In C++, `exit(1)` is used to terminate a program and indicate that it ended with an error, where the integer value `1` typically signifies an abnormal termination.
Here's a code snippet to illustrate its usage:
#include <iostream>
#include <cstdlib> // for exit()
int main() {
std::cout << "An error has occurred." << std::endl;
exit(1); // Exit with error code 1
return 0; // This line will not be executed
}
Understanding the `exit()` Function
What is `exit()`?
The `exit()` function in C++ is a standard library function defined in the `<cstdlib>` header. It allows a program to terminate its execution after performing necessary cleanup tasks. Using this function plays a crucial role in managing program flow, especially when an error occurs or when a condition demands an immediate stop.
Return Values and Their Meanings
When invoking the `exit()` function, it is important to understand that the parameter you pass (often referred to as the "exit status") conveys different meanings to the operating system or calling process. By convention, an exit status of `0` signifies success, while `exit(1)` (or any non-zero value) indicates an error occurred during execution. This practice is fundamental for error checking in scripts and programs that may rely on the exit status of child processes.
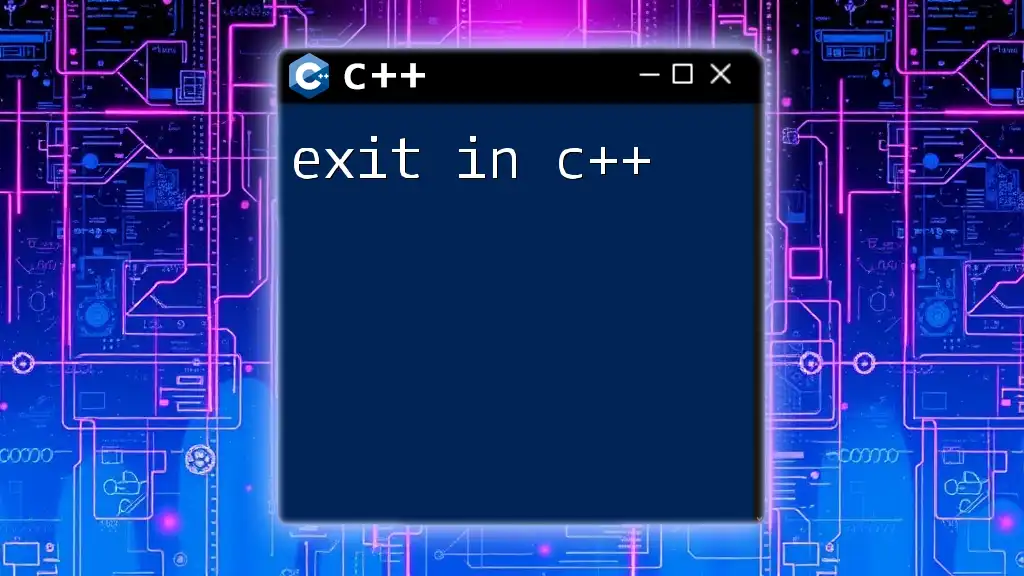
Using `exit(1)` in C++
What Does `exit(1)` Mean?
The call to `exit(1)` is particularly significant. It conveys to the operating system that the program has terminated due to some sort of error. This exit code can be used by the parent process or shell to analyze whether the executed program succeeded or failed and to decide on subsequent actions based on that result.
Syntax of the `exit()` Function
The basic syntax of the `exit()` function is as follows:
void exit(int status);
The parameter `status` can be any integer value, commonly `0` for success or `1` for failure. Here’s a simple example to illustrate this:
#include <cstdlib>
#include <iostream>
int main() {
std::cout << "Exiting with an error!" << std::endl;
exit(1); // Indicates an error occurred
}
In the above snippet, the program outputs a message and then immediately terminates with an exit status of `1`.
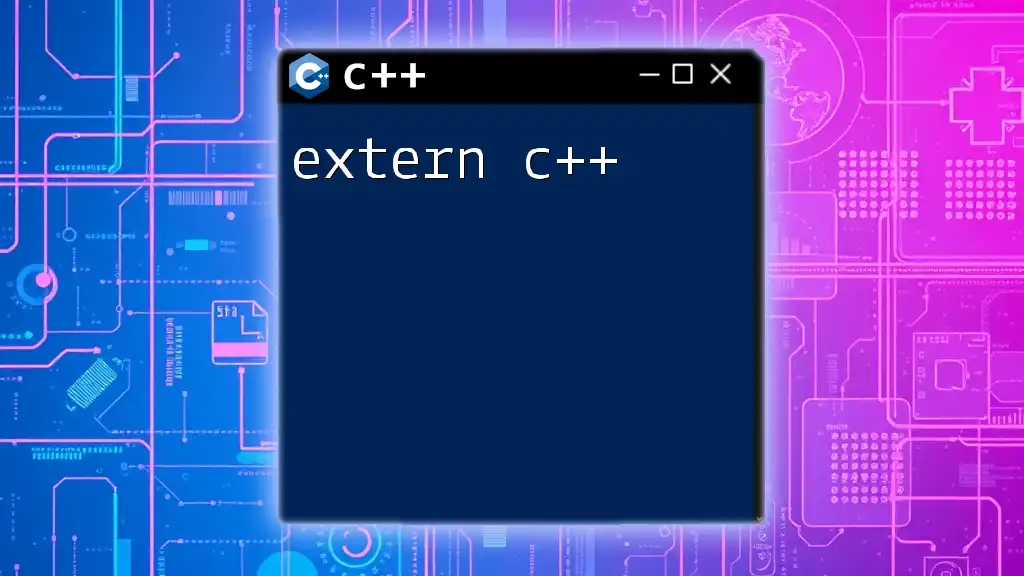
How and When to Use `exit(1)`
Common Scenarios for Using `exit(1)`
There are various situations where you might consider using `exit(1)`, including but not limited to:
-
File Operations: When attempting to open a file, if it fails (for instance if the file doesn’t exist), it’s appropriate to call `exit(1)` to inform the user or log that a critical operation could not be completed.
-
Invalid Inputs: When your program requires certain types of input from a user, and they don’t provide valid data, terminating the program with an error exit code is often necessary.
Best Practices for Using `exit(1)`
While using `exit(1)` is straightforward, there are some best practices to consider:
-
Avoid Abrupt Termination: Before calling `exit(1)`, ensure that any necessary cleanup actions are performed. This may include flushing buffers, closing files, or freeing memory to prevent resource leaks.
-
Informative Messages: Always output an informative error message prior to calling `exit(1)`. This feedback will help users understand why the program has terminated unexpectedly.
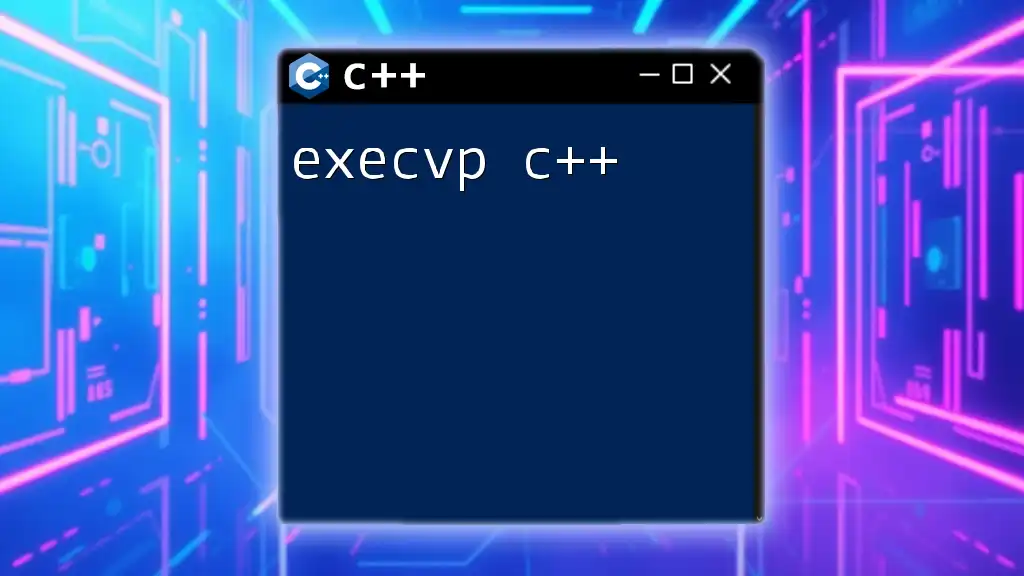
Example Use Cases of `exit(1)`
Simple Example of Error Handling
One common application of `exit(1)` is error handling in file operations. Consider the case of a program trying to open a file that doesn’t exist:
#include <fstream>
#include <cstdlib>
int main() {
std::ifstream file("non_existent_file.txt");
if (!file) {
std::cerr << "Error opening file. Exiting with code 1." << std::endl;
exit(1);
}
// Process file
}
In this example, if the file cannot be opened, an error message is printed to standard error, and the program terminates with `exit(1)`, signaling a failure to the operating environment.
Complex Example: Input Validation
Another scenario where `exit(1)` is commonly used is when validating user input. Here, we can examine a program where the user must provide a positive integer:
#include <iostream>
#include <cstdlib>
int main() {
int userInput;
std::cout << "Enter a positive number: ";
std::cin >> userInput;
if (userInput < 0) {
std::cerr << "Invalid input, exiting with code 1." << std::endl;
exit(1);
}
std::cout << "You entered: " << userInput << std::endl;
}
The above snippet takes user input and validates it. If the input is negative, the program informs the user and quits with an exit code of `1`.
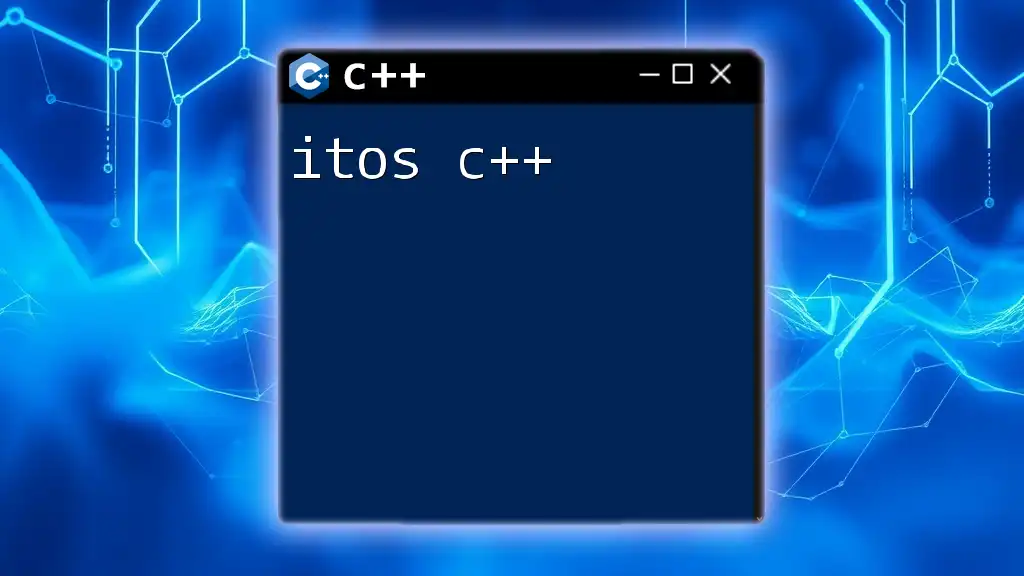
Alternatives to `exit(1)`
Using `return` Statements
While `exit(1)` is useful, you can also opt to use return statements in your `main()` function. Returning a non-zero value achieves the same goal of signaling an error:
int main() {
if (error_condition) {
std::cout << "Error occurred." << std::endl;
return 1; // Equivalent exit code for the program
}
return 0; // Successful completion
}
This method is often preferred in larger applications where you want to preserve the context of the call stack.
Using Exceptions for Error Handling
Alternatively, employing exceptions can provide a more robust error handling framework. Instead of terminating the program immediately with `exit(1)`, you can throw an exception:
#include <iostream>
#include <stdexcept>
int main() {
try {
throw std::runtime_error("An error occurred.");
} catch (const std::exception& e) {
std::cerr << e.what() << std::endl;
exit(1);
}
}
This approach allows for better control over error handling, as it lets you catch and respond to exceptions without immediately leaving the current scope.
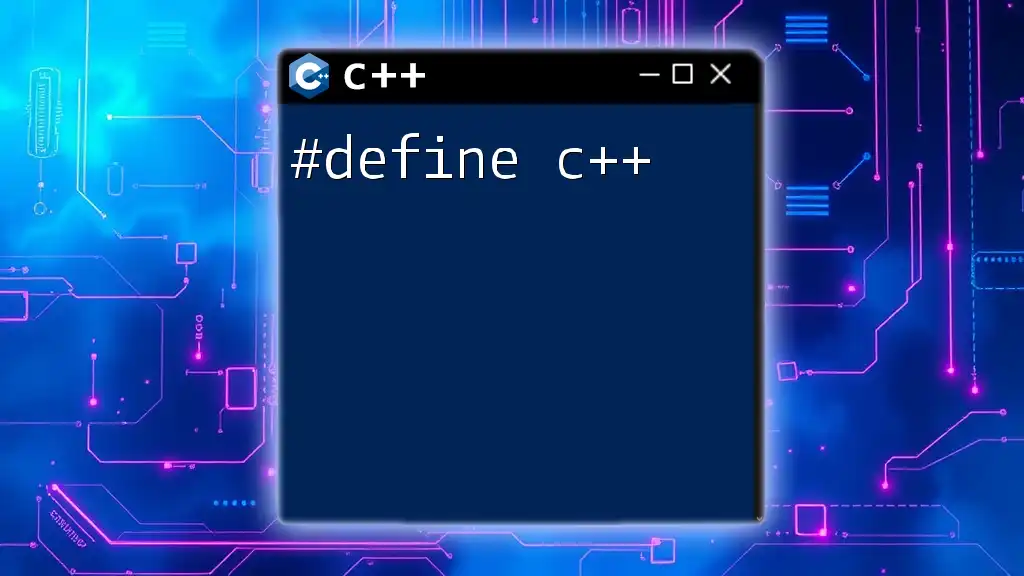
Conclusion
In summary, the `exit(1)` command is a vital tool in C++ for conveying error states when a program encounters issues. Mastering its usage helps you maintain control over program exit behavior, ensuring that clear and informative exit statuses are communicated to the calling environment. As you build your skills in C++, embracing best practices for error management will elevate the quality and reliability of your applications. For further exploration, consider delving into the rich world of error handling techniques and practices available in C++.