The `readline` function in C++ is commonly used to read a line of input from the user, typically from the standard input (stdin).
#include <iostream>
#include <string>
int main() {
std::string input;
std::cout << "Enter a line: ";
std::getline(std::cin, input);
std::cout << "You entered: " << input << std::endl;
return 0;
}
What is `readline`?
`readline` is a powerful library that provides a way to read a line of text from the standard input with advanced input handling features. It allows users to edit the input interactively and provides several features such as command history and autocompletion, making it a critical tool for developing command-line applications in C++. Utilizing `readline` can enhance the user experience by making input more intuitive and manageable compared to standard input methods like `std::cin`.
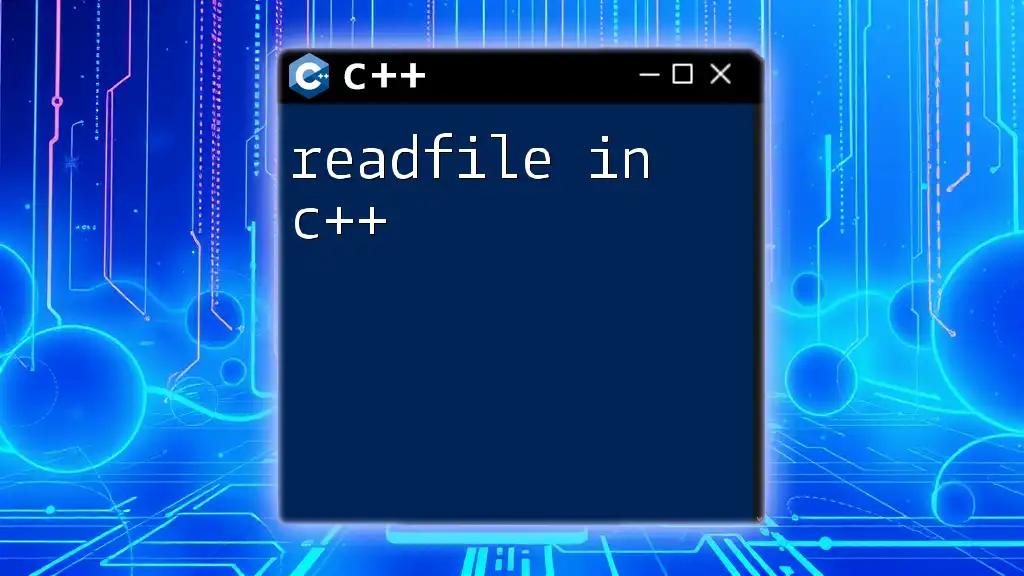
Setting Up `readline` in C++
Before you can start using `readline` in your C++ projects, you need to set it up properly in your development environment. The installation process varies depending on your operating system.
Installation of `readline`
- For Linux: You can easily install the `readline` library using a package manager. For instance, if you're using Ubuntu, you can run the following command:
sudo apt install libreadline-dev
- For macOS: You can install `readline` using Homebrew by executing this command in your terminal:
brew install readline
- Windows: For Windows users, installing `readline` can be done effectively by using Windows Subsystem for Linux (WSL) or other methods that support C++ development tools.
Once installed, you can include the `readline` header in your C++ applications to start using its features.

Basic Usage of `readline`
To start using `readline`, the most basic functionality is to read a line of user input. Below is a simple example demonstrating how to do this:
#include <iostream>
#include <readline/readline.h>
int main() {
char *input = readline("Enter your input: ");
std::cout << "You entered: " << input << std::endl;
free(input);
return 0;
}
In this snippet, `readline` prompts the user for input, displays it, and ensures that dynamically allocated memory is freed after use. This simple functionality highlights how `readline` can make handling user input cleaner and more user-friendly.

Features of `readline`
One of the standout features of `readline` is its ability to provide input editing capabilities. It includes a range of built-in edits and commands that users can utilize, such as:
- Ctrl+A: Move the cursor to the beginning of the line.
- Ctrl+E: Move the cursor to the end of the line.
- Ctrl+K: Delete from the cursor to the end of the line.
These commands allow users to edit their input interactively, creating a better user experience by reducing typing errors.
History Manipulation
`readline` also supports command history, enabling users to recall previous inputs, which is particularly useful for command-line utilities. Below is an example demonstrating how to set up history:
#include <iostream>
#include <readline/readline.h>
#include <readline/history.h>
int main() {
char *input;
while ((input = readline("Input: ")) != nullptr) {
add_history(input); // Adds input to history
std::cout << "You entered: " << input << std::endl;
free(input);
}
return 0;
}
With the `add_history` function, each input is stored and can be recalled using the up and down arrow keys, facilitating quick re-entry of previous commands.
Customizing Prompt Appearance
Another aspect of `readline` is the ability to customize the input prompt. Here is a way to modify the appearance of your prompt using ANSI escape codes:
char *input = readline("\033[1;32mMyCustomPrompt\033[0m> ");
This example changes the prompt to green while giving a clear distinction between the prompt and the user input, enhancing the visual appeal.
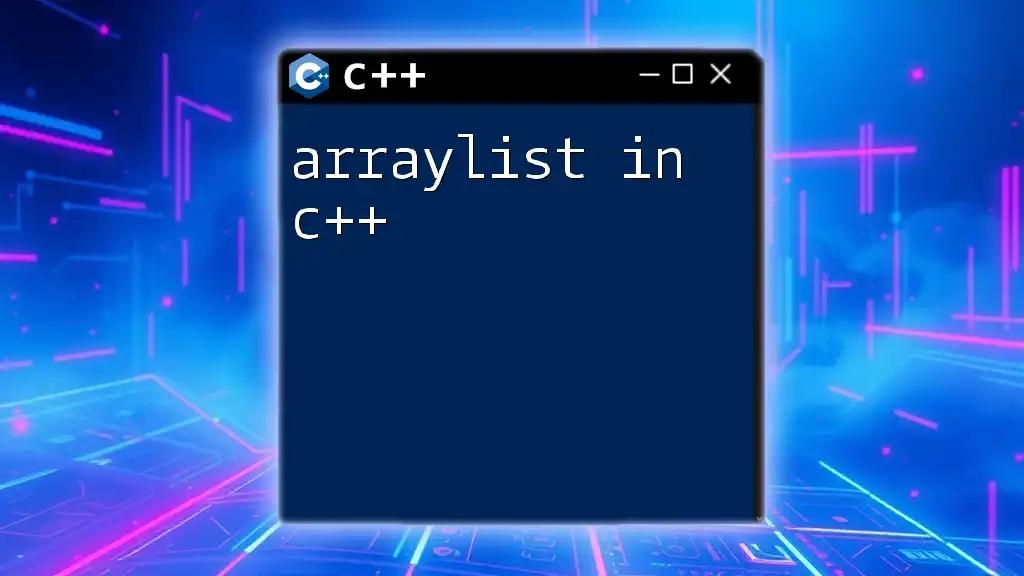
Advanced Features of `readline`
When diving deeper into `readline`, you can explore autocompletion features. Autocompletion can significantly enhance user input by predicting commands or filenames. Below is a brief setup for file name completion:
#include <readline/readline.h>
#include <readline/history.h>
#include <dirent.h>
char **autocomplete(const char *text, int state) {
// Implement file name completion logic here
}
// Setup for readline
Using callback functions, you can define your own logic for autocomplete, which can be particularly useful in applications where commands or file locations are key components.
Using Callback Functions for Extended Input Behaviors
The `readline` library allows the use of callback functions for customizing input behaviors, such as handling specific keystrokes. By creating your own input handling routine, you can respond to specific user actions, providing a richer interaction.

Error Handling with `readline`
Handling errors is crucial when working with input. Common issues can arise, such as null pointer dereferencing if `readline` fails to allocate memory for user input. To mitigate this, always check the output of `readline` before using it. Here's an example of safe error handling:
#include <iostream>
#include <readline/readline.h>
int main() {
char *input = readline("Enter text: ");
if (input) {
std::cout << "You typed: " << input << std::endl;
free(input);
} else {
std::cerr << "Error: Memory allocation failure" << std::endl;
}
return 0;
}
Additionally, always remember to free allocated memory to avoid memory leaks, which could potentially destabilize your application.

FAQs about `readline`
-
What platforms support `readline`?
`readline` is widely supported on Unix-like platforms including Linux and macOS. It can also be utilized in Windows environments through WSL. -
Are there alternatives to `readline` in C++?
While `readline` is popular, alternatives like `linenoise` or `ncurses` exist, each with unique features that might be better suited for specific applications. -
How does `readline` compare to other libraries?
Compared to standard input via `std::cin`, `readline` offers a more robust and user-friendly experience by integrating features like autocompletion and command history.
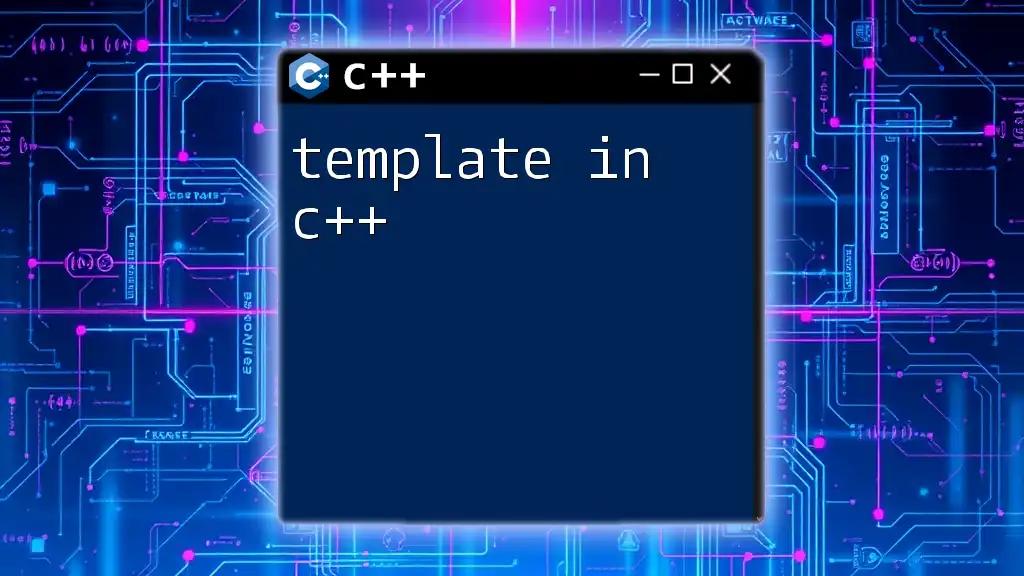
Conclusion
Using `readline in C++` can revolutionize how user input is handled in command-line applications. Its advanced functionality not only simplifies input processing but also enhances the user's experience significantly. As you implement `readline`, consider these features to improve interactivity in your projects. Embrace the potential of `readline`, and you’ll create applications that are not only effective but also user-friendly.
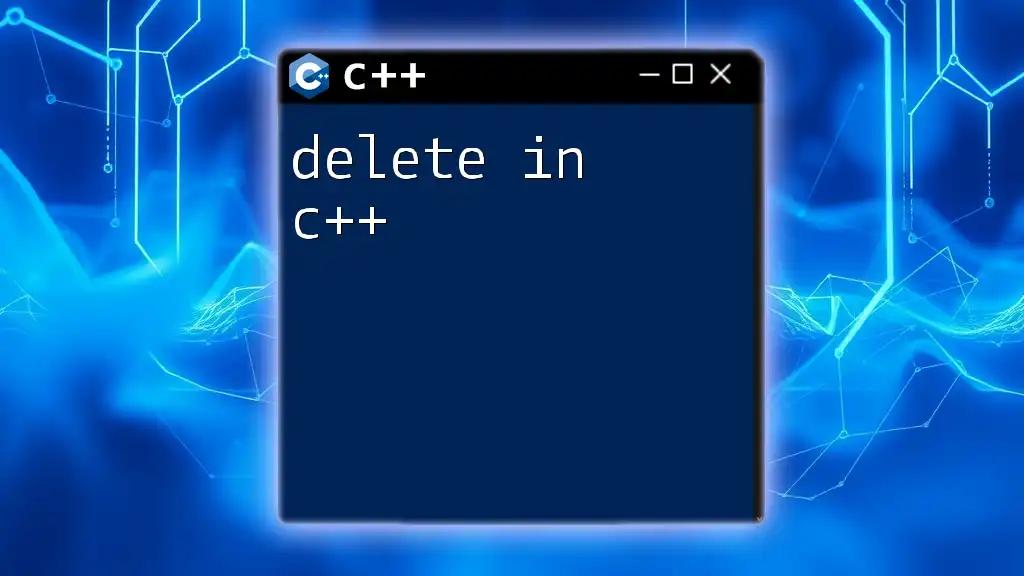
Additional Resources
As you continue to explore the capabilities of `readline`, be sure to refer to its [official documentation](https://www.gnu.org/software/readline/manual/) and other advanced topics in C++ input handling to further enhance your understanding and application of this valuable library.