The `isalnum` function in C++ checks if a given character is alphanumeric (i.e., a letter or a digit) and returns a non-zero value if true; otherwise, it returns zero.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <cctype>
int main() {
char c = 'A';
if (isalnum(c)) {
std::cout << c << " is an alphanumeric character." << std::endl;
} else {
std::cout << c << " is not an alphanumeric character." << std::endl;
}
return 0;
}
What is `isalnum`?
`isalnum` is a function in C++ that helps classify characters based on whether they are alphanumeric or not. Alphanumeric characters include all uppercase and lowercase letters (A-Z, a-z) as well as digits (0-9). This function is particularly useful for input validation and ensures that the data processed in a program meets certain criteria.
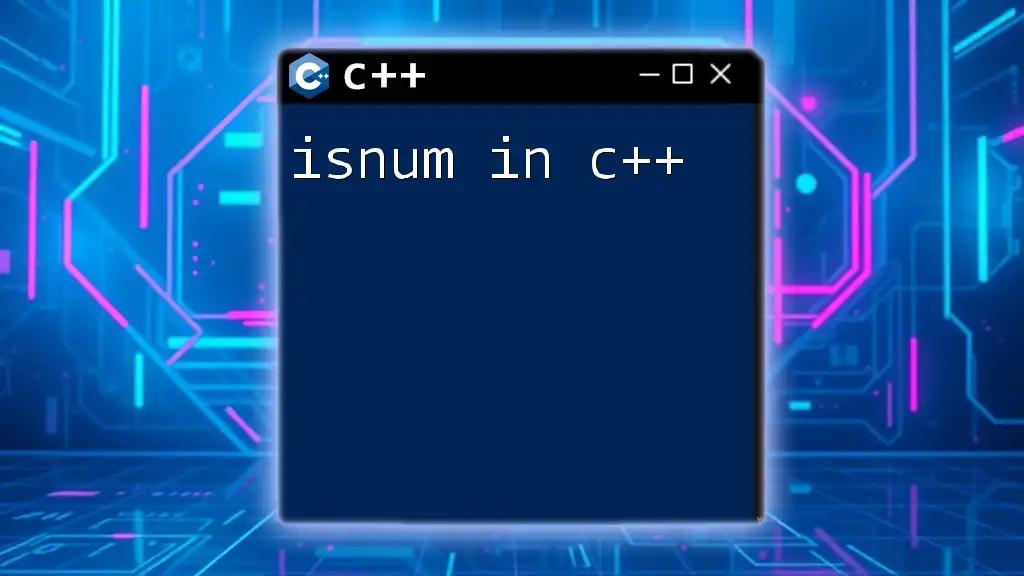
Including the Required Header
To use `isalnum`, you must include the `<cctype>` header file at the beginning of your program. This header provides various character classification functions, such as `isalpha`, `isdigit`, and more. Here's how you can include it in your code:
#include <cctype>
Including this header allows you to utilize not only `isalnum` but also other useful functions that can improve your character handling capabilities in C++.

Syntax of `isalnum`
The syntax for the `isalnum` function is straightforward:
int isalnum(int ch);
Parameter and Return Value
- Parameter: `ch` is the character you want to check.
- Return Value: The function returns a non-zero value if `ch` is an alphanumeric character, and zero otherwise. This return value is often used in conditional statements to decide how to handle characters based on their classification.

Understanding Alphanumeric Characters
Alphanumeric characters consist of:
- A-Z: All uppercase letters
- a-z: All lowercase letters
- 0-9: All digits from zero to nine
Knowing what `isalnum` is looking for is crucial for effective input handling. For example, the character `!` is a special symbol and would not be considered alphanumeric. Understanding this distinction helps in forming robust validation checks in your programs.

How to Use `isalnum` in C++
Basic Example
Using `isalnum` is simple, and it can be demonstrated with a basic example. Here’s a snippet that checks if a specific character is alphanumeric:
#include <iostream>
#include <cctype>
int main() {
char c = '8';
if (isalnum(c)) {
std::cout << c << " is an alphanumeric character." << std::endl;
} else {
std::cout << c << " is not an alphanumeric character." << std::endl;
}
return 0;
}
In this code, we check the character `c`, which holds the value '8'. The output will confirm that '8' is indeed an alphanumeric character.
Checking Characters in a String
In real-world applications, you often need to check multiple characters, typically within a string. For that, you can iterate through each character of the string, as shown in the following example:
#include <iostream>
#include <cctype>
#include <string>
int main() {
std::string input = "Hello123!";
for (char c : input) {
if (isalnum(c)) {
std::cout << c << " is alphanumeric." << std::endl;
} else {
std::cout << c << " is not alphanumeric." << std::endl;
}
}
return 0;
}
In this snippet, the program will check each character in the string `input` and correctly identify each character as either alphanumeric or not. The output would show which characters fall into which category, providing useful feedback for validation purposes.
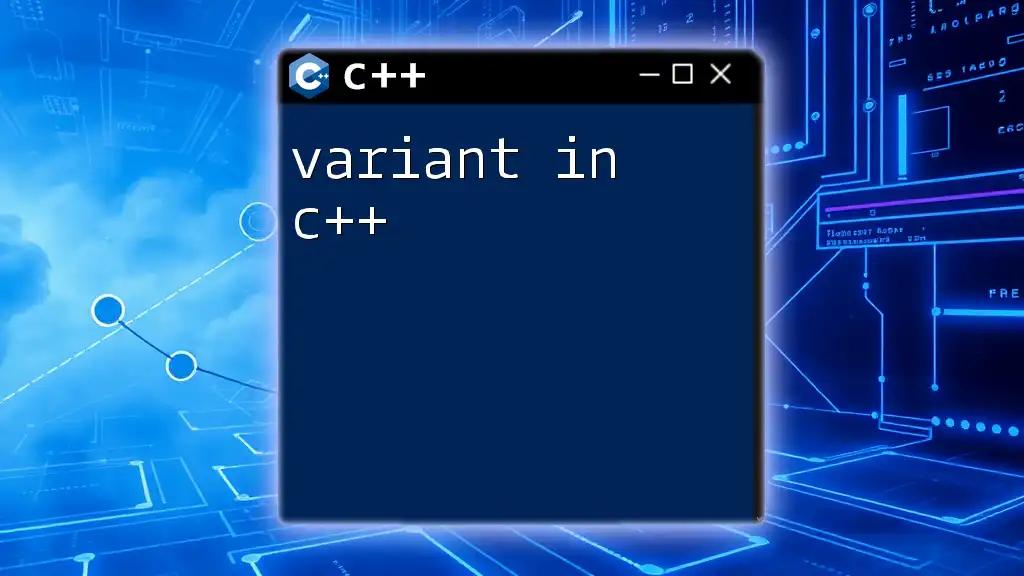
Common Use Cases for `isalnum`
Input Validation
Input validation is crucial in many applications, particularly when dealing with user credentials, such as usernames and passwords. You could use `isalnum` to ensure that only valid characters are included. For example, you can check to confirm that a username contains only alphanumeric characters, rejecting any names that contain symbols or spaces, which may be invalid.
Data Processing
When working with data, especially textual data extracted from files or user input, using `isalnum` enables you to clean and validate the data effectively. For instance, when preparing a dataset for analysis, you might want to eliminate any non-alphanumeric characters to ensure the data's integrity.
Consider a scenario where you need to extract valid alphanumeric characters from a mixed string, facilitating easier manipulation or analysis of clean data.

Limitations of `isalnum`
While `isalnum` is handy, it does come with limitations.
-
Locale Considerations: By default, `isalnum` checks only ASCII characters. This may not be sufficient when dealing with internationalization, where characters from various languages may be expected.
-
Unicode Concerns: If your application requires support for Unicode or wide characters, the regular `isalnum` may not function as expected for these characters.
For broader applications, consider using alternative functions or creating a more advanced validation mechanism to cater to a wider range of character sets.
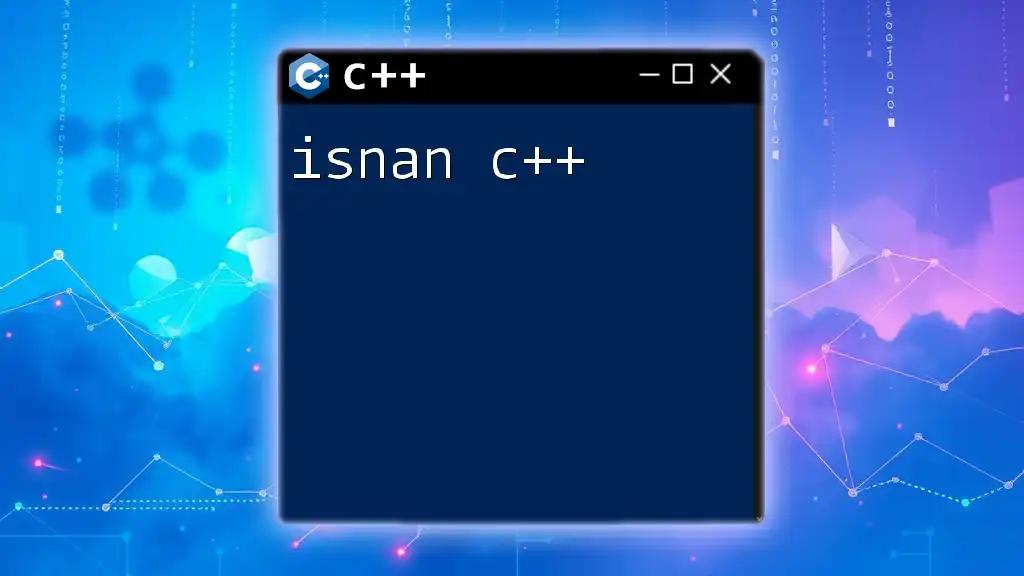
Alternative Functions
In addition to `isalnum`, there are several related functions that might be useful, including:
- `isalpha`: Checks if a character is an alphabetic character (A-Z, a-z).
- `isdigit`: Determines if a character is a numeric digit (0-9).
- Utilize `isalpha` and `isdigit` together for specific checks when necessary.
These functions provide more specific classification, allowing for extensive character handling based on your needs.

Conclusion
In summary, using `isalnum` in C++ is fundamental for character classification, particularly when validating user input and processing data. By ensuring that characters fall into the alphanumeric category, you can create applications that are more robust and user-friendly. As you continue to develop your C++ skills, experimenting with `isalnum` and similar functions will enhance your programming capabilities.

FAQs
What does `isalnum` return for special characters?
`isalnum` will return zero for any special characters, symbols, or whitespace, indicating that they are not alphanumeric.
Can `isalnum` be used with wide characters?
For working with wide characters, consider using `iswalnum`, which performs similar checks with support for wide character sets.
Performance considerations when using `isalnum`
When using `isalnum` in loops, particularly with large datasets, aim for efficiency. Consider optimizing your checks or employing pre-processing methods to ensure performance remains high, especially in computationally intensive applications.
Call to Action
Now that you have a solid understanding of `isalnum in C++`, I encourage you to incorporate it into your projects. Explore additional documentation and resources to expand your knowledge of character processing in C++, and share your findings with the community!