Nested if statements in C++ allow you to check multiple conditions by placing one if statement inside another, enabling more complex decision-making.
Here’s an example in C++:
#include <iostream>
using namespace std;
int main() {
int a = 10, b = 20;
if (a > 0) {
if (b > 0) {
cout << "Both a and b are positive." << endl;
}
}
return 0;
}
What are Nested If Statements?
Nested if statements allow us to execute a block of code based on multiple conditions. They are a powerful feature in C++, enhancing the control flow by allowing decisions to be made beyond a single condition. Essentially, a nested if statement is an if statement contained within another if statement.
Importance of Control Flow in C++
Control flow mechanisms such as if statements, loops, and switches dictate how a program executes based on varying conditions. Understanding nested if statements expands your ability to write more complex and responsive code that can handle various scenarios, making your programs more intelligent and user-friendly.
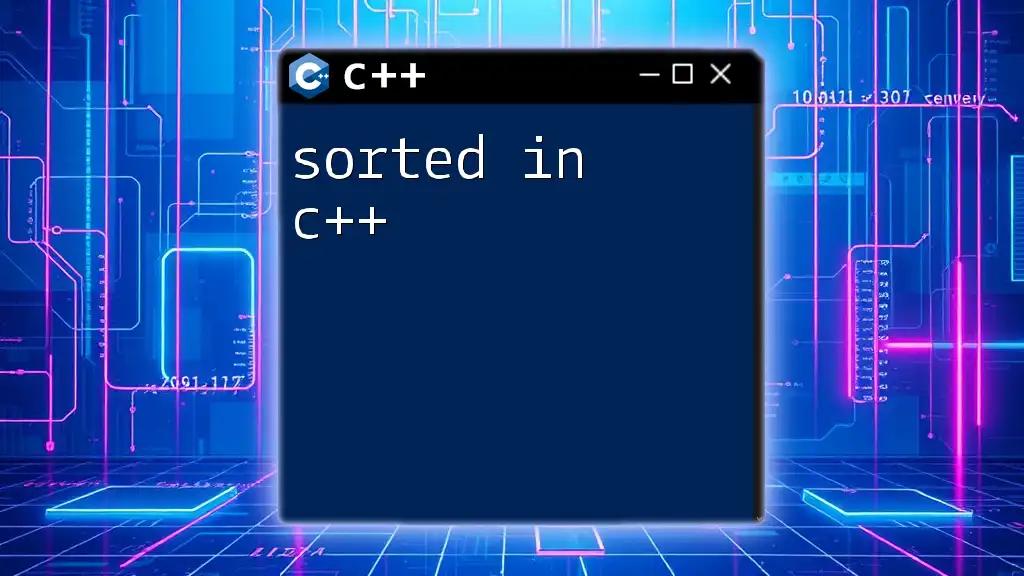
Understanding the Basics of If Statements
Definition of an If Statement
At its core, an if statement checks a condition to determine whether to execute a specific block of code. If the condition evaluates to true, the code inside the if block gets executed; if it evaluates to false, that block is skipped.
Here’s how a single-level if statement looks in C++:
if (condition) {
// Code to execute if condition is true
}
Introduction to Boolean Logic
C++ relies heavily on Boolean logic—true or false values. Each condition written in an if statement evaluates to either true or false, determining the program's path of execution. Remember that understanding how to construct these conditions is crucial for maximizing the utility of nested if statements.

The Structure of Nested If Statements
What is a Nested If Statement?
A nested if statement is simply an if statement placed inside another if statement. By using nested if statements, programmers can evaluate multiple conditions in a sequence, allowing for complex decision-making.
Anatomy of a Nested If Statement
To illustrate, consider the following structure of a nested if statement:
if (condition1) {
// Code block 1
if (condition2) {
// Code block 2
}
}
In this example, Code block 2 only gets executed if both condition1 is true and condition2 is also true. This cascading structure allows you to funnel the logic needed to capture specific scenarios.

Examples of Nested If Statements in C++
Basic Example: Checking Age and Status
Let’s look at a practical scenario: determining if someone is eligible to vote. This example involves checking a person’s age and their citizenship status.
int age;
char status;
std::cout << "Enter age: ";
std::cin >> age;
if (age >= 18) {
std::cout << "Are you a citizen? (Y/N): ";
std::cin >> status;
if (status == 'Y' || status == 'y') {
std::cout << "You are eligible to vote.";
} else {
std::cout << "You are not eligible to vote.";
}
} else {
std::cout << "You are not eligible to vote.";
}
In this example:
- If the age is greater than or equal to 18, we check the status—if they are a citizen.
- The inner if statement then determines if the user can vote based on citizenship, demonstrating a classic use of nested if statements in C++.
Advanced Example: Grading System Implementation
Now, let’s see a more advanced example involving a grading system where scores determine letter grades:
int score;
std::cout << "Enter your score: ";
std::cin >> score;
if (score >= 0 && score <= 100) {
if (score >= 90) {
std::cout << "Grade: A";
} else if (score >= 80) {
std::cout << "Grade: B";
} else if (score >= 70) {
std::cout << "Grade: C";
} else {
std::cout << "Grade: D";
}
} else {
std::cout << "Invalid score. Please enter a score between 0 and 100.";
}
Here, we:
- First check if the score is within a valid range (0-100).
- Depending on the score, we further check the grade category, effectively demonstrating how nested if statements can streamline complex decision trees.

Best Practices for Using Nested If Statements
Readability and Maintainability
When it comes to writing code, clarity and maintainability are paramount. Nested if statements can lead to complex code if overused, which can make it hard to debug and understand. Always strive for readability; consider adding comments when necessary to explain the logic, especially in deep nesting.
Reduce Complexity: Avoiding Deep Nesting
While nested if statements are a valuable tool, relying on excessively deep nesting can be counterproductive. Consider alternative constructs such as:
- Switch Statements for multiple related checks
- Ternary Operators for simple binary conditions
These alternatives can simplify your code and enhance overall clarity.
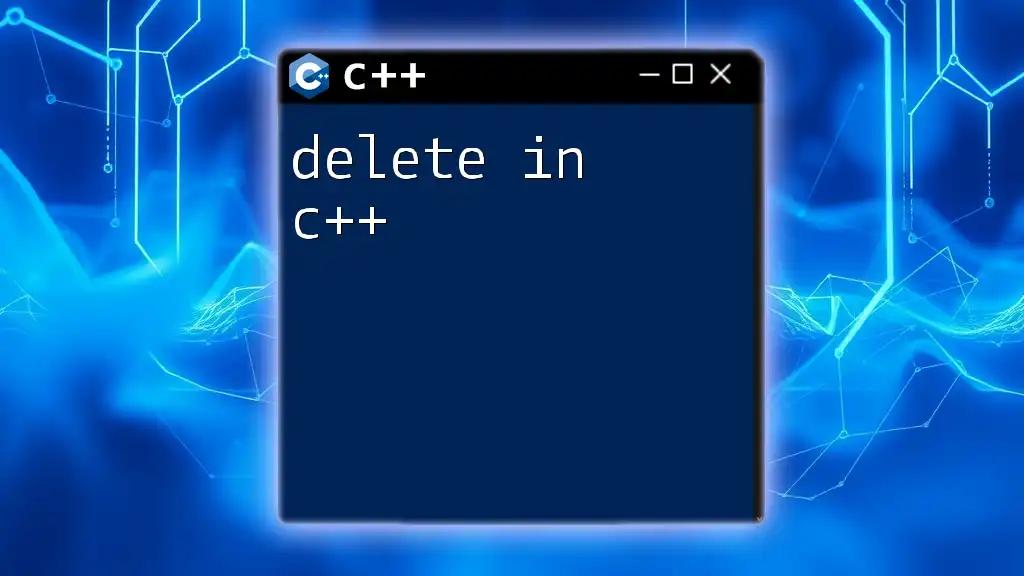
Common Mistakes in Nested If Statements
Overlooking Edge Cases
One common pitfall in using nested if statements is failing to account for all possible edge cases. For instance, in the grading system example, scores below 0 or above 100 are not valid. Always consider how edge cases might affect your logical structure and ensure you handle these conditions gracefully.
Misunderstanding Logical Conditions
Incorrectly using logical operators can lead to unintended behaviors. Ensure you have a solid grasp of how your conditions will interact. A misplaced operator or incorrect precedence can dramatically change the flow of your program.
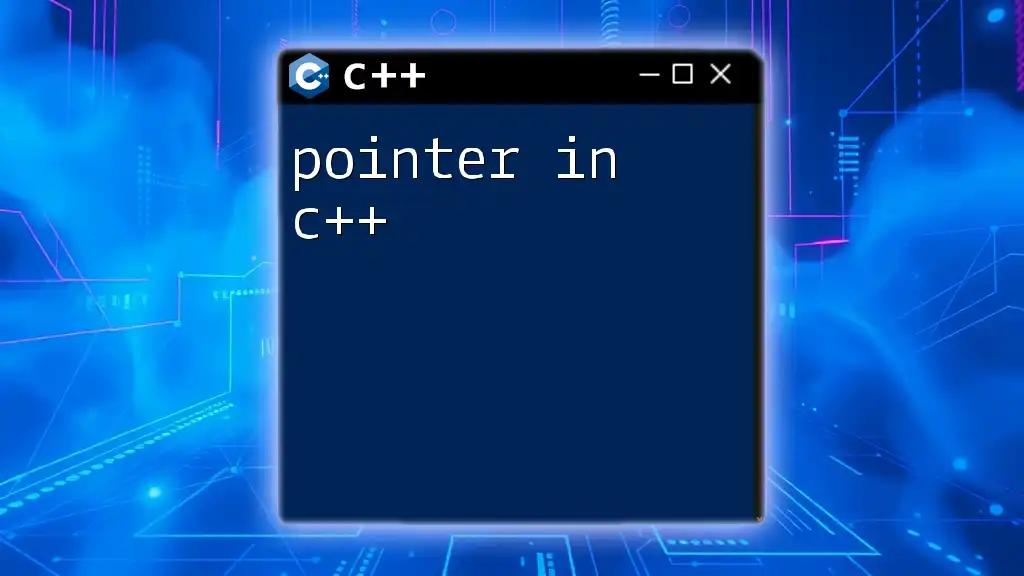
Conclusion: Mastering C++ Nested If Statements
Mastering nested if statements is crucial in C++ development. They provide an advanced way to manage flow control and make your programs adaptable to complex scenarios. Practice by integrating nested if statements into various exercises, gradually increasing their complexity.
As you continue exploring C++, remember that clarity and simplicity are key. By balancing the use of nested structures with alternatives and good coding practices, you’ll enhance your proficiency and build more efficient programs.
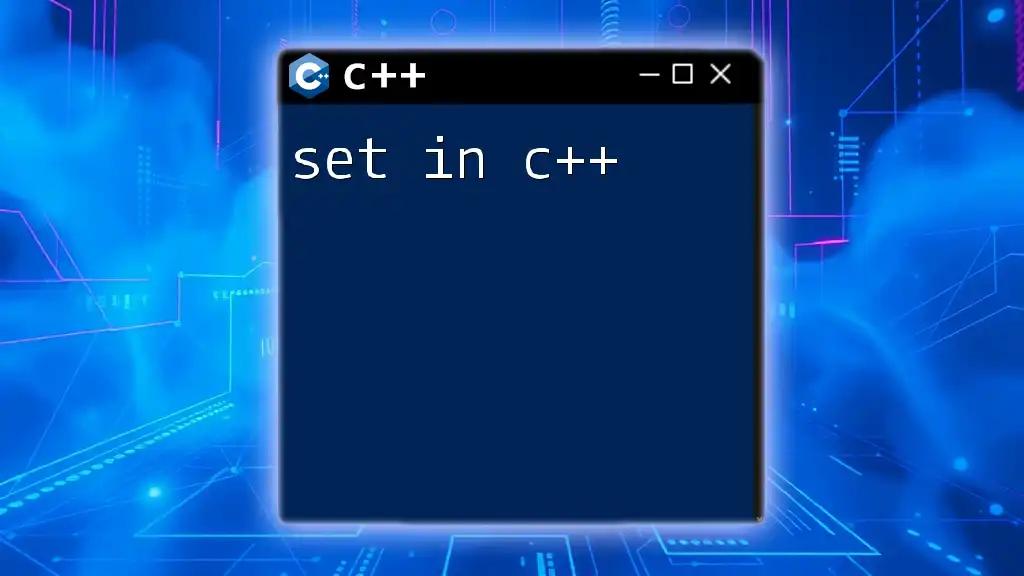
Additional Resources
- Explore the official C++ documentation for further insights on if statements.
- Check out online coding platforms to practice and challenge your understanding of nested if statements in various scenarios.
Continuing on this journey will strengthen your programming skills, allowing you to tackle more sophisticated coding problems with confidence.