"Writing in C++ involves using its syntax to develop programs that can efficiently manipulate data, perform calculations, and manage resources."
Here’s a simple example of a C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++
What is C++?
C++ is a powerful general-purpose programming language that acts as an extension of the C programming language. Developed by Bjarne Stroustrup in the early 1980s, it incorporates object-oriented programming features with procedural programming capabilities. C++ is renowned for its performance, efficiency, and versatility, making it a popular choice for high-performance applications ranging from game development to system software.
Why Learn C++?
Learning C++ comes with multiple advantages. Its wide acceptance in industry applications means that mastering this language can open doors to many career opportunities. C++ is especially prominent in game development, performance-critical applications, and software systems where efficiency and speed are paramount. Furthermore, it equips learners with a deep understanding of computer science fundamentals, especially those involved in low-level programming.
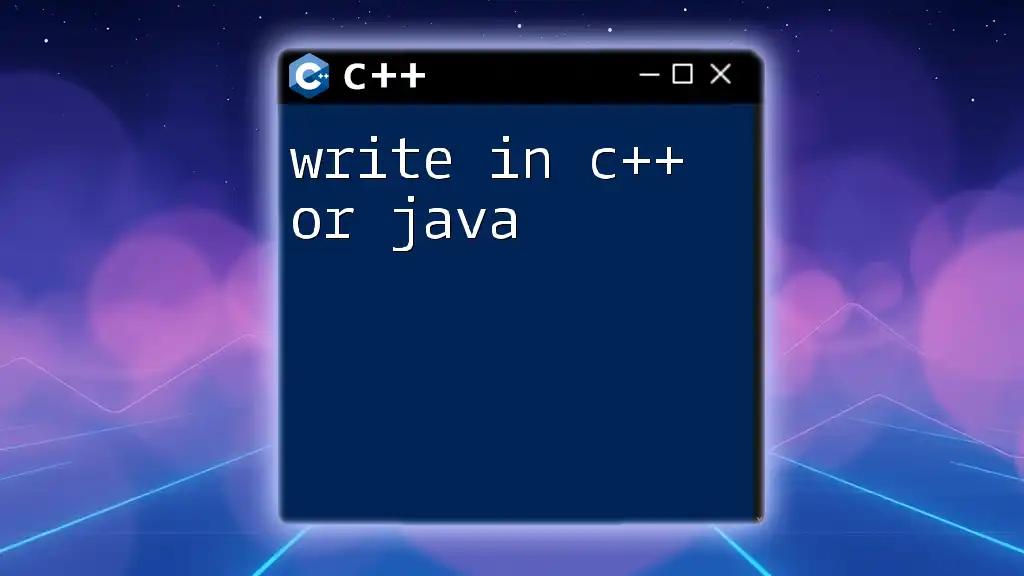
Getting Started with C++
Setting Up Your Environment
Before you can write in C++, you must set up a suitable environment for coding. Here are a few recommendations:
- IDEs: Look into using Integrated Development Environments (IDEs) like Visual Studio Code, Code::Blocks, or CLion. These provide features such as syntax highlighting and debugging tools which enhance your coding experience.
- Compilers: Use compilers such as GCC, MinGW, or Microsoft Visual C++. Proper compiler setup ensures that you can successfully compile and run your C++ code on your machine.
Your First C++ Program
Let’s dive in and try to write a simple program that displays "Hello, World!" to the console. This foundational program is often the first step in learning any programming language.
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this code:
- `#include <iostream>` is a preprocessor directive that tells the compiler to include the standard input-output stream library, enabling the program to perform input and output operations.
- We’re using the `cout` object from the `std` namespace to output our message to the console.
- The `main` function is where program execution begins.
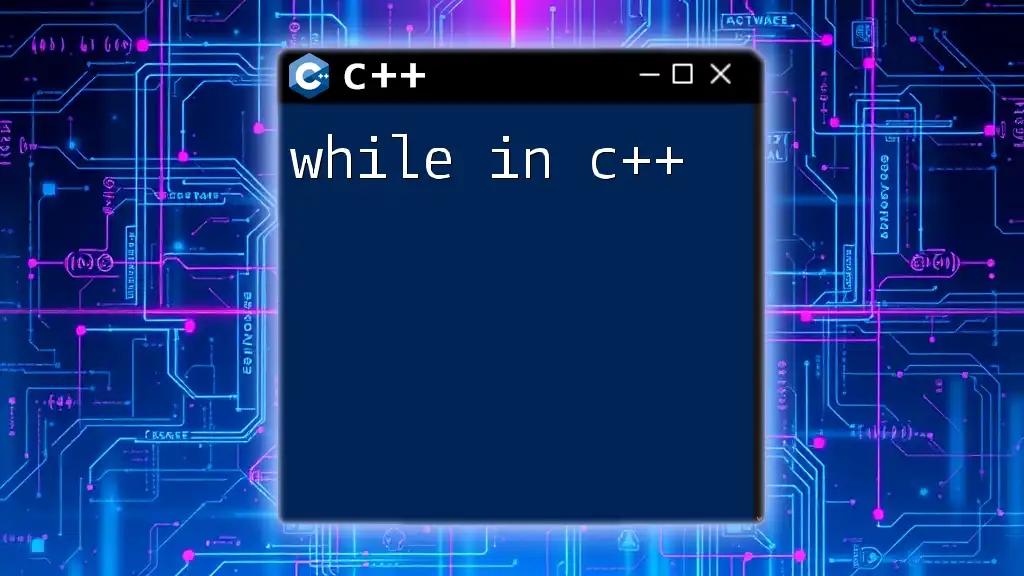
The Basics of Writing in C++
Variables and Data Types
In C++, variables are used to store data. The type of data stored dictates the data type you’ll use, which can be int, float, double, char, or string, among others.
Here’s a simple example of declaring different variables:
int age = 25;
float height = 5.9f;
char initial = 'A';
string name = "Alice";
Understanding data types is crucial, as they govern how much memory you need to allocate and how you can manipulate the data during execution.
Operators
C++ provides various operators that allow you to perform operations on variables. Familiar operators include:
- Arithmetic Operators: `+`, `-`, `*`, `/`, and `%`
- Comparison Operators: `==`, `!=`, `>`, `<`, `>=`, `<=`
For instance, here’s how you can perform some basic operations:
int sum = age + 5; // Addition
bool isAdult = (age >= 18); // Comparison
These operators make it simple to execute common tasks in programming.
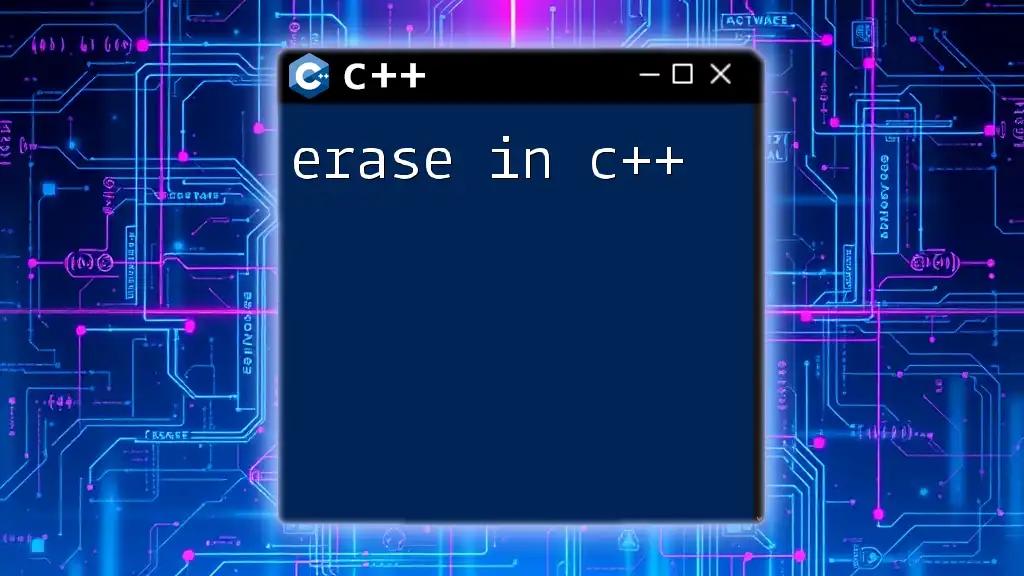
Control Structures
Conditional Statements
In programming, conditional statements allow us to execute certain pieces of code based on specified conditions. The most common forms are `if` statements and `switch` cases.
If Statements:
if (age >= 18) {
cout << "Adult" << endl;
} else {
cout << "Minor" << endl;
}
In this example, the program checks if the person is an adult based on their age.
Switch Cases:
switch (age) {
case 18:
cout << "Just became an adult!" << endl;
break;
default:
cout << "Age not relevant" << endl;
}
Loops
Loops are essential for executing a block of code repeatedly until a specified condition is met.
For Loops:
for (int i = 0; i < 5; i++) {
cout << i << endl;
}
This `for` loop will print numbers from 0 to 4.
While Loops:
int i = 0;
while (i < 5) {
cout << i << endl;
i++;
}
The `while` loop continues as long as the condition remains true, allowing you to control the flow of your program effectively.
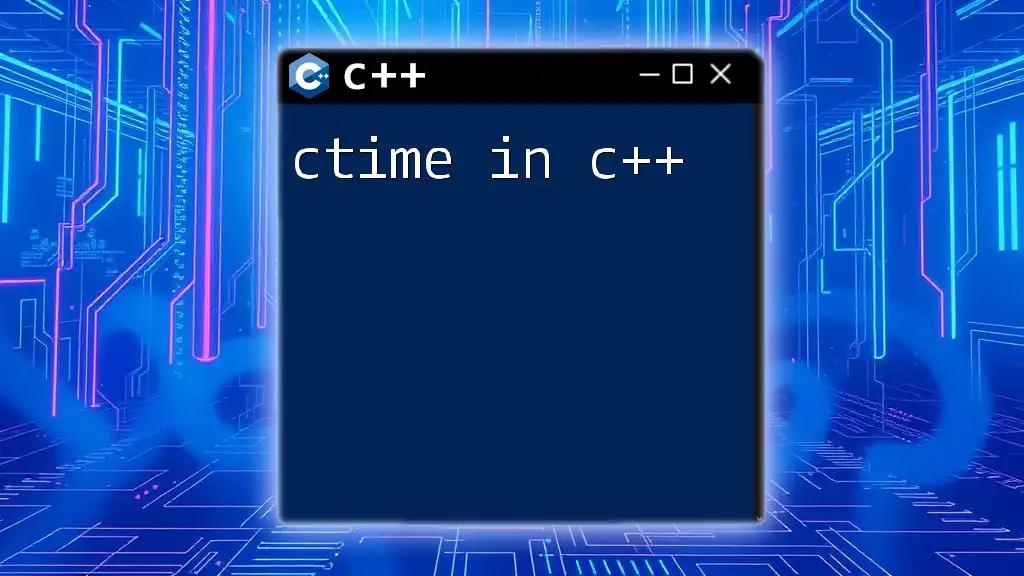
Functions
What are Functions?
Functions are reusable blocks of code designed to perform a specific task. They promote organization and can simplify complex problems.
How to Write and Call Functions
Here’s an example of writing a simple function that adds two numbers:
int add(int a, int b) {
return a + b;
}
int main() {
int result = add(5, 10);
cout << "Sum: " << result << endl;
return 0;
}
In this case, the function `add` takes two integer parameters and returns their sum. In the `main` function, we call `add` and output the result.
Function Overloading
C++ allows you to have multiple functions with the same name, provided they accept different parameters—this is known as function overloading.
int add(int a, int b) {
return a + b;
}
float add(float a, float b) {
return a + b;
}
This helps create more readable and maintainable code.
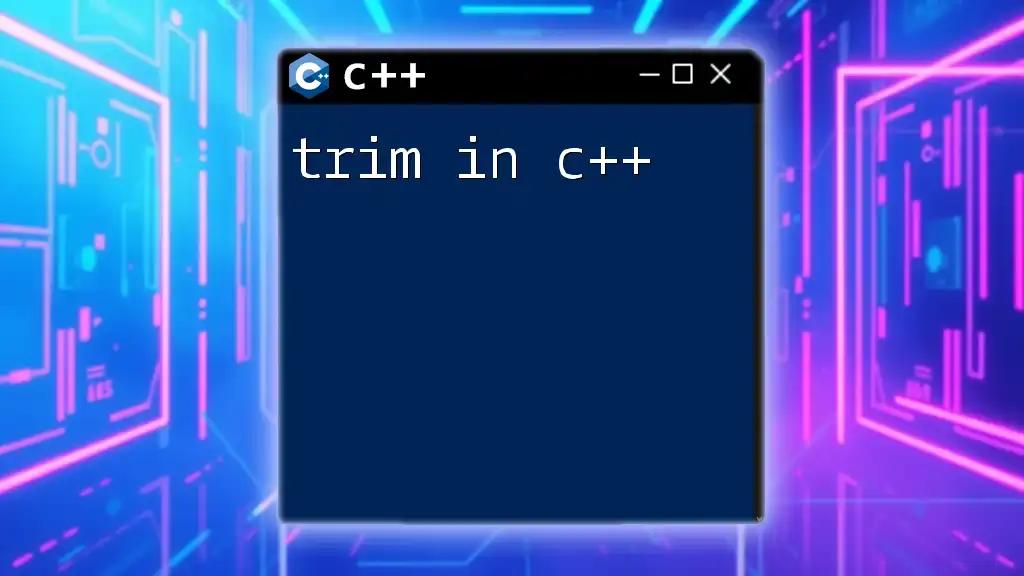
Object-Oriented Programming in C++
Understanding Classes and Objects
Object-oriented programming, a key feature of C++, revolves around the concepts of classes and objects. A class encapsulates data and functions, while an object is an instance of a class.
Here’s an example illustrating a simple class:
class Dog {
public:
string breed;
void bark() {
cout << "Woof!" << endl;
}
};
int main() {
Dog dog;
dog.breed = "Labrador";
dog.bark();
return 0;
}
In this example, `Dog` is our class with a public attribute `breed` and a method `bark`. We create an object `dog` to access its properties and methods.
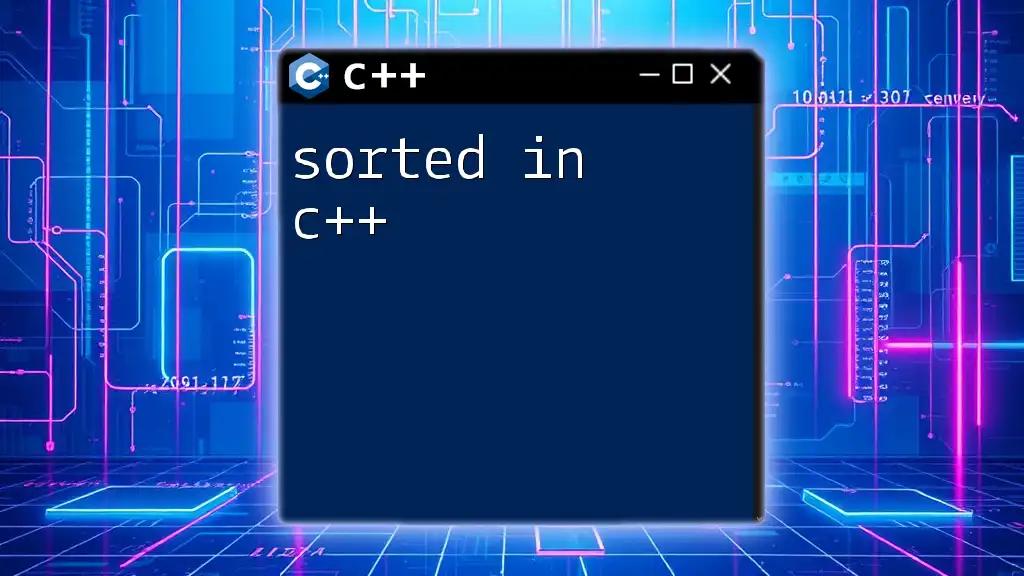
Advanced Concepts
Pointers and References
A deeper understanding of C++ includes pointers, which are variables that store memory addresses. This feature is useful for dynamic memory management and understanding how data is accessed in memory.
Here’s a brief look at pointers:
int a = 10;
int* ptr = &a;
cout << "Value of a: " << *ptr << endl; // Dereferencing
The `&` operator retrieves the address of the variable `a`, while the `*` operator dereferences the pointer to access the value at that address.
Standard Template Library (STL)
The Standard Template Library (STL) is a powerful feature of C++ that provides templates for commonly used data structures such as vectors, lists, and maps. It significantly enhances productivity and efficiency in C++ programming.
Here’s how to use vectors from the STL:
#include <vector>
vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
cout << num << endl;
}
STL allows you to implement complex data structures with ease and is highly optimized for performance.
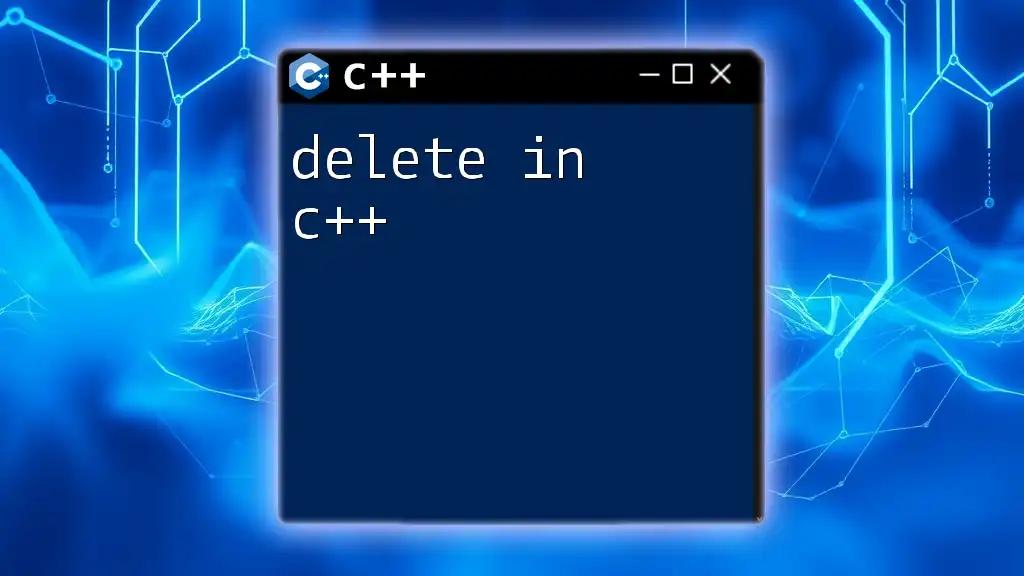
Best Practices and Tips
Code Readability
Writing clear and readable code is vital. Incorporate comments effectively, and choose meaningful variable names. This not only helps others understand your work but also allows you to revisit your own code with ease.
Debugging Techniques
Debugging is an essential part of coding. Utilize tools such as gdb (GNU Debugger) or built-in IDE debuggers to trace errors and issues within your code. Building a habit of testing your code regularly can save time in the long run.
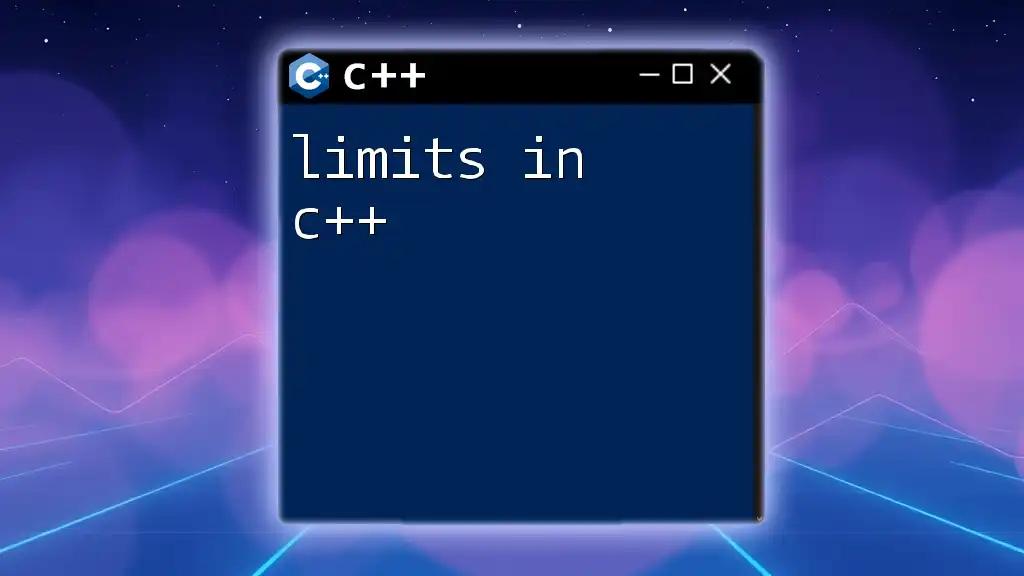
Conclusion
In this comprehensive guide, we have explored the essentials of how to write in C++, ranging from the setup of your development environment to advanced programming concepts. Whether you are a novice or looking to deepen your understanding, the practice of writing code in C++ will elevate your programming skills. Embrace the learning journey, and don’t hesitate to experiment with what you’ve learned.

Call to Action
We encourage you to share your experiences with C++ and join our community to learn more about mastering this language. Sign up for our upcoming tutorials and courses to deepen your understanding of C++ and its applications!