In C++, the `exit()` function is used to terminate a program immediately, optionally providing a status code to indicate success or failure.
#include <cstdlib> // Required for exit()
#include <iostream>
int main() {
std::cout << "Exiting the program." << std::endl;
exit(0); // Exit with success status
}
What is the Exit Function in C++?
The exit function in C++ is a predefined function that terminates a program's execution immediately. It provides a way to gracefully or abruptly end a program based on specific conditions. Understanding how the exit function operates is crucial for effective program control and error handling.
Syntax of the Exit Function in C++
The syntax of the exit function is as follows:
void exit(int status);
- The function takes a single argument, status, which is an integer indicating the termination status of the program.
- A value of `0` typically represents successful completion, whereas a non-zero value signifies that the program encountered an error or an exceptional condition.
Understanding the Parameter: Status Code
The status code you pass to the exit function delivers contextual information about why the program is terminating. The convention is that:
- `0` indicates successful completion.
- Any non-zero value (like `1`, `-1`, etc.) indicates an error condition. Specific non-zero values can carry specific meanings, which can be defined by programmers depending on their context.
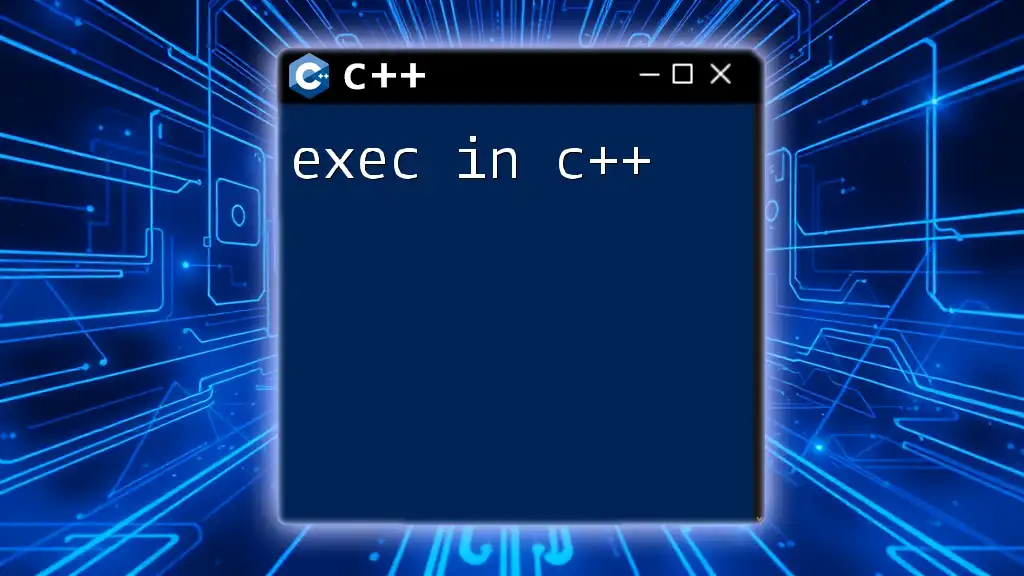
How to Use the Exit Command in C++
Using the exit command effectively can enhance your program's reliability and clarity. You might want to utilize it in various scenarios, including:
- When an error occurs, and immediate termination is necessary.
- When the program execution reaches a certain end condition that does not allow for normal progression.
Example 1: Basic Usage of Exit in C++
Here is a simple code snippet demonstrating the basic usage of the exit function:
#include <iostream>
#include <cstdlib> // for exit
int main() {
std::cout << "Program Starting..." << std::endl;
// Conditional check
if (/* Some error condition */) {
std::cerr << "An error occurred!" << std::endl;
exit(1); // Exiting with a failure status code
}
std::cout << "Program Running..." << std::endl;
exit(0); // Exiting successfully
}
In this example, if an error condition is met, the program outputs an error message and uses `exit(1)` to terminate. If the program runs successfully, `exit(0)` is invoked.
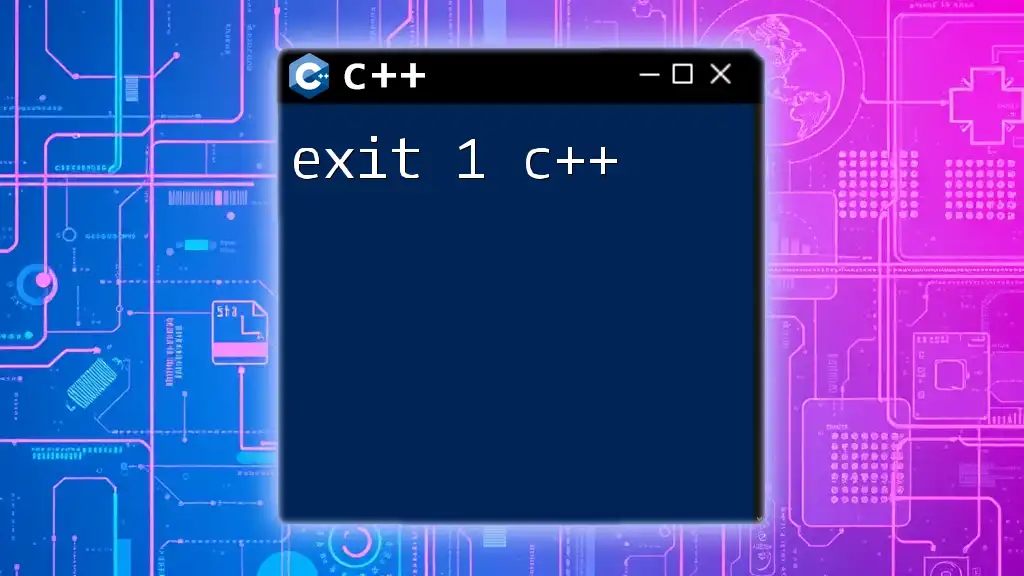
The Role of Exit vs. Return Statement
While both the exit command and the return statement serve to terminate program execution, there are important differences between them. The `return` statement works within the confines of a function and allows the program to exit only from the invoked function. In contrast, `exit()` ends the program immediately, no matter how deeply nested the current function is.
Example 2: Demonstrating the Differences
Here’s an example that highlights this distinction:
#include <iostream>
void checkCondition() {
// Simulating a failure
std::cerr << "Error in function!" << std::endl;
exit(1); // Exit from the program
}
int main() {
std::cout << "Program Starting..." << std::endl;
checkCondition();
std::cout << "This will not print if exit is called." << std::endl;
}
In the code above, when `checkCondition()` is called, it triggers the exit function, and the program terminates before it reaches the next line in `main()`.
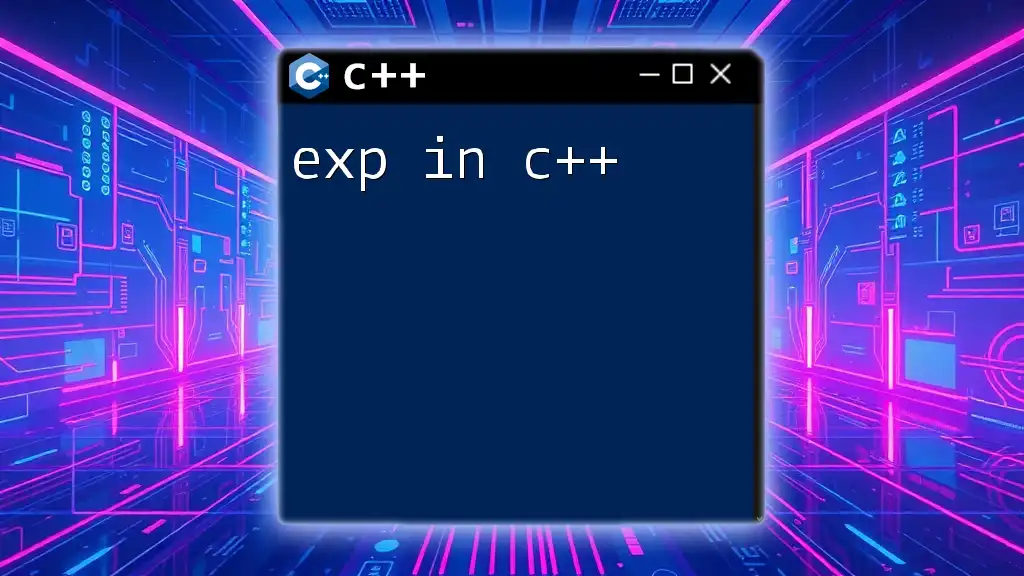
How to Handle Cleanup Before Exiting
When using the exit command, it's essential to manage resources properly. Unreleased resources can lead to memory leaks or other unwanted behaviors in your applications. C++ provides the `atexit()` function, allowing you to register cleanup functions that execute before the program terminates.
Example 3: Registering Cleanup Functions
The following code demonstrates how to register a cleanup function that runs before exiting:
#include <iostream>
#include <cstdlib>
void cleanup() {
std::cout << "Cleaning up resources..." << std::endl;
}
int main() {
std::atexit(cleanup); // Registering cleanup function
// Main program logic...
std::cout << "Program is running." << std::endl;
exit(0); // Cleanup will be executed here
}
In this example, the `cleanup()` function will execute just before the program exits, ensuring that any necessary cleanup tasks are performed.
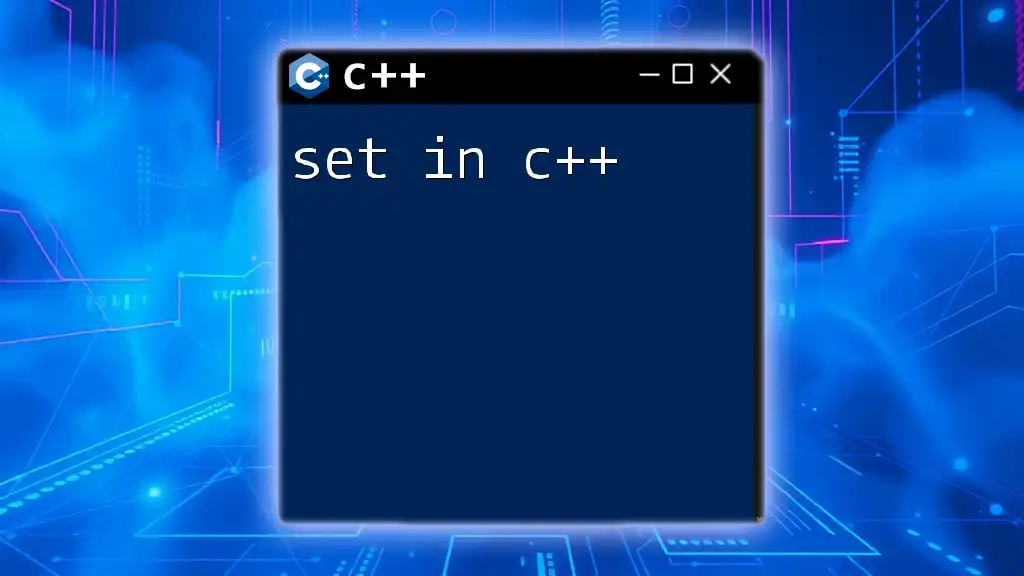
Alternative Methods to Exit a C++ Program
While the exit function is powerful, multiple methods allow for program termination.
- Throwing exceptions: This approach allows you to manage error handling using C++’s exception handling mechanism, enabling more graceful exits.
- Infinite loops: These can be used as a last resort to halt program execution, although they aren't an elegant solution.
- Using return in main(): This method concludes the main function and consequently ends the program without involving the exit function.
Example 4: Using Return in Main
Here’s a simple demonstration using `return`:
#include <iostream>
int main() {
std::cout << "Program Starting..." << std::endl;
// Normal exit without using exit()
return 0; // returning success
}
In this case, the return statement in the `main()` function signifies that the program ended successfully.
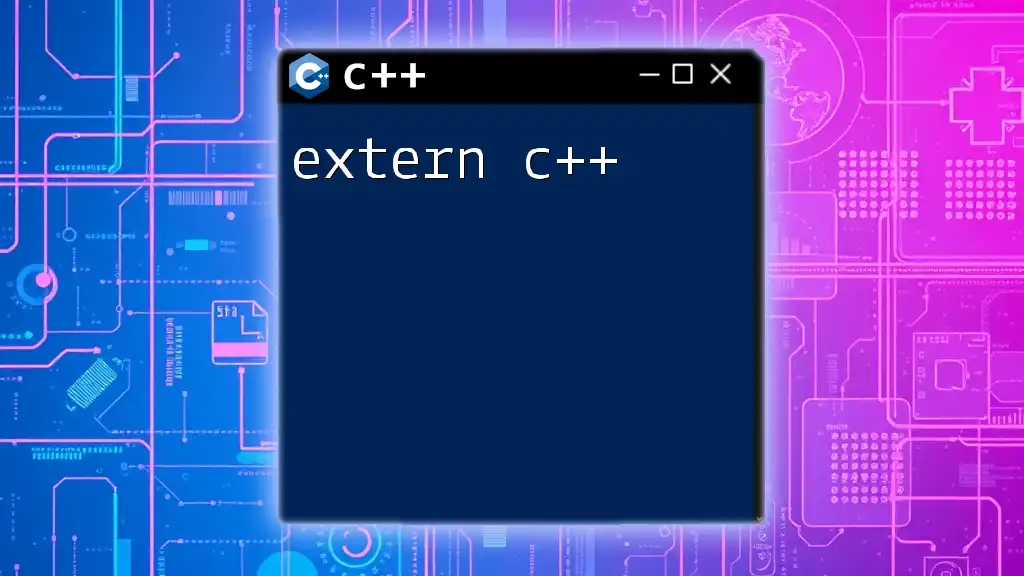
Best Practices for Using the Exit Command in C++
When incorporating the exit command into your C++ programs, consider the following best practices to enhance your code quality:
- Use exit() effectively: Reserve the use of the exit function for critical errors or conditions where a normal program flow cannot continue.
- Avoid abrupt exits: Large-scale programs can become challenging to debug if you bypass normal flow using exit(). Instead, consider handling errors through exceptions or return values where applicable.
- Leverage informative status codes: Customize your exit status codes to provide meaningful information regarding program termination; this can assist in troubleshooting and debugging issues.
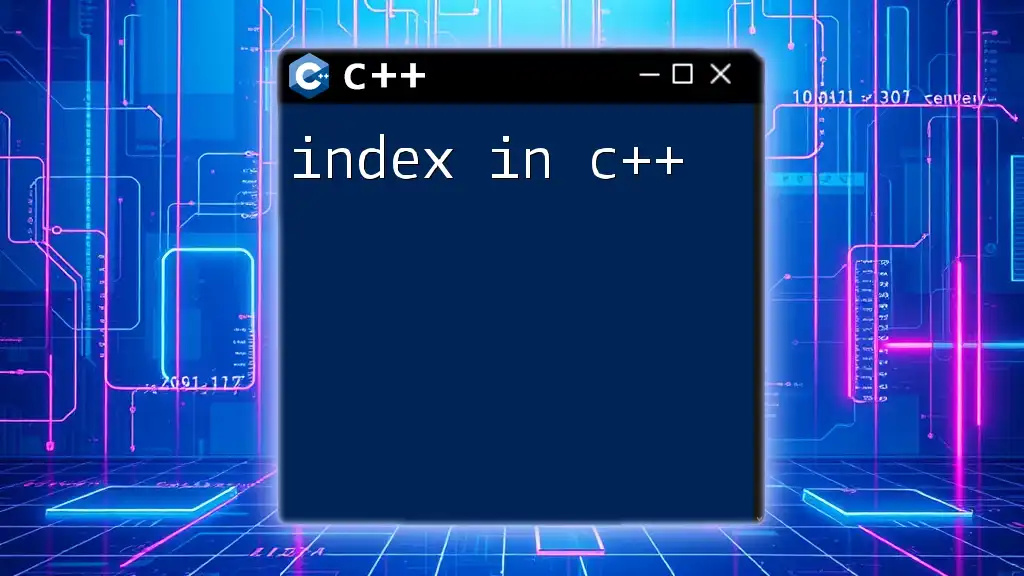
Conclusion
Understanding the exit function in C++ is essential for managing program termination effectively. With a solid grasp on the mechanics of exit, you can enhance not only your program's robustness but also its maintainability. Practicing the use of the exit command alongside familiarization with alternate termination methods leads to more well-structured and efficient C++ applications.
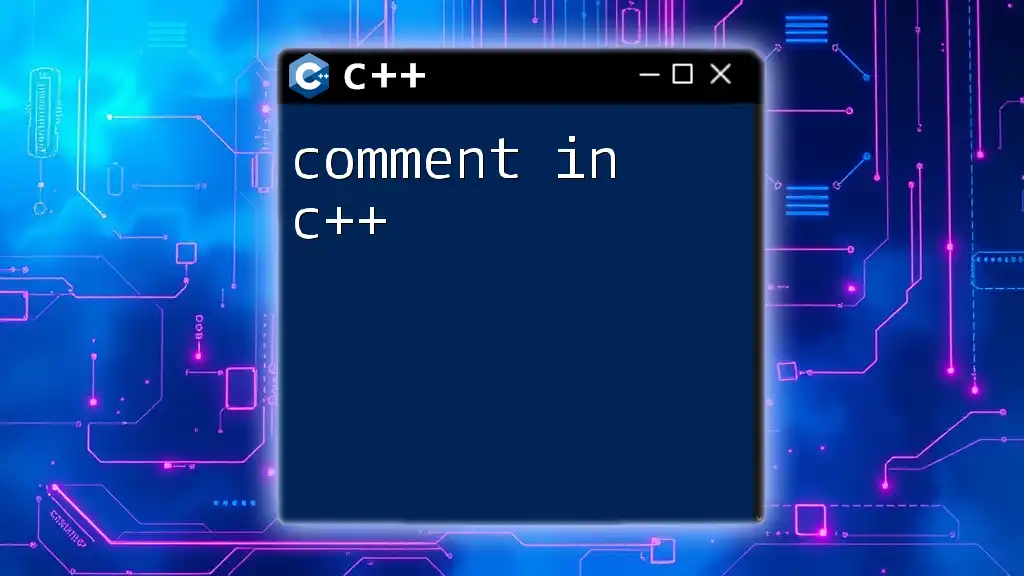
Additional Resources
For more in-depth information about the exit function and related concepts, refer to the official C++ documentation, tutorials, and resources that further explore error handling and program control techniques in C++.