The `gettimeofday` function in C++ is used to retrieve the current time with microsecond precision, typically utilized for measuring the elapsed time during program execution.
Here's a simple example of how to use `gettimeofday`:
#include <iostream>
#include <sys/time.h>
int main() {
struct timeval start, end;
gettimeofday(&start, NULL); // Get start time
// Your code here (e.g., a loop or any process)
gettimeofday(&end, NULL); // Get end time
long seconds = end.tv_sec - start.tv_sec;
long microseconds = end.tv_usec - start.tv_usec;
long elapsed = seconds * 1000000 + microseconds;
std::cout << "Elapsed time: " << elapsed << " microseconds" << std::endl;
return 0;
}
Understanding the gettimeofday Function
What is gettimeofday?
The `gettimeofday` function is a system call in C and C++ that retrieves the current time with a precision measured in microseconds. This function is particularly useful for applications where fine-grained timing information is essential, such as in logging, profiling, or performance measurement. By capturing the elapsed time between two points in a program, developers can gain insights into the performance characteristics of their code.
Syntax of gettimeofday
The syntax for using `gettimeofday` is straightforward. It is generally written as:
int gettimeofday(struct timeval *tv, struct timezone *tz);
- Parameters:
- `struct timeval *tv`: This pointer points to a `timeval` structure where the current time will be stored.
- `struct timezone *tz`: This parameter is often set to `NULL`, as the timezone information is not commonly needed in most applications.
Header Files and Namespaces
To utilize `gettimeofday`, you must include the appropriate header file:
#include <sys/time.h>
This header file defines the `timeval` structure and declares the `gettimeofday` function itself. While not specific to a namespace, it’s common practice to use the `std` namespace, especially when dealing with standard C++ functions in conjunction with C-style APIs.
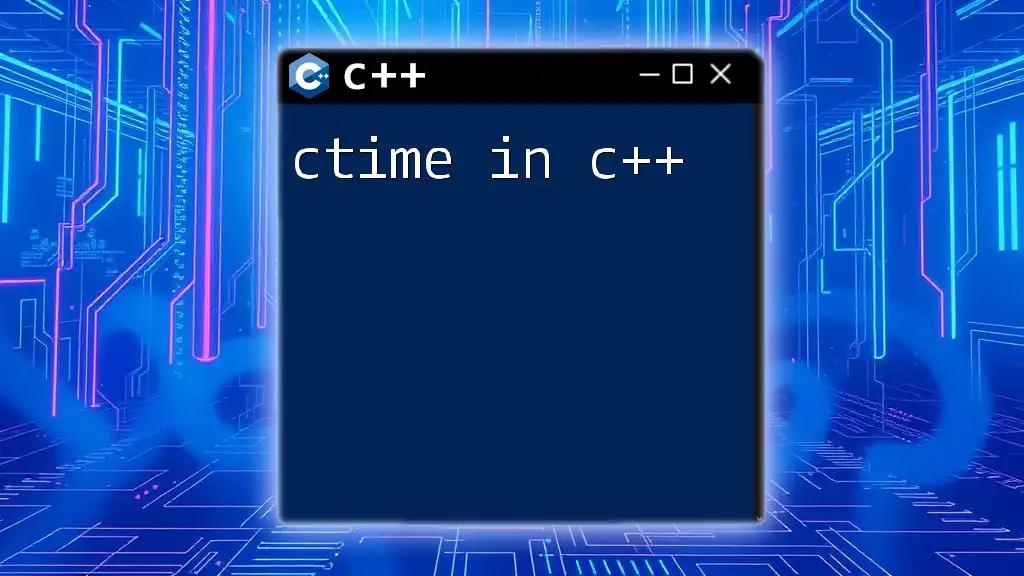
How to Use gettimeofday in C++
Initializing Required Structures
Before calling `gettimeofday`, you need to declare a variable of type `struct timeval` to store the results. Here’s how to initialize it:
struct timeval tv;
Capturing the Current Time
When you’re ready to retrieve the current time, use the `gettimeofday` function as follows:
gettimeofday(&tv, NULL);
After this call, the `tv` structure will be populated with two significant fields:
- `tv_sec`: This represents the number of seconds since the Epoch (00:00:00 UTC, January 1, 1970).
- `tv_usec`: This represents the additional number of microseconds, allowing for a finer resolution.
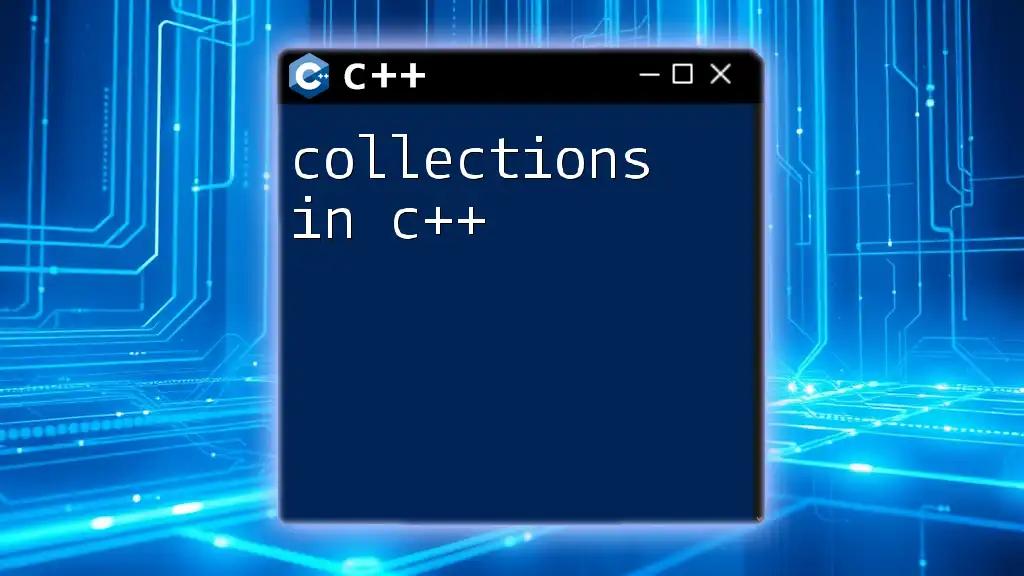
Formatting the Time
Converting struct timeval to Readable Format
Although `gettimeofday` provides the time in a structured format, you might want to convert it into a human-readable form. This can be accomplished by using the `localtime` and `strftime` functions. Here’s a full example:
#include <iostream>
#include <sys/time.h>
#include <ctime>
void printCurrentTime() {
struct timeval tv;
gettimeofday(&tv, NULL);
// Convert to time_t
time_t nowtime = tv.tv_sec;
struct tm nowtm;
localtime_r(&nowtime, &nowtm);
char timestamp[64];
strftime(timestamp, sizeof(timestamp), "%Y-%m-%d %H:%M:%S", &nowtm);
printf("Current Time: %s.%06ld\n", timestamp, tv.tv_usec);
}
In this example, `strftime` formats the time into a more easily understandable string. You’ll see output similar to the following:
Current Time: 2023-10-03 12:34:56.123456
In this output:
- The first part (up to seconds) is formatted as `YYYY-MM-DD HH:MM:SS`.
- The last part shows the microseconds (`tv_usec`).

Applications of gettimeofday in C++
Measuring Elapsed Time
One of the most common uses for `gettimeofday` is timing the execution of specific segments of code. By capturing the start and end times around a function call, you can compute the duration of that function's execution. Here’s a practical example:
#include <iostream>
#include <sys/time.h>
void exampleFunction() {
// Simulate some processing
for (volatile int i = 0; i < 1000000; ++i); // Dummy work
}
int main() {
struct timeval start, end;
gettimeofday(&start, NULL);
exampleFunction(); // Call the function whose execution time you want to measure
gettimeofday(&end, NULL);
long seconds = end.tv_sec - start.tv_sec;
long micros = end.tv_usec - start.tv_usec;
long elapsed = seconds * 1000000 + micros;
std::cout << "Elapsed Time: " << elapsed << " microseconds." << std::endl;
}
In this code:
- You'll start by capturing the current time before invoking `exampleFunction()`.
- After the function completes its execution, you call `gettimeofday` again to capture the end time.
- The elapsed time is then calculated, giving you the duration of the function call.
Real-World Use Cases
Real-world applications of `gettimeofday` include:
- Logging: Appending timestamps to logs for debugging and analysis.
- Profiling: Measuring the execution time of various operations to identify bottlenecks.
- Synchronization: Precise timing for events in distributed systems or network applications.
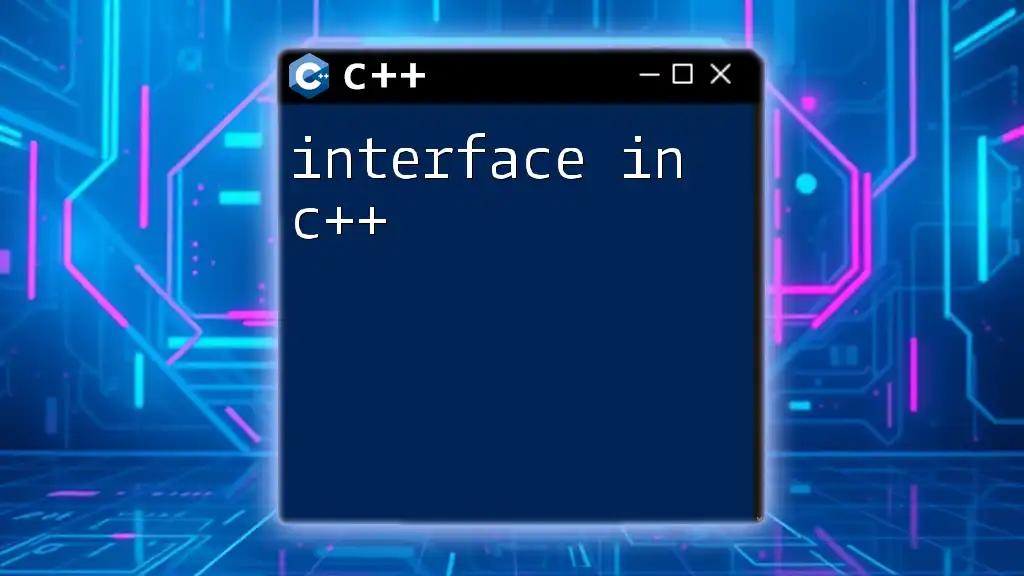
Alternatives to gettimeofday
Using C++11 <chrono> Library
While `gettimeofday` is a reliable function, C++11 introduced the `<chrono>` library, which provides a more modern and type-safe approach to time handling. This library is designed to work seamlessly with the C++ standard library. Here’s a brief demonstration comparing `gettimeofday` with `<chrono>`:
#include <chrono>
#include <iostream>
void exampleFunction() {
// Simulate some processing
}
int main() {
auto start = std::chrono::high_resolution_clock::now();
exampleFunction();
auto end = std::chrono::high_resolution_clock::now();
auto duration = std::chrono::duration_cast<std::chrono::microseconds>(end - start).count();
std::cout << "Elapsed Time: " << duration << " microseconds." << std::endl;
}
This example achieves the same purpose using the `<chrono>` library, offering greater flexibility and type safety.
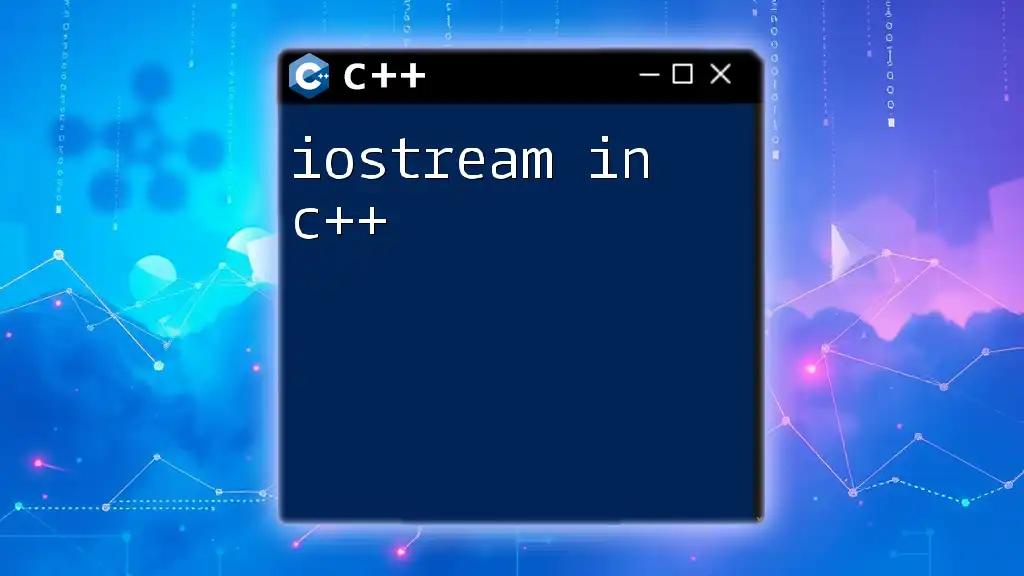
Best Practices and Considerations
When to Use gettimeofday
- Use `gettimeofday` when you require microsecond precision and compatibility with existing C/C++ code that leverages system calls.
- Stick with it when working in legacy codebases where `<chrono>` might not be available.
Performance Implications
While calling `gettimeofday` incurs a slight performance overhead, it’s typically negligible for most applications. However, if you're computing time in tight loops or performance-sensitive areas, consider the impact on execution speed. If necessary, batch calls or minimize the frequency of time checks.
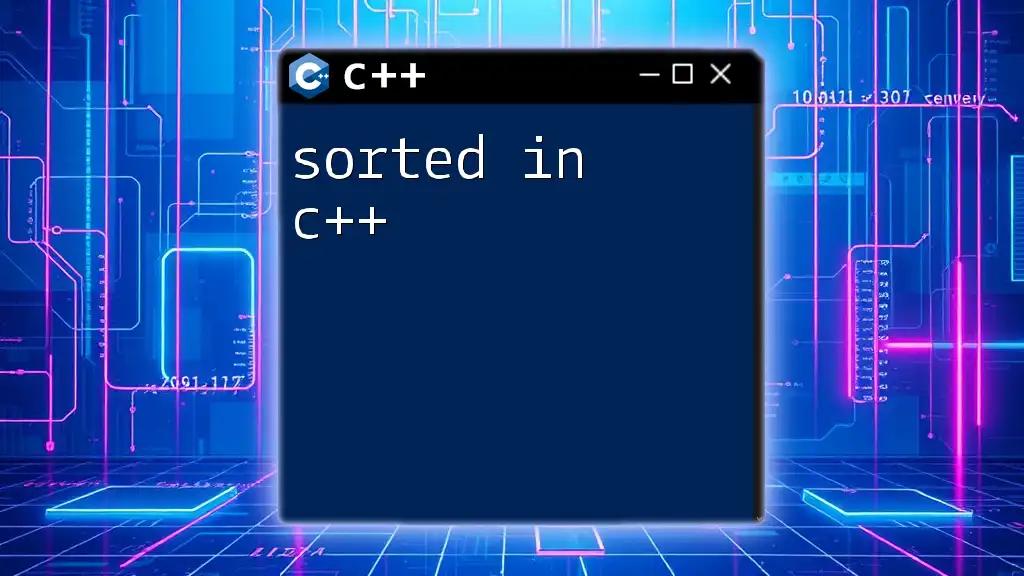
Conclusion
In summary, gettimeofday in C++ provides a powerful mechanism for working with time at a microsecond precision level. It allows developers to capture current time values, format them neatly, and measure elapsed time effectively. While alternatives exist, particularly in modern C++ with the `<chrono>` library, `gettimeofday` remains a valuable tool for many timing-related tasks. Experimenting with both methods can deepen your understanding and leverage the strengths of each approach to optimize your applications.

Additional Resources
For further exploration, consider reviewing official C++ documentation and tutorials on effective use of timing functions. These resources can enhance your understanding and application of time handling in programming.