In C++, you can create a delay in your program using the `std::this_thread::sleep_for` function from the `<thread>` library, which pauses the execution for a specified duration.
#include <iostream>
#include <thread>
#include <chrono>
int main() {
std::cout << "Waiting for 2 seconds..." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(2));
std::cout << "Delay over!" << std::endl;
return 0;
}
Understanding Delays in C++
A delay in programming refers to a pause or suspension of execution for a specified duration. This can be crucial for various applications, such as controlling animation timing, limiting the rate of requests in a server environment, or providing essential pauses during user interactions.
In C++, understanding how to implement a delay correctly is vital, as it can affect both performance and user experience. This guide will explore several methods to achieve a delay in C++ effectively and succinctly.
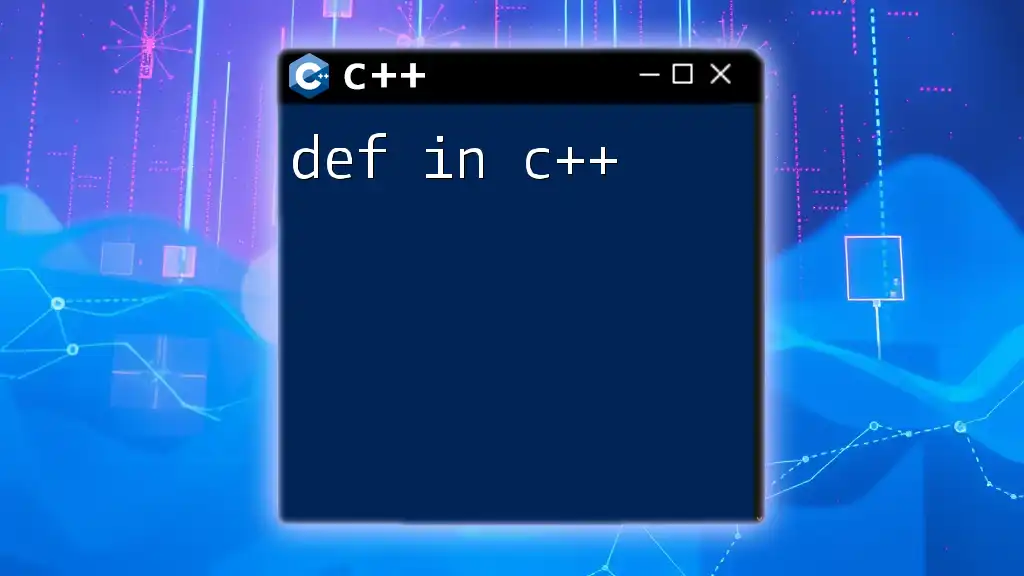
Common C++ Delay Methods
C++ Delay Using `<chrono>` and `<thread>`
The `<chrono>` and `<thread>` libraries in C++ provide robust tools for managing time-related tasks, including pauses and delays. The `<chrono>` library deals with time durations, while `<thread>` allows you to work with multiple threads within your application.
Example of Using std::this_thread::sleep_for
To implement a simple delay in C++, one of the most straightforward methods is to use `std::this_thread::sleep_for` in conjunction with `<chrono>`. Here is how you can do it:
#include <iostream>
#include <thread>
#include <chrono>
int main() {
std::cout << "Starting delay..." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(5));
std::cout << "Delay complete!" << std::endl;
return 0;
}
In this example, the program will print "Starting delay..." and then pause for 5 seconds. After the delay, it outputs "Delay complete!"
- Key Components:
- `std::this_thread::sleep_for`: This command directs the calling thread to sleep for the specified duration.
- `std::chrono::seconds`: This is part of the `chrono` library and indicates the number of seconds to sleep.
Using this method is generally recommended for simple delays due to its readability and ease of use.
C++ Wait Seconds Using a Loop
While `std::this_thread::sleep_for` is a popular choice, you may also implement a delay using a loop. This approach might be useful in more granular control over elapsed time.
Example of Manual Delay Implementation
Here's how you can create a manual delay function:
#include <iostream>
#include <ctime>
void delay(int seconds) {
clock_t start_time = clock();
while (clock() < start_time + seconds * CLOCKS_PER_SEC);
}
int main() {
std::cout << "Starting manual delay..." << std::endl;
delay(5);
std::cout << "Manual delay complete!" << std::endl;
return 0;
}
In this example, the `delay` function utilizes `clock()` to track time. The while loop continues until the elapsed time matches the input duration in seconds.
- Key Components:
- `clock()`: This function returns the number of clock ticks since the program started.
- `CLOCKS_PER_SEC`: A constant that defines how many clock ticks occur in one second.
While a manual delay might seem appealing, it doesn’t yield the CPU, which can lead to performance issues in complex applications. Hence, it is generally not recommended for GUI applications or those that require responsiveness.
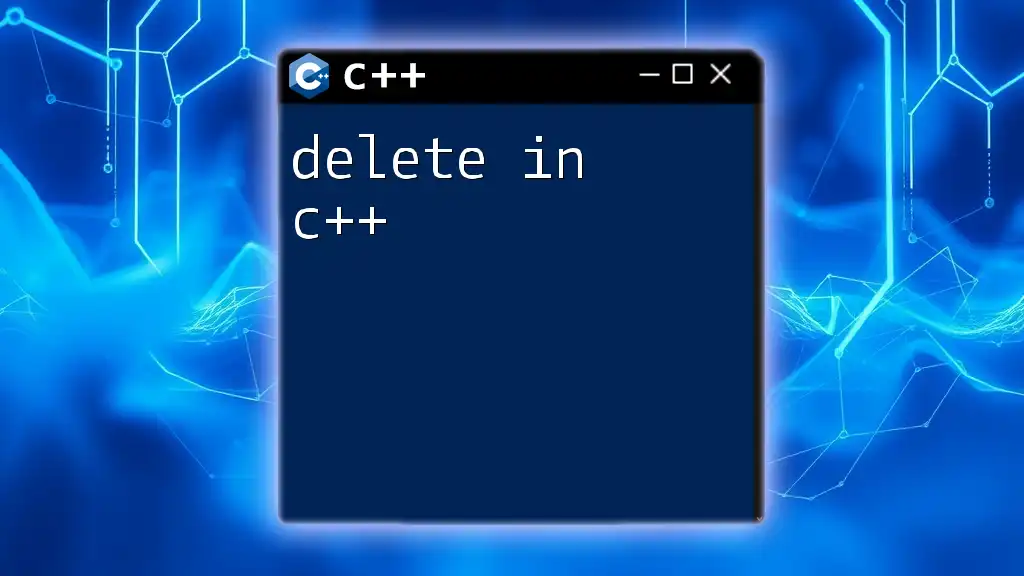
C++ Delay in Multi-threaded Applications
Importance of Proper Timing in Multi-threading
In multi-threaded applications, handling delays becomes significantly more complex. Managing the execution flow properly is crucial to avoid issues such as race conditions and deadlocks. Proper synchronization is essential to ensure that threads work harmoniously, especially when they rely on shared resources.
Using Condition Variables
Condition variables can help manage acknowledgments and timing between threads effectively. They allow one or more threads to wait until notified by another thread.
Example:
#include <iostream>
#include <thread>
#include <chrono>
#include <condition_variable>
std::condition_variable cv;
std::mutex mtx;
bool ready = false;
void waitForDelay() {
std::this_thread::sleep_for(std::chrono::seconds(5));
ready = true;
cv.notify_one();
}
int main() {
std::thread waiter(waitForDelay);
std::cout << "Waiting for delay..." << std::endl;
std::unique_lock<std::mutex> lock(mtx);
cv.wait(lock, [] {return ready;});
std::cout << "Delay completed!" << std::endl;
waiter.join();
return 0;
}
In this code snippet, the `waitForDelay` function pauses for 5 seconds before setting the `ready` variable to true and notifying the main thread. The main thread waits on the condition variable until it is notified.
- Key Components:
- `std::condition_variable`: Useful for waiting and notifying threads.
- `std::unique_lock`: This gives you mutual exclusion while waiting on the condition variable.
Utilizing condition variables ensures better performance and responsiveness in multi-threaded applications compared to busy-waiting in loops.
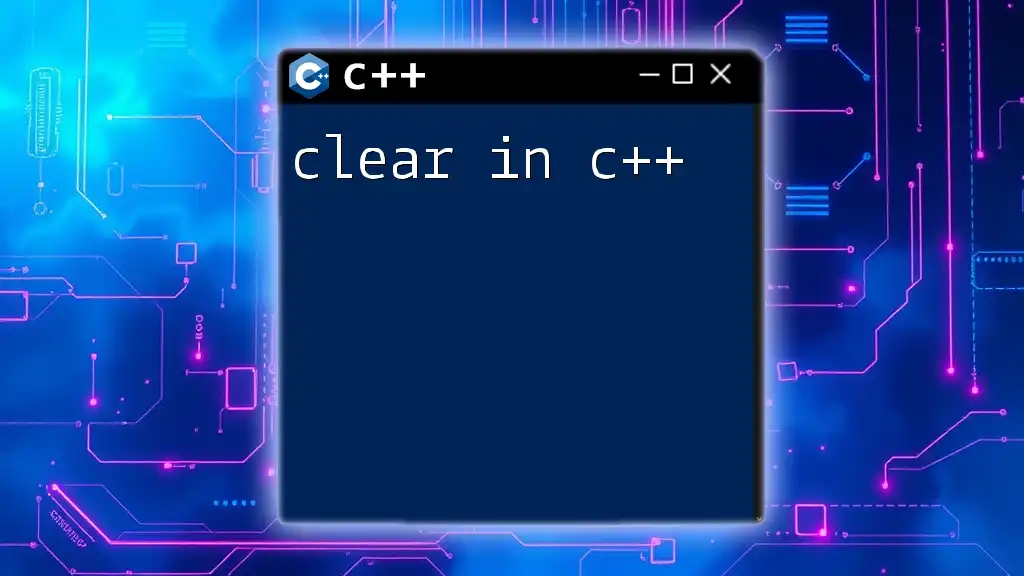
Alternatives to Standard Delay Methods
Async Programming and Futures
With the rapid evolution in programming paradigms, asynchronous programming has gained traction. Using `std::async`, you can manage delays without blocking the main execution thread. This method is particularly useful when you prefer a non-blocking way to incorporate delays.
Example with std::async
Here’s a simple implementation:
#include <iostream>
#include <future>
#include <chrono>
void asyncDelay() {
std::this_thread::sleep_for(std::chrono::seconds(5));
std::cout << "Async delay completed!" << std::endl;
}
int main() {
auto future = std::async(std::launch::async, asyncDelay);
std::cout << "Delayed operation started..." << std::endl;
future.get(); // Wait for completion
return 0;
}
In this example, the `asyncDelay` function runs asynchronously, allowing the main function to remain responsive. The output "Delayed operation started..." appears immediately, and "Async delay completed!" displays after the 5-second pause when the function returns.
- Key Components:
- `std::async`: Utilized to run the function asynchronously.
- `std::future`: Used to retrieve the return value of the asynchronous operation, blocking only when calling `get()`.
This method is advantageous in applications that require a delay without halting the entire system or user interface.
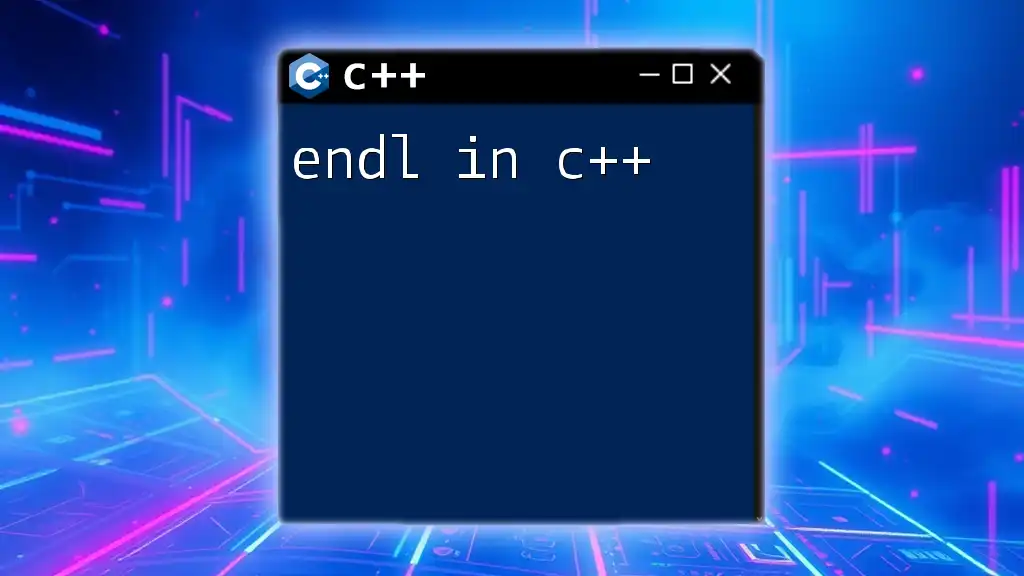
Tips for Implementing Delays in C++
When implementing delays in your applications, consider the following best practices:
- Avoid Unnecessary Delays: Implement delays only when absolutely necessary. Overusing delays can lead to a subpar user experience.
- User Experience: Think of how a delay may affect users. If delays are essential, communicate clearly to users, possibly through status messages or indicators.
- Performance Considerations: Always consider the performance implications, especially in multi-threaded applications. Non-blocking techniques should be preferred when appropriate.
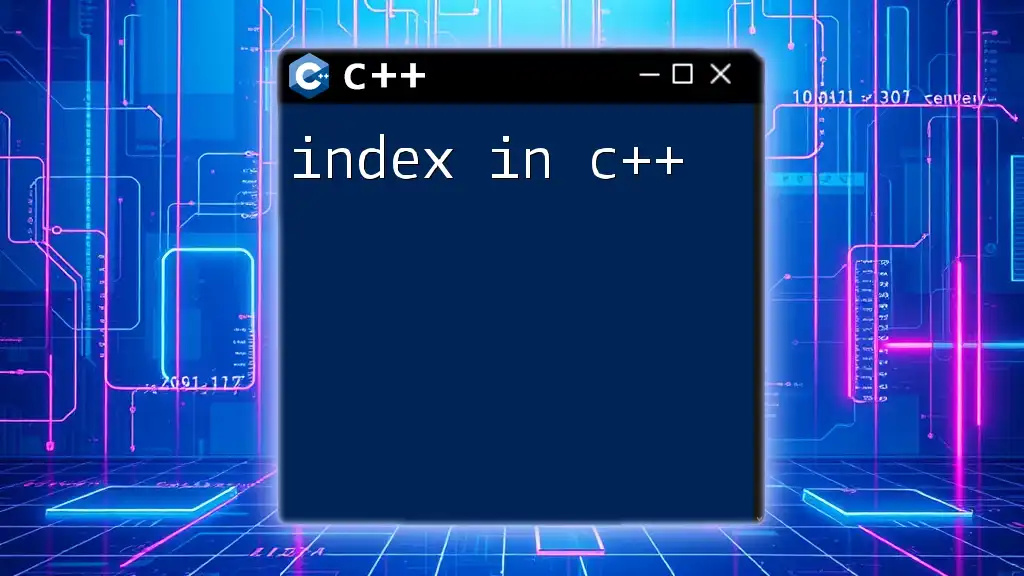
Conclusion
Delays in C++ are an essential aspect of programming that, when implemented correctly, can lead to improved application performance and enhanced user experience. From using the simple `std::this_thread::sleep_for` to handling complex multi-threaded synchronization with condition variables, developers have a myriad of tools at their disposal.
Experiment with the code snippets provided in this guide to deepen your understanding of the delay functionalities in C++. As always, keep learning and exploring additional resources to master the versatile landscape of C++.