The `goto` statement in C++ provides an unstructured jump from the `goto` to a labeled statement within the same function, which can create less readable code but allows for quick exits from loops or error handling.
Here's a simple code snippet demonstrating its use:
#include <iostream>
using namespace std;
int main() {
int num = 0;
start: // label
cout << "Enter a positive number (0 to exit): ";
cin >> num;
if (num != 0) {
cout << "You entered: " << num << endl;
goto start; // jumps back to the label 'start'
}
return 0;
}
Understanding the `goto` Statement in C++
Definition of `goto`
The `goto` statement in C++ provides a way to jump from one part of your program to another, allowing for control flow manipulation. Despite its simplicity, it often sparks debate among programmers regarding its usage and appropriateness. The statement can be found in many programming languages, including older languages like Assembly and C, as well as modern languages like C++.
Importance of Control Flow in Programming
Control flow structures are essential for creating logical and well-organized programs. They dictate the execution sequence of code and help manage complex decision-making processes. While C++ offers various constructs—like loops (`for`, `while`), conditionals (`if`, `switch`), and functions—to structure control flow, `goto` serves as a tool for scenarios where these structures may not effectively handle the desired logic.
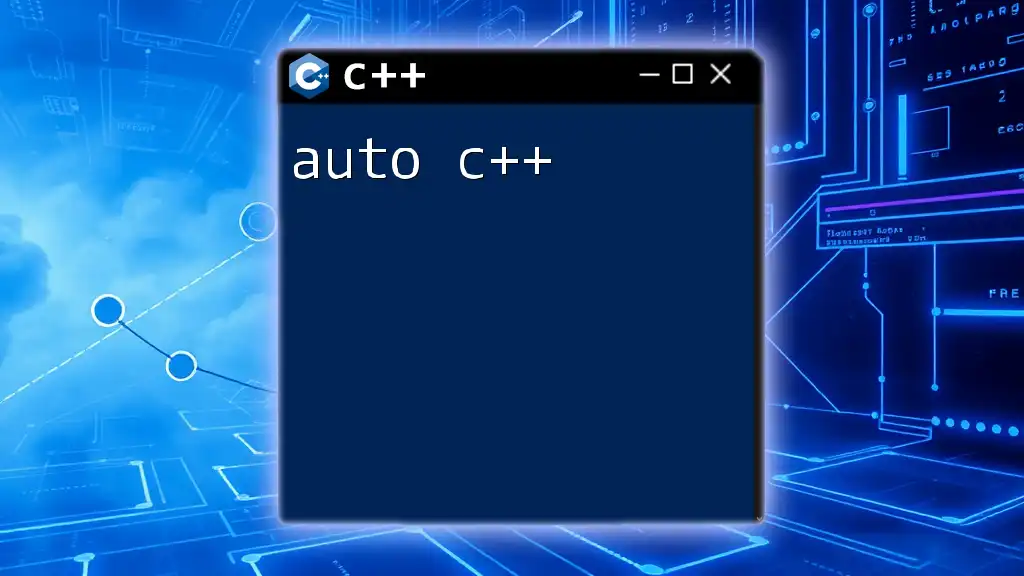
Syntax of the `goto` Statement
General Syntax
The general format of the `goto` statement consists of the following:
goto label;
...
label:
// code to execute
This syntax defines how you jump to a specific line designated by a label. Labels are strings of characters followed by a colon and should not clash with any identifiers in your program.
Creating and Using Labels
Labels are defined using a combination of letters, digits, and underscores, ending with a colon. For instance, a typical label might look like `step1:`. While it is easy to create labels, ensure they are descriptive to maintain code readability.
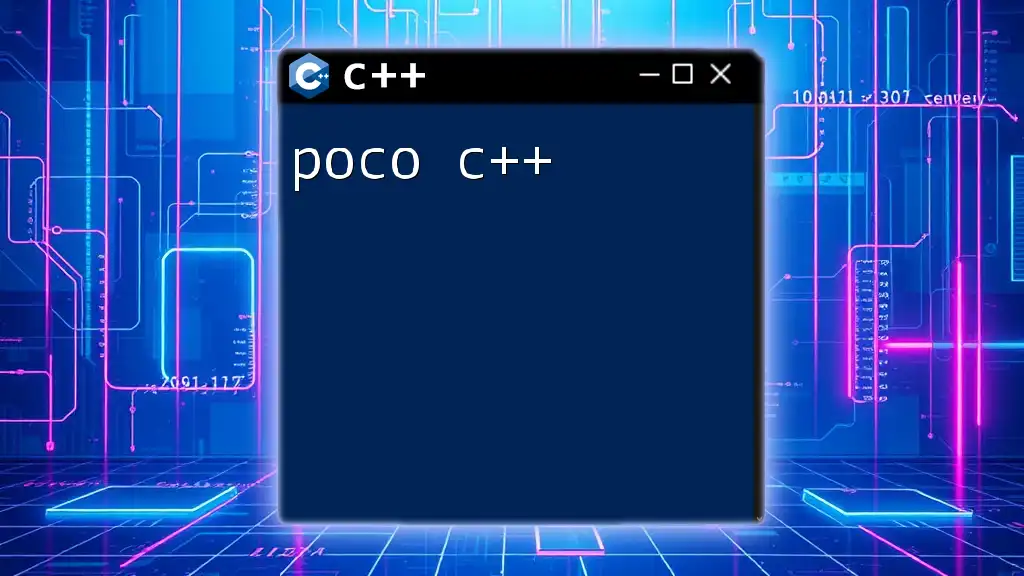
Use Cases of `goto`
Simplifying Complex Control Flow
While some argue that `goto` adds unnecessary complexity, it can actually reduce it in certain situations. For example, when dealing with convoluted logic requiring multiple exits from nested loops, `goto` can simplify the code structure.
Code Snippet Demonstrating Simplification of a Nested Loop
Consider a scenario where you need to exit multiple nested loops based on a specific condition:
#include <iostream>
int main() {
for (int i = 0; i < 5; ++i) {
for (int j = 0; j < 5; ++j) {
if (i == 2 && j == 2) {
goto exit_loops;
}
}
}
exit_loops:
std::cout << "Exited loops.\n";
return 0;
}
In this snippet, if `i` equals `2` and `j` equals `2`, the program will immediately jump to the label `exit_loops`, exiting both loops.
Exiting Multiple Nested Loops
`goto` can be particularly useful for breaking out of deeply nested structures. While structured programming proponents may prefer using function calls or break statements, `goto` simplifies the exit process when many levels of loops are involved.
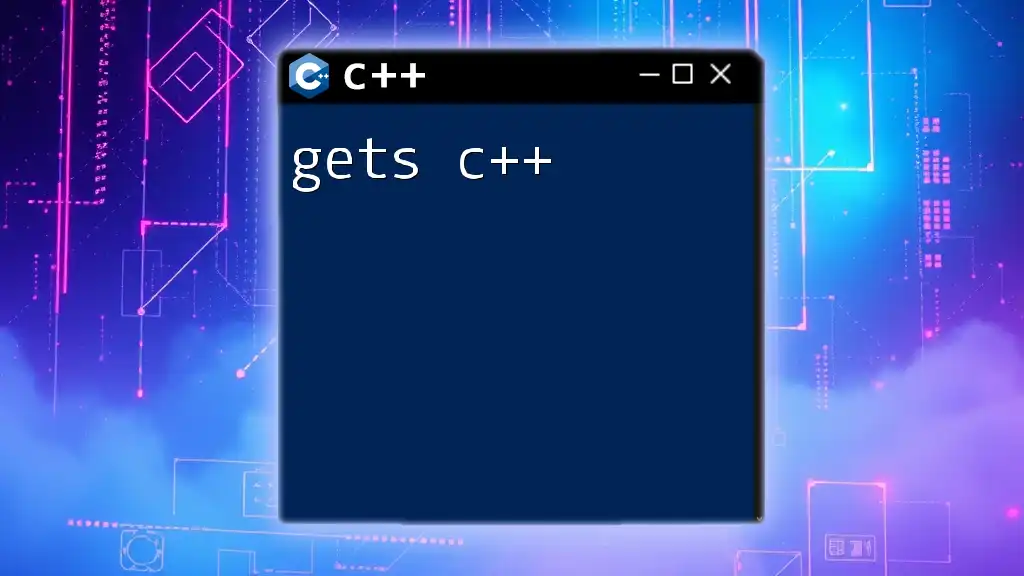
Advantages of Using `goto`
Readability in Certain Contexts
In specific programming scenarios, `goto` can enhance readability. For instance, when handling error management in resource allocation, utilizing `goto` allows for a quick exit to error handling without embedding excessive conditional logic.
Real-Life Example
Imagine cleaning up resources in a multi-level function; instead of placing cleanup code across various conditional branches, a single `goto` to an error label can make the code cleaner:
bool allocateResources() {
// Resource allocation...
if (failure) {
goto cleanup;
}
// More code...
cleanup:
// Cleanup logic...
return success;
}
Performance Considerations
In some cases, `goto` may yield better performance than structured alternatives due to reduced overhead for checks within loops. However, modern compilers often optimize such code effectively, rendering performance differences insignificant in many contexts.
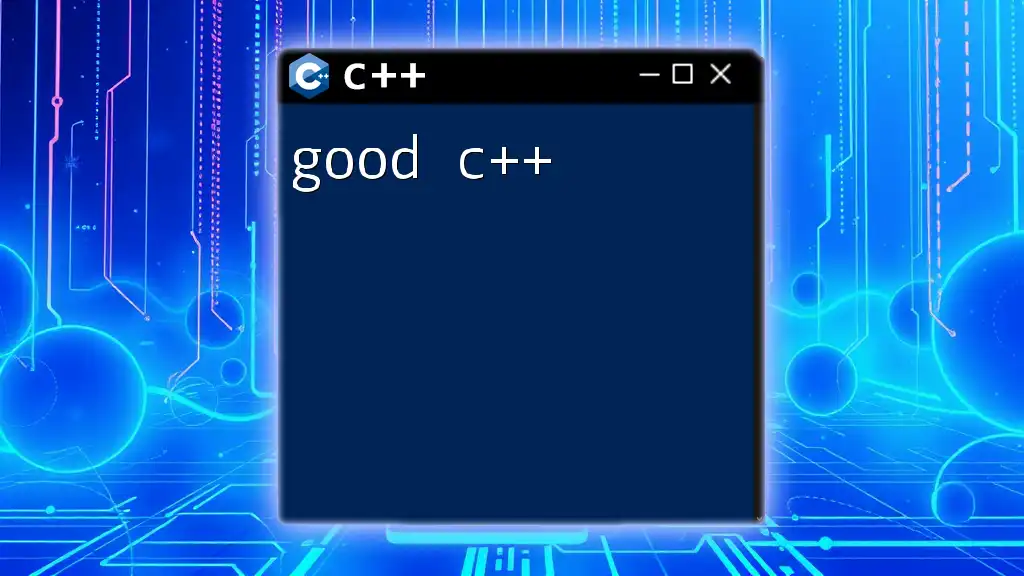
Criticisms and Risks of Using `goto`
Code Maintainability
One of the primary arguments against using `goto` is its potential to create “spaghetti code”—code that is tangled and difficult to follow. It can make debugging more challenging as tracking the program's flow becomes less clear.
Example of `goto` Creating Spaghetti Code
Imagine a complex function where multiple `goto`s jump around erratically. When maintaining or debugging such code, understanding the logic requires considerable effort, often resulting in more errors.
Alternatives to `goto`
Loops and Conditional Statements
Structured programming encourages using loops and conditionals instead of `goto`. These constructs provide a logical flow that makes programs more understandable.
Function Calls
Refactoring code into functions is a robust alternative to using `goto`. Functions encapsulate logic, making code modular and easier to test.
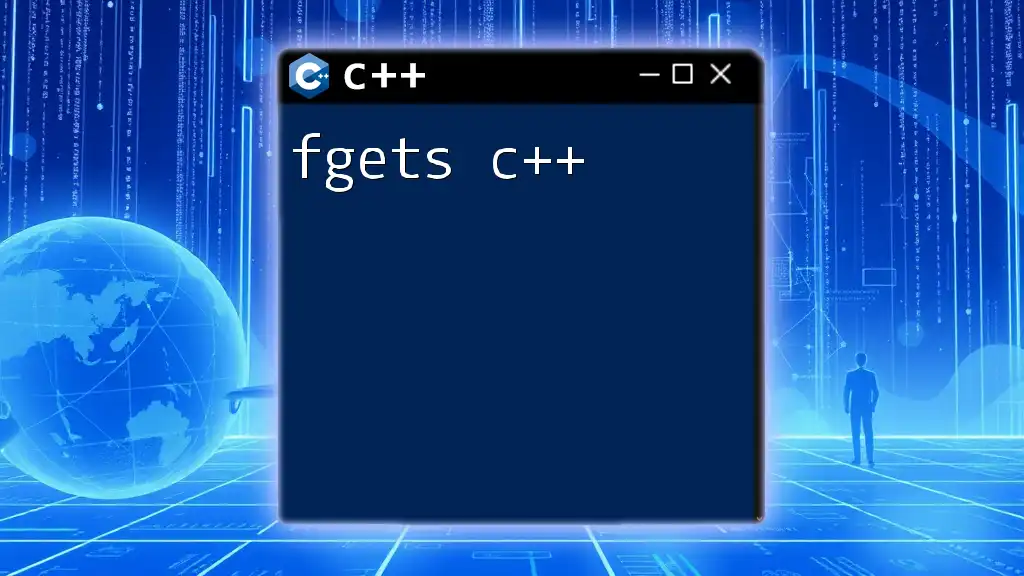
Best Practices for Using `goto`
When to Use `goto`
Despite its controversies, there are specific situations where using `goto` is justified, such as:
- Simplifying error handling in tightly controlled environments.
- Breaking out of multiple nested loops efficiently.
- Providing clarity in certain complex algorithms.
When to Avoid `goto`
To maintain the integrity of your codebase, avoid using `goto` when:
- The code can be effectively managed using loops, conditionals, or function calls.
- There's a risk of creating tangled control flows that impede readability.
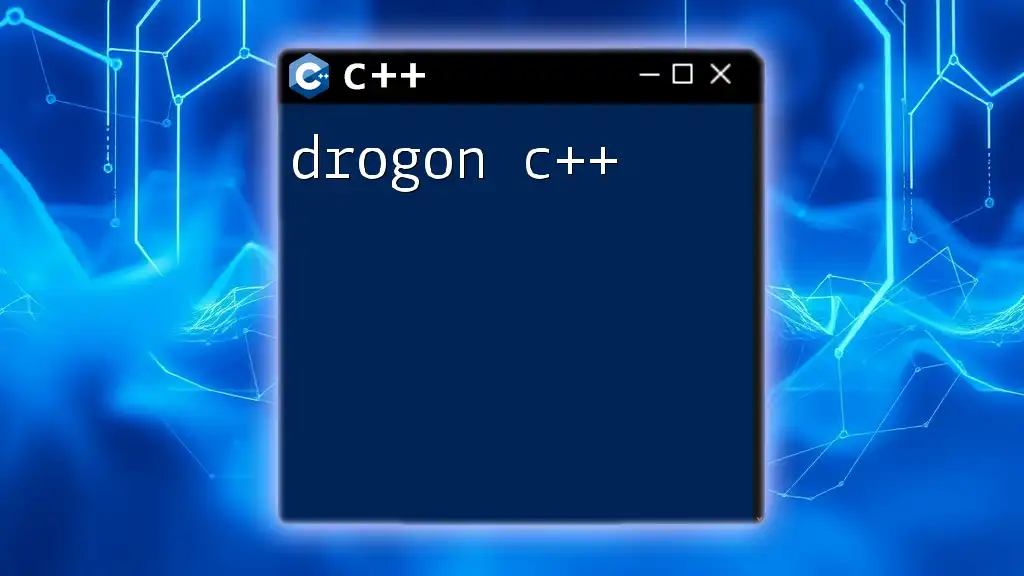
Conclusion
Summary of Key Points
The `goto` statement in C++ offers both benefits and drawbacks. While it can simplify specific control flows and enhance performance in certain cases, its misuse can lead to maintenance challenges and convoluted logic.
Final Thoughts
Using `goto` responsibly is key; developers must weigh its usage against structured programming principles to ensure code remains clean, maintainable, and efficient. Emphasizing best practices when dealing with control flows can contribute to stronger programming habits.
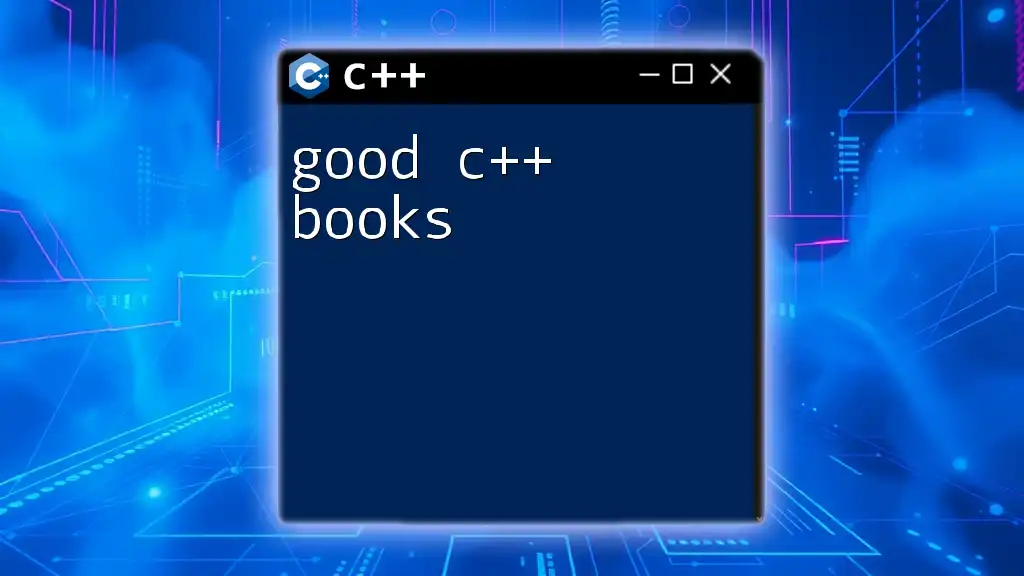
Further Reading and Resources
For those eager to explore C++ control flow further, consider examining textbooks that focus on algorithms and structure programming. Additionally, engaging with online forums can foster discussion around effective use of `goto` and alternatives, broadening your understanding of C++ programming techniques.