In C++, an argument is a value that is passed to a function when it is invoked, allowing the function to operate on that data. Here's a simple example:
#include <iostream>
using namespace std;
void greet(string name) {
cout << "Hello, " << name << "!" << endl;
}
int main() {
greet("Alice"); // "Alice" is the argument passed to the greet function.
return 0;
}
What is an Argument in C++?
An argument is a value that you pass to a function when you call it. Understanding the distinction between arguments and parameters is crucial for mastering functions in C++. A parameter is a variable in the function definition that receives a value. In contrast, an argument is the actual value you pass to that parameter when you invoke the function.
Consider this example to clarify the difference:
void exampleFunction(int parameter) { /* ... */ } // parameter
exampleFunction(5); // argument
In this case, `5` is the argument being passed to the function `exampleFunction()`, while `parameter` is a placeholder in the function definition.
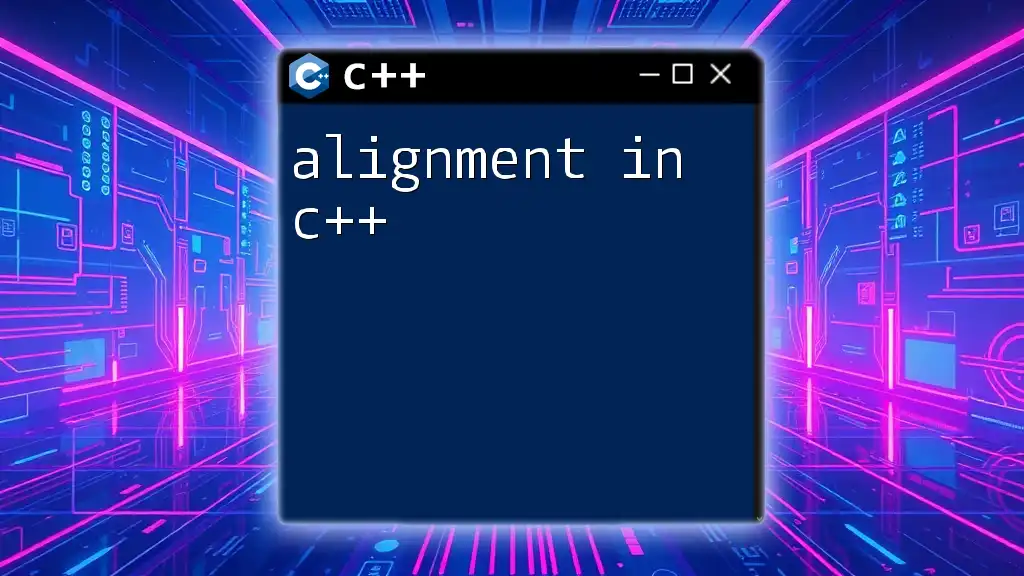
Types of Arguments in C++
Positional Arguments
Positional arguments are related directly to the sequence in which they are defined in the function. When you call a function, you must provide arguments in the same order as the parameters in the function definition.
For instance:
void display(int a, int b) {
std::cout << "First: " << a << ", Second: " << b << std::endl;
}
display(10, 20); // 10 and 20 are positional arguments
In the above example, `10` is passed to `a`, and `20` is passed to `b`. The order matters, and any mistake can lead to incorrect behavior.
Default Arguments
Default arguments allow you to declare a default value for a function parameter. If an argument for that parameter isn’t supplied during the function call, the default value is used.
This can streamline your code and make your functions more versatile:
void showMessage(std::string message = "Hello, World!") {
std::cout << message << std::endl;
}
showMessage(); // Uses default argument
showMessage("Custom message!"); // Uses custom argument
In this example, if you call `showMessage()` without any parameters, "Hello, World!" is printed. However, you can override this default by providing a custom message.
Reference Arguments
Reference arguments allow you to work directly with the original variable that you pass to the function. This means modifications to the reference will affect the actual variable, as opposed to a copy.
Using reference arguments can enhance performance, especially with large data types:
void updateValue(int &ref) {
ref = 100; // Modifies the original variable
}
int num = 10;
updateValue(num);
// num is now 100
In the above code, `num` is modified to `100` because `ref` refers directly to `num`.
Constant Arguments
Using constant arguments (`const`) prevents the function from altering the value of passed parameters. This is a useful practice when you want to ensure that a function cannot modify its input.
void displayValue(const int value) {
std::cout << "Value: " << value << std::endl;
// value cannot be modified here
}
In this scenario, the function `displayValue` receives an integer, but any attempts to modify it within the function body will result in a compilation error. This safeguard can help protect against accidental changes in your code.
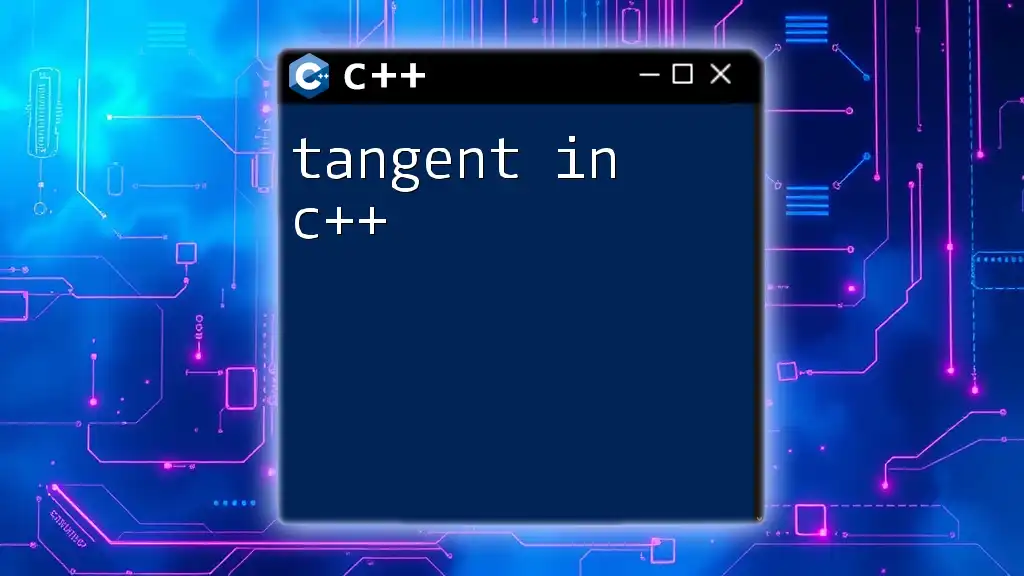
Varied Argument Lists
Variadic Functions
C++ also allows you to create variadic functions, which can take a variable number of arguments. This feature is useful when you don’t know how many parameters might be passed in.
The `stdarg.h` library facilitates this through macros like `va_list`, `va_start`, and `va_end`:
#include <iostream>
#include <cstdarg>
void printNumbers(int count, ...) {
va_list args;
va_start(args, count);
for (int i = 0; i < count; i++) {
std::cout << va_arg(args, int) << " ";
}
va_end(args);
}
printNumbers(3, 1, 2, 3); // Outputs: 1 2 3
Here, `printNumbers()` can accept any count of integers, which makes it very flexible for various situations.
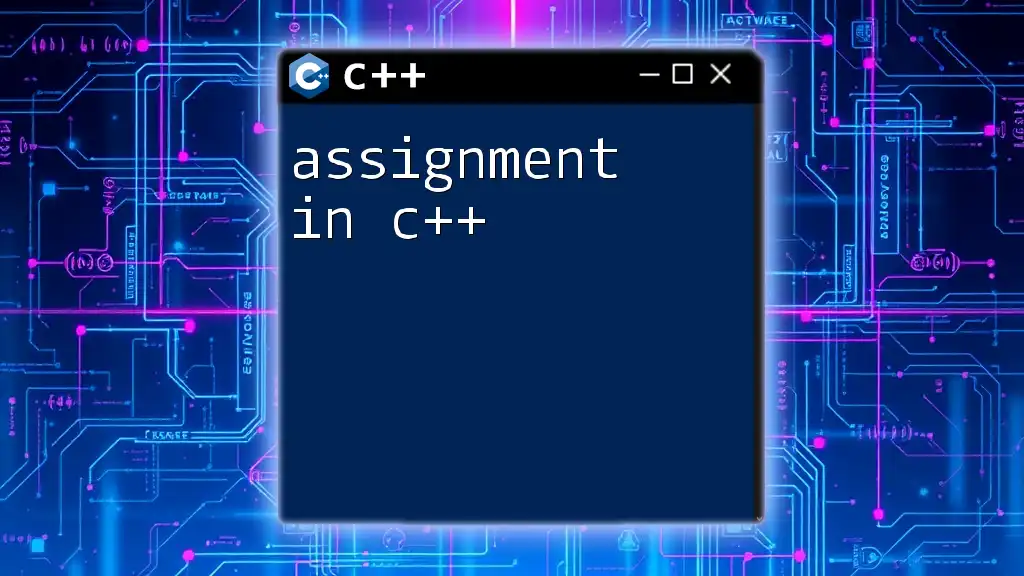
Using Arguments with Function Overloading
Function overloading allows you to create multiple functions with the same name but different types or numbers of parameters. This use of arguments gives you great flexibility in your code design.
void process(int x) {
std::cout << "Processing int: " << x << std::endl;
}
void process(double y) {
std::cout << "Processing double: " << y << std::endl;
}
process(5); // Calls process(int)
process(5.0); // Calls process(double)
In this example, even though both functions are named `process`, C++ differentiates them based on the type of argument passed.
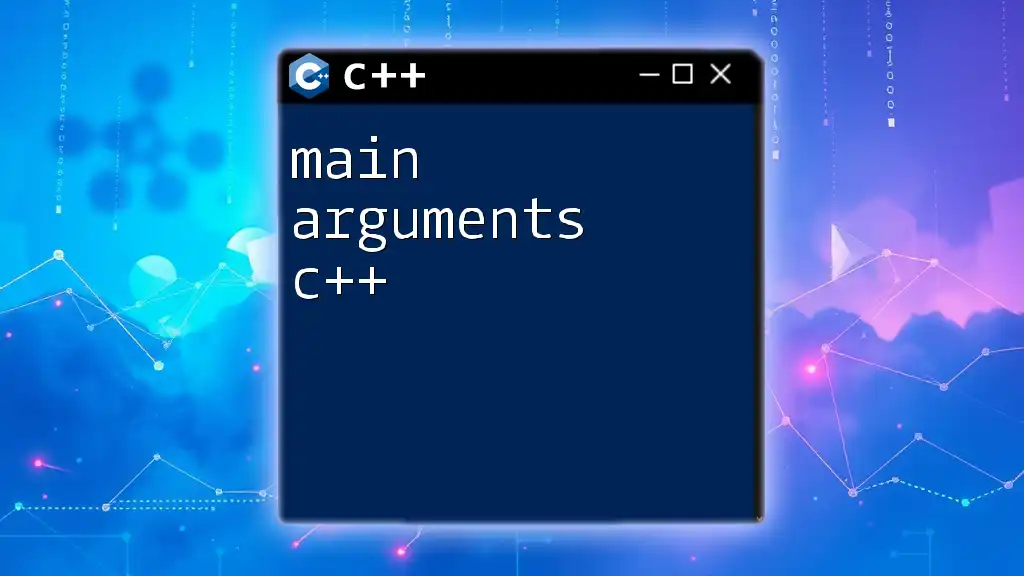
Argument Passing Mechanisms
Pass by Value
When arguments are passed by value, a copy of the original variable is made. This means any changes made within the function do not affect the original variable.
void increment(int n) {
n++; // Only modifies the copy
}
int num = 5;
increment(num);
// num is still 5
This mechanism is straightforward but can be less efficient with large data structures due to the overhead of copying.
Pass by Reference
Conversely, when you pass variables by reference, the function works with the actual variable rather than a copy. Any modifications made to the parameter affect the original variable.
void increment(int &n) {
n++; // Modifies the original variable
}
int num = 5;
increment(num);
// num is now 6
Utilizing pass by reference can significantly improve performance when dealing with large objects, as there is no need to create copies.
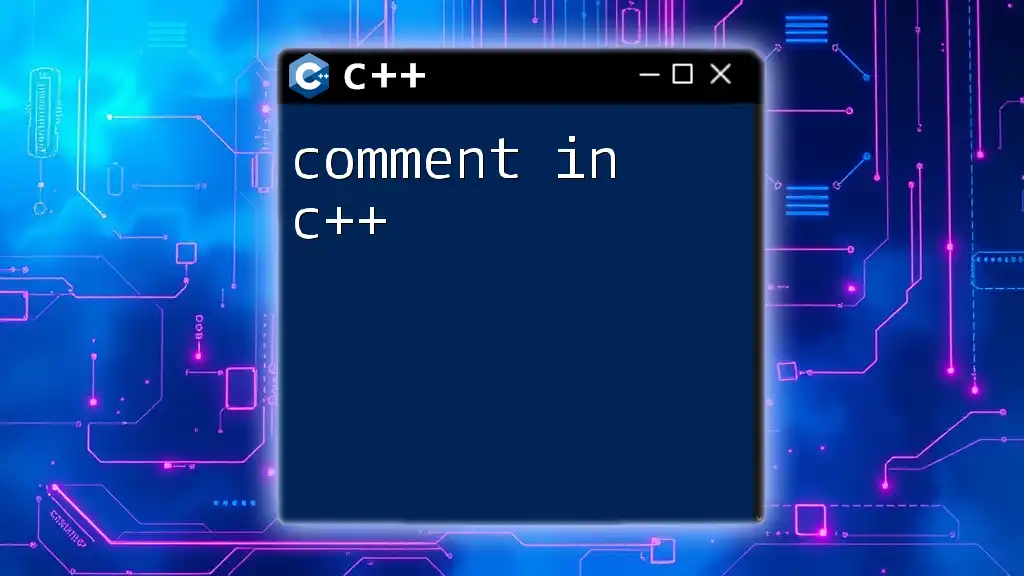
Best Practices for Using Arguments in C++
When working with arguments in C++, consider these best practices:
- Choose Between Value and Reference: Use pass by value when dealing with small data types, while pass by reference is preferable for larger structures.
- Utilize Default Arguments Wisely: Default arguments can make functions more flexible but can also lead to ambiguity in function calls. Use them judiciously.
- Document Parameters Thoroughly: Clear documentation helps others (and future you) understand the intended use and constraints for each parameter.
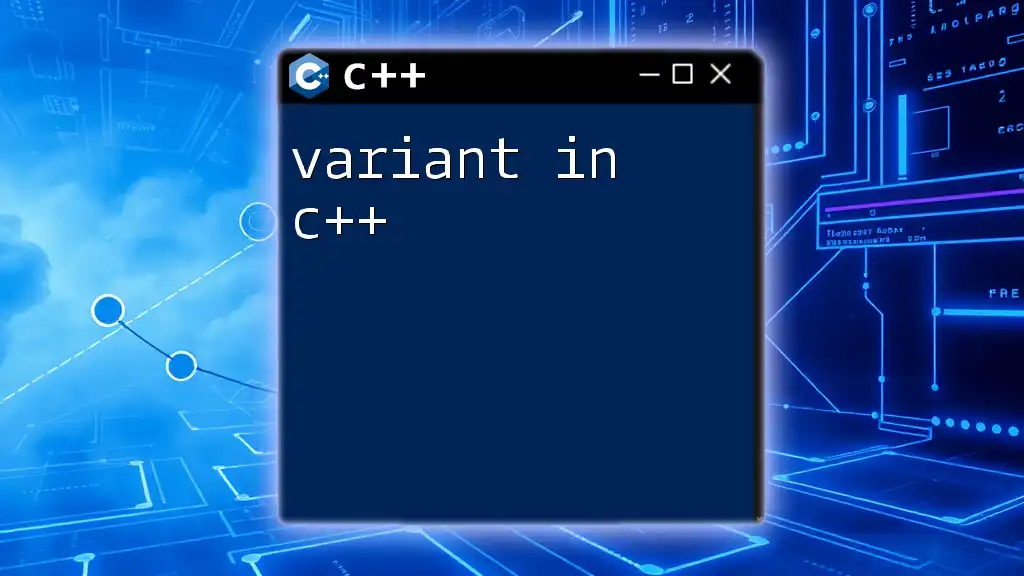
Conclusion
Understanding arguments in C++ is foundational for programming effectively. When you grasp the various types of arguments and how to use them correctly, you unlock the potential for cleaner, more efficient code. Whether you’re working with positional arguments, default values, or more advanced concepts like variadic and reference arguments, mastering these skills will elevate your C++ programming capabilities.
Feel encouraged to write sample functions and experiment with different types of arguments to reinforce your learning!