The "copy" operation in C++ typically refers to duplicating the contents of one variable to another, which can be done using assignment operators or by utilizing standard library functions like `std::copy`.
Here's a simple code snippet demonstrating variable copying using assignment:
#include <iostream>
int main() {
int original = 42; // Original variable
int copy = original; // Copying the value
std::cout << "Copy: " << copy << std::endl;
return 0;
}
Understanding the `copy` Function
What is the `copy` Function?
The `copy` function in C++ is a powerful tool provided by the Standard Template Library (STL) that allows developers to duplicate elements from one range to another. It plays a crucial role in data manipulation, facilitating the movement of elements between disparate containers or arrays while ensuring the integrity of data.
Where is `copy` Used?
Common scenarios for using `copy` include:
- Transferring Data: Moving data between various container types (e.g., from vectors to arrays).
- Modifying Copies: Maintaining a backup of original data while modifying a duplicate.
- Performance Optimization: Improving efficiency by avoiding manual element-by-element copying.
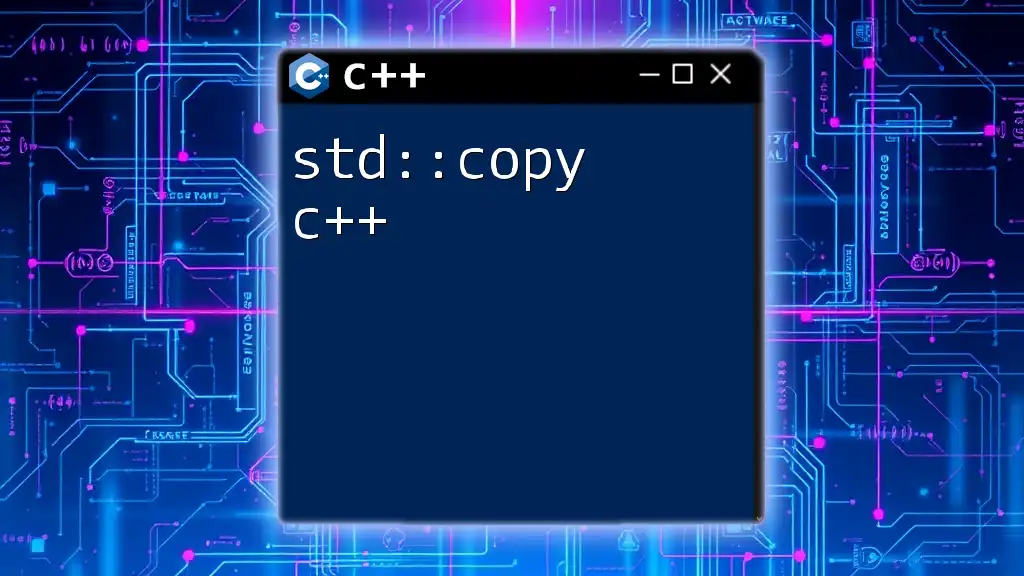
Setting Up the Environment
Requirements
To utilize the `copy` function effectively, make sure your development environment meets the following:
- C++11 or later: This is essential for full compatibility with the STL.
- Necessary Headers: Don’t forget to include the required header `<algorithm>` for access to the `copy` algorithm.
Sample Code Setup
To get started, you can set up a simple C++ program template:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
// Code will be added here
}
This will provide a foundation upon which you can build various examples.
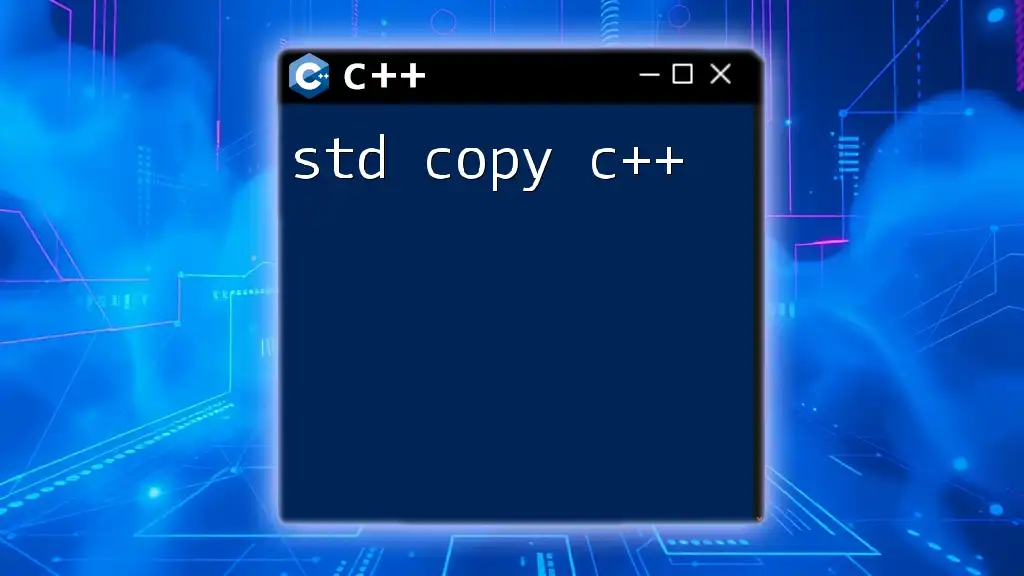
Syntax of the `copy` Function
General Syntax
Understanding the syntax of the `copy` function is critical. It is structured as follows:
std::copy(InputIterator first, InputIterator last, OutputIterator result);
Each component has a specific purpose and understanding these will help you use `copy` effectively.
Parameters Description
- InputIterator: This refers to the starting position of the source range you wish to copy.
- OutputIterator: This indicates where the copied elements should be stored in the destination. This must point to a valid location.
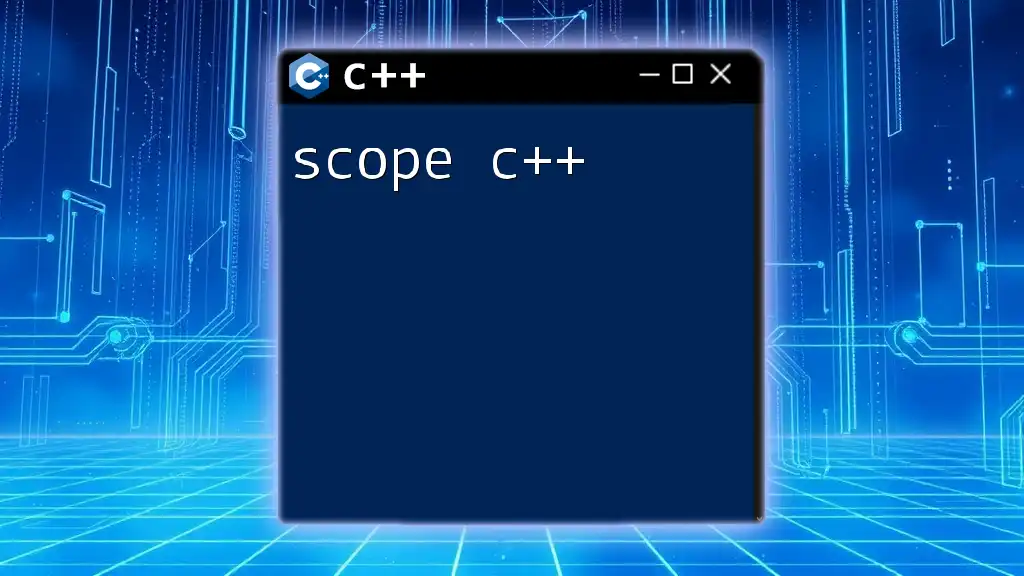
Practical Examples of Using `copy`
Copying Elements from One Array to Another
One of the simplest use cases for `copy` is transferring elements from one array to another. Consider this example:
int main() {
int source[] = {1, 2, 3, 4, 5};
int destination[5];
std::copy(source, source + 5, destination);
// Output the destination array
for (int i : destination) {
std::cout << i << " ";
}
return 0;
}
Here, the function takes the range defined by `source` (from index 0 to 5) and duplicates these elements into `destination`. After running this code, the output will be `1 2 3 4 5`, showcasing how effectively the `copy` function can duplicate data.
Copying Elements from a Vector to a List
Another example is the process of copying data from a vector to a list, which highlights the versatility of the `copy` function:
#include <iostream>
#include <vector>
#include <list>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::list<int> lst;
std::copy(vec.begin(), vec.end(), std::back_inserter(lst));
// Output the list
for (int i : lst) {
std::cout << i << " ";
}
return 0;
}
In this case, `std::back_inserter` allows the program to append elements to the destination list as they are copied, making it a robust solution for dynamic container types. The output will replicate the contents of the vector, affirming the effectiveness of the copy operation.
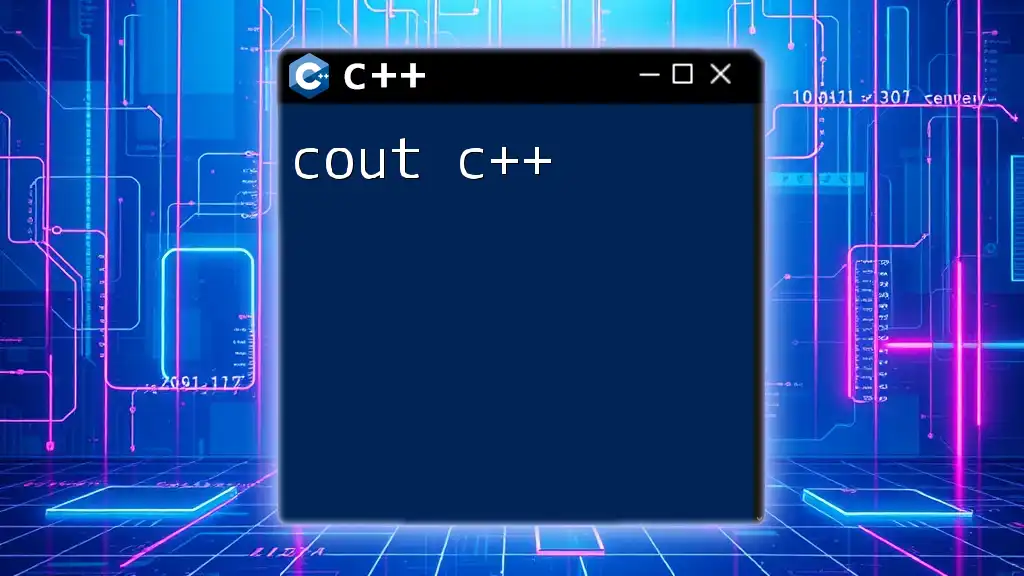
Handling Edge Cases
Copying when the Ranges Overlap
Occasionally, you may face scenarios where the ranges from which you are copying and to which you are copying overlap. Understanding how to handle this case is critical to data integrity. For example:
char str[] = "Hello World";
std::copy(str, str + 5, str + 6); // Copying "Hello" into " World"
In this instance, the first five characters from `str` are copied to a position within the same string. It’s essential to be cautious with overlapping ranges to avoid unexpected behavior. When copying overlapping data, consider using `std::copy_backward` to maintain the order without corruption.
Using `std::copy` with Different Data Types
The versatility of `copy` extends beyond basic data types. You can use it to copy user-defined types as well. Consider this example:
struct Point {
int x, y;
};
Point arr1[] = {{1, 2}, {3, 4}};
Point arr2[2];
std::copy(arr1, arr1 + 2, arr2);
This demonstrates how structures can be effectively handled by `copy`, given that the types involved are copyable. It’s crucial to ensure that the types being copied have defined copy operations (like copy constructors) for smooth execution.
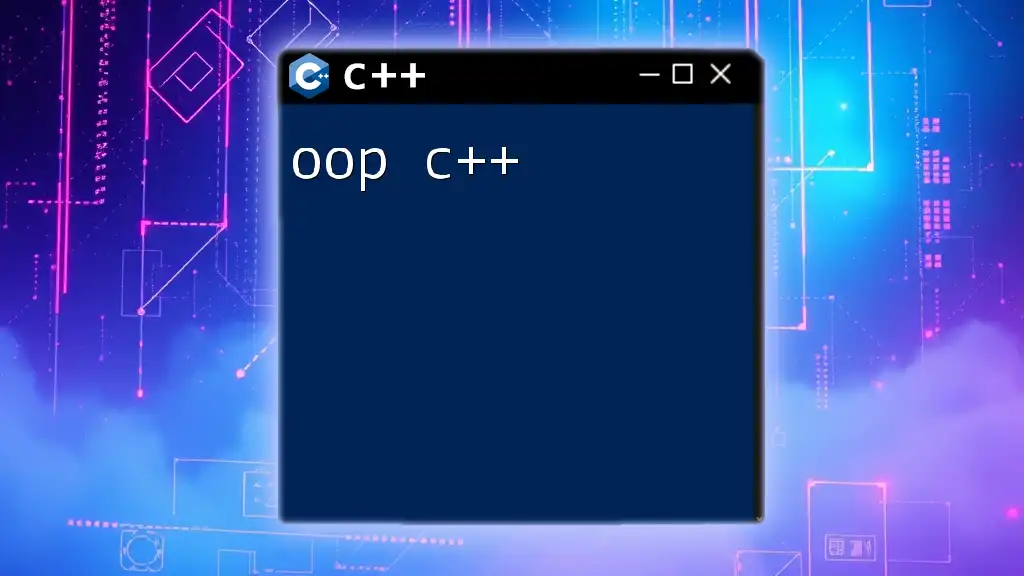
Common Mistakes and Troubleshooting
Not Using the Correct Iterator
One prevalent mistake is neglecting the need for correct iterator usage. If you pass invalid iterators, you will likely encounter runtime errors or undefined behavior. Ensure iterators point to valid ranges every time.
Copying Incompatible Types
C++ enforces strong type checking. Thus, copying incompatible types will lead to compilation errors. For instance, attempting to copy a `std::unique_ptr` to another will invoke an error since it is non-copyable. Therefore, it’s essential to understand the copyability of the types involved.
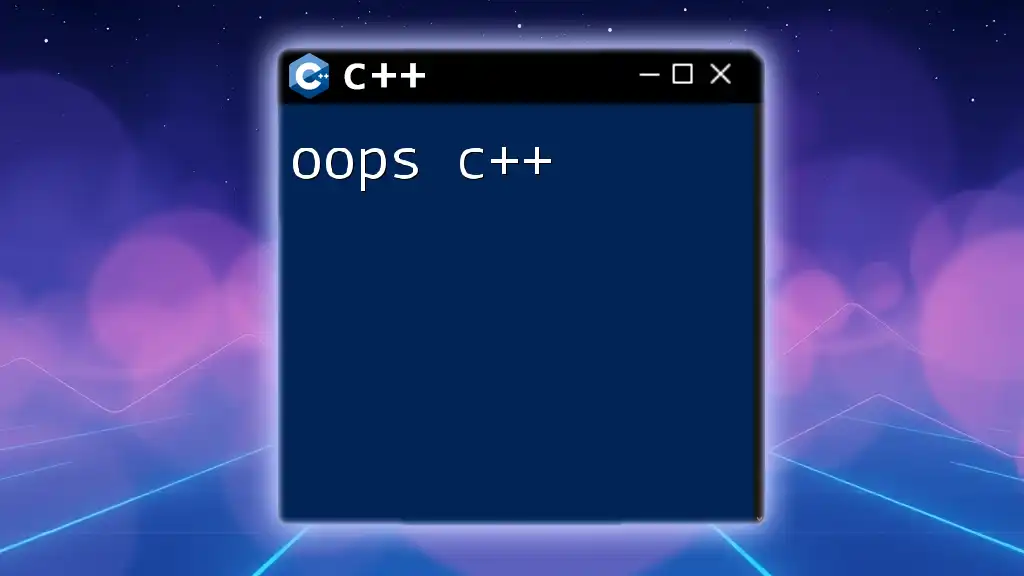
Conclusion
In summary, the `copy` function in C++ is an essential utility for efficient data handling. By understanding its syntax, practical applications, and how to manage edge cases, you can leverage its capabilities to improve your C++ programming. Always remember to implement best practices, and don't hesitate to experiment with `copy` in diverse contexts to deepen your understanding and proficiency in C++.