To permute in C++, you can use the `std::next_permutation` function from the `<algorithm>` header to generate the next lexicographical arrangement of elements in a range. Here's a code snippet demonstrating its usage:
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> nums = {1, 2, 3};
do {
for (int n : nums) {
std::cout << n << " ";
}
std::cout << std::endl;
} while (std::next_permutation(nums.begin(), nums.end()));
return 0;
}
Understanding the Permutation Concept
What is a Permutation?
A permutation refers to an arrangement of all or part of a set of objects, where the order of arrangement is significant. In programming, understanding permutations is crucial for tasks involving order, arrangement, or sequences. For instance, if we have a set of characters, the different ways to arrange those characters would represent various permutations of that set.
It’s important to distinguish permutations from combinations. While a permutation considers the order of items, a combination does not; it simply counts the different groups that can be formed from a set without regard to the order of items.
Applications of Permutations
Permutations find application in various domains:
-
Data Analysis: Permutations assist in sorting data and optimizing search algorithms by identifying different arrangements and sequences.
-
Game Development: In game mechanics, permutations are used to explore all possible arrangements of characters, moves, or outcomes, enhancing gameplay dynamics.
-
Cryptography: In securing data, permutations play a role in creating complex ciphers by rearranging sequences, thus improving security measures.

Basics of C++ Permutations
The Standard Library and Permutation Functions
C++ offers robust tools to handle permutations effectively through the Standard Template Library (STL). The `<algorithm>` header contains powerful functions that simplify the process of generating permutations, chiefly `std::next_permutation` and `std::prev_permutation`. These functions work seamlessly on sequences like arrays or vectors.
Syntax of `std::next_permutation`
The `std::next_permutation` function rearranges the elements of the input sequence into the next lexicographical permutation. Here’s how to use it:
#include <algorithm>
#include <vector>
#include <iostream>
std::vector<int> vec = {1, 2, 3};
std::next_permutation(vec.begin(), vec.end());
This code snippet demonstrates how `std::next_permutation` alters the sequence of `vec` to the next arrangement based on lexicographic order. If `vec` is initially `{1, 2, 3}`, calling this function modifies it to `{1, 3, 2}`, which is the next permutation.
Syntax of `std::prev_permutation`
In contrast, the `std::prev_permutation` function finds the previous lexicographical permutation. The syntax is similar to that of `std::next_permutation`:
std::prev_permutation(vec.begin(), vec.end());
This operation would take our earlier arrangement of `{1, 3, 2}` back to `{1, 2, 3}`. Understanding both functions allows developers to navigate both directions of permutations.

Generating All Permutations
Using `std::generate` and `std::next_permutation`
To generate all possible permutations of a set, you can utilize a loop alongside `std::next_permutation`. Here’s a complete example:
#include <algorithm>
#include <vector>
#include <iostream>
std::vector<int> numbers = {1, 2, 3};
std::sort(numbers.begin(), numbers.end()); // Ensure we start from the smallest permutation
do {
// Print the current permutation
for (int number : numbers) {
std::cout << number << " ";
}
std::cout << std::endl;
} while (std::next_permutation(numbers.begin(), numbers.end()));
In this code, we first sort the vector to ensure we begin with the smallest permutation. The `do-while` loop continues to call `std::next_permutation`, printing each new permutation until all arrangements have been generated. This method is efficient and leverages the STL to handle permutation generation elegantly.
Custom Permutation Logic
For cases where built-in functions are not sufficient, you can implement a recursive algorithm to generate permutations manually. Here’s how you can do this:
void permute(std::vector<int>& nums, int l, int r) {
if (l == r) {
for (int num : nums) std::cout << num << " ";
std::cout << std::endl;
}
else {
for (int i = l; i <= r; i++) {
std::swap(nums[l], nums[i]);
permute(nums, l + 1, r);
std::swap(nums[l], nums[i]); // backtrack
}
}
}
In this recursive function, we swap elements to create different arrangements, exploring all permutations by moving through each index (`l` to `r`). The backtracking mechanism—swapping elements back after yielding a permutation—ensures that every distinct arrangement is explored. This approach provides flexibility and a deep understanding of how permutations can be generated programmatically.

Performance Considerations
Time Complexity of Permutations
It’s essential to consider the performance implications of generating permutations. The time complexity of generating all permutations of `n` elements is O(n!). With increasing `n`, this can lead to performance issues, as the number of permutations grows factorially. For example, 5 elements result in 120 permutations, while 10 elements result in 3,628,800 permutations.
Given this rapid growth, it’s crucial to evaluate whether generating all permutations is necessary for your application. If you only need a few or specific permutations, consider optimizing your logic accordingly.
Optimizing Permutation Functions
To improve performance when working with permutations, consider the following strategies:
-
Use of Efficient Data Structures: Employ arrays or vectors that allow for quick access and modification.
-
Iterative vs. Recursive Approaches: While recursive approaches are often clearer, they can consume more stack space and result in higher overhead. Iteratively generating permutations can sometimes yield better performance.
-
Memoization Techniques: If certain permutations are computed multiple times (as with overlapping subproblems), storing these results can significantly enhance performance.

Conclusion
In this guide, we explored the concept of permutations in C++ using the powerful tools provided by the STL. With a clear understanding of functions like `std::next_permutation` and `std::prev_permutation`, alongside methods for generating all permutations, programmers can effectively utilize permutations in their computational tasks.
We encourage you to experiment with generating permutations in C++ and apply the concepts discussed here to solve real-world problems. Engaging with permutations not only enhances your programming skills but also provides a fundamental understanding applicable across various domains.

Additional Resources
For further exploration, consider diving into the official C++ documentation for the `<algorithm>` library and seeking out online courses or textbooks that provide a deeper understanding of combinatorial algorithms and their applications in software development.
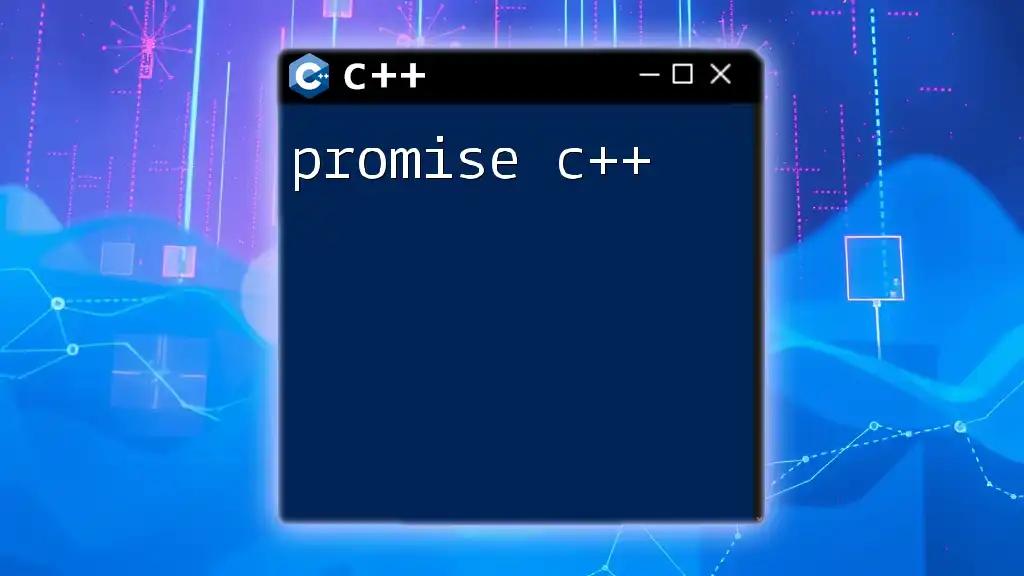
Call to Action
We’d love to hear about your experiences with permutations in C++. Share your thoughts, challenges, and successes in the comments! Joining our community will provide you with additional insights and opportunities for collaborative learning.