The Least Common Multiple (LCM) of two integers in C++ can be calculated using the formula: `LCM(a, b) = abs(a * b) / GCD(a, b)`, where GCD is the Greatest Common Divisor.
Here's a code snippet demonstrating how to find the LCM in C++:
#include <iostream>
using namespace std;
// Function to compute GCD
int gcd(int a, int b) {
return b == 0 ? a : gcd(b, a % b);
}
// Function to compute LCM
int lcm(int a, int b) {
return abs(a * b) / gcd(a, b);
}
int main() {
int a = 12, b = 18;
cout << "LCM of " << a << " and " << b << " is " << lcm(a, b) << endl;
return 0;
}
Understanding LCM (Least Common Multiple)
What is LCM?
LCM, or the Least Common Multiple, is the smallest multiple that is evenly divisible by two or more numbers. For example, the LCM of 3 and 4 is 12 because it is the smallest number that both 3 and 4 can divide without leaving a remainder. Understanding LCM is crucial in various mathematical applications and programming algorithms.
When to Use LCM
LCM is often used in problems that involve finding common denominators, synchronization of cyclical events, and in various algorithms where multiple factors need to be considered together. It also has a close relationship with the GCD (Greatest Common Divisor), which can be utilized for its computation.
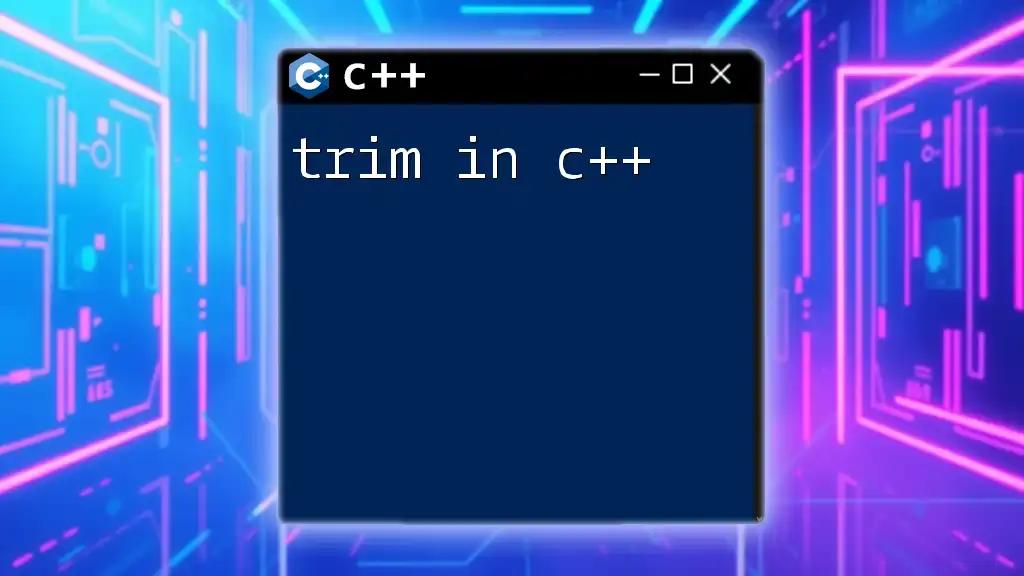
Calculating LCM in C++
Mathematical Formula for LCM
To compute the LCM of two numbers effectively, we can use the relationship between LCM and GCD: LCM(a, b) = (a * b) / GCD(a, b). This formula lets us calculate the LCM based on the GCD, optimizing the process.
Implementing GCD in C++
Before calculating LCM, we need a function to determine the GCD. The GCD is essential as it simplifies the computation of LCM. Here’s a simple implementation of the GCD using the Euclidean algorithm:
Example Code for GCD
int gcd(int a, int b) {
while(b != 0) {
int temp = b;
b = a % b;
a = temp;
}
return a;
}
In this code, we repeatedly apply the remainder operation until the second number (`b`) becomes zero, at which point the first number (`a`) contains the GCD.
LCM Function in C++
With the GCD function in place, we can define our LCM function. Here’s how to implement it:
Example Code for LCM
int lcm(int a, int b) {
return (a * b) / gcd(a, b);
}
This implementation uses the previously defined GCD function to derive the LCM efficiently.

Advanced LCM Calculations
LCM of Multiple Numbers
To extend the LCM computation for more than two numbers, we can loop through an array of integers or use recursion. The idea is to keep calculating the LCM pairwise.
Example Code for LCM of Multiple Numbers
int lcmMultiple(int arr[], int n) {
int lcmValue = arr[0];
for (int i = 1; i < n; i++) {
lcmValue = lcm(lcmValue, arr[i]);
}
return lcmValue;
}
In this function, `lcmMultiple` takes an array of integers and computes the LCM by iteratively applying the LCM function.
LCM Using STL
With the introduction of C++17, the Standard Template Library (STL) now provides the `std::lcm` function, making LCM calculations more straightforward. This function handles the calculations internally, providing both clarity and efficiency.
Example Code for STL LCM
#include <numeric> // For std::lcm
int main() {
int a = 15, b = 20;
int result = std::lcm(a, b);
std::cout << "LCM of " << a << " and " << b << " is " << result << std::endl;
}
This snippet demonstrates how to use the STL's built-in function to compute the LCM directly.

Optimizing LCM in C++
Performance Considerations
When working on LCM calculations, especially with larger integers or multiple numbers, it is important to consider the time complexity. The use of GCD significantly improves the efficiency over a direct approach of finding multiples.
Handling Edge Cases
Programming robust logic requires special consideration of edge cases. Ensure to handle scenarios like:
- Zero: The LCM of any number with zero is typically defined as zero.
- Negative Numbers: Generally, LCM is defined for non-negative integers. Handling such values requires adequate input validation.
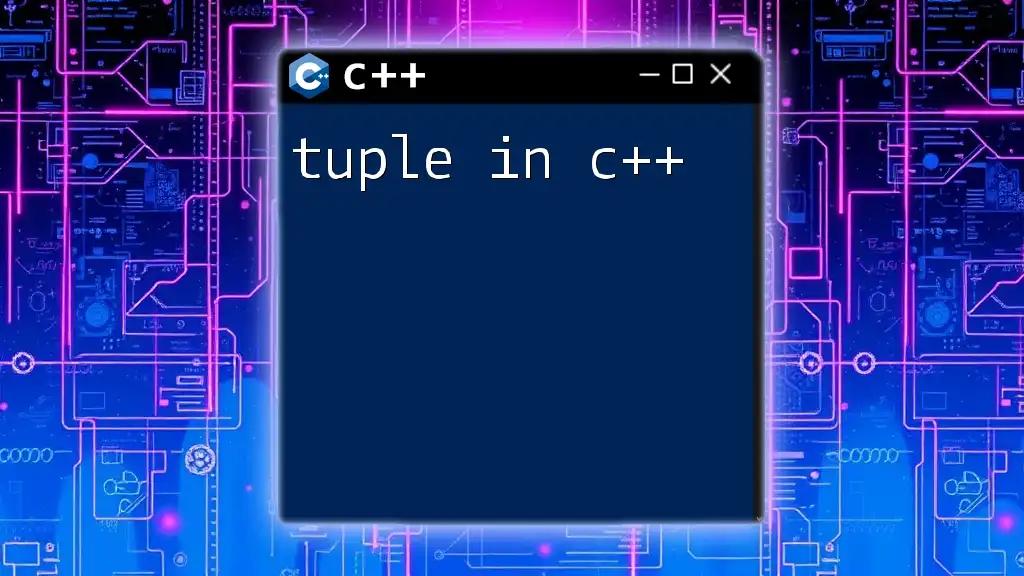
Practical Applications of LCM
Real-world Examples of LCM Use
- Scheduling Problems: LCM is useful for determining when repeated events will coincide, such as the timing of buses or trains.
- Synchronizing Periodic Events: LCM helps in programming cases where multiple tasks occur at different intervals and need to be synchronized.
- Data Structure Operations: In algorithms involving repeated occurrences, LCM plays a crucial role.
Simulating LCM Problems
Creating practical examples by simulating real-world scenarios can enhance understanding. Implementing a project where schedules or periodic events are managed would be an excellent hands-on experience.

Conclusion
Summary of Key Points
In this guide, we explored how to compute LCM in C++, understood its mathematical foundations, and implemented it effectively using both custom functions and the STL. By leveraging the relationship between GCD and LCM, we can arrive at optimal solutions for various problems.
Further Reading and Resources
For continued learning, explore resources on C++ algorithms, mathematical functions in programming, and optimization techniques.
Call to Action
Take the concepts learned in this article and apply them in your own C++ projects. Experiment with different LCM problems to deepen your understanding and proficiency in using C++.