The `auto` keyword in C++ allows for automatic type deduction, making the code cleaner and easier to read by letting the compiler infer the variable's type based on its initializer.
auto x = 42; // x is deduced to be of type int
auto name = "John"; // name is deduced to be of type const char*
Understanding `auto`
What is `auto`?
The `auto` keyword in C++ is a powerful feature introduced in C++11 that enables automatic type deduction. Rather than explicitly specifying the type of a variable, the compiler infers the type based on the initializer. This can significantly reduce verbosity and enhance clarity in your code.
Why Use `auto`?
Using `auto` offers several compelling advantages:
- Simplifies Code: It reduces boilerplate code, allowing programmers to focus on the logic rather than the types.
- Enhances Maintainability: Because types are inferred, changes to the type of a variable can be made without requiring type updates throughout the code.
- Handles Complex Types: With `auto`, working with complicated data structures, such as those found in the Standard Template Library (STL), becomes more manageable.
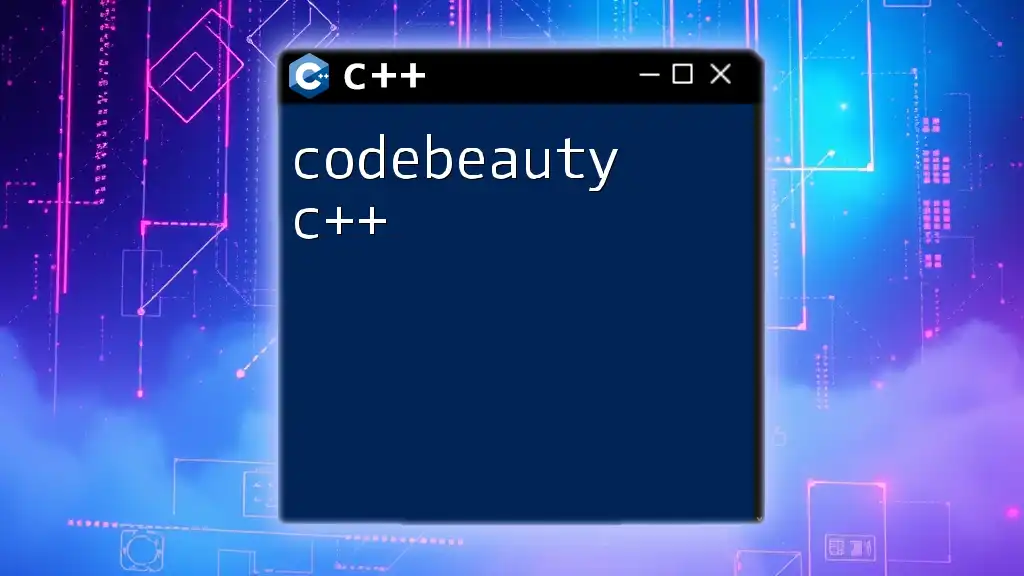
Understanding Type Inference
How Type Inference Works
When you declare a variable using `auto`, the C++ compiler examines the expression assigned to the variable and determines its type at compile-time. This process is called type deduction. It’s important to note that type inference is context-sensitive; the type deduced depends entirely on what the variable is initialized with.
Examples of Type Inference
Consider the following examples that illustrate how `auto` works in practice:
- Simple variable declaration:
auto x = 10; // x is inferred as int
- Using `auto` with different data types:
auto y = 5.5; // y is inferred as double
auto z = "Hello"; // z is inferred as const char*
In these examples, the compiler determines the most appropriate types based on the assigned values.
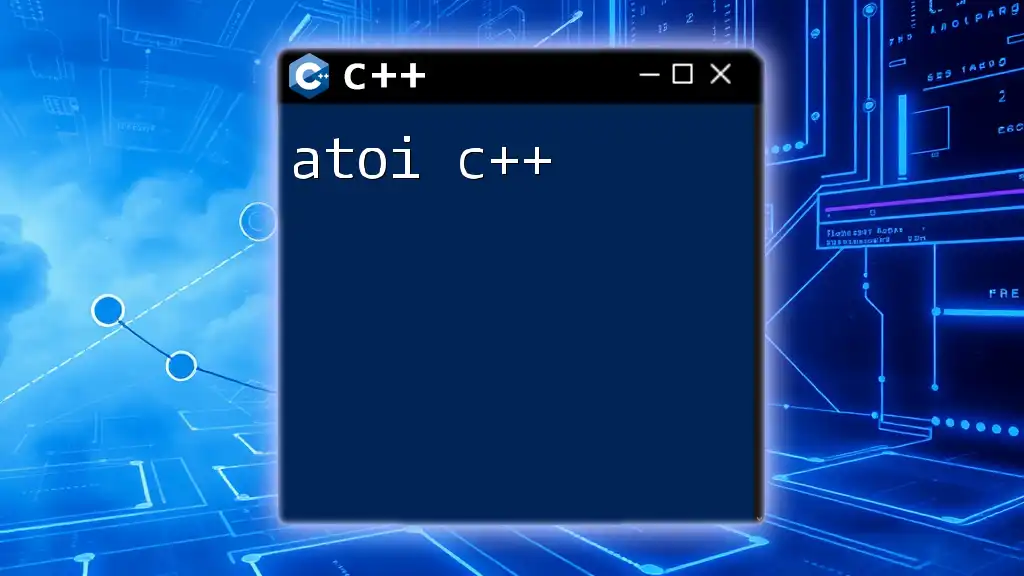
Practical Use Cases for `auto`
Simplifying STL Code
One of the primary areas where `auto` shines is in the context of the Standard Template Library (STL). For example, consider the following usage of `auto` with a lambda function and the `std::for_each` algorithm:
#include <vector>
#include <algorithm>
std::vector<int> vec = {1, 2, 3, 4, 5};
std::for_each(vec.begin(), vec.end(), [](auto& n) { std::cout << n << ' '; });
In this snippet, `auto` allows the lambda to accept any data type, enhancing flexibility while maintaining readability.
Working with Iterators
Using `auto` with iterators is another effective use case. It eliminates the tedious syntax often required for iterator types:
std::vector<int> vec = {1, 2, 3, 4, 5};
for (auto it = vec.begin(); it != vec.end(); ++it) {
std::cout << *it << ' ';
}
Here, `auto` automatically determines the correct type of the iterator without requiring the programmer to specify the exact type.
Dealing with Containers and Collections
When iterating over STL collections like `std::map`, `auto` again proves its utility in enhancing readability:
#include <map>
std::map<std::string, int> scores = {{"Alice", 90}, {"Bob", 85}};
for (auto& pair : scores) {
std::cout << pair.first << ": " << pair.second << '\n';
}
The use of `auto&` allows for efficient access to map elements without extra overhead, maintaining clarity and performance.
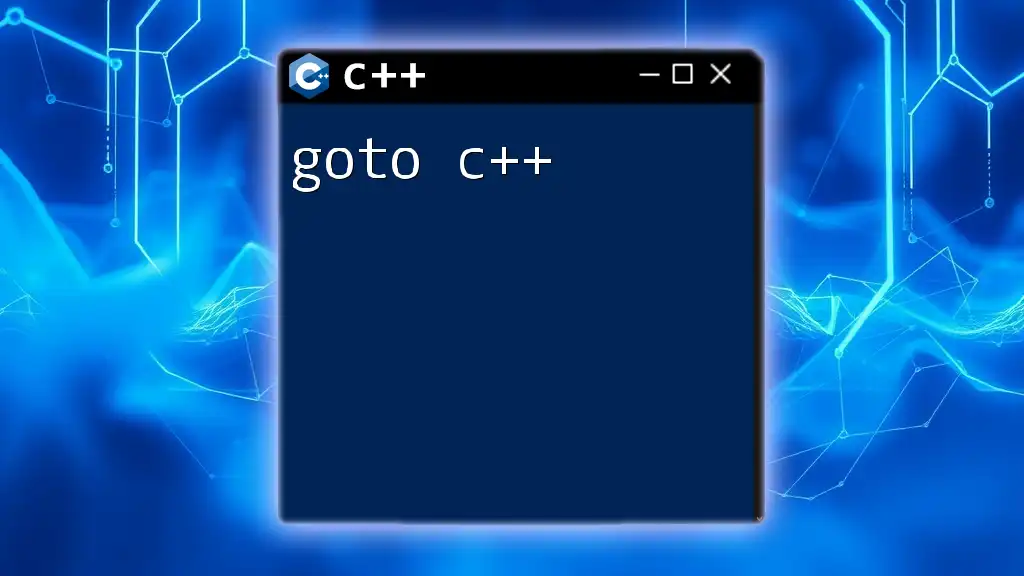
Limitations and Considerations
When Not to Use `auto`
While `auto` can greatly streamline code, there are circumstances where its use may lead to confusion:
- Ambiguity: In cases where the initializer can yield multiple possible types, the deduced type may not align with expectations.
- Loss of Type Information: Using `auto` might obscure the type of a variable, making it harder to understand the code's intention.
For example:
const auto value = 10; // value is inferred as const int
In scenarios involving `const` or references, it's crucial to be explicit about the type to avoid unintended consequences.
Impact on Code Clarity
Using `auto` can sometimes lead to ambiguity. It’s essential to strike a balance; while brevity is a strength, clarity is paramount. In cases where readability may suffer, explicitly declaring the type is recommended.
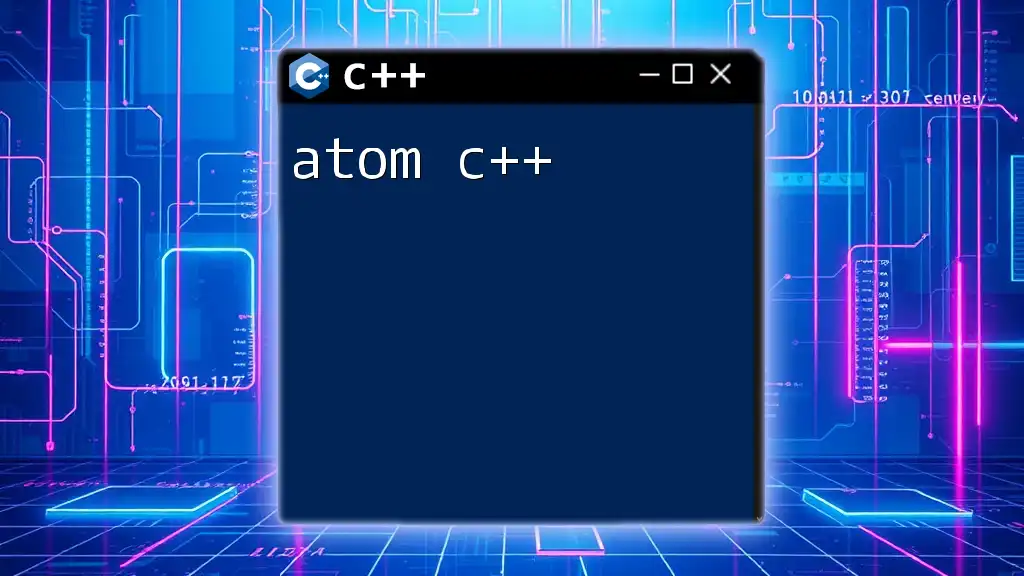
Advanced Usage of `auto`
Declaring Function Return Types
Another sophisticated use of `auto` lies in function return types, particularly for achieving generic programming:
auto add(int a, int b) {
return a + b; // inferred as int
}
This simplifies function signatures and enhances flexibility, particularly when dealing with templates or overloading.
Lambda Expressions with `auto`
`auto` also shines in lambda expressions, enabling function-like constructs to handle various types seamlessly:
auto lambda = [](auto x, auto y) {
return x + y;
};
std::cout << lambda(5, 3.2) << '\n'; // Demonstrates mixed types
Here, `auto` allows the lambda to accept different types for `x` and `y`, showcasing its versatility.
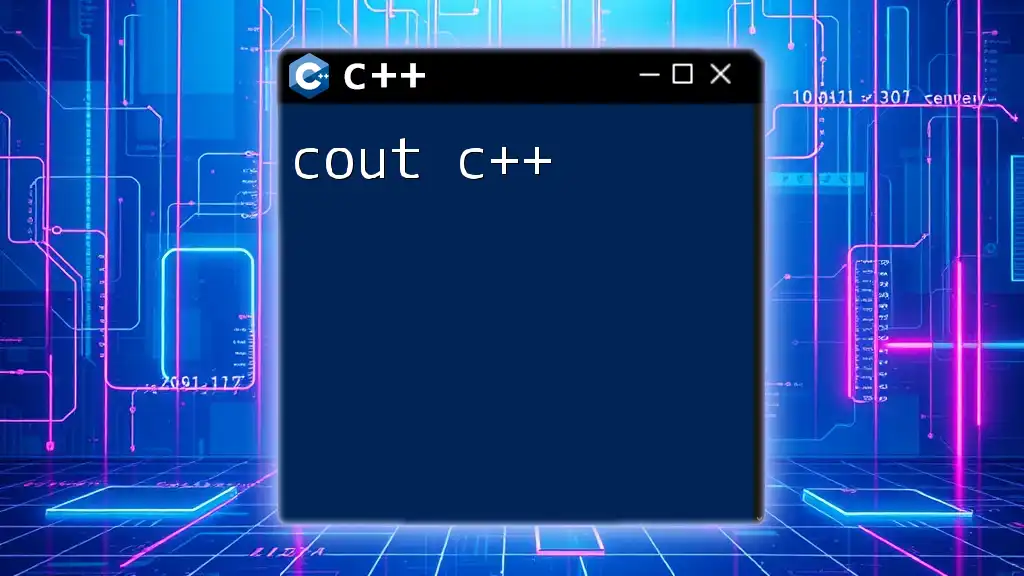
Best Practices for Using `auto`
Code Readability
To ensure that utilizing `auto` enhances rather than detracts from readability, consider these best practices:
- When declaring variables, prefer `auto` for simple types where the type is obvious from the initialization.
- Avoid `auto` when the type is important for understanding code behavior; prefer explicit types in such cases.
Performance Considerations
Using `auto` does not impact performance negatively; however, awareness of const-ness and references is essential. For example:
const auto& large_container = getLargeContainer(); // Using reference
This declaration helps prevent unnecessary copies while maintaining clear intent.
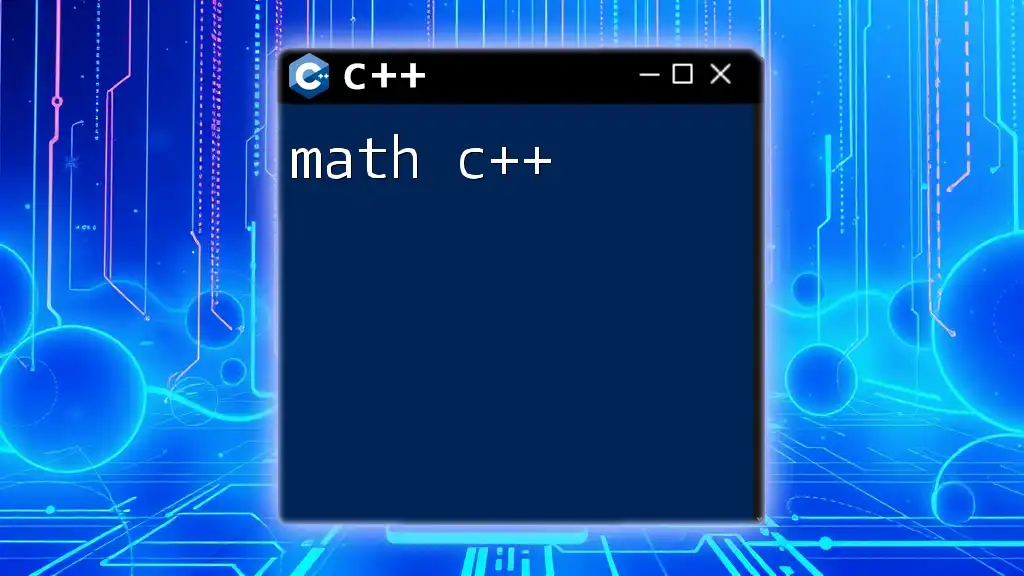
Conclusion
Recap of Key Points
Using `auto c++` effectively can transform how you write code. It allows for flexible, readable, and maintainable code without sacrificing performance.
Final Thoughts
As a programmer, experimenting with `auto` can lead to cleaner and more efficient designs. Challenge yourself to integrate `auto` into your coding practices, sharing your experiences with the community for continuous improvement.
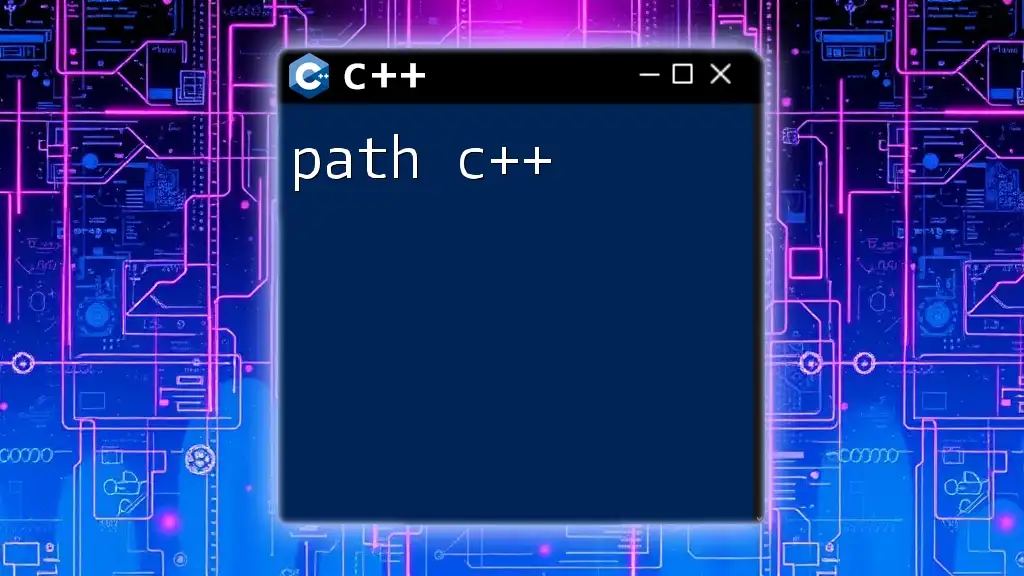
Additional Resources
Recommended Reading
For further exploration of `auto` and C++, consider familiarizing yourself with books, tutorials, and online resources that delve deeper into modern C++ practices.
Community Examples
Engage with fellow developers by sharing your code snippets and use cases of `auto`. This not only enhances learning but also enriches the community's knowledge base.