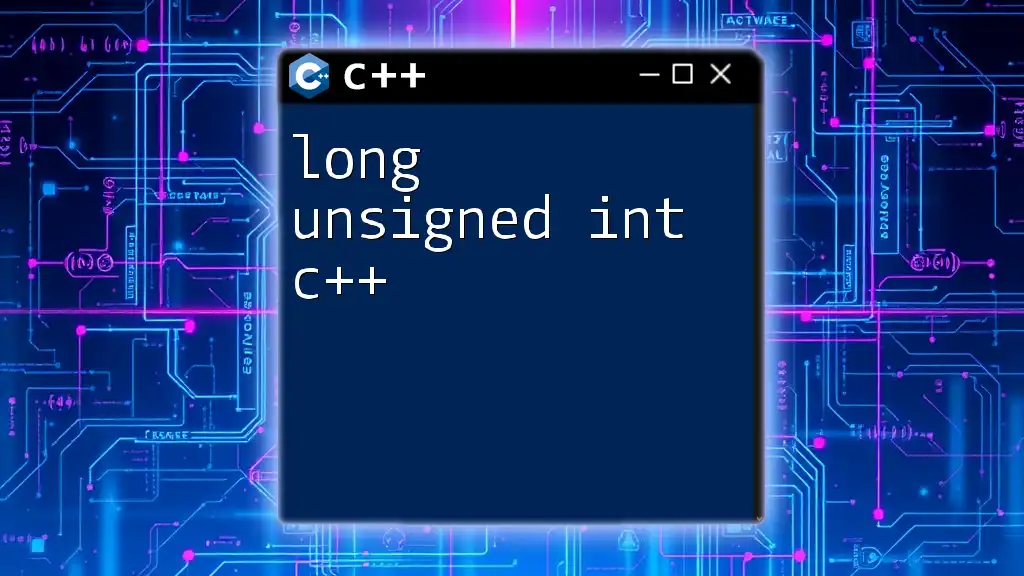
Characteristics of `unsigned int`
Memory Allocation and Range
In a typical 32-bit system, both `int` and `unsigned int` are allocated 4 bytes in memory. However, the significant difference lies in the range of values they can store. An `unsigned int` can maximize its range by allowing all bits to contribute to the value rather than reserving a bit for the sign. The range of `unsigned int` therefore is from 0 to 4,294,967,295. This range makes `unsigned int` particularly useful for cases where only non-negative numbers are needed, such as counting items or indexing arrays.
Advantages of Using `unsigned int`
Using `unsigned int` provides several advantages, which include:
- Wider Range: For applications requiring larger values without the need for negative numbers, `unsigned int` doubles the positive range compared to a signed integer.
- Performance: Operations involving unsigned integers can be marginally more efficient on some platforms.
Certainly, careful consideration is needed while utilizing this data type to avoid unintended behavior, particularly when interfacing with signed types.
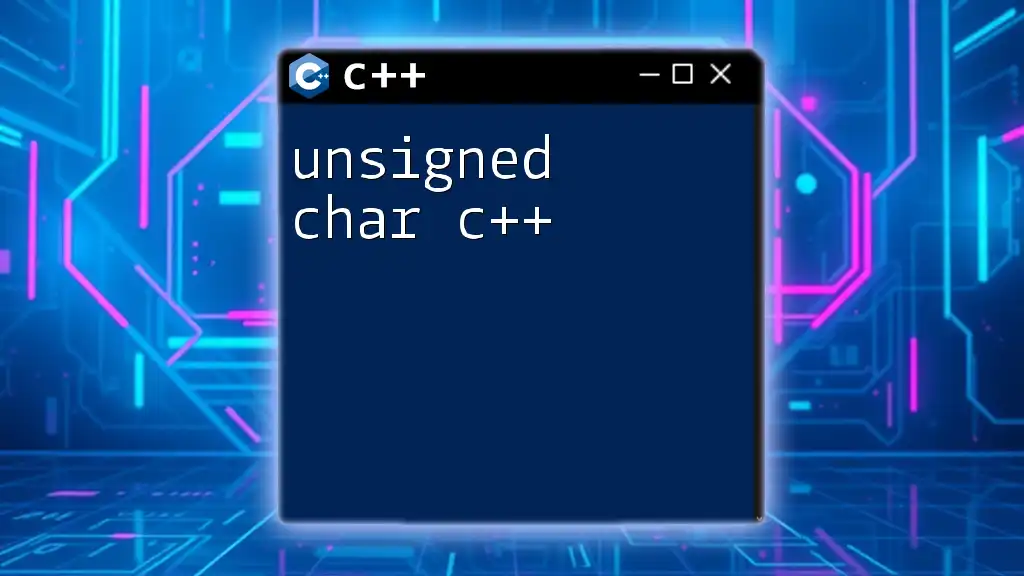
Comparison with Other Integer Types
`int` vs `unsigned int`
The distinction between `int` and `unsigned int` becomes crucial during calculations where negative values are not applicable. For instance, in loops or counting scenarios, using `unsigned int` can help prevent logic errors that arise from attempting to represent negative counts.
Example comparison:
int a = -1; // a can be negative, true for signed integers
unsigned int b = 1; // b must be positive
Other Unsigned Types in C++
C++ provides several other types of unsigned integers, including `unsigned short`, `unsigned long`, and `unsigned long long`, each with varying ranges and memory allocations. It's important to choose the correct type based on the required storage and range:
- `unsigned short`: Typically 16-bits, ranges from 0 to 65,535.
- `unsigned long`: Typically 32-bits on many systems, covering the same range as `unsigned int` in general usage.
- `unsigned long long`: Usually 64-bits, ranges from 0 to 18,446,744,073,709,551,615.
When choosing between these types, always consider the range needed for your application alongside memory constraints.
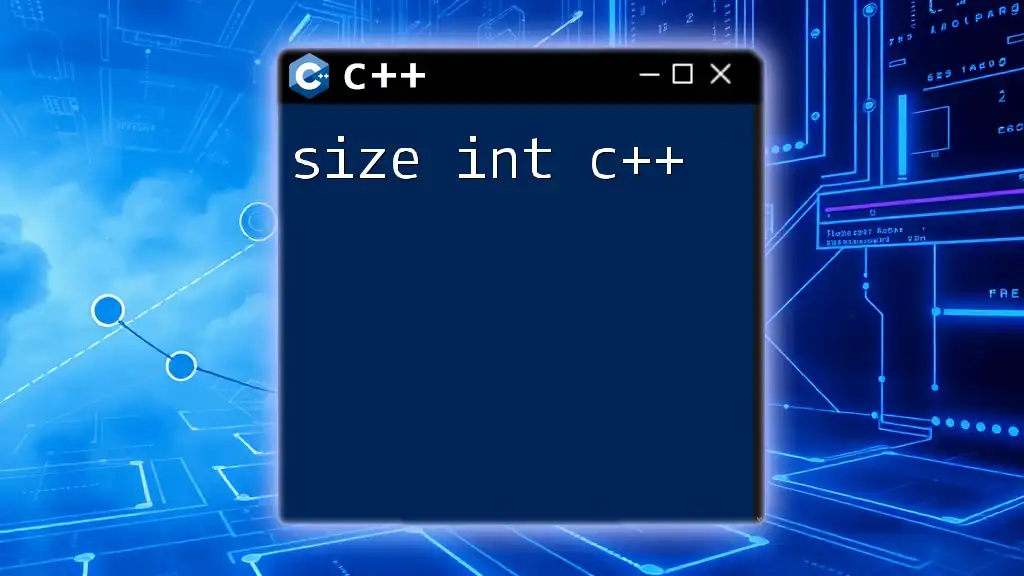
Using `unsigned int` in C++ Programs
Declaring and Initializing `unsigned int`
Declaring an `unsigned int` follows the same syntax as other variable types. Here’s how to declare and initialize it:
unsigned int count = 0;
Arithmetic Operations with `unsigned int`
Performing arithmetic operations with `unsigned int` is straightforward. However, special attention is needed for overflow and underflow conditions:
unsigned int a = 300;
unsigned int b = 200;
unsigned int result = a + b; // result will be 500
Should the result exceed the maximum value (e.g., `4,294,967,295`), it will wrap around to zero due to overflow, thereby creating unexpected results.
Type Casting and Conversion
Type casting allows explicit conversion between different data types, which can be particularly useful when interacting between signed and unsigned types. Here’s an illustration:
int negative = -5;
unsigned int positive = static_cast<unsigned int>(negative); // results in a large positive number
This conversion emphasizes caution, as interpreting negative values in an unsigned context can lead to misleading interpretations.
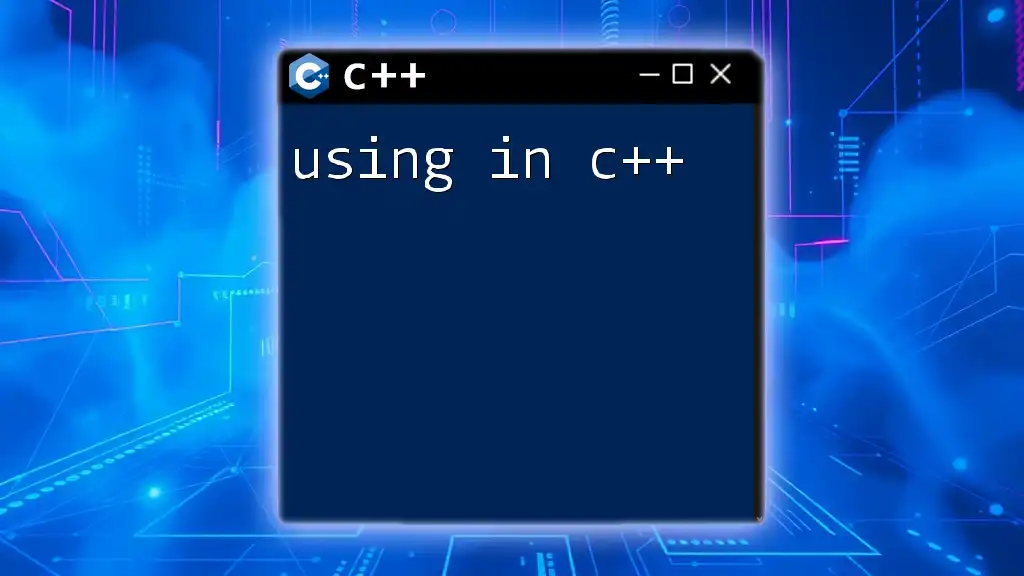
Best Practices for Using `unsigned int`
When to Use `unsigned int`
Utilizing `unsigned int` is advantageous in scenarios where negative values are unnecessary, such as:
- Counting objects.
- Hash tables where indices cannot be negative.
- Network protocols that specify non-negative message identifiers.
Common Mistakes to Avoid
While `unsigned int` is versatile, pitfalls exist:
- Mistakenly mixing with signed types: Always ensure clear awareness of type interactions to avoid logical errors.
- Overflow scenarios: Always check potential overflow from calculations to avoid unexpected behaviors.
By being aware of these mistakes and applying best practices, developers can harness the full potential of `unsigned int` safely.
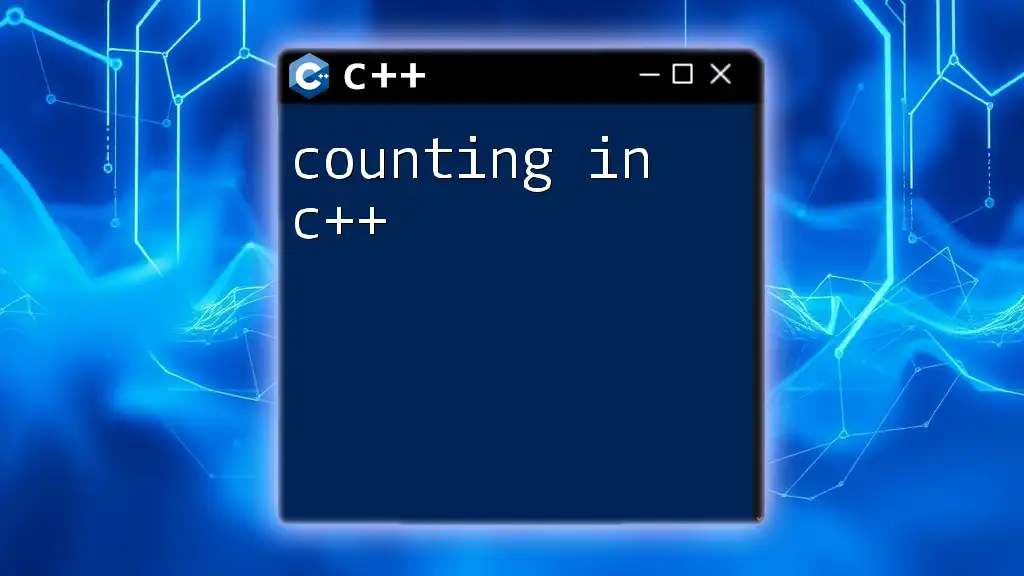
Conclusion
Summary of Key Points
Understanding the characteristics of `unsigned int` is essential for effective C++ programming. Its advantages, such as a larger range and efficiency in non-negative scenarios, make it a powerful tool. However, caution is advised when converting between signed and unsigned integers to avoid pitfalls.
Further Learning Resources
For those looking to deepen their knowledge of C++ and specifically `unsigned int`, a variety of resources are available, including textbooks like "C++ Primer" by Lippman, courses on platforms like Coursera and Udemy, and expansive documentation available on cppreference.com.
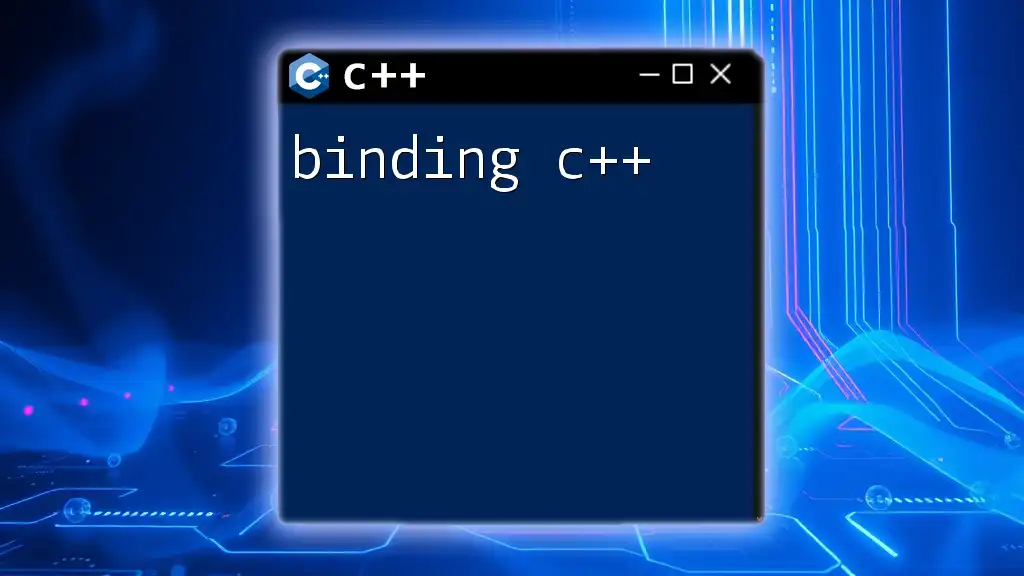
Call to Action
I encourage you to practice using `unsigned int` in your projects and explore how this data type can simplify your coding challenges. Feel free to share experiences or questions regarding the use of `unsigned int` in C++, as engaging with a community can greatly enhance your learning journey.