In C++, properties can be implemented using accessor (getter) and mutator (setter) methods to control access to class attributes in a concise manner.
class PropertyExample {
private:
int value;
public:
int getValue() const { return value; }
void setValue(int v) { value = v; }
};
Understanding Properties in C++
What is a Property?
In programming, a property is a special kind of class member that combines the functionality of an attribute with controlled access and validation. Properties usually consist of a getter and a setter method, which allow you to retrieve and modify the value respectively. This encapsulation promotes data integrity, ensuring that external codes cannot directly manipulate sensitive components of a class without going through these controlled interfaces.
Why Use Properties in C++?
Using properties in your C++ classes provides several key advantages over traditional public attributes:
- Encapsulation: Properties allow you to hide the internal representation of a class, exposing only the data that should be accessible.
- Validation: Setting properties through a setter method means you can validate the data before assigning it, preventing inappropriate or erroneous values.
- Modification Agility: If you ever need to change how a property works (e.g., adding logging, modifying value constraints), you can do this without changing the class interface.
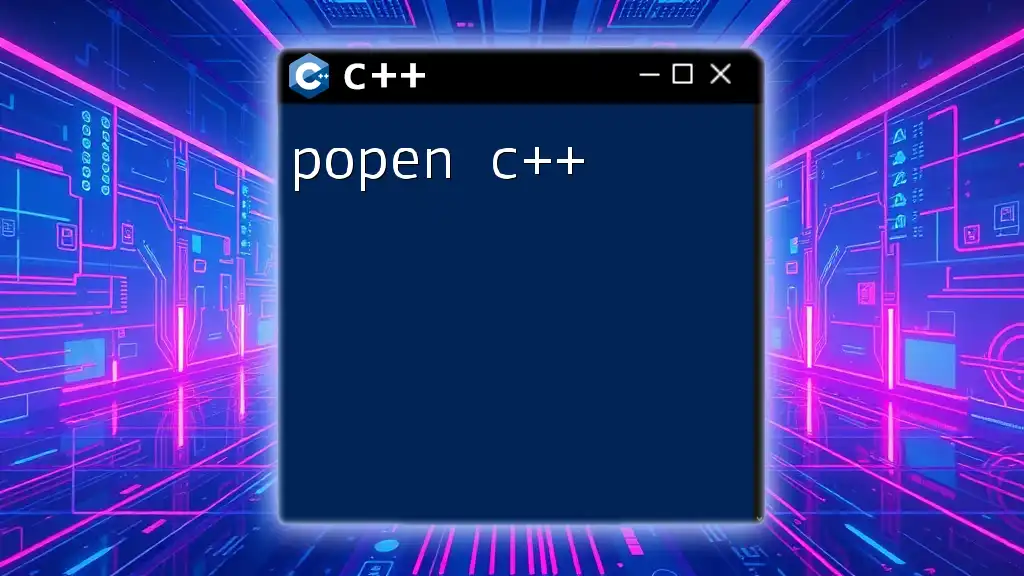
Implementing C++ Properties
Basic Property Example
Let's start with a simple class that demonstrates property implementation via getter and setter methods.
class Person {
private:
std::string name;
public:
const std::string& getName() const { return name; }
void setName(const std::string& newName) { name = newName; }
};
In this example:
- The `name` attribute is private, making it inaccessible from outside of the `Person` class.
- The `getName()` method allows external code to read the value of `name` safely, while the `setName()` method allows changing the value in a controlled manner.
Using Getter and Setter Methods
It's essential to follow best practices when using getters and setters. They should never do heavy computations or modify the internal state unless necessary. Here’s an example of a class implementing validation logic in the setter.
class BankAccount {
private:
double balance;
public:
double getBalance() const { return balance; }
void setBalance(double amount) {
if(amount >= 0) {
balance = amount;
} else {
std::cerr << "Balance cannot be negative!" << std::endl;
}
}
};
This `BankAccount` class:
- Allows users to retrieve the balance but enforces that the balance cannot be set to a negative value.
- Enhances the integrity of the `balance` variable by protecting it from invalid assignments.
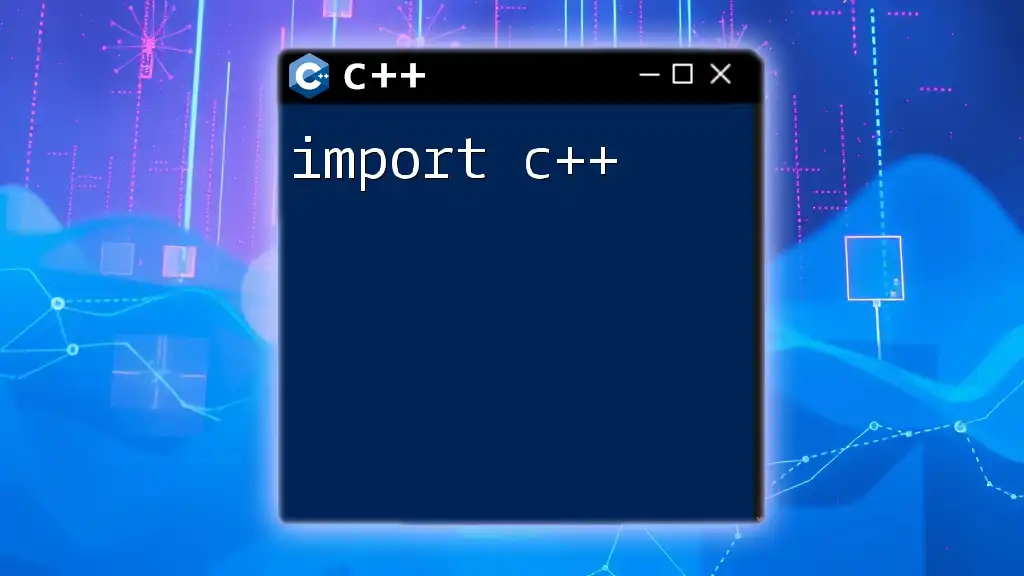
Advanced Property Features in C++
Property Macros
Using macros can significantly streamline property creation when you have many properties in a class. However, it is essential to weigh advantages against potential downsides such as reduced readability and debugging complexity.
#define PROPERTY(type, name) \
private: type name; \
public: \
type get##name() const { return name; } \
void set##name(type value) { name = value; }
By defining a `PROPERTY` macro, we can avoid boilerplate code, making our classes cleaner. Here’s how you could use this macro in a class definition:
class Employee {
PROPERTY(std::string, name)
PROPERTY(double, salary)
};
Leveraging Templates for Properties
Templates can be employed to create generic properties that can handle various data types while maintaining type safety.
template<typename T>
class ValueHolder {
private:
T value;
public:
T getValue() const { return value; }
void setValue(T newValue) { value = newValue; }
};
This `ValueHolder` class can now be reused for any type, providing a robust method for storing and accessing different kinds of values.
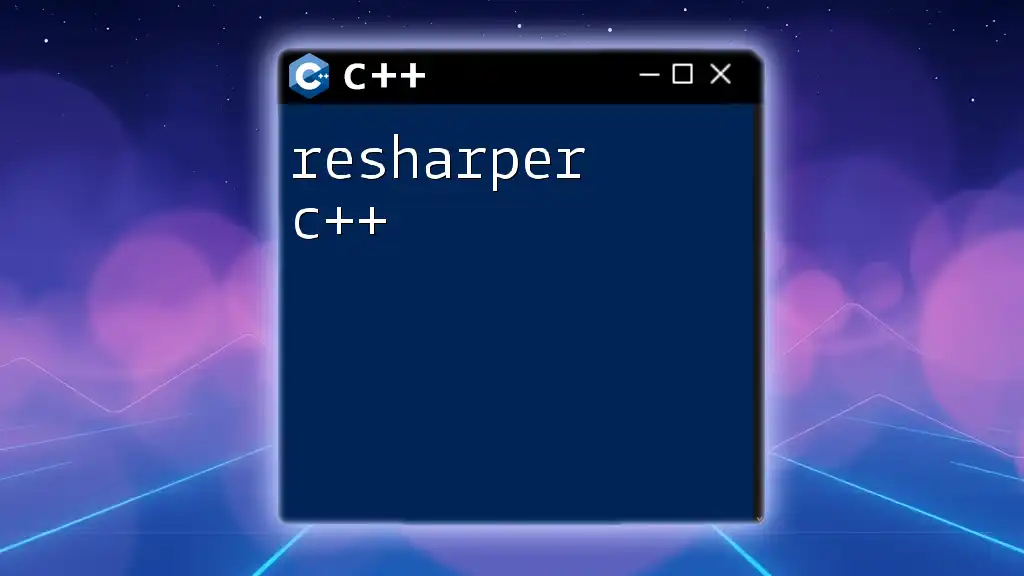
Properties in Modern C++
Introduction to C++20 Concepts
C++20 has introduced several powerful features that can enhance property design, such as concepts. Concepts allow developers to define constraints on template parameters, clarifying the expectations of property types.
Example: Using std::optional for Properties
Using `std::optional` can add a layer of expressiveness and safety to properties. By leveraging this feature, you can easily handle cases where a value might be absent.
#include <optional>
class User {
private:
std::optional<std::string> email;
public:
std::optional<std::string> getEmail() const { return email; }
void setEmail(const std::string& newEmail) {
email = newEmail;
}
};
In this example:
- The `email` property is represented as an `std::optional`, meaning it can either hold a value or indicate an absence of value.
- This allows for safer state handling and reduces the risk of null pointer exceptions.
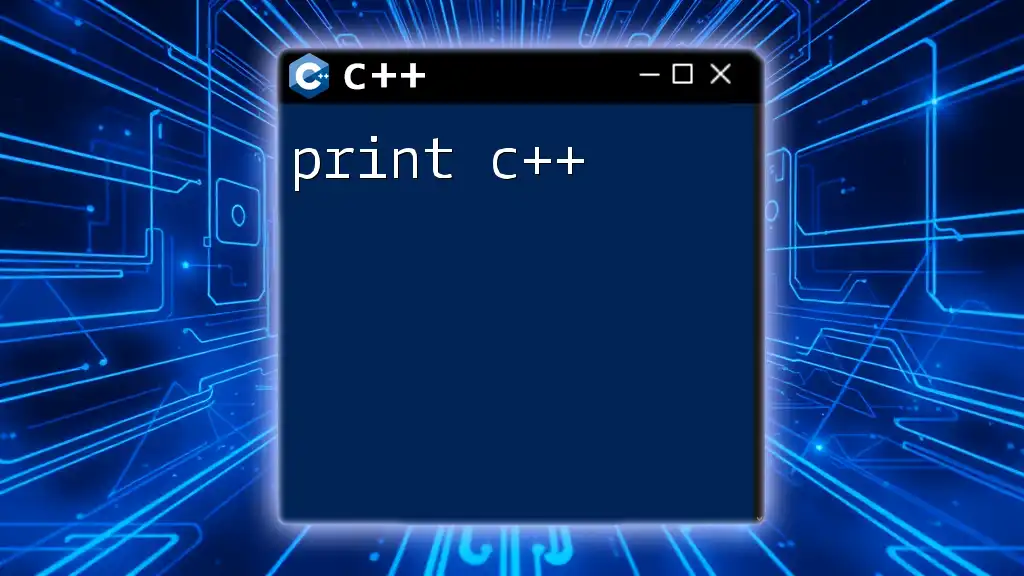
Common Pitfalls in Using Properties
Inefficient Accessors
When designing property accessors, it's crucial to ensure that they aren't performing heavy computations or causing side effects, as this can lead to performance degradation. Always keep the getter methods lightweight.
Mismanagement of State
State management can become tricky when properties interact in complex ways. You may run into potential issues of state inconsistency if setters are not carefully handled. For example, if setting one property inadvertently invalidates another, this requires a combination of checks within each setter.
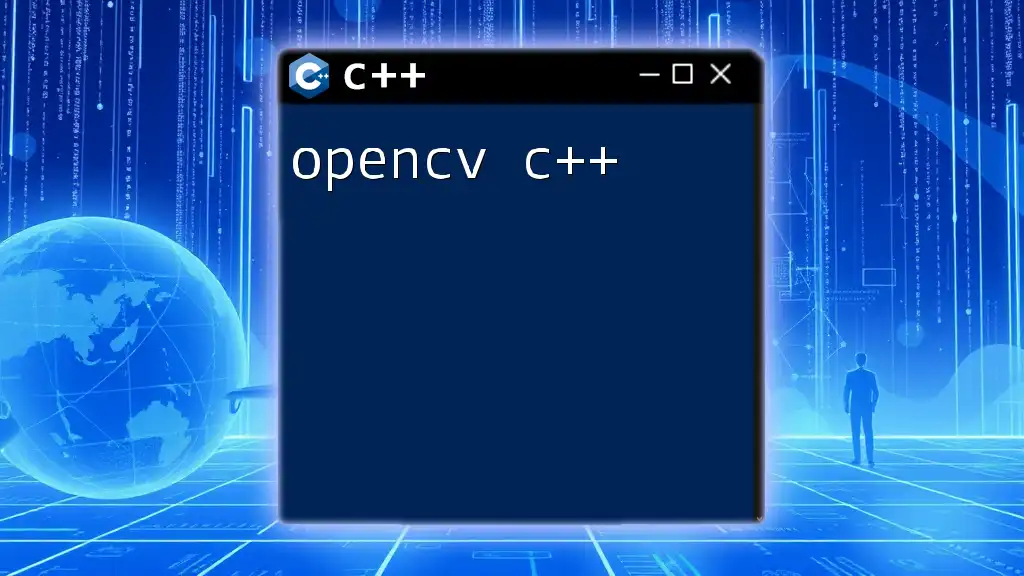
Conclusion
Incorporating properties into C++ programming can enhance data integrity, improve code organization, and allow for greater flexibility in managing class attributes. The examples and concepts presented provide a solid foundation for understanding how to implement properties effectively in your C++ projects.
With these insights, we encourage you to explore and apply properties in your programs, enhancing both the functionality and robustness of your C++ code. Join our learning platform for more concise and effective tutorials to master essential C++ concepts!
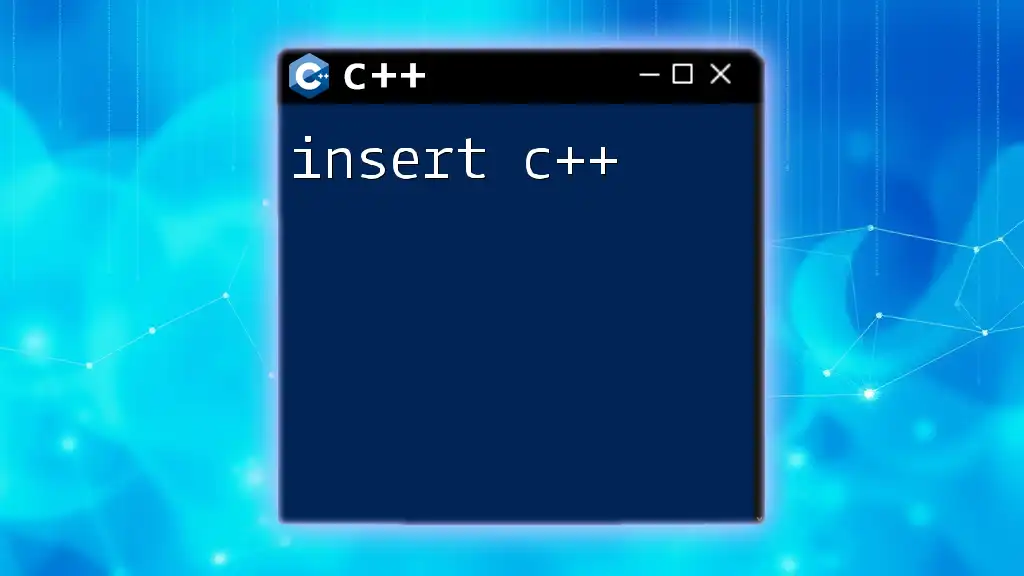
Additional Resources
For further reading and resources, consider consulting official C++ documentation or exploring advanced C++ programming literature to deepen your understanding of properties and their applications.