In C++, `endl` is a manipulator used to insert a newline character into the output stream and flush the stream, ensuring that all output is displayed immediately.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // Output with newline and flush
return 0;
}
What is `endl`?
`endl` is a manipulator in C++ that is commonly used for outputting a newline character and flushing the output buffer simultaneously. It is part of the `<iostream>` library, which allows for input and output operations in C++. Understanding `endl` is essential for effective console output formatting, especially when clarity and readability of output matter.
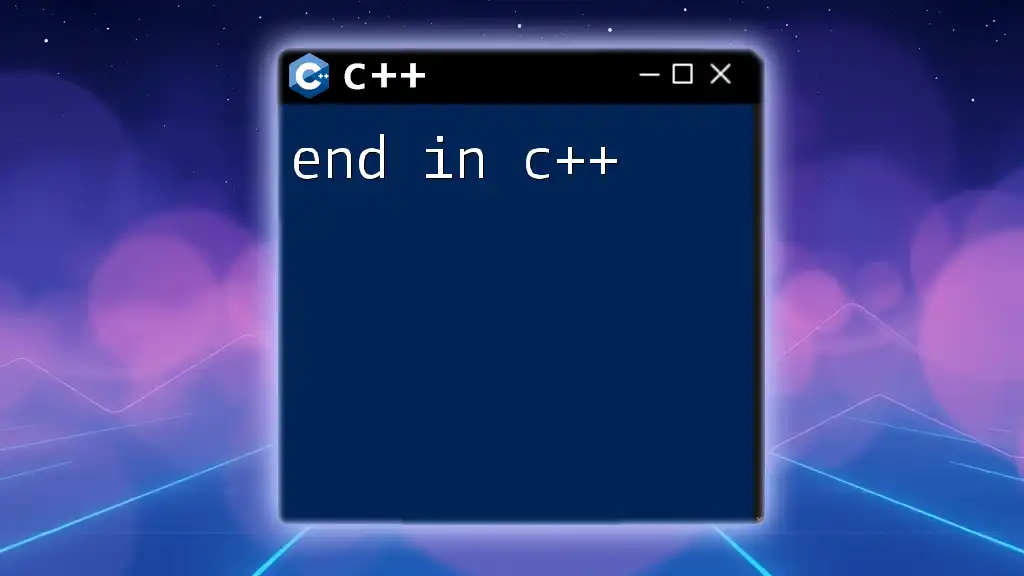
Syntax of `endl`
The syntax for using `endl` is straightforward. It is used in conjunction with the output stream, typically `cout`, as shown below:
std::cout << std::endl;
This syntax illustrates how `endl` fits seamlessly into the C++ I/O system, acting as a connector that enhances output operations.
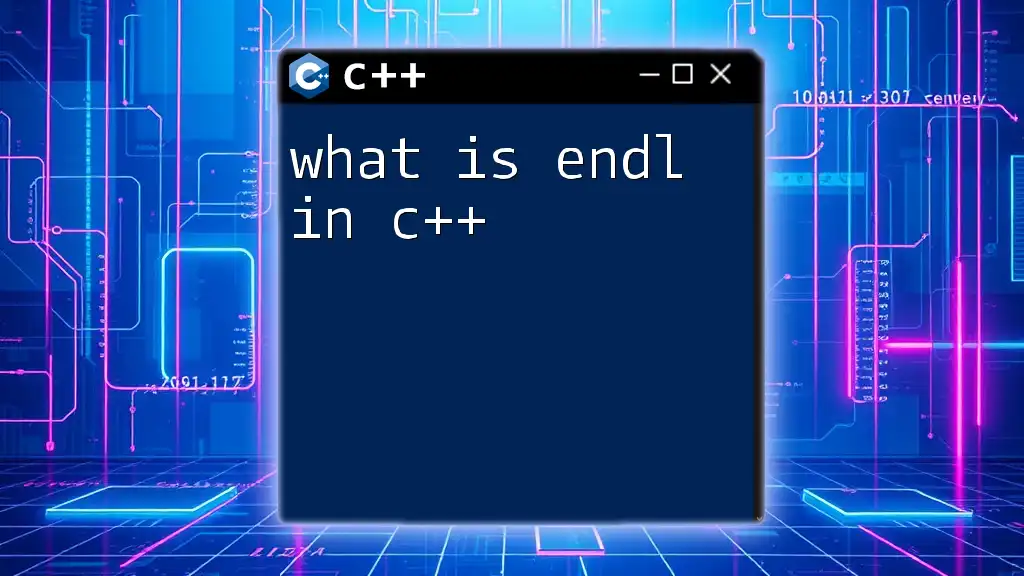
How `endl` Works
Using `endl` effectively means understanding its functionality. Primarily, `endl` outputs a newline character, which moves the cursor down to the next line. Additionally, it flushes the output buffer, ensuring that all data is immediately displayed.
The Relationship Between `endl` and the Output Buffer
The output buffer holds data meant for output but does not necessarily display it right away. When `endl` is used, it triggers a flush of this buffer, ensuring timely visibility of the output. This mechanism is particularly crucial in scenarios where real-time data monitoring is involved, such as in logs or user interactions.
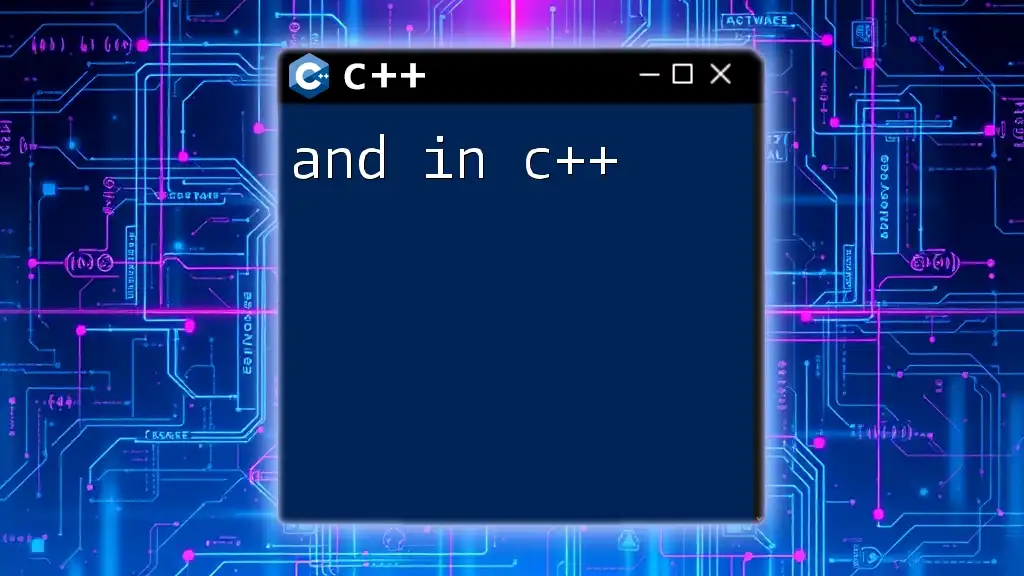
Basic Usage Examples
To see `endl` in action, here’s a basic example:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this snippet, `endl` moves the output to the next line after printing "Hello, World!". This simple usage demonstrates the core ability of `endl` to control line endings in a C++ program.
Combining with Other Output Statements
`endl` can be effectively combined with multiple output statements. For instance:
cout << "This is a line." << endl << "This is another line." << endl;
This code snippet illustrates how you can concatenate different `cout` statements with `endl` effectively, ensuring each statement appears on a new line.
Styling Console Output
Using `endl` can enhance the readability of console applications. For example:
cout << "First Statement" << endl;
cout << "Second Statement" << endl << endl; // Adding extra space
Here, the additional `endl` creates a blank line between the two statements, making the output aesthetically pleasing and easier to read.
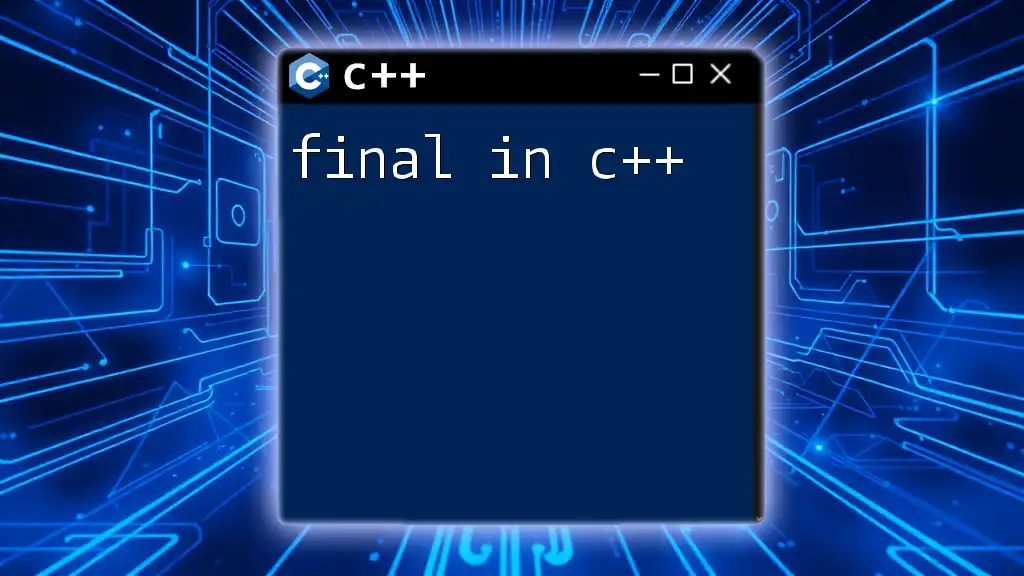
Performance Considerations
While `endl` has its uses, it’s essential to consider performance implications, especially in loops or frequently executed code blocks.
Frequent Use of `endl` vs. `\n`
One critical difference between `endl` and the newline character `\n` is performance. Every time `endl` is invoked, it flushes the output buffer, which can lead to a performance bottleneck. Conversely, `\n` simply moves to the next line without flushing the buffer:
for (int i = 0; i < 5; i++) {
cout << "Line " << i + 1 << endl; // Slower
}
for (int i = 0; i < 5; i++) {
cout << "Line " << i + 1 << "\n"; // Faster
}
The above loops illustrate that using `\n` is more efficient when multiple lines are printed in succession.
When to Use Which
As a rule of thumb:
- Use `endl` when you need immediate feedback, such as during debugging or logging scenarios.
- Use `\n` for bulk output where speed is paramount.
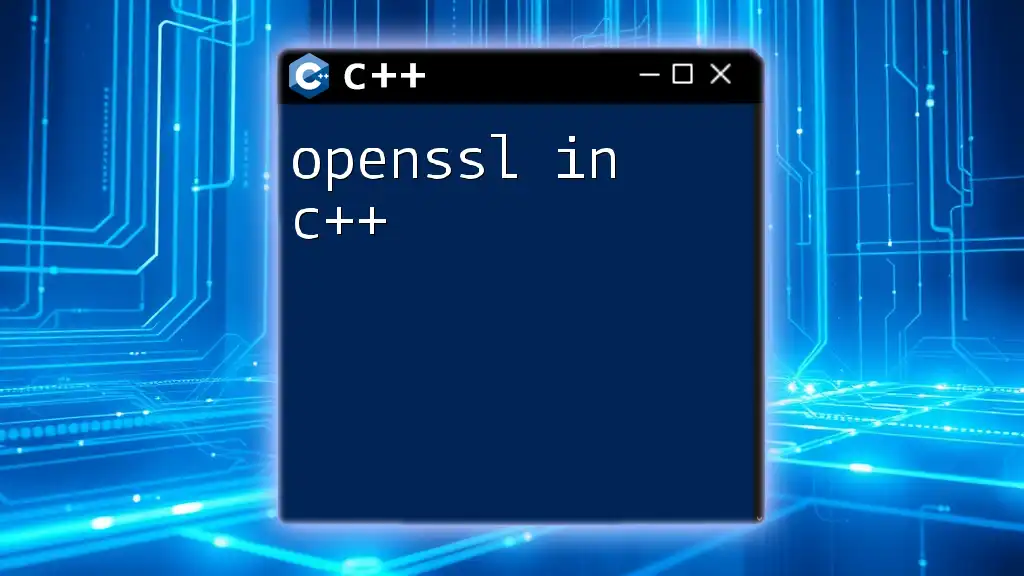
Alternatives to `endl`
In addition to `endl`, C++ provides other ways to flush output.
Other Flushing Mechanisms
One alternative is `std::flush`, which allows you to control when to flush the output buffer without adding a line break. For example:
cout << "Flushing output..." << flush;
This line outputs the text and immediately flushes the buffer without moving to a new line.
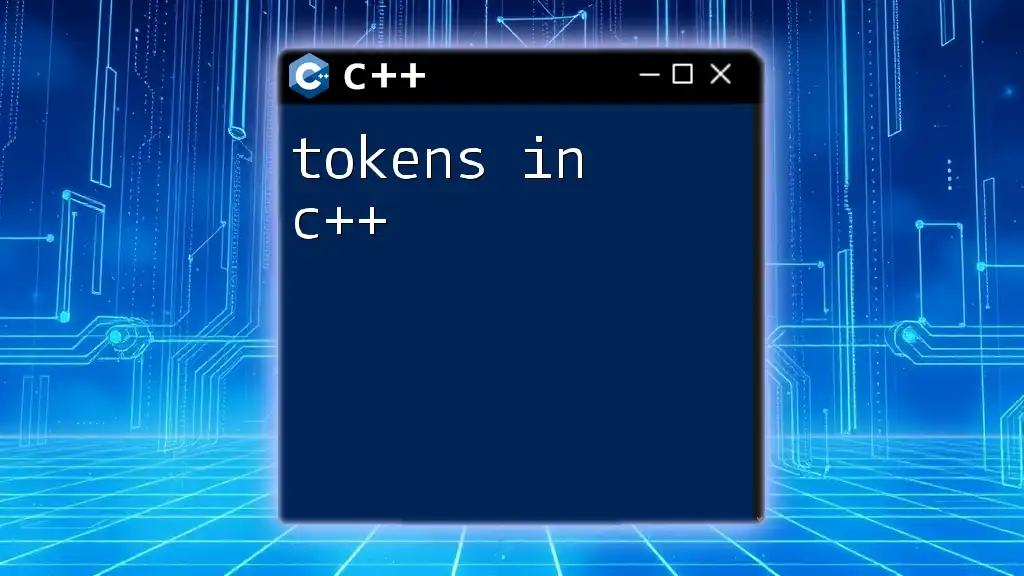
Effective Usage Strategies
To leverage `endl` wisely in your programming practices, consider these strategies:
- Use `endl` for clarity in output, especially in console applications where line breaks add meaning.
- Optimize performance by using `\n` in tight loops or when printing many lines.
Common Pitfalls to Avoid
One of the most frequent mistakes among beginners is overusing `endl`, particularly in performance-sensitive contexts. Always assess whether each `endl` instance is necessary for ensuring that output gives you the readability or control you require.
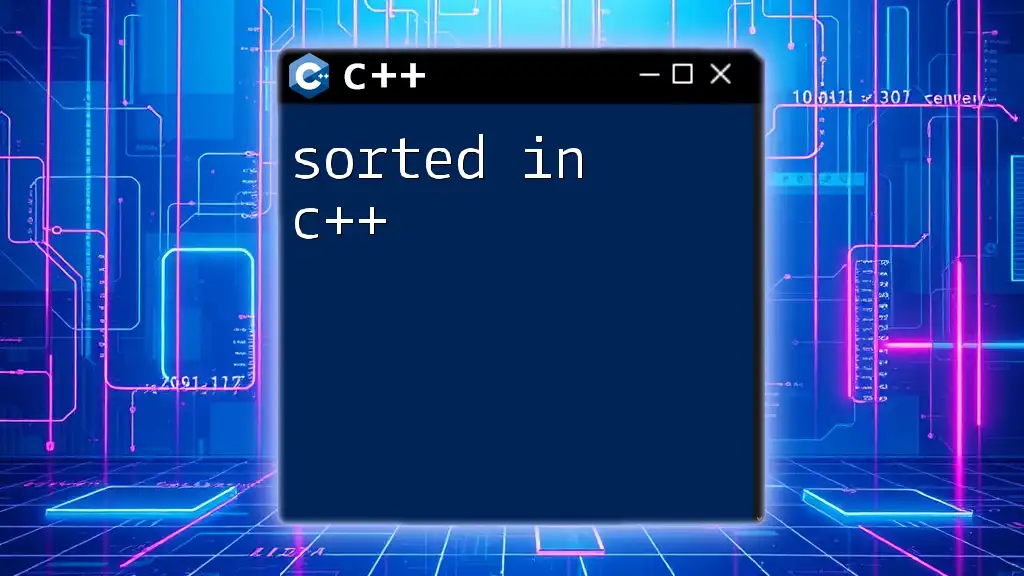
Summary of Key Points
In summary, `endl` is a powerful tool for managing output in C++. It not only adds a line break but also ensures timely flushing of the output buffer. While it's essential for scenarios requiring immediate feedback, it’s crucial to consider performance implications in bulk outputs. By understanding its functionality and practicing effective usage strategies, you can harness the full potential of `endl` in your C++ programming.
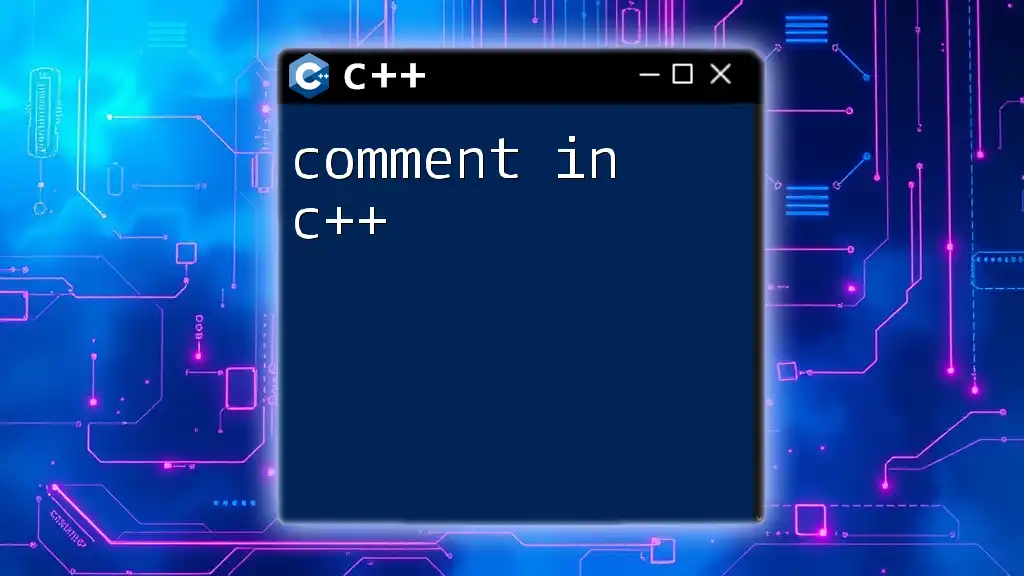
Further Reading and Learning
To deepen your understanding of `endl` and C++ in general, consider exploring the official C++ documentation and tapping into additional resources, such as notable programming books and tutorials that cover C++ fundamentals. Experimenting with different output methods will further solidify your command of output in C++.