In C++, the `itos` function is commonly used to convert integer values to their corresponding string representations.
#include <iostream>
#include <string>
std::string itos(int value) {
return std::to_string(value);
}
int main() {
int num = 42;
std::string strNum = itos(num);
std::cout << "The string representation is: " << strNum << std::endl;
return 0;
}
Understanding `itos`
`itos` is a commonly referred term for a function that converts an integer into a string representation in C++. The ability to transform an integer into a string is crucial for various programming tasks, such as concatenating numbers with text, displaying numerical results in user interfaces, or generating formatted output.
Importance of Converting Integers to Strings
The necessity of converting integers to strings arises frequently in programming projects. Whether you're handling user input, creating dynamic messages, or writing data to files, string conversion is an essential operation. This conversion allows you to utilize integers in contexts that require text, improving the versatility of your applications.
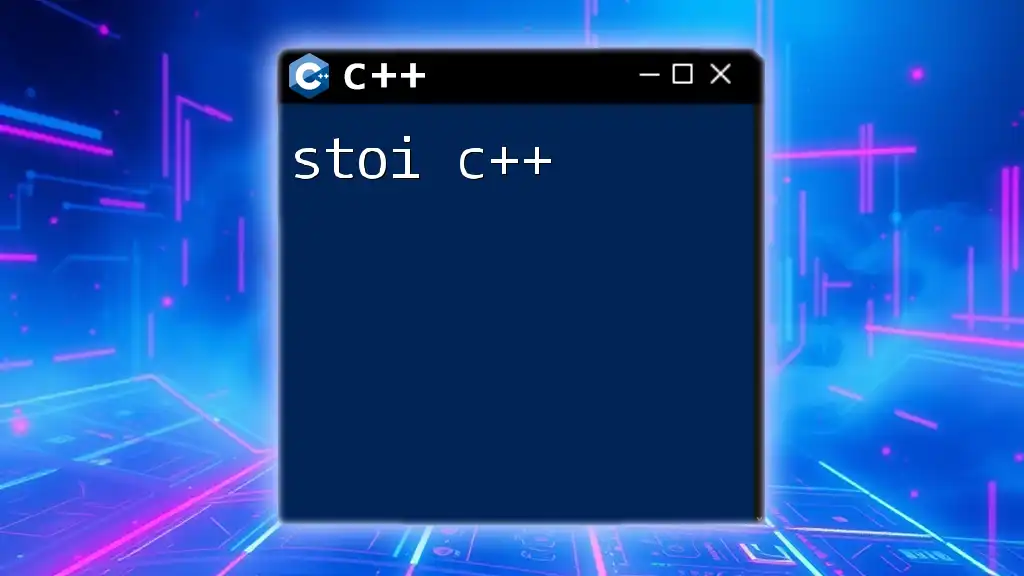
Basics of String Conversion
Overview of Built-in Functions
C++ provides several built-in methods for converting integers to strings. Among these, `std::to_string` stands out as a popular choice for its simplicity and reliability. However, understanding how custom implementations work can deepen your knowledge of C++ and boost your programming skills.
The `std::to_string` Function
The `std::to_string` function is a straightforward method for converting an integer to a string. Here’s the syntax:
std::string to_string(int value);
Example: Converting an Integer to String
#include <iostream>
#include <string>
int main() {
int num = 42;
std::string str = std::to_string(num);
std::cout << "The string representation is: " << str << std::endl;
return 0;
}
This simple code will yield the output:
The string representation is: 42
Creating a Custom `itos` Function
While `std::to_string` is convenient, creating a custom `itos` function can enhance your understanding of string manipulation in C++. However, a custom implementation comes with its own challenges—including accurately handling corner cases like negative numbers, zero, and integer overflows.
Code Snippet: Basic Custom `itos`
Here’s a basic example of a custom `itos` function using `std::to_string`:
std::string itos(int value) {
return std::to_string(value);
}
Explanation of the Code: This function leverages the existing `std::to_string` functionality to convert the integer `value` into its string representation seamlessly. However, it's crucial to understand that while this may be sufficient for many cases, robustness in code is always preferable.
Handling Edge Cases in `itos`
Negative Numbers
You need to modify the function to account for negative integers. Here’s how you could do it:
std::string itos(int value) {
if (value < 0) {
return "-" + itos(-value);
}
// Rest of the conversion logic
}
This implementation first checks if the given `value` is negative. If so, it prepends a hyphen to the string result of its absolute value.
Zero and Special Cases
Additionally, you should handle the case of zero explicitly:
std::string itos(int value) {
if (value == 0) return "0";
// Rest of the logic
}
This guarantees your `itos` function can accurately return "0" as its string representation.
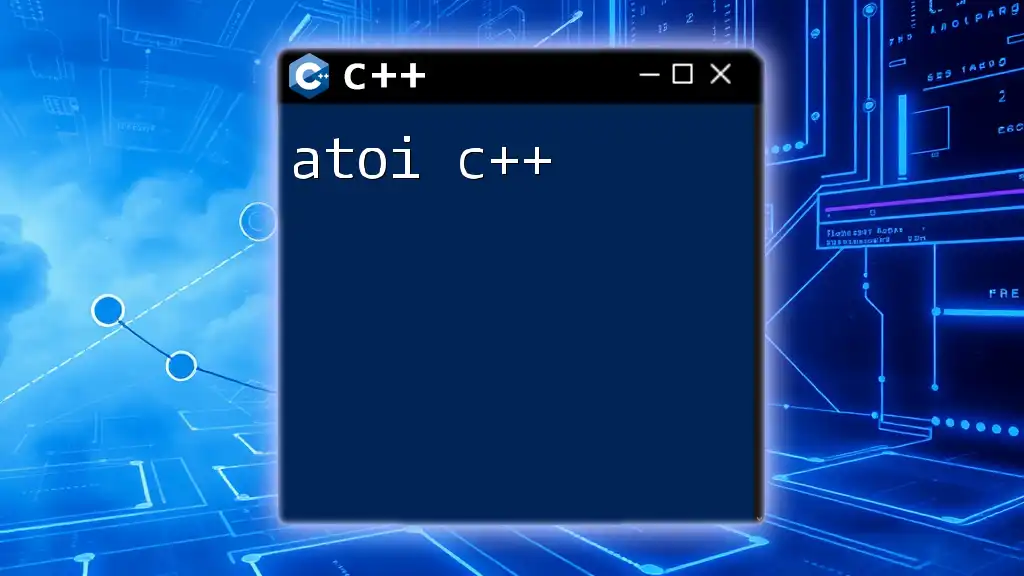
Advanced Features of `itos`
Formatting Options
In certain applications, you may wish to format integers with additional specifications, such as adding leading zeros. Here’s how you can enhance the `itos` function:
#include <iomanip>
#include <sstream>
std::string itos(int value, int width) {
std::ostringstream oss;
oss << std::setw(width) << std::setfill('0') << value;
return oss.str();
}
Usage: If you call `itos(42, 5)`, it will output "00042". This feature is especially useful in contexts like generating formatted output for tables or log files.
Converting Other Integer Types
You can also extend your `itos` functionality to accommodate different integer types, such as `long` and `short`.
std::string itos(long value);
std::string itos(short value);
These variations will allow you to maintain flexibility regardless of your integer type.
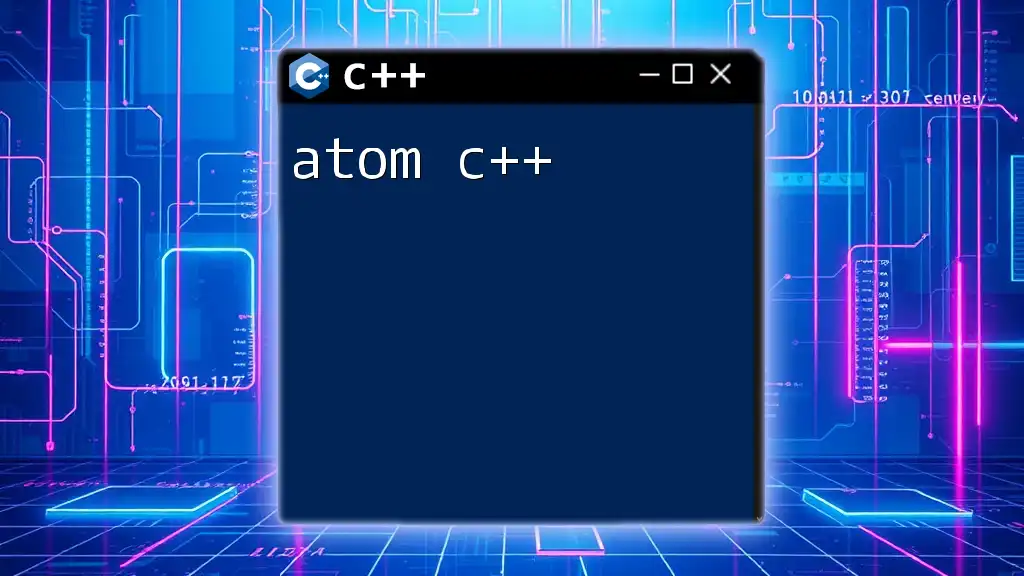
Performance Considerations
Efficiency of Different Methods
When developing performance-sensitive applications, it's essential to consider the efficiency of string conversion methods. A benchmark comparing your custom `itos` function against `std::to_string` can help you discern which method better suits your specific use case.
Memory Management
Understanding the memory implications of conversions is crucial. String conversion can allocate memory dynamically, so it's vital to write efficient code, especially in loops or frequently called functions.
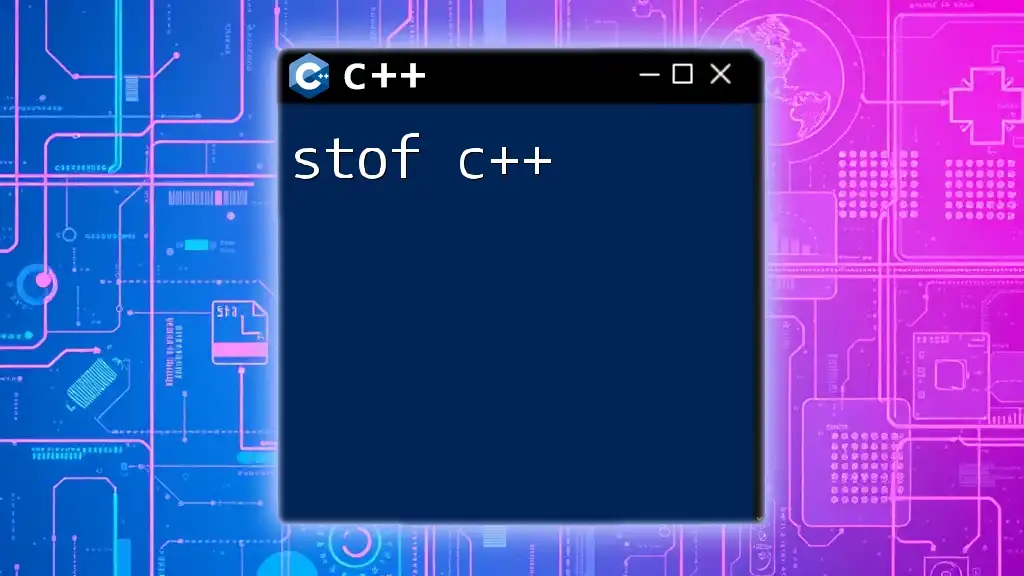
Common Mistakes and Troubleshooting
Debugging the `itos` Implementation
Even experienced programmers can encounter errors when implementing the `itos` function. Some frequent errors include incorrect handling of negative integers or faulty logic that fails to account for special cases. Implementing thorough testing and debugging techniques will help you catch and correct these issues, ensuring the reliability of your function.
FAQs about `itos`
Q: Can I use `itos` for real-time applications?
A: Yes, but ensure you profile your function to confirm that its performance meets your application's requirements.
Q: Is `std::to_string` less efficient?
A: Not necessarily; the efficiency may depend on context. Evaluate performance according to your specific needs.
Q: How do I handle conversion errors?
A: Ensure that your `itos` function encapsulates error handling, particularly when managing divergent integer types or edge cases.
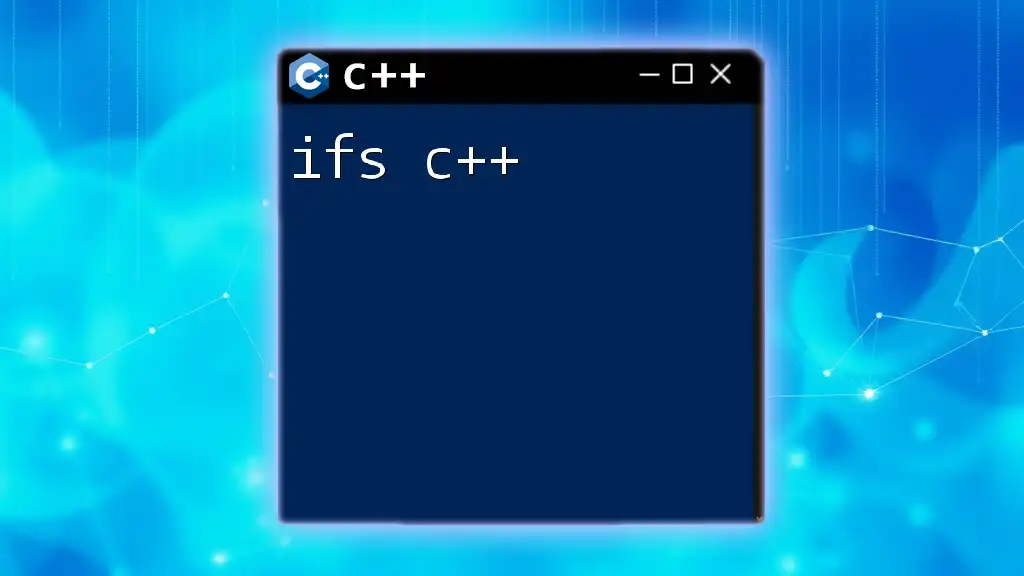
Conclusion
In summary, the `itos` function in C++ plays a significant role in converting integers to strings. Understanding its implementations and applications equips you with valuable programming tools. Your journey doesn't have to end here; I encourage you to dive deeper and implement your own variations of `itos`, playing with edge cases and optimizing for performance.
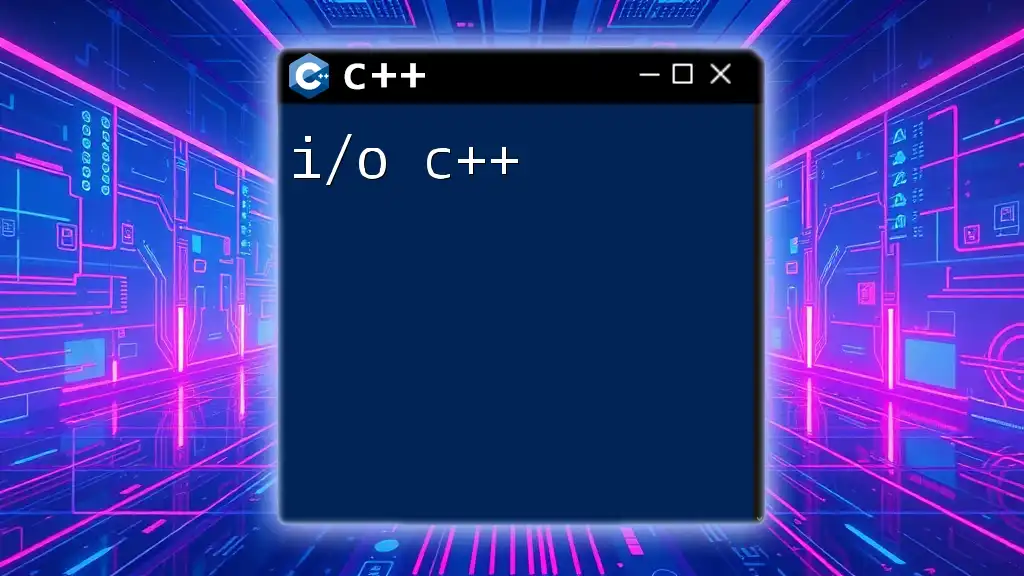
Additional Resources
To enhance your learning, consider exploring additional articles on advanced string manipulation, C++ programming best practices, and community forums where you can engage with other developers. Continuous learning is the key to mastering C++ and its vast capabilities.